In this article, we will have a detailed exploration of top 60+ Hibernate Interview Questions.We’ve categorized the Hibernate Interview Questions into three levels: Basic, Intermediate, and Advanced. This categorization allows you to navigate through the content based on your proficiency level and gradually build your expertise.
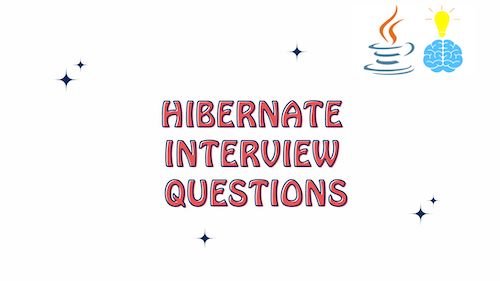
Basic Level : Hibernate Interview Questions
Let’s begin with the basic Hibernate interview questions.
What are ORM Tools?
ORM(Object-Relational Mapping) tools are software frameworks and libraries that simplify the interaction between object-oriented code in programming languages like Java,Python etc and relational databases.
Popular ORM tools in Java:
- Hibernate
- EclipseLink
- MyBatis
- jOOQ (Java Object Oriented Querying)
- TopLink
What is Hibernate?
Hibernate is an open-source Java-based Object-Relational Mapping (ORM) framework. Hibernate abstracts low-level JDBC (Java Database Connectivity) operations and provides a high-level, intuitive API for working with relational databases.
What are the advantages of Hibernate over JDBC?
Advantages of Hibernate over JDBC:
- Object-Relational Mapping (ORM):Hibernate provides a higher-level, object-oriented abstraction over relational databases, allowing developers to work with Java objects instead of raw SQL queries and result sets.
- Productivity:Hibernate significantly reduces the amount of boilerplate code required for database operations. This results in faster development and fewer opportunities for coding errors.
- Database Independence:Hibernate abstracts the database-specific SQL syntax, making it possible to switch between different database systems with minimal code changes. This enhances portability and flexibility.
- Automatic Table Creation and Schema Management:Hibernate can automatically generate database tables based on your Java entity classes, eliminating the need for manual table creation and schema synchronization.
- Caching:Hibernate includes caching mechanisms (first-level and second-level cache) that can greatly improve application performance by reducing the number of database queries.
- Lazy Loading:Hibernate supports lazy loading of associated objects, which means that it loads related data from the database only when needed, improving efficiency and reducing data transfer overhead.
- Transaction Management:Hibernate provides built-in transaction management capabilities, simplifying the handling of database transactions and ensuring data integrity.
- Concurrency Control:Hibernate offers optimistic locking and versioning mechanisms, helping manage concurrent access to database records and preventing data conflicts.
- Query Language (HQL):Hibernate Query Language (HQL) allows developers to write database queries in a more intuitive, SQL-like syntax, making it easier to work with complex queries.
- Integration with Java EE and Spring:Hibernate integrates seamlessly with Java EE and the Spring Framework, making it suitable for a wide range of Java-based enterprise applications.
- Community and Ecosystem:Hibernate has a large and active community, which means there are plenty of resources, documentation, and third-party libraries available to support developers.
- Scalability:Hibernate is capable of handling complex data models and large datasets, making it suitable for both small and large-scale applications.
List of the databases supported by Hibernate
Hibernate supports a wide range of relational databases. Here is a list of some of the databases that Hibernate commonly supports:
- MySQL
- PostgreSQL
- Oracle Database
- Microsoft SQL Server
- IBM Db2
- SQLite
- HSQLDB (HyperSQL Database)
- Sybase
- Informix
- Apache Derby
- MariaDB
- Amazon RDS (Relational Database Service)
- Google Cloud SQL
- MongoDB (with the help of third-party extensions like Hibernate OGM)
- SAP HANA
What are the different features of Hibernate?
Here are some of the different features of Hibernate:
- Automatic Table Generation:Hibernate can automatically generate database tables based on your Java entity classes, reducing the need for manual table creation and schema management.
- Database Independence(Custom Dialects):Hibernate abstracts database-specific SQL syntax, enabling your application to work with various relational databases without significant code changes. Hibernate allows you to define custom database dialects to adapt to specific database systems.
- Caching:Hibernate offers caching mechanisms (first-level and second-level cache) to improve application performance by reducing the number of database queries.
- Enhanced Fetching Strategies:Hibernate supports various fetching strategies, such as eager and lazy loading, to control how related data is fetched from the database.
- Query Language (HQL):Hibernate Query Language (HQL) allows developers to write database queries using an SQL-like syntax, making it more intuitive and object-oriented.
- Named Queries and Native SQL:Allows developers to define and use named queries for common operations and provides the flexibility to execute native SQL queries when needed.
- Criteria Query:Hibernate provides a Criteria API that allows developers to create type-safe and dynamic queries using a programmatic approach. Criteria queries are particularly useful for building complex queries without writing raw SQL.
- Automatic Dirty Checking:Hibernate employs automatic dirty checking to track changes made to objects in the session. When the session is flushed or a transaction is committed, Hibernate detects changes and generates the necessary SQL statements to update the database, improving data consistency.
- Transaction Management:Hibernate provides built-in transaction management capabilities, simplifying the handling of database transactions and ensuring data integrity.
- Versioning:Hibernate supports versioning of entities, allowing you to keep track of different versions of an object and manage concurrent updates effectively.
- Concurrency Control:Offers mechanisms like optimistic locking and versioning to manage concurrent access to database records and prevent data conflicts.
- Association Mapping:Supports mapping relationships between entities, such as one-to-one, one-to-many, and many-to-many associations.
- Collection Mapping:Allows mapping collections (e.g., lists, sets, maps) of objects to database tables, enabling more complex data structures.
- Connection Pooling Integration:It can be integrated with various connection pooling libraries, enhancing database connection management and efficiency.
