Exception handling in Java is a mechanism that allows developers to handle runtime errors, unexpected events, and exceptional situations that can occur during the execution of a program.
Java Exception Hierarchy
It’s essential to have a good understanding of the Exception Hierarchy for proper exception handling in Java.
Let us have a look into the Java Exception Hierarchy.
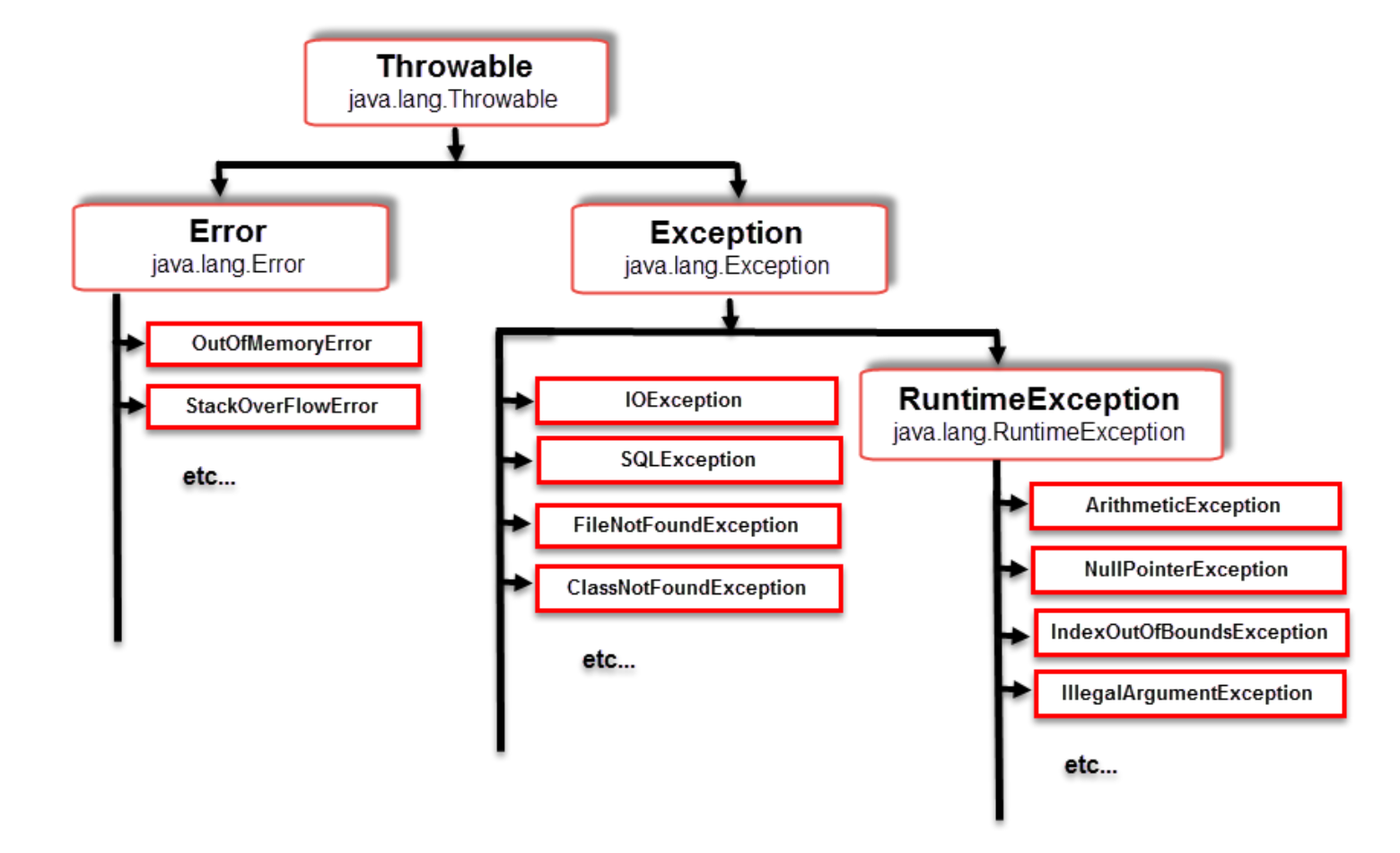
Throwable class
At the top of the Java Exceptions Hierarchy is the Throwable class.This class is the superclass of all errors and exceptions. Only objects that are instances of this class (or one of its subclasses) are thrown by the Java Virtual Machine or can be thrown by the Java throw statement. Similarly, only this class or one of its subclasses can be the argument type in a catch clause.
