In this article, we will do an in-depth exploration of Understanding the Chain of Responsibility Design Pattern in Java Design Pattern in Java. So, let’s get started.
What is Chain of Responsibility Design Pattern in Java?
Chain of Responsibility design pattern in Java is used to decouple the sender of the request from the receiver of the request.

Benefits of Using Chain of Responsibility Design pattern in Java
The benefits of using the Chain of Responsibility pattern in Java include:
- Flexibility: Firstly, this pattern allows for dynamic and flexible handling of requests by providing multiple handlers that can be configured and combined in various ways.
- Decoupling: Secondly it promotes loose coupling between the sender of a request and its receivers, allowing them to operate independently and be easily modified or replaced without affecting the rest of the system.
- Extensibility: Additional handlers can be added to the chain without modifying existing code, making it easy to extend the functionality of the system.
- Responsibility separation: Another advantage of the pattern is responsibility separation. Each handler in the chain is responsible for a specific task or condition, promoting a clear separation of concerns and improving code organization.
- Runtime configuration: Furthermore, the chain can be configured at runtime, allowing for dynamic changes in the order or composition of the handlers based on system requirements.
- Reusability: Additionally, the pattern promotes reusability. Handlers can be reused across different chains or scenarios, reducing code duplication and promoting modular design.
- Error handling: Moreover,this pattern provides a systematic approach to handling errors or exceptions by allowing multiple handlers to try and handle a request until a suitable one is found.
- Scalability: Lastly,this pattern can easily accommodate a large number of handlers, making it suitable for systems with complex processing logic or varying levels of responsibility.
Overall, the Chain of Responsibility pattern helps in building more flexible, maintainable, and extensible Java applications by promoting modular design, separation of concerns, and runtime configurability.
Real-World Use Cases:Chain of Responsibility Design pattern in Java
The Chain of Responsibility design pattern in Java is commonly used in various scenarios where a request needs to be processed through a series of handlers. Some of the common use cases include:
- Logging and Error Handling: Firstly, this pattern is often used to handle logging and error handling in Java applications. Each handler in the chain can perform specific logging tasks or handle different types of errors, providing a modular and customizable approach to error management.
- Authentication and Authorization: Furthermore, In security-related applications, the Chain of Responsibility pattern can be used for authentication and authorization processes. Each handler can verify different aspects of user credentials or permissions, allowing for flexible and configurable security checks.
- Request Processing Pipelines: Moreover, this pattern is suitable for building request processing pipelines, where each handler performs a specific task or validation on the incoming request. Examples include parsing and validating input data, applying transformations, and performing business logic.
- Event Handling: This pattern can be used in event-driven systems, where events need to be processed by multiple handlers in a specific order.
- Workflow Management: The pattern can be applied in workflow management systems, where tasks or steps need to be processed sequentially. Each handler in the chain represents a step in the workflow and can perform its specific actions or validations before passing the task to the next handler.
- Request Filtering and Routing: Additionally, we can use this pattern for request filtering and routing in web applications. Each handler can check request parameters, perform URL mapping, or apply filters based on specific criteria to determine the appropriate action or response.
