In this article , we will explore “Garbage Collection Optimization in Java”. So, let’s get started.
Introduction: Garbage Collection Optimization in Java
Garbage collection (GC) is a crucial aspect of memory management in Java applications. It involves reclaiming memory occupied by objects that are no longer in use, preventing memory leaks, and ensuring the efficient utilization of system resources. However, optimizing garbage collection isn’t a one-size-fits-all task; it involves balancing three key performance goals: latency, throughput, and footprint.
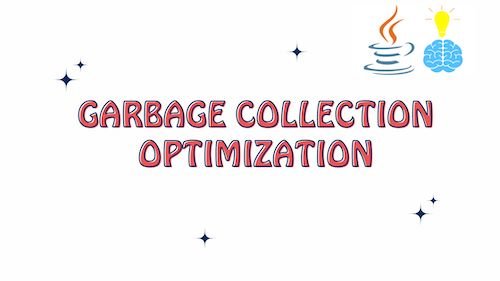
GC Performance Goals
1. Latency
Latency, in the context of garbage collection, refers to the pauses induced by the Java Virtual Machine (JVM) as it performs garbage collection. For Java applications, especially those requiring high responsiveness, minimizing latency is critical. There are two key latency goals:
a. Mean Pause Time
This represents the average time a garbage collection pause takes. Lowering the mean pause time ensures that your application remains responsive.
b. Maximum Pause Time
The maximum pause time is the longest single pause experienced by your application due to garbage collection. Minimizing the maximum pause time is essential to prevent significant disruptions.
2. Throughput
Throughput is the percentage of wall-clock time the JVM has available for executing the application. In other words, it’s the amount of time your application spends doing useful work. Achieving high throughput means your application can efficiently process requests and tasks.
It’s important to note that high throughput doesn’t necessarily mean low latency. Your application might achieve high throughput while still experiencing occasional longer pauses.
3. Footprint
The footprint of garbage collection is the amount of memory that the JVM consumes to execute your application. For memory-constrained environments, keeping the footprint in check is crucial.
The Two-Out-Of-Three Principle
In practice, it’s often challenging to optimize garbage collection for all three performance goals simultaneously. Therefore, it’s recommended to prioritize two goals and make trade-offs with the third. Let’s explore some scenarios:
a. Throughput and Latency
You might prioritize high throughput and acceptable mean pause times. To achieve this, you allocate more memory to the heap. However, longer pauses (maximum pause time) may occur due to larger heap sizes.
b. Latency and Footprint
If minimizing latency is your top priority, you allocate less memory to the heap to reduce mean pause times. Unfortunately, this might increase the frequency of garbage collection (higher throughput), impacting the overall application throughput.
c. Throughput and Footprint
For scenarios where a small memory footprint is crucial, you allocate less memory to the heap, improving mean pause times. However, this might reduce the overall throughput of the application.
In summary, optimizing garbage collection involves a delicate balancing act. It’s rare to achieve all performance goals simultaneously, so prioritize the two that align best with your application’s requirements.
Strategies for Garbage Collection Optimization
1. Reduce GC Frequency
For generational GC algorithms, you can decrease collection frequency by:
- Reducing Object Allocation/Promotion: Minimize object creation and promotion to older generations.
- Increasing Generation Size: Enlarge the size of generations to reduce their frequency.
2. Reduce GC Pause Duration
To decrease young generation GC pause duration, consider reducing the young generation size. However, closely monitor the impact on overall throughput and latency, as this may increase the frequency of garbage collection.
3. Choose the Right GC Algorithm
Different garbage collection algorithms have varying impacts on CPU usage and memory overhead. For instance:
- Concurrent (CMS) GC increases CPU usage but has shorter pause times.
- G1 GC uses concurrent and parallel phases to achieve predictable pause times but may impose a larger memory overhead.
4. JVM Tuning
Utilize JVM parameters to fine-tune garbage collection behavior:
-Xms
and-Xmx
: Set boundaries on heap size to control garbage collection predictability.-XX:+UseG1GC
: Use the Garbage First (G1) Collector.-XX:MaxGCPauseMillis
: Set a target for maximum GC pause time.-XX:ParallelGCThreads
and-XX:ConcGCThreads
: Configure the number of threads used during parallel and concurrent phases.
5. Avoid System.gc()
Invoking System.gc()
triggers stop-the-world Full GC events. To prevent this, consider using -XX:+DisableExplicitGC
to disable System.gc()
calls in your application.
Conclusion: Garbage Collection Optimization in Java
Garbage collection optimization in Java is a multidimensional challenge, involving trade-offs between latency, throughput, and footprint. By understanding your application’s requirements and carefully tuning garbage collection strategies, JVM parameters, and memory allocation, you can strike the right balance and ensure your Java application performs optimally while meeting its service-level agreements.