In this article, we will have a detailed discussion on various aspects of Strategy Design Pattern in Java. So, let’s get started.
What is Strategy Design Pattern in Java ?
Strategy Design Pattern in Java allows one of a family of algorithms to be selected on the fly at runtime.Strategy lets the algorithm vary independently from the clients that use it.
Example: Strategy Design Pattern in Java
In the below example, we will use strategy pattern for creating different sorting algorithms and using the appropriate sorting strategy at runtime.
Class Diagram for Our Example
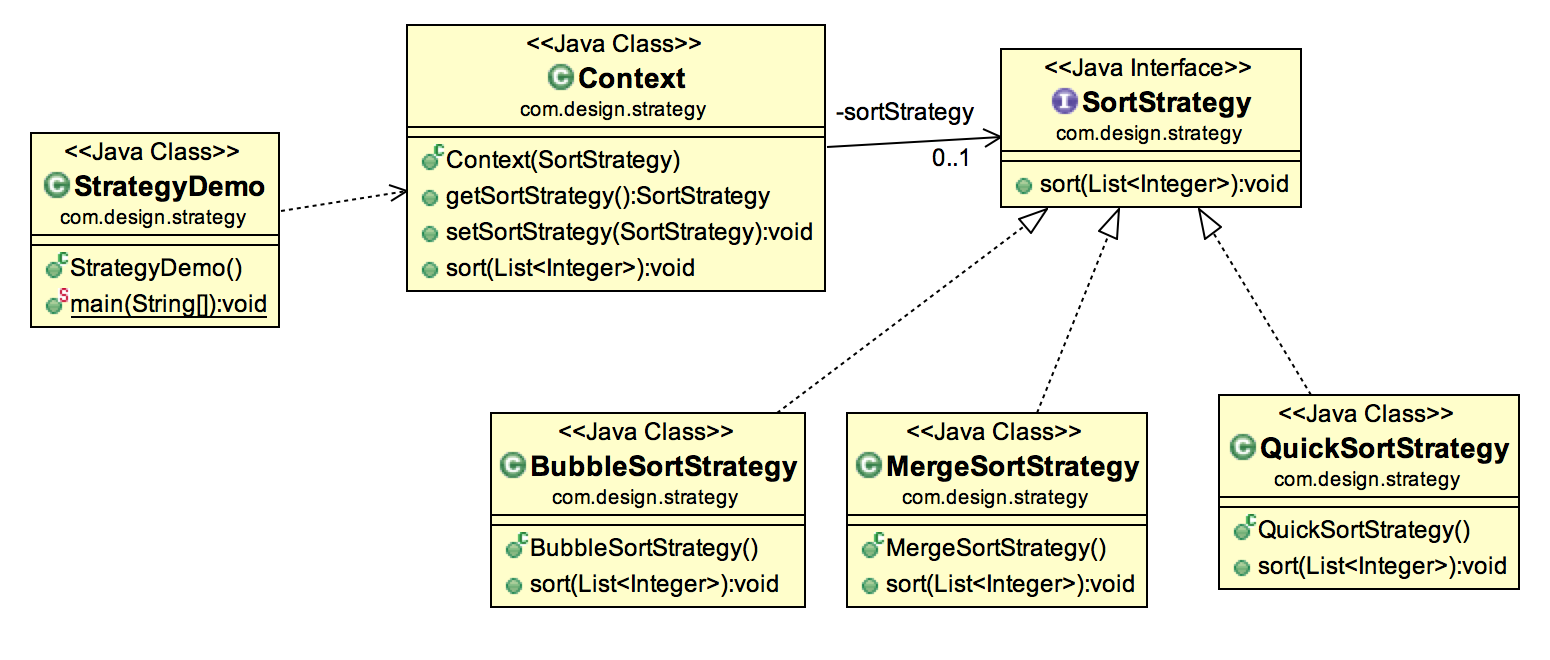
Java Code: Strategy Design Pattern in Java
// SortStrategy Interface
package com.design.strategy;
import java.util.List;
public interface SortStrategy {
public void sort(List numbers);
}
// QuickSortStrategy Class
package com.design.strategy;
import java.util.List;
public class QuickSortStrategy implements SortStrategy {
@Override
public void sort(List numbers) {
System.out.println("Using QuickSort Strategy for Sorting");
}
}
// MergeSortStrategy Class
package com.design.strategy;
import java.util.List;
public class MergeSortStrategy implements SortStrategy {
@Override
public void sort(List numbers) {
System.out.println("Using MergeSort Strategy for Sorting");
}
}
// BubbleSortStrategy Class
package com.design.strategy;
import java.util.List;
public class BubbleSortStrategy implements SortStrategy {
@Override
public void sort(List numbers) {
System.out.println("Using BubbleSort Strategy for Sorting");
}
}
// Context Class
package com.design.strategy;
import java.util.List;
public class Context {
private SortStrategy sortStrategy;
public Context(SortStrategy sortStrategy) {
this.sortStrategy = sortStrategy;
}
public SortStrategy getSortStrategy() {
return sortStrategy;
}
public void setSortStrategy(SortStrategy sortStrategy) {
this.sortStrategy = sortStrategy;
}
public void sort(List numbers) {
sortStrategy.sort(numbers);
}
}
// StrategyDemo Class
package com.design.strategy;
import java.util.Arrays;
import java.util.List;
public class StrategyDemo {
public static void main(String[] args) {
Integer[] numbers = {
3,
8,
10
};
List numberList = Arrays.asList(numbers);
Context context = new Context(new MergeSortStrategy());
context.sort(numberList);
context.setSortStrategy(new BubbleSortStrategy());
context.sort(numberList);
context.setSortStrategy(new QuickSortStrategy());
context.sort(numberList);
}
}
Using MergeSort Strategy
for Sorting
Using BubbleSort Strategy
for Sorting
Using QuickSort Strategy
for Sorting
Real-Life Use-Cases of Strategy Design Pattern in Java
- Sorting Algorithms: Different sorting algorithms (e.g., bubble sort, quick sort) can be implemented as strategies, allowing easy swapping between algorithms without changing the client code.