- Event Handling:Hibernate provides event listeners and interceptors that allow you to customize and extend its behavior at various points in the object lifecycle, enabling custom logic and validations.
- Batch Processing:Hibernate supports batch processing of SQL statements, which can be more efficient for bulk data operations.
- Security:Supports security measures, such as SQL injection prevention, to enhance the security of database interactions.
What are the different core components of the Hibernate?
Hibernate consists of several core components that form the foundation of its Object-Relational Mapping (ORM) framework. Here are the different core components of Hibernate:
- Configuration:
- The Configuration component is responsible for initializing Hibernate by reading configuration files (typically in XML format) and creating a configuration object that holds various settings, including database connection details, Hibernate dialect, and mapping information.
- SessionFactory:
- The SessionFactory is a critical component in Hibernate. It is responsible for creating and managing Session instances. It is thread-safe and immutable, typically configured during application startup, and serves as a factory for creating Session objects.
- Session:
- The Session is a short-lived, non-thread-safe object representing a single unit of work with the database. It is created from a SessionFactory and used for performing database operations, managing transactions, and caching data during a particular database interaction.
- Transaction Management:
- Hibernate integrates with Java EE or Spring transaction management frameworks to handle database transactions effectively. Transactions ensure data consistency and integrity.
- Entities and Entity Classes:
- Entities are Java objects that represent data in the database. Entity classes are Java classes annotated with Hibernate-specific annotations or configured through XML mappings. They define how Java objects are mapped to database tables and columns.
- Mapping Files and Annotations:
- Mapping files (XML) or annotations (Java) define the mapping between Java entities and database tables. These configurations tell Hibernate how to persist and retrieve data in a relational database.
- Hibernate Query Language (HQL):
- HQL is a powerful query language similar to SQL, designed for querying Java objects rather than database tables. Developers can use HQL to write database queries in an object-oriented manner.
- Criteria API:
- Hibernate provides a Criteria API that allows developers to create type-safe and dynamic queries programmatically. It offers a more structured and object-oriented approach to querying data.
- Caching:
- Hibernate includes caching mechanisms, including first-level and second-level caches. These caches help improve performance by reducing the number of database queries, making data retrieval faster.
- Listeners and Interceptors:
- Hibernate provides listeners and interceptors that allow developers to customize and extend its behavior at various points in the object lifecycle. This can be useful for implementing custom logic, validation, and auditing.
List out some important interfaces of the Hibernate
Here are some important interfaces in the Hibernate framework:
- SessionFactory:
SessionFactory
is a critical interface responsible for creating and managingSession
instances. It’s typically created once per application and serves as a factory for obtainingSession
objects.
- Session:
Session
represents a single unit of work with the database. It provides methods for CRUD operations, querying, and managing transactions.Session
is a short-lived, non-thread-safe object.
- Transaction:
- The
Transaction
interface represents a database transaction. It provides methods to begin, commit, or rollback transactions. Transactions help maintain data integrity during database operations.
- The
- Query:
- The
Query
interface allows you to create and execute HQL (Hibernate Query Language) queries. It provides methods for specifying query parameters, pagination, and result retrieval.
- The
- Criteria:
- The
Criteria
interface offers a programmatic way to create queries using a type-safe and object-oriented approach. It allows you to build queries dynamically based on criteria.
- The
- Configuration:
- The
Configuration
interface is used to configure Hibernate settings, such as database connection properties and dialect. It’s used to initialize Hibernate and create theSessionFactory
.
- The
What is a Hibernate configuration file?
A Hibernate configuration file is a crucial part of configuring Hibernate for a Java application. It typically contains settings and properties required for Hibernate’s operation. In most cases, it’s named hibernate.cfg.xml
and is written in XML format.
Here’s a brief example of a Hibernate configuration file:
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydb</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">rootpassword</property>
<!-- Specify dialect -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQL5Dialect</property>
<!-- Echo all executed SQL to stdout -->
<property name="hibernate.show_sql">true</property>
<!-- Update the database schema if necessary -->
<property name="hibernate.hbm2ddl.auto">update</property>
<!-- Mapping files or annotated classes -->
<mapping class="com.example.model.Employee" />
<mapping class="com.example.model.Department" />
</session-factory>
</hibernate-configuration>
In this example:
- The configuration file starts with the
hibernate-configuration
root element. - Inside the
session-factory
element, you specify various Hibernate properties such as the database connection details, dialect, and whether to show SQL queries. - The
hibernate.hbm2ddl.auto
property is set to “update,” which means Hibernate will automatically update the database schema based on the entity mappings. Be cautious when using this in production, as it may lead to data loss. - The
<mapping>
elements list the entity classes (in this case,Employee
andDepartment
) that Hibernate should manage. These classes can be annotated with Hibernate annotations or configured using separate XML mapping files (.hbm.xml
) referenced here.
The Hibernate configuration file plays a crucial role in initializing Hibernate and specifying how it should interact with the database and manage entity mappings.
What is a Hibernate mapping file?
In Hibernate, a mapping file (often referred to as an HBM file) is used to define the mapping between Java objects (entities) and database tables. These mapping files specify how the properties of a Java class correspond to the columns in a database table. Each entity typically has its own mapping file.
Here’s an example of a Hibernate mapping file (HBM file) for a simple entity class called Employee
that maps to a database table:
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="com.example.model">
<class name="Employee" table="employee">
<id name="id" type="long">
<column name="employee_id" />
<generator class="increment" />
</id>
<property name="firstName" type="string">
<column name="first_name" length="50" not-null="true" />
</property>
<property name="lastName" type="string">
<column name="last_name" length="50" not-null="true" />
</property>
<property name="email" type="string">
<column name="email" length="100" unique="true" not-null="true" />
</property>
<!-- More properties and mappings here -->
</class>
</hibernate-mapping>
In this example:
- The mapping file starts with the
hibernate-mapping
root element. - The
package
attribute in thehibernate-mapping
element specifies the Java package where the entity class is located. - The
<class>
element defines the mapping for theEmployee
entity. It specifies the database table name (employee
) and the Java class name (Employee
). - The
<id>
element defines the primary key (id
) of the entity. It specifies the column name in the database (employee_id
) and the generator strategy (increment
) for generating primary key values. <property>
elements map entity properties (firstName
,lastName
,email
) to table columns. They specify the property name, data type, column name, length, and constraints (e.g.,not-null
,unique
).
