In this article, we will explore different sorting algorithms in Java, their working principles, time and space complexities, and their practical applications.
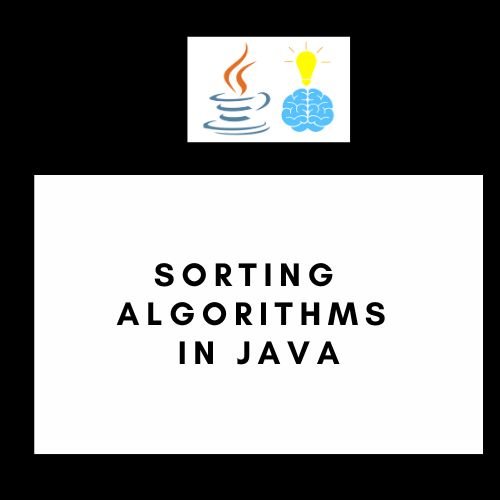
Bubble Sort
Bubble Sort is one of the simple and intuitive sorting algorithms in Java. It repeatedly compares adjacent elements and swaps them if they are in the wrong order. The largest element “bubbles” to the end of the array in each pass. Despite its simplicity, Bubble Sort has a time complexity of O(n^2), making it less efficient for large datasets.
Read More : Bubble Sort in Java: A Easy Sorting Algorithm
Selection Sort
Selection Sort is one of the frequently used sorting algorithms in Java. It works by repeatedly selecting the smallest element from the unsorted part of the array and swapping it with the element at the beginning of the sorted part. It continues until the entire array is sorted. Selection Sort also has a time complexity of O(n^2), but it performs better than Bubble Sort in practice due to fewer swaps.
Read More : Selection Sort in Java: A Simple Sorting Algorithm
Insertion Sort
Insertion sort is a very commonly used sorting algorithms in Java. It Sort builds the final sorted array one element at a time. It iterates through the array, comparing each element with the already sorted portion and placing it in the correct position. Insertion Sort has an average and best-case time complexity of O(n), making it efficient for small or nearly sorted arrays.
Read More: Insertion Sort in Java: An Easy toUnderstand Sorting Algorithm
Merge Sort
Merge Sort is a divide-and-conquer algorithm that divides the array into two halves, recursively sorts them, and then merges them back into a single sorted array. It has a time complexity of O(n log n) and is known for its stability and guaranteed performance. Merge Sort is often preferred for large datasets or when stability is important.
Read More: Merge Sort in Java: An Efficient Sorting Algorithm
Quick Sort
Quick Sort is another divide-and-conquer algorithm that chooses a pivot element, partitions the array around the pivot, and recursively sorts the subarrays on either side of the pivot. It has an average time complexity of O(n log n), but in the worst-case scenario, it can degrade to O(n^2). Quick Sort is widely used due to its efficiency and in-place sorting.
Read More : Quick Sort in Java: A Fast and Efficient Sorting Algorithm
Heap Sort
Heap Sort is based on the binary heap data structure. It first builds a max heap from the array and then repeatedly extracts the maximum element, placing it at the end of the array. Heap Sort has a time complexity of O(n log n) and is often used when a stable sort is not required.
Read More : Heap Sort in Java: Efficient Sorting with Binary Heaps
Radix Sort
Radix Sort is a non-comparative sorting algorithm that sorts numbers by grouping them based on individual digits. It processes the digits from the least significant to the most significant, achieving linear time complexity. Radix Sort is particularly useful for sorting integers with a fixed number of digits.
Read More : Radix Sort in Java: Sort Numbers Digit by Digit
Conclusion: Sorting algorithms in Java
Sorting algorithms play a vital role in organizing and manipulating data efficiently. Each sorting algorithm has its advantages and trade-offs in terms of time complexity, space complexity, and stability. You have a variety of sorting algorithms in Java at your disposal, and selecting the right one can significantly impact the performance of your applications.
Related Article : Different Ways to Sort Array in Java