In this comprehensive article, we will learn the concepts of DFS(Depth First Search) and BFS(Breadth First Search) and then look into the key differences between DFS and BFS. Finally, we will discuss the various use-cases and applications of DFS and BFS.
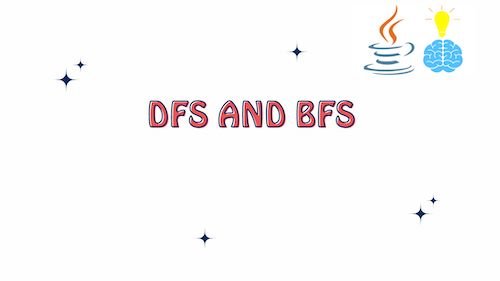
What is DFS(Depth First Search) ?
Depth First Search (DFS) is a graph traversal algorithm used to explore and navigate through graph or tree structures. It’s named “depth-first” because it explores as far as possible along one branch before backtracking.
Example:
Let’s take a look at the structure of Depth First Search (DFS) on a simple tree. Consider the following binary tree:
A
/ \
B C
/ \ \
D E F
Starting from node A, let’s go through the steps of the DFS algorithm:
- Start at node A.
- Mark node A as visited.
- Explore node A’s left child (B).
- Move to node B.
- Mark node B as visited.
- Explore node B’s left child (D).
- Move to node D.
- Mark node D as visited.
- No unvisited child nodes for node D.
- Backtrack to node B.
- Explore node B’s right child (E).
- Move to node E.
- Mark node E as visited.
- No unvisited child nodes for node E.
- Backtrack to node B.
- Backtrack to node A.
- Explore node A’s right child (C).
- Move to node C.
- Mark node C as visited.
- Explore node C’s right child (F).
- Move to node F.
- Mark node F as visited.
- No unvisited child nodes for node F.
- Backtrack to node C.
The traversal order in this example would be A -> B -> D -> E -> C -> F.
Different Traversal Orders for DSF
Depth First Search (DFS) can have three different traversal orders, each with its own characteristics. Let’s explore these traversal orders using a simple binary tree:
A
/ \
B C
/ \ \
D E F
Here’s the tree structure for reference. Now, let’s look at the different DFS traversal orders:
- Preorder Traversal (Node-Left-Right): In preorder traversal, the algorithm visits the current node, then its left subtree, and finally its right subtree.Traversal Order: A -> B -> D -> E -> C -> F
- Inorder Traversal (Left-Node-Right): In inorder traversal, the algorithm visits the left subtree, then the current node, and finally the right subtree.Traversal Order: D -> B -> E -> A -> C -> F
- Postorder Traversal (Left-Right-Node): In postorder traversal, the algorithm visits the left subtree, then the right subtree, and finally the current node.Traversal Order: D -> E -> B -> F -> C -> A
Here’s a summary of the different traversal orders using the provided binary tree:
Preorder: A -> B -> D -> E -> C -> F
Inorder: D -> B -> E -> A -> C -> F
Postorder: D -> E -> B -> F -> C -> A
Each traversal order has its own use cases and advantages. For example, inorder traversal of a binary search tree gives nodes in ascending order, making it useful for sorted data retrieval. Preorder traversal can be useful in creating a copy of the tree, while postorder traversal is often used in deleting nodes from a tree.
Java Code Example for DSF
import java.util.*;
class Node {
int data;
Node left;
Node right;
Node(int data) {
this.data = data;
left = null;
right = null;
}
}
class BinaryTree {
Node root;
void inOrder(Node root) {
if (root != null) {
inOrder(root.left);
System.out.print(root.data + " ");
inOrder(root.right);
}
}
void preOrder(Node root) {
if (root != null) {
System.out.print(root.data + " ");
preOrder(root.left);
preOrder(root.right);
}
}
void postOrder(Node root) {
if (root != null) {
postOrder(root.left);
postOrder(root.right);
System.out.print(root.data + " ");
}
}
}
public class Main {
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.root = new Node(1);
tree.root.left = new Node(2);
tree.root.right = new Node(3);
tree.root.left.left = new Node(4);
tree.root.left.right = new Node(5);
System.out.println("inOrder: ");
tree.inOrder(tree.root);
System.out.println("\npreOrder: ");
tree.preOrder(tree.root);
System.out.println("\npostOrder: ");
tree.postOrder(tree.root);
}
}
inOrder:
4 2 5 1 3
preOrder:
1 2 4 5 3
postOrder:
4 5 2 3 1
What is BFS(Breadth First Search) ?
Breadth First Search (BFS) is a graph traversal algorithm used to systematically explore and navigate through graph or tree structures. Unlike Depth First Search (DFS) that delves as deep as possible before backtracking, BFS explores nodes level by level, starting from the root (or source) node and moving outward to neighboring nodes.
Example:
Let’s visualize a simple tree and go through the steps of Breadth First Search (BFS) on that tree. Consider the following binary tree:
A
/ \
B C
/ \ \
D E F
Starting from node A, let’s walk through the BFS steps:
- Start at node A.