This mapping file defines the mapping between the Employee
entity class and the employee
database table. Similar mapping files would be created for other entity classes in your application.
Hibernate Annotations vs Mapping Files
Hibernate offers two ways to define mappings: using annotations (e.g., @Entity
, @Table
, @Column
) within Java classes or using external XML mapping files (HBM files). You can choose either approach or even mix them.
Here’s an example of a Hibernate entity class Employee
with mappings defined using Hibernate annotations:
import javax.persistence.*;
@Entity
@Table(name = "employee")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "employee_id")
private Long id;
@Column(name = "first_name", length = 50, nullable = false)
private String firstName;
@Column(name = "last_name", length = 50, nullable = false)
private String lastName;
@Column(name = "email", length = 100, unique = true, nullable = false)
private String email;
// Constructors, getters, setters, and other properties
}
In this example:
- The
@Entity
annotation indicates that the classEmployee
is a JPA entity, which Hibernate recognizes. - The
@Table
annotation specifies the name of the database table associated with the entity. In this case, it’s named “employee.” - The
@Id
annotation marks theid
field as the primary key of the entity. - The
@GeneratedValue
annotation specifies the strategy for generating primary key values. In this example, it uses the identity strategy. @Column
annotations are used to map class properties to table columns. These annotations specify column names, data types, lengths, and constraints such asnullable
andunique
.
With annotations, you can define entity mappings directly within your Java classes, which can make the code more concise and readable compared to XML mapping files.
What is a dialect in Hibernate?
In Hibernate, a dialect is a configuration setting that defines the specific database you are using, along with its nuances, such as SQL syntax, data types, and query optimization strategies. Hibernate uses dialects to generate database-specific SQL statements and adapt its behavior to work seamlessly with various database systems.
Here are some examples of Hibernate dialects for different databases.
Database System | Hibernate Dialect Class |
---|---|
MySQL | org.hibernate.dialect.MySQLDialect |
PostgreSQL | org.hibernate.dialect.PostgreSQLDialect |
Oracle | org.hibernate.dialect.OracleDialect |
Microsoft SQL Server | org.hibernate.dialect.SQLServerDialect |
HSQL Database | org.hibernate.dialect.HSQLDialect |
H2 Database | org.hibernate.dialect.H2Dialect |
Derby Database | org.hibernate.dialect.DerbyDialect |
MariaDB | org.hibernate.dialect.MariaDBDialect |
SQLite | org.hibernate.dialect.SQLiteDialect |
DB2 | org.hibernate.dialect.DB2Dialect |
Sybase ASE | org.hibernate.dialect.SybaseASEDialect |
Informix | org.hibernate.dialect.InformixDialect |
What is HQL (Hibernate Query Language)?
Hibernate Query Language (HQL) is a powerful and flexible query language used in Hibernate for querying and manipulating data in a relational database using object-oriented syntax. HQL is similar to SQL (Structured Query Language) but is designed to work with Java objects and entity classes instead of database tables and columns.
Here’s an overview of HQL along with an example:
HQL Features:
- HQL allows you to perform CRUD (Create, Read, Update, Delete) operations on entities.
- It supports complex queries, including joins, subqueries, and aggregate functions.
- HQL queries are database-agnostic, meaning you can write queries that work across different database systems without modification.
- HQL provides support for named parameters, which enhance query reusability and security.
HQL Example:
Suppose you have an entity class called Employee
, and you want to retrieve a list of employees with a salary greater than a specified amount. Here’s how you can write an HQL query for this task:
String hql = "SELECT e FROM Employee e WHERE e.salary > :salaryThreshold";
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
try {
Query<Employee> query = session.createQuery(hql, Employee.class);
query.setParameter("salaryThreshold", 50000.0); // Set the named parameter value
List<Employee> employees = query.getResultList();
for (Employee employee : employees) {
System.out.println("Employee Name: " + employee.getFirstName() + " " + employee.getLastName());
}
tx.commit();
} catch (Exception e) {
if (tx != null) {
tx.rollback();
}
e.printStackTrace();
} finally {
session.close();
}
In this example:
- We define an HQL query using the
SELECT
statement to retrieve allEmployee
objects where the salary is greater than a specified threshold. The:salaryThreshold
is a named parameter. - We open a Hibernate
Session
and begin a transaction. - We create an HQL query using
session.createQuery()
, specifying the HQL string and the entity class (Employee.class
). - We set the value for the named parameter
salaryThreshold
usingquery.setParameter()
. - We execute the query using
query.getResultList()
to retrieve a list ofEmployee
objects that meet the criteria. - Finally, we iterate through the result list and print employee names.
This is a basic example, and HQL supports much more complex queries, including joins, projections, and subqueries, making it a versatile tool for querying and manipulating data in Hibernate-based applications.
What is SessionFactory in Hibernate?
SessionFactory is responsible for creating and managing Session instances. It is thread-safe and immutable, typically configured during application startup, and serves as a factory for creating Session objects.