- Resource Management: We can use this pattern for managing resources in a hierarchical manner. Each handler can handle a specific level of resource allocation or deallocation, ensuring proper handling and releasing of resources.
Example: Chain of Responsibility Design pattern in Java
In the below example, we will use this pattern for making different types of Cakes.
Class Diagram
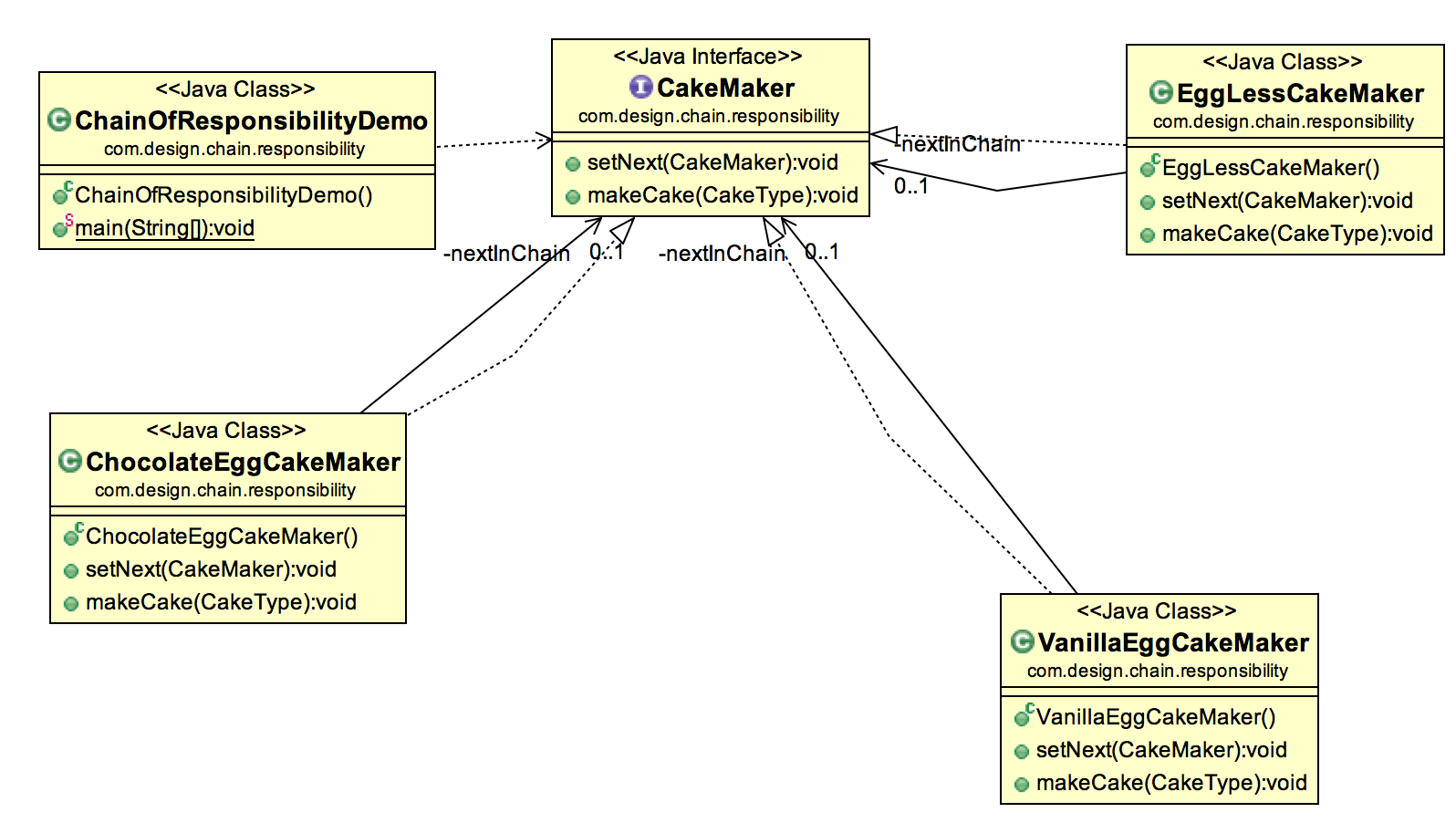
Java Code
// CakeMaker Interface
package com.design.chain.responsibility;
public interface CakeMaker {
public abstract void setNext(CakeMaker nextInChain);
public abstract void makeCake(CakeType cakeType);
}
// CakeType Enum
package com.design.chain.responsibility;
public enum CakeType {
EGGLESS,
EGG_VANILLA,
EGG_CHOLOCOLATE;
}
// EggLessCakeMaker Class
package com.design.chain.responsibility;
public class EggLessCakeMaker implements CakeMaker {
private CakeMaker nextInChain;
@Override
public void setNext(CakeMaker nextInChain) {
this.nextInChain = nextInChain;
}
@Override
public void makeCake(CakeType cakeType) {
if (cakeType == null) {
System.out.println("please set the cake type");
return;
}
if (CakeType.EGGLESS.equals(cakeType)) {
System.out.println("Eggless Cake made");
return;
}
nextInChain.makeCake(cakeType);
}
}
// VanillaEggCakeMaker Class
package com.design.chain.responsibility;
public class VanillaEggCakeMaker implements CakeMaker {
private CakeMaker nextInChain;
@Override
public void setNext(CakeMaker nextInChain) {
this.nextInChain = nextInChain;
}
@Override
public void makeCake(CakeType cakeType) {
if (cakeType == null) {
System.out.println("please set the cake type");
return;
}
if (CakeType.EGG_VANILLA.equals(cakeType)) {
System.out.println("Vanilla Egg Cake made");
return;
}
nextInChain.makeCake(cakeType);
}
}
// ChocolateEggCakeMaker Class
package com.design.chain.responsibility;
public class ChocolateEggCakeMaker implements CakeMaker {
private CakeMaker nextInChain;
@Override
public void setNext(CakeMaker nextInChain) {
this.nextInChain = nextInChain;
}
@Override
public void makeCake(CakeType cakeType) {
if (cakeType == null) {
System.out.println("please set the cake type");
return;
}
if (CakeType.EGG_CHOLOCOLATE.equals(cakeType)) {
System.out.println("Chocolate Egg Cake made");
return;
}
nextInChain.makeCake(cakeType);
}
}
// ChainOfResponsibilityDemo Class
package com.design.chain.responsibility;
public class ChainOfResponsibilityDemo {
public static void main(String[] args) {
CakeMaker eggLessCakeMaker = new EggLessCakeMaker();
CakeMaker vanillaEggCakeMaker = new VanillaEggCakeMaker();
CakeMaker chocolateEggCakeMaker = new ChocolateEggCakeMaker();
eggLessCakeMaker.setNext(chocolateEggCakeMaker);
chocolateEggCakeMaker.setNext(vanillaEggCakeMaker);
eggLessCakeMaker.makeCake(CakeType.EGG_CHOLOCOLATE);
eggLessCakeMaker.makeCake(CakeType.EGG_VANILLA);
eggLessCakeMaker.makeCake(CakeType.EGGLESS);
}
}
// Output
Chocolate Egg Cake made
Vanilla Egg Cake made
Eggless Cake made
For more comprehensive understanding you can go through the book by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, also known as the “Gang of Four” (GoF) Design Patterns: Elements of Reusable Object-Oriented Software
Conclusion: Chain of Responsibility Design pattern in Java
In conclusion, this article delved deep into understanding the Chain of Responsibility Design Pattern in Java. The Chain of Responsibility pattern offers a way to decouple request senders from receivers, enabling flexible and dynamic handling of requests. Its benefits include flexibility, decoupling, extensibility, and runtime configuration. Real-world use cases span from logging and error handling to authentication, request processing pipelines, event handling, and more. By utilizing this pattern, developers can create more modular, maintainable, and extensible Java applications. The provided example of cake making using the Chain of Responsibility pattern demonstrated its practical implementation.
Must Read Design Pattern Articles: