Unchecked exceptions in Java, also known as runtime exceptions, are exceptions that are not required to be caught or declared in a method’s throws clause. These exceptions are typically caused by programming errors or unexpected conditions and often represent scenarios that are recoverable with proper coding practices.
List of Unchecked Exception in Java
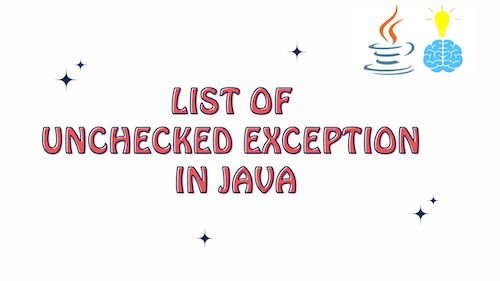
Here is a list of some common unchecked exception in Java:
- ArithmeticException
- ArrayIndexOutOfBoundsException
- NullPointerException
- NumberFormatException
- ConcurrentModificationException
- IllegalArgumentException
- IllegalStateException
- ClassCastException
ArithmeticException
Thrown when an exceptional arithmetic condition has occurred. For example, an integer “divide by zero” throws an instance of this class.


ArrayIndexOutOfBoundsException
Thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.


NullPointerException
Thrown when an application attempts to use null in a case where an object is required.


NumberFormatException
Thrown to indicate that the application has attempted to convert a string to one of the numeric types, but that the string does not have the appropriate format.


ConcurrentModificationException
This exception may be thrown by methods that have detected concurrent modification of an object when such modification is not permissible.
For example, it is not generally permissible for one thread to modify a Collection while another thread is iterating over it.


IllegalArgumentException
Thrown to indicate that a method has been passed an illegal or inappropriate argument.


IllegalStateException
Signals that a method has been invoked at an illegal or inappropriate time. In other words, the Java environment or Java application is not in an appropriate state for the requested operation.


ClassCastException
Thrown when an attempt is made to cast an object to an incompatible class.
public class ClassCastExceptionExample {
public static void main(String[] args) {
try {
// Creating an Integer object
Object obj = new Integer(10);
// Attempting to cast Integer to String
String str = (String) obj; // This line will throw ClassCastException
} catch (ClassCastException e) {
System.out.println("ClassCastException caught: " + e.getMessage());
}
}
}
Output
ClassCastException caught: java.lang.Integer cannot be cast to java.lang.String
Conclusion : List of Unchecked Exception in Java
In this article, we explored the list of Unchecked Exception in Java in great details. Hopefully, this will enable developers to write Java Code effectively keeping in mind these unchecked exceptions.
Read More : List of Checked Exception in Java