Here’s how you can create a SessionFactory
in Hibernate:
Set up Hibernate Configuration:First, you need to create a Hibernate configuration file (usually named hibernate.cfg.xml
), which contains database connection information, mapping details, and other Hibernate configuration settings. Here’s an example configuration file:
<!-- hibernate.cfg.xml -->
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/your_database</property>
<property name="hibernate.connection.username">your_username</property>
<property name="hibernate.connection.password">your_password</property>
<!-- Hibernate dialect for your database -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- Specify mapping files or annotated classes -->
<mapping resource="com/example/YourEntity.hbm.xml"/>
<!-- Add more mappings if needed -->
<!-- Enable the Hibernate cache -->
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
<!-- Other Hibernate properties -->
</session-factory>
</hibernate-configuration>
Java Code to Create SessionFactory
:
In your Java code, you can create the SessionFactory
using the Configuration
class and the configuration file you created earlier. Here’s an example of how to do this:
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateUtil {
private static final SessionFactory sessionFactory;
static {
try {
// Create the SessionFactory from hibernate.cfg.xml
sessionFactory = new Configuration().configure("hibernate.cfg.xml").buildSessionFactory();
} catch (Throwable ex) {
System.err.println("Initial SessionFactory creation failed. " + ex);
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
What is Session in Hibernate ?
In Hibernate, a Session is a fundamental and integral part of the framework. It represents a single unit of work with the database and serves as an interface between your Java application code and the database. Sessions are used for performing database operations, including CRUD (Create, Read, Update, Delete) operations, querying, and managing transactions.
The Session is a short-lived, non-thread-safe object. It is created from a SessionFactory.
Here’s an example of how to obtain a Session
:
SessionFactory sessionFactory = HibernateUtil.getSessionFactory();
Session session = sessionFactory.openSession();
// Use the session to perform database operations
session.close(); // Don't forget to close the session when you're done
SessionFactory vs Session in Hibernate
Here’s a comparison between SessionFactory
and Session
in Hibernate:
Aspect | SessionFactory | Session |
---|---|---|
Purpose | Manages Hibernate configuration and provides a factory for creating Session instances. | Represents a single unit of work and a conversation between the application and the database. |
Lifecycle | Typically, there is only one SessionFactory per application, and it’s usually created once during application startup and shared throughout the application’s lifetime. | Multiple Session instances can be created and destroyed as needed during the application’s runtime. |
Thread Safety | SessionFactory is thread-safe and can be safely shared among multiple threads in a multi-threaded environment. | Session is not thread-safe, and each thread should have its own Session instance. |
Caching | SessionFactory caches metadata about the mapped entities, such as class-to-table mappings and SQL query plans, which improves performance. | Session manages the first-level cache (also known as the persistence context), which caches loaded entities during its lifespan. |
Database Connection | Manages database connections and connection pooling, ensuring efficient database access. | Represents a single database connection and transaction. |
Entity Operations | Does not perform actual database operations or manage transactions but provides Session instances to perform these operations. | Executes database operations, manages transactions, and provides methods to perform CRUD (Create, Read, Update, Delete) operations on entities. |
Transaction Management | SessionFactory does not directly manage transactions but can be configured to use various transaction management strategies. | Session is responsible for managing transactions, including starting, committing, or rolling back transactions. |
Entity Persistence | Does not directly persist or retrieve entities but delegates these operations to Session instances. | Used to persist, retrieve, update, and delete entities in the database. |
Resource Cleanup | Typically, the SessionFactory is created once and kept open for the application’s entire lifespan. | Each Session should be explicitly closed after use to release database connections and resources. |
Contextual Information | Stateless and does not store contextual information related to a specific unit of work. | Holds contextual information related to a specific unit of work, including loaded entities, pending changes, and transactional state. |
Memory Footprint | Relatively lightweight compared to Session instances, as it primarily manages configuration and metadata. | Potentially heavier in terms of memory usage, as it holds the first-level cache and transactional state. |
Creation Overhead | Creating a SessionFactory can be relatively expensive, so it’s usually created once and reused. | Creating a Session instance is relatively lightweight and can be done as needed. |
Best Practices | Create one SessionFactory for the entire application and reuse it. | Create a new Session for each unit of work (e.g., HTTP request, business operation) and close it when done. |
Differences between getCurrentSession() and openSession() methods of SessionFactory in Hibernate.
Aspect | getCurrentSession() | openSession() |
---|---|---|
Session Creation | Returns the current Session associated with the current context (thread or transaction). If one exists, it’s reused; otherwise, it may create a new Session . | Creates a new Session instance every time it’s called. |
Lifecycle & Management | Managed environment; Hibernate controls Session lifecycle, often tied to the current transaction or thread. You can configure the hibernate.current_session_context_class property to specify the context management strategy. Common values include: thread : Associates the Session with the current thread.jta : Associates the Session with the current JTA (Java Transaction API) transaction. | Manual; you create and manage Session instances explicitly. |
Thread Safety | Thread-safe within the current transaction or thread context. | Not inherently thread-safe; manual management is needed. |
Transaction Handling | Automatic transaction management if configured properly. | Requires manual transaction handling with beginTransaction() , commit() , and rollback() . |
Resource Cleanup | Automatically closed by Hibernate when the context (transaction or thread) ends. | Must be explicitly closed using close() to release resources. |
Use Cases | Suited for declarative transaction management in managed environments (e.g., Java EE, Spring). | Provides fine-grained control over Session lifecycle, often used in standalone applications or when explicit transaction control is needed. |
What are different Collection Types in Hibernate?
Hibernate provides various collection types to map associations between entities and collections of values. These collection types enable you to model relationships between entities in a more object-oriented manner. Here are some of the commonly used collection types in Hibernate:
- Set: A
Set
collection represents an unordered collection of elements where each element must be unique. In Hibernate, you can use aSet
to represent a one-to-many or many-to-many relationship between entities. - List: A
List
collection represents an ordered collection of elements where duplicate elements are allowed. In Hibernate, you can use aList
to represent a one-to-many relationship where the order of elements matters. - Bag: A
Bag
is similar to aList
but without the constraint of maintaining element order. It allows duplicate elements. In Hibernate, you can use aBag
for one-to-many associations when the order of elements doesn’t matter. - Map: A
Map
collection represents a collection of key-value pairs where each key is unique. In Hibernate, you can use aMap
to represent a one-to-many or many-to-many relationship where you want to associate entities with keys. - Array: An
Array
collection represents an ordered collection of elements similar to a Java array. In Hibernate, you can use anArray
for one-to-many associations, but it’s less commonly used compared to other collection types. - Primitive Arrays: Hibernate supports primitive arrays (e.g.,
int[]
,double[]
) as collection types to map to columns in the database. - SortedSet, SortedMap: These are variations of
Set
andMap
collections that maintain elements in a sorted order based on a comparator or natural ordering of elements. - Immutable Collections: Hibernate supports immutable collection types, which are read-only and do not allow modifications after initialization. This is useful for mapping read-only associations.
