Arrays in Java are essential data structures that allow developers to store and manipulate collections of elements efficiently. They provide a convenient way to work with multiple values of the same type. This comprehensive guide will explore Java arrays, covering their basics, operations, multi-dimensional arrays, and best practices. Whether you are a beginner or an experienced Java developer, understanding arrays in Java is crucial for building robust and efficient applications.
Introduction to Arrays in Java
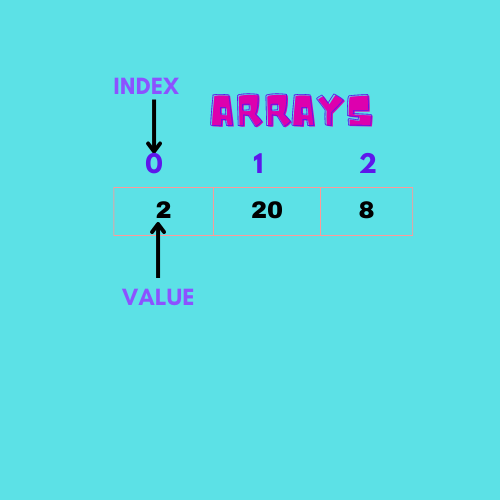
Arrays in Java are contiguous blocks of memory that store fixed-size sequences of elements of the same type. They offer an efficient way to store and access multiple values under a single variable name. Java arrays are accessed using index. The index is an integer value that ranges from 0 to the length of the array minus one. For an array of size N, valid indexes are from 0 to N-1. Attempting to access an index outside this range will result in an “IndexOutOfBoundsException.”
Declaring and Initializing Arrays
In Java, arrays are declared using square brackets [] after the element type. They can be initialized with values during declaration or later using the new keyword. Here’s an example:
// Declaration and initialization
int[] numbers = {1, 2, 3, 4, 5};
// Declaration followed by initialization
String[] names;
names = new String[]{"John", "Jane", "Alice"};
Accessing and Modifying Array Elements
Array elements are accessed and modified using their respective indexes. Indexing starts at 0 and goes up to array.length - 1
.
Here’s an example:
int[] numbers = {10, 20, 30, 40, 50};
System.out.println(numbers[0]); // Output: 10
numbers[2] = 35;
System.out.println(numbers[2]); // Output: 35
Array Length and Bounds Checking
The array.length
property provides the length or size of an array. It is useful for iterating over arrays and performing bounds checking to avoid accessing elements beyond the array’s bounds. Here’s an example:
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
// Bounds checking
if (index >= 0 && index < numbers.length) {
System.out.println(numbers[index]);
} else {
System.out.println("Invalid index!");
}
Iterating Over Arrays
Java provides various ways to iterate over arrays, including the traditional for
loop, enhanced for-each
loop, and Arrays.stream()
with lambda expressions. Here are examples:
int[] numbers = {1, 2, 3, 4, 5};
// Traditional for loop
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
// Enhanced for-each loop
for (int num : numbers) {
System.out.println(num);
}
// Using Streams and Lambda Expressions
Arrays.stream(numbers).forEach(System.out::println);
Searching and Sorting Arrays in Java
Java provides methods from the java.util.Arrays
class to search for specific elements or sort arrays. Here are examples:
int[] numbers = {5, 2, 8, 4, 1};
// Searching for an element
int index = Arrays.binarySearch(numbers, 8);
System.out.println("Index of 8: " + index); // Output: Index of 8: 2
// Sorting the array
Arrays.sort(numbers);
System.out.println("Sorted Array: " + Arrays.toString(numbers)); // Output: Sorted Array: [1, 2, 4, 5, 8]
Types of Arrays in Java
We have the following two types of Arrays in Java
- Two-Dimensional Arrays
- Multi-Dimensional Arrays
Two-Dimensional Arrays
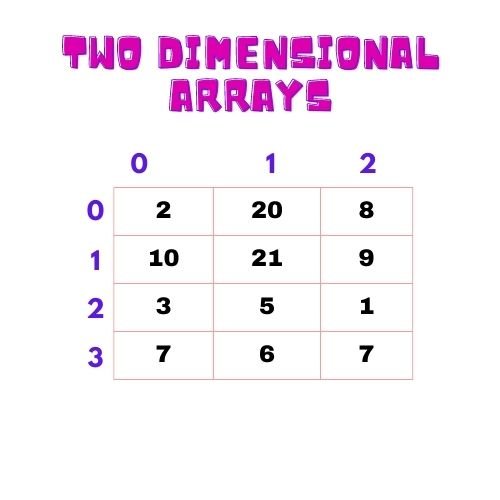
Two-dimensional arrays are arrays of arrays, forming a matrix-like structure. They provide a way to represent tabular data and grids. Here’s an example:
int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
System.out.println(matrix[0][1]); // Output: 2
matrix[1][2] = 10;
System.out.println(matrix[1][2]); // Output: 10
Multi-Dimensional Arrays
Java supports multi-dimensional arrays beyond two dimensions. They are created using nested arrays. Here’s an example of a three-dimensional array:
int[][][] cube = {
{{1, 2}, {3, 4}},
{{5, 6}, {7, 8}}
};
System.out.println(cube[0][1][0]); // Output: 3
cube[1][0][1] = 9;
System.out.println(cube[1][0][1]); // Output: 9
Common Operations on Multi-Dimensional Arrays
