Java 8 Date Time API was designed to overcome all the flaws in the legacy date time implementations. Some of the features of new Java 8 Date Time API are:
- Immutability: All the classes in the new Date Time API are immutable and good for multithreaded environments.
- Separation of Concerns: It defines separate classes for Date, Time, DateTime, Timestamp, Timezone etc.
- Utility operations: All the new Date Time API classes comes with methods to perform common tasks, such as plus, minus, format, parsing, getting separate part in date/time etc.
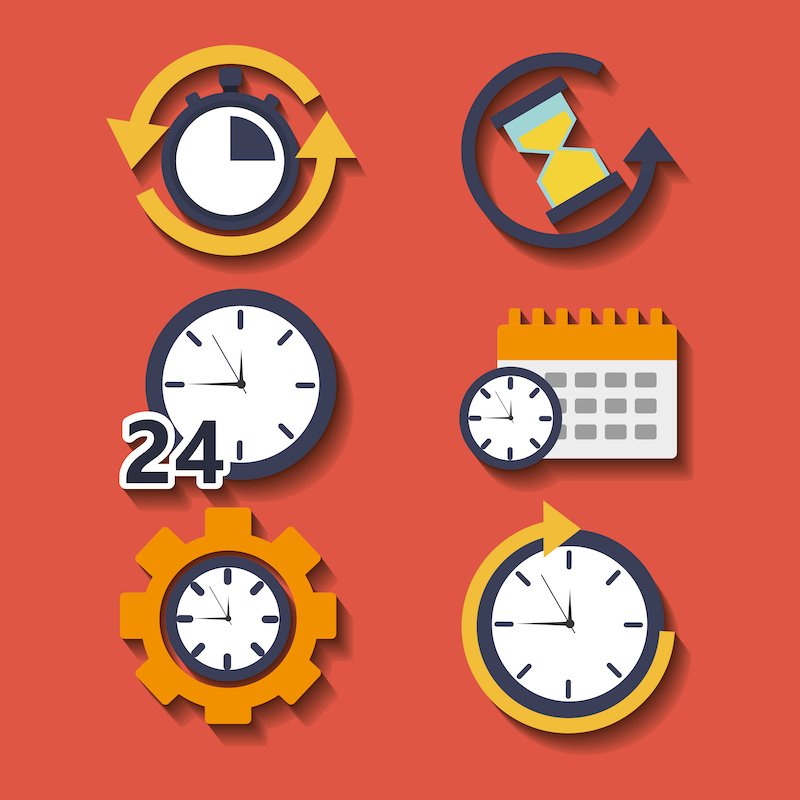
Let’s look into some of the important classes of Java 8 Date Time API.
LocalDate
- LocalDate is an immutable class that represents Date with default format of yyyy-MM-dd. We can use now() method to get the current date.
- We can also provide input arguments for year, month and date to create LocalDate instance.
- This class provides overloaded method for now() where we can pass ZoneId for getting date in specific time zone.
- This class provides the same functionality as java.sql.Date.
Java Code Snippet
Here’s a sample Java code snippet using LocalDate to demonstrate how to work with dates:
import java.time.LocalDate;
public class LocalDateExample {
public static void main(String[] args) {
// Create a LocalDate object representing today's date
LocalDate currentDate = LocalDate.now();
// Print the current date
System.out.println("Current Date: " + currentDate);
// Access individual components of the date
int year = currentDate.getYear();
int month = currentDate.getMonthValue();
int day = currentDate.getDayOfMonth();
// Print the components of the date separately
System.out.println("Year: " + year);
System.out.println("Month: " + month);
System.out.println("Day: " + day);
}
}
Output:
Current Date: 2023-07-05
Year: 2023
Month: 7
Day: 5
In the code above, we first create a LocalDate object called currentDate using the now() method, which represents the current date. We then print the current date using System.out.println(). Next, we access individual components of the date (year, month, and day) using the corresponding methods (getYear(), getMonthValue(), and getDayOfMonth()) and print them separately.
The output displays the current date in the format “yyyy-MM-dd”, followed by the individual components (year, month, and day) of the date.
LocalTime
LocalTime is an immutable class whose instance represents a time in the human readable format. It’s default format is hh:mm:ss.zzz. Just like LocalDate, this class provides time zone support and creating instance by passing hour, minute and second as input arguments.
Java Code Snippet
Here’s a sample Java code snippet using LocalTime to demonstrate how to work with time:
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
public class LocalTimeExample {
public static void main(String[] args) {
// Create a LocalTime object representing the current time
LocalTime currentTime = LocalTime.now();
// Print the current time using a specific format
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("HH:mm:ss");
String formattedTime = currentTime.format(formatter);
System.out.println("Current Time: " + formattedTime);
// Access individual components of the time
int hour = currentTime.getHour();
int minute = currentTime.getMinute();
int second = currentTime.getSecond();
// Print the components of the time separately
System.out.println("Hour: " + hour);
System.out.println("Minute: " + minute);
System.out.println("Second: " + second);
}
}
Output
Current Time: 13:45:30
Hour: 13
Minute: 45
Second: 30
In the code above, we create a LocalTime object called currentTime using the now() method, which represents the current time. We then use a DateTimeFormatter to format the time in the “HH:mm:ss” pattern. The formatted time is printed using System.out.println().
Next, we access individual components of the time (hour, minute, and second) using the corresponding methods (getHour(), getMinute(), and getSecond()) and print them separately.
The output displays the current time in the format “HH:mm:ss”, followed by the individual components (hour, minute, and second) of the time.
LocalDateTime
LocalDateTime is an immutable date-time object that represents a date-time, with default format as yyyy-MM-dd-HH-mm-ss.zzz. It provides a factory method that takes LocalDate and LocalTime input arguments to create LocalDateTime instance.
Java Code Snippet
Here’s a sample Java code snippet using LocalDateTime to demonstrate how to work with date and time together:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class LocalDateTimeExample {
public static void main(String[] args) {
// Create a LocalDateTime object representing the current date and time
LocalDateTime currentDateTime = LocalDateTime.now();
// Print the current date and time using a specific format
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = currentDateTime.format(formatter);
System.out.println("Current Date and Time: " + formattedDateTime);
// Access individual components of the date and time
int year = currentDateTime.getYear();
int month = currentDateTime.getMonthValue();
int day = currentDateTime.getDayOfMonth();
int hour = currentDateTime.getHour();
int minute = currentDateTime.getMinute();
int second = currentDateTime.getSecond();
// Print the components of the date and time separately
System.out.println("Year: " + year);
System.out.println("Month: " + month);
System.out.println("Day: " + day);
System.out.println("Hour: " + hour);
System.out.println("Minute: " + minute);
System.out.println("Second: " + second);
}
}
Output
Current Date and Time: 2023-07-05 13:45:30
Year: 2023
Month: 7
Day: 5
Hour: 13
Minute: 45
Second: 30
In the code above, we create a LocalDateTime object called currentDateTime using the now() method, which represents the current date and time. We then use a DateTimeFormatter to format the date and time in the “yyyy-MM-dd HH:mm:ss” pattern. The formatted date and time are printed using System.out.println().
Next, we access individual components of the date and time (year, month, day, hour, minute, and second) using the corresponding methods and print them separately.
The output displays the current date and time in the specified format, followed by the individual components (year, month, day, hour, minute, and second) of the date and time.
Instant
Instant class is used to work with machine readable time format, it stores date time in unix timestamp.
Java Code Snippet
Here’s a sample Java code snippet using Instant to work with instant timestamps:
import java.time.Instant;
public class InstantExample {
public static void main(String[] args) {
// Get the current timestamp in UTC
Instant currentTimestamp = Instant.now();
// Print the current timestamp
System.out.println("Current Timestamp: " + currentTimestamp);
// Get the epoch timestamp in milliseconds
long epochMillis = currentTimestamp.toEpochMilli();
// Print the epoch timestamp
System.out.println("Epoch Timestamp (Milliseconds): " + epochMillis);
// Create an Instant from an epoch timestamp
Instant fromEpoch = Instant.ofEpochMilli(epochMillis);
// Print the Instant created from the epoch timestamp
System.out.println("Instant from Epoch Timestamp: " + fromEpoch);
}
}
Output
Current Timestamp: 2023-07-05T13:45:30.123456Z
Epoch Timestamp (Milliseconds): 1678909530123
Instant from Epoch Timestamp: 2023-07-05T13:45:30.123Z
In the code above, we first obtain the current timestamp in UTC using Instant.now(). We then print the current timestamp.
Next, we convert the Instant to an epoch timestamp in milliseconds using toEpochMilli() and store it in the epochMillis variable. We print the epoch timestamp.
We then create a new Instant object fromEpoch using Instant.ofEpochMilli() by passing the epoch timestamp. Finally, we print the Instant object created from the epoch timestamp.
The output displays the current timestamp in the default ISO-8601 format with UTC offset, followed by the epoch timestamp in milliseconds, and the Instant object created from the epoch timestamp.
ZonedDateTime
The ZonedDateTime class in the Java 8 date time API represents a date and time with time zone information.
Java Code Snippet
Here’s a sample Java code snippet using ZonedDateTime to work with date, time, and time zone:
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class ZonedDateTimeExample {
public static void main(String[] args) {
// Create a LocalDateTime object representing a specific date and time
LocalDateTime localDateTime = LocalDateTime.of(2023, 7, 5, 13, 45, 30);
// Specify a time zone
ZoneId zoneId = ZoneId.of("America/New_York");
// Create a ZonedDateTime object by combining LocalDateTime and ZoneId
ZonedDateTime zonedDateTime = ZonedDateTime.of(localDateTime, zoneId);
// Print the ZonedDateTime using a specific format
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss z");
String formattedDateTime = zonedDateTime.format(formatter);
System.out.println("Zoned Date and Time: " + formattedDateTime);
// Access individual components of the ZonedDateTime
int year = zonedDateTime.getYear();
int month = zonedDateTime.getMonthValue();
int day = zonedDateTime.getDayOfMonth();
int hour = zonedDateTime.getHour();
int minute = zonedDateTime.getMinute();
int second = zonedDateTime.getSecond();
String zone = zonedDateTime.getZone().toString();
// Print the components of the ZonedDateTime separately
System.out.println("Year: " + year);
System.out.println("Month: " + month);
System.out.println("Day: " + day);
System.out.println("Hour: " + hour);
System.out.println("Minute: " + minute);
System.out.println("Second: " + second);
System.out.println("Time Zone: " + zone);
}
}
Output
Zoned Date and Time: 2023-07-05 13:45:30 EDT
Year: 2023
Month: 7
Day: 5
Hour: 13
Minute: 45
Second: 30
Time Zone: America/New_York
In the code above, we first create a LocalDateTime object localDateTime representing a specific date and time. We then specify a time zone using ZoneId by providing the zone ID as a string. In this example, we use “America/New_York”.