- Custom Collection Types: You can define your custom collection types by implementing the
org.hibernate.usertype.UserCollectionType
interface to handle complex collection mapping scenarios.
Differences between Sorted and Ordered Collections in Hibernate
Aspect | Sorted Collections | Ordered Collections |
---|---|---|
Interface | java.util.SortedSet or java.util.SortedMap | java.util.List |
Ordering Strategy | Automatically sorts elements based on a specified comparator or natural ordering. | Maintains elements in the order in which they were added. |
Duplicate Elements | Does not allow duplicate elements. | Allows duplicate elements and preserves their order. |
Hibernate Mapping | Typically specified as a SortedSet or SortedMap in Hibernate mapping. | Typically specified as a List in Hibernate mapping. |
Use Cases | Useful when elements need to be sorted automatically based on a specific criterion (e.g., alphabetical order, numeric order). | Suitable when you need to maintain elements in a specific order and may have duplicate elements. |
List Important Methods of Session Class in Hibernate
Here are the important methods of the Session
class in Hibernate:
Method | Description |
---|---|
beginTransaction() | Begins a new database transaction. |
beginTransaction(IsolationLevel level) | Begins a transaction with the specified isolation level. |
clear() | Clears the session, detaching all objects. |
close() | Closes the session, releasing resources. |
createSQLQuery(String sql) | Creates a native SQL query. |
createQuery(String hql) | Creates an HQL (Hibernate Query Language) query. |
delete(Object obj) | Deletes an object from the database. |
disconnect() | Disconnects the session from the database. |
doWork(Work work) | Executes a database-related work unit. |
evict(Object obj) | Detaches an object from the session. |
flush() | Synchronizes the session’s state with the database. |
get(Class<?> clazz, Serializable id) | Retrieves an object by its primary key. |
getTransaction() | Retrieves the current transaction or null if none is active. |
isConnected() | Checks if the session is connected to the database. |
isDirty() | Checks if the session contains any dirty (modified) objects. |
load(Class<?> clazz, Serializable id) | Lazy-loads an object by its primary key. |
lock(Object obj, LockMode lockMode) | Locks an object with the specified lock mode. |
merge(Object obj) | Merges the state of a detached object with the current session. |
open() | Opens the session if it is closed. |
persist(Object obj) | Makes a transient instance persistent. |
refresh(Object obj) | Refreshes an object’s state from the database. |
reconnect() | Reconnects the session to the database. |
save(Object obj) | Saves an object to the database. |
saveOrUpdate(Object obj) | Saves or updates an object based on its existence in the database. |
update(Object obj) | Updates an object in the database. |
Differences between save() and persist() methods of Session Object
Aspect | save() | persist() |
---|---|---|
Operation | Inserts the provided entity into the database. | Make a transient instance persistent. |
Return Type | Returns generated id and return type is serializable. | Returns void |
Database Interaction | May execute an INSERT statement immediately to persist the entity in the database, potentially within the current transaction boundary. | Does not guarantee an immediate database INSERT. The actual INSERT may occur at a later time, during a flush or transaction commit, and is often within the current transaction boundary. |
Suitability for Detached Entities | Can be used with detached entities. | Not suitable for detached entities |
Transaction Boundaries | It can save object within transaction boundaries and outside boundaries. | It can only save object within the transaction boundaries. |
Use Cases | Commonly used when you want to insert a new entity into the database and retrieve the generated primary key or when dealing with detached entities within the current transaction boundary. | Often used when you want to make a transient entity persistent and manage it within the session, but you are not concerned about the immediate database INSERT within the current transaction boundary. |
Differentiate between save() and saveOrUpdate() methods in the Hibernate Session
Aspect | save() | saveOrUpdate() |
---|---|---|
Use with New Entities | Used for brand new entities not associated with the session. | Works for both new entities and those already associated with the session. |
Database Interaction | Inserts the entity into the database. | Inserts if new, updates if already exists. |
Return Type | Returns the generated identifier. | Returns nothing (void ). |
Use Cases | For new entities you want to save and retrieve their primary keys. | For both new and existing entities, handles inserts and updates as needed. |
What are the differences between update() and merge() methods in Hibernate?
Aspect | update() | merge() |
---|---|---|
Return Type | Does not return anything (void ). | Returns a reference to the managed entity after the merge operation. |
Existing Object in Session | update() method can not be used when the same object already exists in the session | merge() can be used when the same object exists in the session. |
Use Cases | If you are sure that the session does not contains an already persistent instance with the same identifier, then use update to save the data in hibernate. | If you want to save your modifications at any time with out knowing about the state of an session, then use merge(). |
Differences between get() and load() methods in Hibernate
Aspect | get() | load() |
---|---|---|
Loading Entity | Loads the entity from the database immediately when called. | Loads a proxy or placeholder for the entity, and the actual loading occurs only when you access its properties. |
Database Interaction | Executes a SELECT query to load the entity immediately. | Generates a proxy object and delays the SELECT query until you access the entity’s data. |
Return Type | Returns the actual entity object or null if not found. | Returns a proxy object of the entity without hitting the database initially. |
Lazy Loading | Does not support lazy loading; loads the entire entity immediately. | Supports lazy loading; loads data from the database only when you access the entity’s properties. |
Exception on Not Found | Does not throw an exception if the entity is not found; returns null . | Throws an exception (ObjectNotFoundException) when you access the proxy and the entity is not found in the database. |
Use Cases | Suitable when you need to load an entity and work with its data immediately. | Useful when you want to minimize database queries and load an entity only when needed, potentially reducing overhead. |
How to enable SQL query logs in Java Hibernate Applications?
Before enabling SQL query logging, you need to set up a logging framework in your Java application. Popular logging frameworks like Log4j, Logback etc.
Configure Hibernate to use the same logging framework as your application. In your Hibernate configuration file (e.g., hibernate.cfg.xml
), specify the logging settings. Here’s an example using Log4j as the logging framework:
<property name="hibernate.show_sql">true</property>
<property name="hibernate.format_sql">true</property>
<property name="hibernate.use_sql_comments">true</property>
hibernate.show_sql
: Set this property totrue
to enable SQL query logging to the console.hibernate.format_sql
: Set this property totrue
to format SQL queries for better readability.hibernate.use_sql_comments
: Set this property totrue
to include comments in SQL queries with additional information.
Intermediate Level : Hibernate Interview Questions
Now, let’s explore Intermediate level of Hibernate interview questions.
What is the use of lock() method in Session Object?
The lock()
method in Session is used to explicitly obtain a database lock on a specific entity, which can be helpful in controlling concurrent access and managing transactions.
Here’s how the lock()
method works:
- Locking Mode: You specify the locking mode, which determines the type of lock to acquire. Hibernate supports various locking modes, such as
LockMode.READ
(for shared read locks) andLockMode.UPGRADE
(for exclusive write locks), among others. - Entity: You provide the entity object you want to lock.
- Lock Scope: Optionally, you can specify the scope of the lock, which may include a specific lock timeout or lock scope (e.g., Pessimistic vs. Optimistic locking).
- Transaction Control: Typically, you use
lock()
within an active transaction. The lock will be held until the transaction is committed or rolled back, ensuring that the database row remains locked for the duration of the transaction.
Usage scenarios for lock()
include:
- Pessimistic Locking: Ensuring exclusive access to an entity for updates to prevent concurrent modifications. For example, when two users try to edit the same data simultaneously, you can use
lock()
method to serialize access. - Optimistic Locking: Implementing a version-based locking mechanism where you acquire a lock only when you are about to update an entity. This helps prevent lost updates and conflicts when multiple users work with the same data.
- Batch Processing: When processing a large number of records in batch operations, you might use
lock()
to ensure that records are processed sequentially to avoid conflicts.
What is Java Persistence API (JPA)?
JPA is a specification that defines a standard API for ORM in Java. It provides a standardized way to interact with relational databases, enabling developers to work with database entities using Java objects and annotations.
While JPA is a specification, there are multiple third-party implementations available, such as Hibernate, EclipseLink, and OpenJPA, that provide the actual ORM functionality.
JPA vs Hibernate
Here’s a comparison of JPA and Hibernate:
Aspect | JPA | Hibernate |
---|---|---|
Definition | JPA is a specification. | Hibernate is an ORM framework that implements the JPA specification. |
Flexibility | JPA is a standard and provides a common API for different providers, making it flexible to switch providers. | Hibernate provides more advanced features and fine-grained control over ORM, making it less flexible when switching to another JPA provider. |
Performance Tuning | JPA provides basic performance tuning capabilities, but some advanced optimizations may require provider-specific configurations. | Hibernate offers a wide range of performance-tuning options and optimizations specific to Hibernate features. |
Vendor Lock-In | JPA aims to reduce vendor lock-in by providing a standard API. | Hibernate, while implementing JPA, may introduce some level of vendor lock-in due to its additional features. |
Ecosystem Integration | JPA can be easily integrated with other Java EE technologies like EJB, CDI, and JTA. | Hibernate can also be integrated with other Java EE technologies, but it might require more configuration. |
Extra Features | JPA provides a standardized set of features. Additional features depend on the specific JPA provider. | Hibernate offers a wide array of additional features beyond the JPA standard, such as caching strategies, custom types, and advanced querying options. |
Read More : JPA vs Hibernate: Comprehensive Comparison(2023)
Spring Data JPA vs Hibernate
Aspect | Spring Data JPA | Hibernate |
---|---|---|
Purpose | Spring Data JPA is a part of the larger Spring Data project and provides a simplified data access layer on top of JPA (Java Persistence API). It focuses on simplifying common data access tasks. | Hibernate is a standalone Object-Relational Mapping (ORM) framework that provides a comprehensive solution for mapping Java objects to relational databases. |
Integration with Spring | Spring Data JPA is tightly integrated with the Spring Framework, making it easy to work with Spring components like Dependency Injection and AOP (Aspect-Oriented Programming). | Hibernate can be integrated with Spring, but it requires more configuration and doesn’t have the same level of integration as Spring Data JPA. |
Repository Pattern | Spring Data JPA encourages the use of the Repository pattern, where you define interfaces that extend JpaRepository or CrudRepository to perform database operations with minimal code. | Hibernate does not provide a built-in Repository pattern, so you have to write more custom data access code. |
Query Methods | Spring Data JPA allows you to create repository methods by simply defining method names using a specific naming convention. It generates SQL queries based on method names. | Hibernate provides more control over query construction using HQL (Hibernate Query Language) or native SQL queries. |
Flexibility | Spring Data JPA abstracts some of the complexities of JPA, which can be beneficial for simple use cases but might limit flexibility for complex queries and mappings. | Hibernate offers more fine-grained control and flexibility, making it suitable for complex scenarios. |
Portability | Spring Data JPA is built on top of JPA, making it relatively portable across different JPA providers. You can switch JPA providers (e.g., from Hibernate to EclipseLink) with minimal code changes. | Hibernate is tightly coupled with its own ORM implementation, which might make switching to another JPA provider more challenging. |
Learning Curve | Spring Data JPA aims to simplify data access, so it may have a lower learning curve, especially for developers familiar with the Spring ecosystem. | Hibernate provides a wide range of features and can have a steeper learning curve due to its complexity. |
Additional Features | Spring Data JPA primarily focuses on simplifying common data access tasks, whereas Hibernate offers a broader set of features, including caching strategies, custom types, and advanced querying options. | Hibernate provides more extensive and advanced features beyond the JPA standard, making it a powerful choice for complex database applications. |
What are different Hibernate Annotations?