- Add node A to the queue.
- Visit node A (print its value) and mark it as visited.
- Enqueue node A’s children (B and C).
- Dequeue node B from the queue.
- Visit node B and mark it as visited.
- Enqueue node B’s children (D and E).
- Dequeue node C from the queue.
- Visit node C and mark it as visited.
- Enqueue node C’s children (F).
- Dequeue node D from the queue.
- Visit node D and mark it as visited.
- Dequeue node E from the queue.
- Visit node E and mark it as visited.
- Dequeue node F from the queue.
- Visit node F and mark it as visited.
The traversal order in this example would be A -> B -> C -> D -> E -> F.
Java Code Example for BSF
import java.util.*;
class Node {
int data;
Node left;
Node right;
Node(int data) {
this.data = data;
left = null;
right = null;
}
}
class BinaryTree {
Node root;
void levelOrder(Node root) {
if (root == null)
return;
Queue<Node> q = new LinkedList<>();
q.add(root);
while (!q.isEmpty()) {
Node n = q.remove();
System.out.print(n.data + " ");
if (n.left != null)
q.add(n.left);
if (n.right != null)
q.add(n.right);
}
}
}
public class Main {
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.root = new Node(1);
tree.root.left = new Node(2);
tree.root.right = new Node(3);
tree.root.left.left = new Node(4);
tree.root.left.right = new Node(5);
System.out.println("\nlevelOrder: ");
tree.levelOrder(tree.root);
}
}
levelOrder:
1 2 3 4 5
Difference Between DFS and BFS
Let’s explore the key differences between DFS and BFS.
Aspect | Depth First Search (DFS) | Breadth First Search (BFS) |
---|---|---|
Traversal Order | Goes as deep as possible before backtracking | Explores level by level |
Data Structure | Typically uses a stack (recursion or explicit) | Uses a queue |
Shortest Path | May not find the shortest path | Guarantees finding shortest path |
Memory Usage | Consumes less memory | Can consume more memory |
Use Cases | Maze solving, topological sorting, finding connected components | Shortest path, finding neighbors |
Exploration Pattern | Deeper before broader exploration | Broader before deeper exploration |
Complexity | Can be less efficient in large graphs or deep trees | Generally more efficient and predictable |
Use Cases and Applications of DSF and BSF
Let’s look into the various use-cases and applications of DSF and BSF.
Use Cases and Applications of DSF
- Maze Solving: DFS can be used to navigate through mazes or puzzles by exploring paths deeply before backtracking.
- Topological Sorting: DFS is often used to find a linear ordering of nodes in directed acyclic graphs, such as scheduling tasks with dependencies.
- Finding Connected Components: DFS can identify connected components in an undirected graph, helping to understand graph structure.
- Pathfinding: It can be applied to find paths between nodes, even in cases where the shortest path isn’t necessary.
- Parsing and Traversal: It’s used in parsing and evaluating expressions or tree structures in languages or compilers.
- Decision Tree Traversal: In decision tree algorithms, DFS helps explore possible outcomes and make decisions.
- Graph Algorithms: Various graph algorithms use DFS as a building block to solve complex problems.
Use Cases and Applications of BSF
- Shortest Pathfinding: BFS guarantees finding the shortest path in an unweighted graph or tree.
- Web Crawling: BFS can be used to crawl and index web pages layer by layer, ensuring efficient discovery.
- Social Network Analysis: BFS helps identify relationships and connections between users in social networks.
- Level-Wise Traversal: BFS can be useful in tree and graph traversal where exploring one level before moving to the next is essential.
- Puzzle Solving: BFS is effective for solving sliding puzzles, where each move represents a level of exploration.
- Minimum Spanning Tree: BFS is used to find the minimum spanning tree in a graph, a tree that spans all nodes with the minimum possible edge weight.
- Network Broadcast: BFS can simulate a broadcast operation in computer networks to reach all nodes from a single source.
- Geographical Mapping: BFS is applied in mapping and geographical navigation to find paths and distances.
Conclusion : DSF and BSF
In this comprehensive article, we delved into the concepts of DFS and BFS algorithms, providing a clear understanding of their functionalities and differences. DFS explores graph or tree structures by going as deep as possible along one branch before backtracking, whereas BFS traverses nodes level by level, starting from the root and moving outward to neighboring nodes.
The article highlighted the three different traversal orders for DFS: Preorder, Inorder, and Postorder, each with distinct characteristics and applications. It also offered Java code examples for both DFS and BFS implementations, demonstrating their practical usage.
The differences between DFS and BFS were outlined, including their traversal order, data structures (stack vs. queue), effectiveness in finding the shortest path, memory usage, and use cases. DFS is suitable for maze solving, topological sorting, finding connected components, and other scenarios where deeper exploration is required. On the other hand, BFS excels in shortest pathfinding, web crawling, social network analysis, and situations that demand broader exploration before depth.
Overall, understanding the nuances of DFS and BFS, along with their various applications, equips us with versatile tools for navigating and analyzing graph and tree structures in different problem domains.