Next, we create a ZonedDateTime object zonedDateTime by combining the localDateTime and zoneId. We then use a DateTimeFormatter to format the ZonedDateTime in the desired format. The formatted date and time are printed using System.out.println().
We also access individual components of the ZonedDateTime (year, month, day, hour, minute, second, and time zone) using the corresponding methods and print them separately.
The output displays the zoned date and time in the specified format, followed by the individual components (year, month, day, hour, minute, second, and time zone) of the ZonedDateTime.
DateTimeFormatter – Parsing and Formatting
We can use DateTimeFormatter to uniformly format dates and times with predefined or user-defined patterns.
The DateTimeFormatter class contains a set of predefined (constant) instances which can parse and format dates from standard date formats. This saves you the trouble of defining a date format for a DateTimeFormatter. The DateTimeFormatter class contains the following predefined instances:
- BASIC_ISO_DATE
- ISO_LOCAL_DATE
- ISO_LOCAL_TIME
- ISO_LOCAL_DATE_TIME
- ISO_OFFSET_DATE
- ISO_OFFSET_TIME
- ISO_OFFSET_DATE_TIME
- ISO_ZONED_DATE_TIME
- ISO_INSTANT
- ISO_DATE
- ISO_TIME
- ISO_DATE_TIME
- ISO_ORDINAL_TIME
- ISO_WEEK_DATE
Java Code Snippet
Here’s a sample Java code snippet using DateTimeFormatter to format and parse dates:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeFormatterExample {
public static void main(String[] args) {
// Create a LocalDateTime object representing a specific date and time
LocalDateTime dateTime = LocalDateTime.of(2023, 7, 5, 13, 45, 30);
// Create a DateTimeFormatter for formatting and parsing dates
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
// Format the LocalDateTime using the DateTimeFormatter
String formattedDateTime = dateTime.format(formatter);
System.out.println("Formatted Date and Time: " + formattedDateTime);
// Parse a string into a LocalDateTime using the DateTimeFormatter
String dateString = "2023-07-05 13:45:30";
LocalDateTime parsedDateTime = LocalDateTime.parse(dateString, formatter);
System.out.println("Parsed Date and Time: " + parsedDateTime);
}
}
Output
Formatted Date and Time: 2023-07-05 13:45:30
Parsed Date and Time: 2023-07-05T13:45:30
In the code above, we first create a LocalDateTime object dateTime representing a specific date and time. We then create a DateTimeFormatter formatter with the desired format pattern “yyyy-MM-dd HH:mm:ss”.
Next, we format the dateTime object into a string representation using the format() method of DateTimeFormatter. The formatted date and time are stored in the formattedDateTime variable and printed using System.out.println().
Additionally, we demonstrate parsing a string representation of a date and time (dateString) back into a LocalDateTime object using the parse() method of DateTimeFormatter. The parsed date and time are stored in the parsedDateTime variable and printed.
The output displays the formatted date and time based on the pattern “yyyy-MM-dd HH:mm:ss”, followed by the parsed date and time in the default LocalDateTime format.
Conclusion
In conclusion, the Java 8 Date Time API provides developers with a powerful and intuitive framework for seamless time manipulation. With its comprehensive set of classes and methods, the API simplifies common time-related operations and empowers developers to efficiently handle dates, times, and time zones.
The Java 8 Date Time API offers improved functionality over its predecessor, the java.util.Date and java.util.Calendar classes. It introduces new classes such as LocalDate, LocalTime, LocalDateTime, and ZonedDateTime, which provide clearer and more precise representations of dates, times, and time zones.
By leveraging the Java 8 Date Time API, developers can easily perform operations like date arithmetic, parsing and formatting, time zone conversions, and more. The API’s immutable nature ensures thread safety and facilitates writing cleaner and more maintainable code.
Moreover, the API embraces modern date and time standards, adhering to the ISO-8601 format and supporting various commonly used patterns. It also integrates seamlessly with other Java 8 features, such as lambda expressions and the Stream API, enabling concise and expressive coding.
In summary, the Java 8 Date Time API offers a robust and efficient solution for handling date and time in Java applications. Its streamlined design, improved functionality, and compatibility with other Java 8 features make it an indispensable tool for developers seeking seamless time manipulation. By embracing the Java 8 Date Time API, developers can elevate their code to new levels of clarity, precision, and reliability.
Related Articles :