Exception vs Error
Exception | Error | |
What is it? | Exception is something which can be handled in the program.Exceptions represent logical errors in the application code. | Errors are thrown by JVM.It can’t be handled and hence when it occurs the program gets terminated. |
Can we recover? | We can handle it by using try and catch block or by throwing back the exception to the calling class. | Error is thrown by JVM in a scenario which is fatal and there is no way for the application to recover from that error. For example: OutOfMemoryError. |
Checked or Unchecked? | Exceptions can be either Checked or Unchecked. | Errors by default are unchecked.Compiler don’t check if they are handled in code. |
Checked vs Unchecked Exception
Checked | Unchecked | |
What is it? | These are checked at compile time. If some code within a method can throw a checked exception, then the method must either handle the exception or it must specify the exception using throws keyword. | These are not checked at compiled time |
What may cause the occurrence of Exception? | CheckedException represent scenario with higher failure rate .Checked exceptions are mostly related to resources(files/db/stream/socket etc). | These exceptions occurs because of bad programming.Unchecked exceptions are purely programmatic errors, wrong calculation, null data or even failures in business logic can lead to runtime exceptions. |
Which classes? | All classes that inherit from class Exception but not RuntimeException are considered to be checked exceptions. | RuntimeException and it’s subclasses are unchecked exception.
Classes that inherit from class Error are considered to be unchecked. |
Examples | IOException SQLException DataAccessException ClassNotFoundException InvocationTargetException | NullPointerException ArrayIndexOutOfBound IllegalArgumentException IllegalStateException. |
Should we catch and handle it? | The motive of checked exception is that at compile time if the resources are not available the application should define an alternative behaviour to handle this in the catch/finally block or throws back the exception to the client class for handling it. | Catching runtime exceptions indicates a code smell; it means that you’re covering up a coding error.You wouldn’t get a NullPointerException unless you put necessary null checks in the code.RuntimeExceptions could be prevented by fixing your code in the first place. It is more appropriate to treat them as faults in the program rather than merely catching them during program execution. Blanket-catching everything – either Exception or Throwable is not a good practice because you’re assuming that you can recover from any exceptional behavior. There are some cases in which you shouldn’t, or realistically can’t (i.e. OutOfMemoryError for catch(Throwable t)). |
When should we make this type of custom exception? | If a client can reasonably be expected to recover from an exception, make it a checked exception. | If a client cannot do anything to recover from the exception, make it an unchecked exception. |
Exception Handling in Java with Examples
Exception handling in Java involves several keywords and constructs to effectively manage and respond to exceptions. Here’s a brief overview of how to handle exceptions using try
, catch
, finally
, throw
, and throws
:
try-catch
The try
block is used to enclose the code that might throw exceptions. The catch
block follows the try
block and catches specific exceptions that are thrown within the try
block. It allows you to handle the exception by providing appropriate code to address the exception scenario.
try {
// Code that may throw exceptions
} catch (ExceptionType1 exception1) {
// Handle exception1
} catch (ExceptionType2 exception2) {
// Handle exception2
}
finally
The finally
block is used to specify code that should be executed regardless of whether an exception occurs or not. It’s commonly used for cleanup operations that need to be performed, such as releasing resources like files or network connections.
try {
// Code that may throw exceptions
} catch (ExceptionType exception) {
// Handle exception
} finally {
// Cleanup code
}
throw
The throw
keyword is used to explicitly throw an exception from within your code. You can use this when you encounter a situation where an exception should be raised to indicate an error or unexpected condition.
if (condition) {
throw new CustomException("An error occurred.");
}
throws
The throws
keyword is used in a method declaration to indicate that the method may throw specific types of exceptions. It’s used to declare checked exceptions that might be thrown by the method, and it informs callers of the method’s potential exception scenarios. The actual handling of these exceptions is deferred to the calling code.
public ReturnType methodName() throws ExceptionType1, ExceptionType2 {
// Method code that may throw exceptions
}
Custom Exceptions in Java
You can define your own custom exception classes by extending the built-in Exception
class or one of its subclasses, such as RuntimeException
. This allows you to create meaningful exception types that match the specific errors in your application.
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
try-with-resources
The try-with-resources
statement is a feature introduced in Java 7 that simplifies and enhances resource management by automatically closing resources like files, streams, or network connections when they’re no longer needed. This mechanism ensures that resources are properly released, even in the presence of exceptions, without the need for explicit finally
blocks or manual resource management.
The syntax of the try-with-resources
statement looks like this:
try (ResourceType resource = new ResourceType()) {
// Code that uses the resource
} catch (ExceptionType exception) {
// Exception handling code
}
Common Use Cases: Exception Handling in Java
Java Exceptions play a crucial role in managing exceptional scenarios or errors within a Java program. These exceptions are utilized in various use cases to handle exceptional conditions effectively.
- Input Validation: Java exceptions are commonly employed for input validation, ensuring that user inputs meet the expected criteria. They help in handling errors when the input fails to conform to the specified format or range, using exceptions in Java to manage such scenarios.
- File Handling: When performing file operations in Java, such as reading, writing, or closing files, handling exceptions in Java is essential. Exceptions are used to manage errors like file not found or permission issues, ensuring proper file handling.
- Network Operations: Java exceptions are vital in network-related operations, such as handling connection timeouts or socket errors. By utilizing exceptions in Java, developers can effectively manage errors during network communication.