Here are some of the most commonly used Hibernate annotations:
- @Entity:
- Marks a class as an entity, representing a database table.
- Used to specify that an instance of the class can be persisted to the database.
- @Table:
- Defines the database table to which an entity is mapped.
- Allows you to specify the table name, schema, and other table-related properties.
- @Id:
- Specifies the primary key property of an entity.
- Used to mark a field or property as the unique identifier for the entity.
- @GeneratedValue:
- Configures how primary key values are generated for an entity.
- Commonly used with
@Id
to specify automatic generation strategies likeIDENTITY
orSEQUENCE
.
- @Column:
- Maps an entity attribute (field or property) to a database column.
- Allows you to specify column name, type, length, and other column properties.
- @OneToMany:
- Defines a one-to-many association between entities.
- Used to specify that an entity has a collection of related entities.
- @ManyToOne:
- Defines a many-to-one association between entities.
- Specifies that an entity has a single association to another entity.
- @JoinColumn:
- Specifies the column used for joining an entity association.
- Commonly used with
@ManyToOne
and@OneToOne
to customize the join column name.
- @OneToOne:
- Defines a one-to-one association between entities.
- Specifies that an entity has a single association to another entity.
- @ManyToMany:
- Defines a many-to-many association between entities.
- Used to specify that an entity has a collection of related entities, and vice versa.
- @JoinTable:
- Specifies the join table used in a many-to-many association.
- Allows you to customize the name of the join table and the columns used for the association.
- @Cascade:
- Specifies cascade operations to be applied to associated entities.
- Defines which operations (e.g., persist, merge, delete) should be propagated to associated entities.
- @Fetch:
- Configures the fetching strategy for an association.
- Determines whether associated data should be loaded eagerly or lazily.
- @Transient:
- Marks a field or property as non-persistent, indicating that it should not be mapped to a database column.
- Useful for fields that should be excluded from database persistence.
- @Version:
- Marks a field as a version attribute used for optimistic locking.
- Allows Hibernate to check for concurrent updates when updating an entity.
What are the different States of an Entity in Hibernate?
In Hibernate, entities go through different states during their lifecycle as they are managed by the Hibernate framework. These states are often referred to as the “lifecycle states” of an entity. The primary lifecycle states of a Hibernate entity are as follows:
- Transient State:
- In this state, the entity is not associated with any Hibernate Session (Persistence Context).
- It does not have a corresponding database record.
- It is typically a newly created object using the
new
operator in Java.
- Persistent State:
- In this state, the entity is associated with a Hibernate Session (Persistence Context). You associate the entity with a Hibernate Session by using methods like
session.save()
orsession.persist()
. - It has a corresponding database record.
- Any changes made to the entity while in this state are tracked by Hibernate and can be synchronized with the database during the session’s flush or transaction commit.
- In this state, the entity is associated with a Hibernate Session (Persistence Context). You associate the entity with a Hibernate Session by using methods like
- Detached State:
- In this state, the entity was once associated with a Hibernate Session but is no longer. You close the Hibernate Session, or the entity is explicitly detached using methods like
session.detach()
. - It may still have a corresponding database record, but it is no longer managed by Hibernate.
- Changes made to a detached entity are not automatically synchronized with the database.
- In this state, the entity was once associated with a Hibernate Session but is no longer. You close the Hibernate Session, or the entity is explicitly detached using methods like
- Removed (Deleted) State:
- In this state, the entity was associated with a Hibernate Session and has been marked for deletion. You mark the entity for removal using
session.delete()
. - It will be deleted from the database during the session’s flush or transaction commit.
- In this state, the entity was associated with a Hibernate Session and has been marked for deletion. You mark the entity for removal using
What are the various types of Caching in Hibernate?
Hibernate provides several caching mechanisms to improve the performance of database operations by reducing the need to repeatedly fetch data from the database. These caching mechanisms help Hibernate store and retrieve data efficiently. The various types of caching in Hibernate include:
- First-Level Cache (Session Cache):
- Also known as the session cache, it is the most basic level of caching in Hibernate.
- It is associated with a Hibernate
Session
. - It stores the entities (objects) that have been queried or loaded during the current session.
- It ensures that multiple requests for the same entity within a session result in a single database query.
- Second-Level Cache:
- The second-level cache is a shared cache that spans multiple sessions within the same application.
- It stores data at the granularity of entities, collections, and queries.
- It can be configured with various cache providers, such as Ehcache, Infinispan, or Hazelcast.
- It helps reduce database load and improves performance for frequently accessed data.
- Hibernate entities can be marked as cacheable using annotations or XML configurations.
- Query Cache:
- The query cache stores the results of queries and their associated data, allowing Hibernate to avoid re-executing the same queries with the same parameters.
- It can be used in conjunction with the second-level cache for further performance improvement.
- Query caching is especially useful for read-heavy applications where the same queries are executed frequently.
What are the differences between first-level cache and second-level cache in Hibernate?
Aspect | First-Level Cache (Session Cache) | Second-Level Cache |
---|---|---|
Scope | Associated with a single Hibernate Session . | Shared across multiple Hibernate sessions in the same application. |
Data Granularity | Caches individual objects (entities) loaded or queried in a session. | Caches entities, collections, and query results at a higher level of granularity. |
Lifetime | Short-lived, tied to the lifecycle of the session. Cleared when the session is closed or objects are detached. | Long-lived, can persist data across multiple sessions and even application restarts. |
Concurrency | Not suitable for concurrent access by multiple sessions or threads within the same session. | Can be safely accessed by multiple threads and sessions concurrently. Supports multi-user scenarios. |
Customization | Limited configurability; managed automatically by Hibernate. | Highly configurable, with options for cache providers, eviction policies, and cache regions. |
Use Cases | Managing object state within a single session to reduce database queries for the same objects during that session. | Caching shared data across sessions or users, improving database performance and application scalability. |
What is Query Cache in Hibernate?
