In this article, we will explore different ways to implement binary search in Java, discuss the logical steps involved, analyze its time and space complexity, explore its applications, and conclude with the importance of this algorithm in programming.
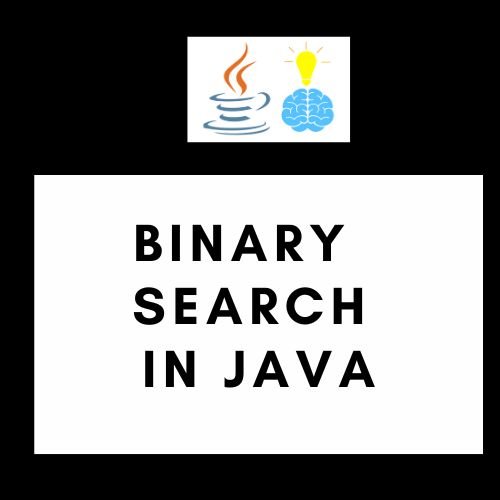
What is a Binary Search?
Binary search is a fundamental searching algorithm used to find a specific target element in a sorted array. It employs a divide-and-conquer strategy to efficiently search for the desired element.
Different Approaches to Implement Binary Search in Java
We can follow two approaches to implement Binary Search in Java.
- Iterative Approach
- Recursive Approach
Logical Steps:
Logical steps for implementing Binary Search in Java.
- Set the low and high pointers to the start and end indices of the array, respectively.
- Compute the mid index as the average of low and high.
- Compare the target element with the element at the mid index.
- If the target matches the mid element, return the mid index.
- If the target is smaller than the mid element, update the high pointer to mid – 1 and repeat from step 2.
- If the target is larger than the mid element, update the low pointer to mid + 1 and repeat from step 2.
- Continue narrowing down the search range until the target is found or the search range becomes empty.
Iterative Approach
The iterative implementation of binary search in Java involves dividing the array into two halves and repeatedly narrowing down the search range until the target element is found or the search range becomes empty. Here’s a sample Java code for iterative binary search:
public class BinarySearch {
public static int binarySearchIterative(int[] arr, int target) {
int low = 0;
int high = arr.length - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1; // Element not found
}
public static void main(String[] args) {
int[] arr = {2, 5, 8, 12, 16, 23, 38, 56, 72, 91};
int target = 16;
int result = binarySearchIterative(arr, target);
if (result == -1) {
System.out.println("Element not found in the array.");
} else {
System.out.println("Element found at index: " + result);
}
}
}
Output
Element found at index: 4
Recursive Approach
The recursive implementation of binary search in Java follows a similar divide-and-conquer approach, but it uses a recursive function to perform the search. Here’s a sample Java code for recursive binary search:
public class BinarySearch {
public static int binarySearchRecursive(int[] arr, int target, int low, int high) {
if (low <= high) {
int mid = low + (high - low) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
return binarySearchRecursive(arr, target, mid + 1, high);
} else {
return binarySearchRecursive(arr, target, low, mid - 1);
}
}
return -1; // Element not found
}
public static void main(String[] args) {
int[] arr = {2, 5, 8, 12, 16, 23, 38, 56, 72, 91};
int target = 16;
int result = binarySearchRecursive(arr, target, 0, arr.length - 1);
if (result == -1) {
System.out.println("Element not found in the array.");
} else {
System.out.println("Element found at index: " + result);
}
}
}
Output
Element found at index: 4
Time Complexity
The time complexity of binary search in Java is O(log n) as the search range gets halved in each iteration. This makes binary search significantly faster than linear search for large datasets.
Space Complexity
The space complexity of binary search is O(1) as it only requires a few additional variables to perform the search and does not require extra memory proportional to the input size.
Applications of Binary Search
- Searching in sorted arrays or collections.
- Finding boundaries or positions in ordered datasets.
- Implementing data structures like binary search trees.
- Solving optimization problems with monotonic functions.
Conclusion
Binary search is a powerful algorithm for searching sorted arrays efficiently. Its divide-and-conquer approach and logarithmic time complexity make it a preferred choice for searching large datasets. In Java, binary search can be implemented iteratively or recursively, offering flexibility in solving different problems. Understanding binary search and its implementations is crucial for efficient searching and solving various programming challenges.
Related Article: Linear Search in Java: Simple Search Algorithm