- Payment Processing: Payment methods (credit card, PayPal, etc.) can be encapsulated as strategies, making it simple to add or modify payment options.
- File Format Converters: Converting files from one format to another (e.g., CSV to JSON) can be achieved using strategy pattern, enabling extensibility for new formats.
- Logging Levels: Implementing various logging levels (debug, info, error) as strategies allows dynamic switching of logging behavior.
Pros of Strategy Design Pattern in Java
- Open/Closed Principle: You can add new strategies without modifying existing client code, promoting code extensibility.
- Single Responsibility Principle: Each strategy encapsulates a specific behavior, making code more modular and maintainable.
- Enhanced Flexibility: Changing behavior at runtime becomes possible, facilitating dynamic adaptation to different situations.
- Code Reusability: Strategies can be reused across different parts of an application, reducing redundant code.
Cons of Strategy Design Pattern in Java
- Increased Complexity: Implementing multiple strategies may introduce additional classes, potentially increasing code complexity.
- Overhead: The strategy pattern can lead to an overhead of additional objects and method calls, which may impact performance for simple cases.
Best Practices:Strategy Design Pattern in Java
- Identify Variations: Identify the varying behaviors in your application that could be encapsulated as strategies.
- Define Strategy Interface: Create an interface or abstract class to define the common methods for all strategies.
- Implement Concrete Strategies: Create concrete classes that implement the strategy interface, each representing a specific behavior.
- Client Context: Implement a context class that contains a reference to the selected strategy and delegates calls to it.
- Dependency Injection: Use constructor or setter injection to inject the desired strategy into the client context.
- Configuration and Initialization: Handle strategy selection and initialization at the appropriate point in your application.
- Avoid Premature Optimization: Use the strategy pattern where varying behaviors are truly required; don’t overcomplicate simple cases.
Conclusion: Strategy Design Pattern in Java
In this extensive article on Strategy Design Pattern in Java, we covered the following:
- Strategy Design Pattern essence.
- Example: Practical sorting algorithms example.
- Real-life use in sorting, payments, format conversion, and logging.
- Pros: Open/Closed, Single Responsibility, flexibility, reusability.
- Cons: Complexity, overhead.
- Best practices: Identify, define, implement, context, injection, judicious use.
Must-Read Design Pattern Articles