In this article, we will have a detailed discussion on various aspects of “Triggers in SQL”. Let’s get started.
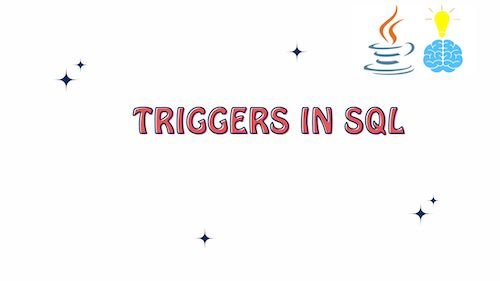
What are Triggers in SQL ?
Triggers in SQL are database objects that are automatically executed in response to specific events or actions that occur within a database. These triggers are defined in the database and associated with a particular table, and they can be set to activate before or after events such as INSERT, UPDATE, DELETE, or other database operations. Triggers are often used to enforce data integrity, automate tasks, or log changes in a database.
Types of Triggers in SQL
There are two main types of SQL triggers:
- Before Triggers (or FOR EACH ROW Triggers): These triggers are fired before a specific event (e.g., INSERT, UPDATE, DELETE) occurs on a row in a table. They are often used to validate data or modify it before it is written to the table. Before triggers are executed for each row affected by the triggering event.
- After Triggers (or AFTER Triggers): These triggers are executed after a specific event (e.g., INSERT, UPDATE, DELETE) occurs on a table. They are commonly used for tasks such as logging changes, sending notifications, or performing additional database operations based on the event that triggered them.
Syntax of a Trigger in SQL
The syntax for creating triggers in SQL varies slightly depending on the database management system (DBMS) you are using, as different DBMSs may have their own syntax extensions.
Here’s the general syntax for creating triggers in SQL:
CREATE [OR REPLACE] TRIGGER trigger_name
{BEFORE | AFTER | INSTEAD OF} {INSERT | UPDATE | DELETE}
ON table_name
[FOR EACH ROW]
[WHEN (condition)]
BEGIN
-- Trigger body (SQL statements or procedures)
END;
Let’s break down the elements of this syntax:
CREATE TRIGGER
: This statement is used to create a new trigger.OR REPLACE
(optional): Some database systems allow you to useOR REPLACE
to modify an existing trigger with the same name.trigger_name
: This is the name you assign to your trigger. It should be unique within the schema.BEFORE | AFTER | INSTEAD OF
: Specifies when the trigger should be fired.BEFORE
triggers are executed before the event (e.g., INSERT, UPDATE, DELETE).AFTER
triggers are executed after the event.INSTEAD OF
triggers are used in certain DBMSs like SQL Server for views, and they replace the default action of the triggering event.
INSERT | UPDATE | DELETE
: Specifies the type of event that triggers the trigger.ON table_name
: Specifies the name of the table associated with the trigger.FOR EACH ROW
(optional): This clause is used in row-level triggers. It indicates that the trigger will execute once for each row affected by the triggering event.WHEN (condition)
(optional): You can use this clause to specify a condition that must be true for the trigger to fire. If the condition evaluates to false, the trigger won’t execute.BEGIN
andEND
: These keywords enclose the trigger body, which consists of SQL statements or procedures to be executed when the trigger is fired.
Examples of After Trigger in SQL
Let’s take an example of creating a trigger that captures insert, update, and delete operations on an “employees” table. This trigger logs these operations into an “audit_log” table, which stores information about the action, the affected employee’s ID, and a timestamp.
Create Statement of employees Table
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
job_title VARCHAR(100),
salary DECIMAL(10, 2),
hire_date DATE
);
Create Statement of audit_log Table
CREATE TABLE audit_log (
log_id INT PRIMARY KEY AUTO_INCREMENT,
action VARCHAR(10) NOT NULL,
employee_id INT,
timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Create an AFTER INSERT Trigger(Mysql 8.1.0 version Syntax Used here)
DELIMITER //
CREATE TRIGGER employees_insert_trigger
AFTER INSERT ON employees
FOR EACH ROW
BEGIN
-- Insert operation
INSERT INTO audit_log (action, employee_id, timestamp)
VALUES ('INSERT', NEW.employee_id, NOW());
END;
//
DELIMITER ;
Now, let’s insert a record into employees table
-- Insert a new employee record
INSERT INTO employees (employee_id, first_name, last_name, job_title, salary, hire_date)
VALUES (1, 'John', 'Doe', 'Software Engineer', 75000.00, '2023-09-11');
After this INSERT operation, the “audit_log” will show the below records:
SELECT * FROM audit_log;
+--------+--------+-------------+---------------------+
| log_id | action | employee_id | timestamp |
+--------+--------+-------------+---------------------+
| 1 | INSERT | 1 | 2023-09-11 00:00:00 |
+--------+--------+-------------+---------------------+
Create an AFTER UPDATE Trigger(Mysql 8.1.0 version Syntax Used here)
DELIMITER //
CREATE TRIGGER employees_update_trigger
AFTER UPDATE ON employees
FOR EACH ROW
BEGIN
-- Update operation
INSERT INTO audit_log (action, employee_id, timestamp)
VALUES ('UPDATE', OLD.employee_id, NOW());
END;
//
DELIMITER ;
Now, let’s update a record in employees table
UPDATE employees
SET salary = 80000.00
WHERE employee_id = 1;
After this Update operation, the “audit_log” will show the below records:
SELECT * FROM audit_log;
+--------+--------+-------------+---------------------+
| log_id | action | employee_id | timestamp |
+--------+--------+-------------+---------------------+
| 1 | INSERT | 1 | 2023-09-11 00:00:00 |
| 2 | UPDATE | 1 | 2023-09-11 12:34:56 |
+--------+--------+-------------+---------------------+
Create an AFTER DELETE Trigger(Mysql 8.1.0 version Syntax Used here)
DELIMITER //
CREATE TRIGGER employees_delete_trigger
AFTER DELETE ON employees
FOR EACH ROW
BEGIN
-- Delete operation
INSERT INTO audit_log (action, employee_id, timestamp)
VALUES ('DELETE', OLD.employee_id, NOW());
END;
//
DELIMITER ;
Let’s delete a record in employees table
DELETE FROM employees
WHERE employee_id = 1;
After this Delete operation, the “audit_log” will show the below records:
SELECT * FROM audit_log;
+--------+--------+-------------+---------------------+
| log_id | action | employee_id | timestamp |
+--------+--------+-------------+---------------------+
| 1 | INSERT | 1 | 2023-09-11 00:00:00 |
| 2 | UPDATE | 1 | 2023-09-11 12:34:56 |
| 3 | DELETE | 1 | 2023-09-11 23:45:00 |
+--------+--------+-------------+---------------------+
Before Trigger Example
Here’s an example of a BEFORE INSERT trigger in SQL. This trigger captures insert operations on an “employees” table and modifies the data before it is actually inserted into the table.
-- Create a BEFORE INSERT trigger
DELIMITER //
CREATE TRIGGER employees_before_insert_trigger
BEFORE INSERT ON employees
FOR EACH ROW
BEGIN
-- Modify the data before insertion (for example, capitalize the first letter of the first_name)
SET NEW.first_name = CONCAT(UCASE(LEFT(NEW.first_name, 1)), LCASE(SUBSTRING(NEW.first_name, 2)));
END;
//
DELIMITER ;
In this example:
- We create a BEFORE INSERT trigger named
employees_before_insert_trigger
. - The trigger is associated with the “employees” table and is set to activate before an INSERT operation on that table.
FOR EACH ROW
indicates that this is a row-level trigger, and it will be executed for each row affected by the INSERT operation.- Inside the trigger, we modify the data before insertion. In this case, we capitalize the first letter of the
first_name
column using SQL string functions.
Now, when you insert a new record into the “employees” table, the trigger will automatically capitalize the first letter of the first_name
before the data is inserted.
Here’s an example of how to insert a new employee record:
-- Insert a new employee record
INSERT INTO employees (employee_id, first_name, last_name, job_title, salary, hire_date)
VALUES (1, 'john', 'Doe', 'Software Engineer', 75000.00, '2023-09-11');
After executing this INSERT operation, you can query the “employees” table to see the modified data:
SELECT * FROM employees;
The result should show that the first_name
has been capitalized as a result of the BEFORE INSERT trigger:
+-------------+------------+-----------+------------+--------+------------+
| employee_id | first_name | last_name | job_title | salary | hire_date |
+-------------+------------+-----------+------------+--------+------------+
| 1 | John | Doe | Software Engineer | 75000.00 | 2023-09-11 |
+-------------+------------+-----------+------------+--------+------------+
Best Practices for using Triggers in SQL
Using triggers in SQL can be powerful, but it should be done with care to ensure they don’t negatively impact database performance or lead to unexpected behaviors. Here are some best practices for using triggers in SQL:
- Use Triggers Sparingly: Triggers introduce complexity into your database schema and can make it harder to maintain and troubleshoot. Only use triggers when necessary to enforce data integrity, automate tasks, or log changes.
- Document Triggers: Clearly document the purpose, behavior, and conditions of each trigger. This will help other developers understand and maintain the database.
- Keep Triggers Simple: Triggers should be concise and focused on a specific task. Avoid long and complex trigger logic that is hard to understand or debug.
- Be Mindful of Performance: Triggers can impact database performance, especially if they involve complex operations or modify large datasets. Test the performance of your triggers and optimize them as needed.
- Avoid Endless Loops: Be careful when modifying the same table that triggered the trigger. Recursive triggers can lead to infinite loops. Some database systems provide mechanisms to prevent this.
- Testing and Validation: Thoroughly test triggers in a controlled environment before deploying them in a production database. Consider edge cases and error scenarios.
- Security: Ensure that only authorized users have permissions to create, modify, or delete triggers. Triggers should not introduce security vulnerabilities.
- Version Control: Include trigger definitions in your version control system alongside your database schema. This helps track changes and facilitates collaboration.
Conclusion: Triggers in SQL
This article provided a comprehensive overview of “Triggers in SQL.” It began by defining what triggers are in the context of a relational database and explained their purpose, which includes enforcing data integrity, automating tasks, and logging changes. The article discussed the two main types of SQL triggers: Before Triggers and After Triggers, each with its distinct use cases.
Furthermore, the article provided the general syntax for creating triggers in SQL, highlighting key elements such as trigger timing, event type, table association, and trigger body. Examples of trigger creation for INSERT, UPDATE, and DELETE operations were presented, along with the resulting log entries in an “audit_log” table.
To ensure best practices included using triggers sparingly, documenting triggers thoroughly, keeping trigger logic simple, and being mindful of performance implications. It emphasized the importance of testing and validation, security considerations, version control, and clear documentation.
Related Articles :