- Database Operations: When working with databases, exceptions in Java are used to handle errors during database connections, queries, or transactions. Exceptions like SQLException are commonly utilized to manage database-related errors.
- Concurrency and Multithreading: Java exceptions are significant in concurrent and multithreaded environments. They help in managing errors that may occur during thread execution or synchronization, using exceptions in Java to handle such scenarios.
Best Practices: Exception Handling in Java
Here are five best practices for exception handling in Java:
- Proper Exception Hierarchy: Define custom exception classes to represent specific exceptional scenarios, extending the base
Exception
class or its subclasses in Java. This helps in organizing and categorizing exceptions in Java effectively. - Specific Exception Handling: Handle exceptions at an appropriate level of granularity. Catch specific exceptions using try-catch blocks to provide targeted error handling for different exceptional situations in your Java code.
- Logging and Error Messages: Utilize logging frameworks to log exception details, including stack traces and contextual information. This facilitates effective debugging and troubleshooting of exceptions in Java. Additionally, provide meaningful error messages to enhance the user experience and aid in issue resolution.
- Avoid Swallowing Exceptions: Avoid suppressing or ignoring exceptions in Java without appropriate handling. Swallowing exceptions can lead to silent failures and make it challenging to diagnose and fix issues. Handle exceptions appropriately, either by resolving the problem or propagating the exception to higher levels of the application.
- Resource Cleanup with Finally: Use the
finally
block to ensure proper cleanup of resources, such as closing files, database connections, or network sockets. Thefinally
block is executed regardless of whether an exception occurs, providing a reliable mechanism for resource cleanup in Java.
By following these best practices for exception handling in Java, you can enhance code reliability, maintainability, and provide a better user experience. Exception handling in Java is essential for robust error management, ensuring smooth execution of your applications even in the face of unexpected situations.
FAQs: Exception Handling in Java
Q: What is a Java exception?
A: A Java exception is an event that occurs during the execution of a program, disrupting the normal flow of instructions. It represents an exceptional condition or error that needs to be handled.
Q: What is the difference between error and exception in Java?
A: In Java, errors are serious issues caused by the environment or JVM, usually unrecoverable, such as OutOfMemoryError. Exceptions are application-related issues that can be anticipated and handled, like NullPointerException. Errors are not typically caught or handled, while exceptions can be caught using try-catch blocks.
Q: What is the purpose of exception handling in Java?
A: Exception handling allows developers to handle and recover from exceptional situations in a controlled manner. It helps maintain the stability of the program by preventing abrupt termination and providing an opportunity to gracefully handle errors.
Q: What is the difference between checked and unchecked exceptions?
A: Checked exceptions are checked by the compiler at compile-time and must be declared or handled. Unchecked exceptions, on the other hand, are not checked at compile-time and do not require explicit handling.
Q: How can exceptions be thrown in Java?
A: Exceptions can be thrown using the throw statement or by calling methods that may throw exceptions. The throw statement explicitly throws an exception object, while methods can declare the exceptions they may throw using the throws clause.
Q: How can java exceptions be caught and handled?
A: Exceptions can be caught and handled using try-catch blocks. The code that may throw an exception is placed within the try block, and specific exceptions or exception types are caught and handled in the catch block.
Q: What is the role of the finally block in exception handling in Java?
A: The finally block is used to specify code that will be executed regardless of whether an exception occurs or not. It is commonly used to release resources or perform cleanup operations.
Q: Can we catch multiple exceptions in a single catch block?
A: Yes, it is possible to catch multiple exceptions in a single catch block by separating them with the pipe (|) symbol. This allows for a common handling mechanism for multiple related exceptions.
Q: What is the difference between catch and finally blocks?
A: The catch block is used to handle specific exceptions, whereas the finally block is used to specify code that must be executed regardless of whether an exception is thrown or not.
Q: Can we create custom exceptions in Java?
A: Yes, developers can create custom exceptions by extending the built-in Exception class or one of its subclasses. This allows for the creation of application-specific exception types.
Q: How can we ensure proper cleanup of resources in exception handling?
A: To ensure proper cleanup of resources, the try-finally block can be used. Resources are acquired within the try block and released in the finally block, ensuring they are properly cleaned up regardless of whether an exception occurs or not.
These FAQs provide a solid starting point for understanding exception handling in Java. They cover key concepts and address common questions that arise when working with exceptions in Java.
Conclusion: Exception Handling in Java
By understanding the distinction between exceptions and errors, as well as the difference between checked and unchecked exceptions, developers can make informed decisions about how to handle exceptional situations in their code. Proper exception handling in java helps improve code robustness, maintainability, and enhance overall application stability.
Java Exceptions Must-Read Articles:
- try, catch, and finally in Java
- throw and throws in Java
- Try with Resources
- Difference between Checked and Unchecked Exception in Java
- List of Checked Exception in Java
- List of Unchecked Exception in Java
- Common Errors in Java
- Custom Exception in Java
- Java Exception Handling Interview Questions with Answers
+1 for the last point. Very useful.