In this article, we’ll look at the complexities of multiple inheritance in Java, the infamous “diamond problem,” and the various approaches Java developers can take to achieve code reuse and maintainable class hierarchies.
Introduction: Multiple Inheritance in Java
Creators of the Java had the vision to create a simple object oriented language which can be learnt easily.
James Gosling the father of Java has outlined the below in a white paper titled “Java: an Overview”:
JAVA omits many rarely used, poorly understood, confusing features of C++ that in our experience bring more grief than benefit. This primarily consists of operator overloading (although it does have method overloading), multiple inheritance, and extensive automatic coercions.
In the designers’ opinion, multiple inheritance causes more problems and confusion than it solves. The designers’ extensive C++ experience taught them that multiple inheritance just wasn’t worth the headache.
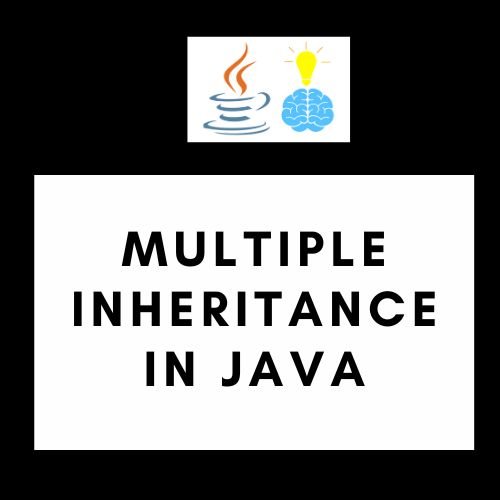
Why Multiple Inheritance is not Supported in Java?
Let us look into some of the reasons which enforced Java creators to not opt for Multiple Inheritance.
1.The Famous Diamond problem:

In the above figure, we can see that class B and C inherit class A. Now let us assume that B and C override the same inherited method and they provide their own implementation. Now D inherits from both B and C doing multiple inheritance. D should inherit that overridden method, which overridden method will be used? Will it be from B or C? Here we have an ambiguity.
Here’s a simple Java code example illustrating the diamond problem and how it’s avoided using the single inheritance model in Java:
class A {
void foo() {
System.out.println("A's foo");
}
}
class B extends A {
@Override
void foo() {
System.out.println("B's foo");
}
}
class C extends A {
@Override
void foo() {
System.out.println("C's foo");
}
}
class D extends B, C { // This line would cause a compilation error in Java
// ...
}
public class Main {
public static void main(String[] args) {
D d = new D();
d.foo(); // Which foo() method should be called? From B or from C?
}
}
In this example, we have classes A
, B
, C
, and D
. A
is the base class, while B
and C
inherit from A
and override its foo()
method. The class D
is intended to inherit from both B
and C
.
However, if you try to compile this code in Java, you’ll encounter a compilation error. Java doesn’t support multiple inheritance with classes, which is how the diamond problem is avoided.
2.To keep things simple:
As discussed in the beginning of the blog, the creators of Java wanted to keep it very simple so they opted out complex things like multiple inheritance.Multiple inheritances complicates the design and creates problem during casting, constructor chaining etc. So it was omitted for the sake of keeping simplicity of Java language.
3.Very very less usage:
How many times have we faced a situation where we are stranded and facing the wall because of the lack of support for multiple inheritance in java? Since it is rarely required, multiple inheritance can be safely omitted considering the complexity it has for implementation. It is not worth the hassle and the path of simplicity is chosen.Even if it is required it can be substituted with alternate design.
Alternatives to Multiple Inheritance in Java
- Interface Inheritance: Java allows a class to implement several interfaces using interface inheritance. Interfaces specify a contract that classes must follow, but they do not provide any implementation. A class can inherit behavior from many sources by implementing numerous interfaces. This is a typical method for achieving “multiple inheritance” in Java.
- Composition:Composition is the process of creating objects of other classes within your class in order to utilize their functionality. This is known as the “HAS-A” relationship. You can achieve code reuse without directly inheriting from several classes by combining your classes with instances of other ones. This follows the principle of Favour Composition over Inheritance.
- Default Methods in Interfaces (Java 8 and later): In Java 8, default methods in interfaces were introduced, allowing you to give method implementations directly in interfaces. This allows interfaces to be extended without breaking existing implementations.
- Wrapper Classes: You can create wrapper classes that contain instances of the classes you want to inherit from. These wrappers expose the desired functionality by forwarding method calls to the contained instances.
FAQs: Multiple Inheritance in Java
Here are some common questions that readers might have along with their brief answers:
- What is multiple inheritance in Java? Multiple inheritance refers to a scenario where a class inherits attributes and methods from more than one superclass. Java does not support multiple inheritance with classes due to the complexities it introduces.
- What is the “diamond problem” in Java? The diamond problem occurs when a class inherits from two classes that share a common base class. It leads to method ambiguity and conflicts in the inheritance hierarchy.
- Why doesn’t Java support multiple inheritance? Java opted for single inheritance to avoid the diamond problem and simplify class hierarchies, enhancing code clarity and predictability.
- What are the alternatives to multiple inheritance in Java? Java offers alternatives like interface inheritance, composition, default methods in interfaces, and wrapper classes to achieve code reuse without traditional multiple inheritance.
- How does interface inheritance work in Java? Interface inheritance allows a class to implement multiple interfaces, providing a way to inherit behaviors from various sources without the complexities of multiple inheritance.
- What is composition and how is it used as an alternative? Composition involves creating objects of other classes within your class to reuse functionality. It’s a “HAS-A” relationship that promotes code modularity and flexibility.
- What are default methods in interfaces? Default methods in interfaces (introduced in Java 8) provide default implementations for methods in interfaces. They allow extending interfaces without breaking existing implementations.
- How do wrapper classes address the lack of multiple inheritance? Wrapper classes encapsulate instances of other classes and expose their functionalities. They promote code reuse without directly inheriting from multiple classes.
Conclusion: Multiple Inheritance in Java
Java’s lack of multiple inheritance compresses class hierarchies, enhancing clarity and manageability. Alternatives like as interface inheritance, composition, and default methods provide various alternatives to code reuse.