Working with multi-dimensional arrays involves operations such as accessing elements, iterating over dimensions, and performing calculations. Here’s an example:
int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
// Accessing elements
System.out.println(matrix[1][2]); // Output: 6
// Iterating over rows and columns
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
Best Practices for Working with Arrays in Java
When working with arrays in Java, consider the following best practices:
- Use meaningful variable names to enhance code readability.
- Initialize arrays with appropriate sizes to avoid resizing and unnecessary memory allocation.
- Perform bounds checking to prevent index out-of-bounds errors.
- Be mindful of the time and space complexity of array operations.
- Consider using the enhanced for-each loop when iterating over arrays.
- Utilize the utility methods provided by the
java.util.Arrays
class for common operations. - Avoid direct modification of array lengths to maintain data integrity.
- Document your code to provide clarity on the purpose and usage of arrays.
FAQs about Arrays in Java
Here are some frequently asked questions about arrays in Java:
Q1. Can arrays in Java store elements of different types?
No, arrays in Java can only store elements of the same type. If you need to store elements of different types, consider using collections such as ArrayList or creating an array of objects.
Q2. What happens if we try to access an array element with an index beyond its bounds?
Accessing an array element beyond its bounds will result in an ArrayIndexOutOfBoundsException
. It is important to perform bounds checking to avoid such errors.
Q3. Can we change the size of an array after it is created?
No, the size of an array in Java is fixed upon creation and cannot be changed. If you need a dynamically resizable collection, consider using ArrayList or other data structures.
Q4. Can we have an array with no elements in Java?
Yes, it is possible to create an array with zero elements. This is often referred to as an empty array.
Q5. Can we sort arrays containing objects of custom classes?
Yes, you can sort arrays containing objects of custom classes by implementing the Comparable
interface or by providing a custom Comparator
implementation.
Q6. What is the difference between arrays and ArrayList in Java?
Arrays have a fixed size and provide direct access to elements using indexes, while ArrayLists are dynamically resizable and offer additional methods for manipulating and accessing elements.
Q7. Can we have arrays of multi-dimensional arrays in Java?
Yes, it is possible to have arrays of multi-dimensional arrays in Java, allowing for complex data structures and representations.
Q8. Can we create an array with a non-positive size?
No, the size of an array must be a positive integer. Attempting to create an array with a non-positive size will result in a NegativeArraySizeException
.
Q9. How do we determine the length of a multi-dimensional array in Java?
The length of a multi-dimensional array can be determined using the array.length
property for each dimension. For example, array.length
gives the length of the first dimension, and array[i].length
gives the length of the second dimension.
Q10. Are arrays in Java dynamically allocated or statically allocated?
Arrays in Java are dynamically allocated, meaning memory is allocated for them at runtime, based on their size and type.
Conclusion
Arrays are fundamental data structures in Java that enable efficient storage and manipulation of multiple elements. This comprehensive guide has covered the basics of arrays, including their declaration, initialization, accessing elements, and common operations. Additionally, it explored multi-dimensional arrays and provided best practices for working with arrays effectively. By understanding arrays in Java, you can enhance your programming skills and build more efficient and reliable applications.
Remember to leverage the flexibility and power of arrays in Java while considering best practices and optimizing your code for readability and performance. Happy coding with Java arrays!