The Query Cache in Hibernate is a caching mechanism that stores the results of queries and their associated data. It is used to avoid re-executing the same queries with the same parameters, which can improve the performance of read-heavy applications. The Query Cache works in conjunction with the second-level cache to cache query results.
Here’s a code example of how to enable and use the Query Cache in Hibernate:
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
import org.hibernate.Query;
public class HibernateQueryCacheExample {
public static void main(String[] args) {
// Create a Hibernate configuration and set up a SessionFactory
Configuration configuration = new Configuration();
configuration.configure("hibernate.cfg.xml"); // Load Hibernate configuration file
SessionFactory sessionFactory = configuration.buildSessionFactory();
// Create a Hibernate Session
Session session = sessionFactory.openSession();
try {
// Enable the Query Cache for a specific query
Query query = session.createQuery("FROM Employee WHERE department = :dept");
query.setParameter("dept", "HR");
query.setCacheable(true); // Enable the Query Cache for this query
// Perform the query
List<Employee> employees = query.list();
// Subsequent executions of the same query with the same parameters will use the cache
List<Employee> cachedEmployees = query.list();
// Commit the transaction
Transaction transaction = session.beginTransaction();
transaction.commit();
// Close the session
session.close();
// The second execution of the query will use the Query Cache
// and won't generate a new SQL query
} catch (Exception e) {
e.printStackTrace();
session.getTransaction().rollback();
} finally {
if (session.isOpen()) {
session.close();
}
sessionFactory.close();
}
}
}
In this example:
- We configure Hibernate using a configuration file (hibernate.cfg.xml) and create a
SessionFactory
. - We open a Hibernate
Session
. - We create a query to retrieve employees from the “HR” department. We enable the Query Cache for this query using
query.setCacheable(true)
. - We execute the query using
query.list()
to retrieve the employees. - Subsequent executions of the same query with the same parameters (e.g.,
query.list()
) will use the Query Cache, avoiding a new database query. - We commit the transaction and close the session.
By enabling the Query Cache, Hibernate stores the query results and their associated data in the cache, which can significantly improve query performance, especially for frequently executed queries with the same parameters.
What is Criteria Query in Hibernate?
In Hibernate, Criteria Query is a programmatic and type-safe way to construct queries using a set of Java API classes instead of writing HQL (Hibernate Query Language) or native SQL queries as strings. Criteria Query allows you to build queries using a fluent API and is often preferred when you need to create dynamic queries or perform complex searches on your data.
Here’s an example of how to use Criteria Query in Hibernate:
Assuming you have an entity class named Product
that represents products in a database:
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "id")
private Long id;
@Column(name = "name")
private String name;
@Column(name = "price")
private BigDecimal price;
// Getters and setters
}
Now, let’s create a Criteria Query to retrieve all products with a price greater than a certain threshold:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
import org.hibernate.Criteria;
import org.hibernate.criterion.Restrictions;
import java.util.List;
public class CriteriaQueryExample {
public static void main(String[] args) {
// Create a Hibernate configuration and build a session factory
Configuration configuration = new Configuration().configure();
SessionFactory sessionFactory = configuration.buildSessionFactory();
// Open a session
Session session = sessionFactory.openSession();
// Begin a transaction
Transaction transaction = session.beginTransaction();
// Create a Criteria instance for the Product class
Criteria criteria = session.createCriteria(Product.class);
// Add a restriction to filter products with a price greater than 50.00
criteria.add(Restrictions.gt("price", new BigDecimal("50.00")));
// Execute the query and get the results as a list of products
List<Product> products = criteria.list();
// Display the products
for (Product product : products) {
System.out.println("Product Name: " + product.getName() + ", Price: " + product.getPrice());
}
// Commit the transaction and close the session
transaction.commit();
session.close();
// Close the session factory
sessionFactory.close();
}
}
In this example, we first create a Criteria
instance for the Product
class and then use the Restrictions.gt
method to add a condition that filters products with a price greater than 50.00. Finally, we execute the query and retrieve the results as a list of Product
objects.
Criteria Query provides a flexible way to construct queries using a programmatic approach and is particularly useful when you need to build dynamic queries based on user input or other runtime conditions.
What is a Named SQL query in Hibernate?
In Hibernate, a Named SQL Query is a pre-defined SQL query that is associated with a name and stored in a mapping file or annotated within an entity class. Named SQL queries are typically used when you need to execute complex SQL queries that cannot be easily expressed using HQL (Hibernate Query Language) or Criteria API. They provide a way to encapsulate SQL queries and make them more maintainable and reusable.
Here’s an example of how to define and use a Named SQL Query in Hibernate:
- Define the Named SQL Query in a Hibernate XML mapping file (e.g.,
Product.hbm.xml
) or using annotations:
Hibernate XML Mapping File (product.hbm.xml):
<hibernate-mapping>
<sql-query name="findProductsByCategory">
<![CDATA[
SELECT * FROM products
WHERE category = :category
]]>
</sql-query>
</hibernate-mapping>
OR
Using Annotations (inside an entity class):
import org.hibernate.annotations.NamedNativeQuery;
import org.hibernate.annotations.NamedQuery;
@Entity
@NamedNativeQuery(
name = "findProductsByCategory",
query = "SELECT * FROM products WHERE category = :category",
resultClass = Product.class
)
public class Product {
// Entity fields, getters, and setters
}
- Use the Named SQL Query in your Java code:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
public class NamedSQLQueryExample {
public static void main(String[] args) {
// Create a Hibernate configuration and build a session factory
Configuration configuration = new Configuration().configure();
SessionFactory sessionFactory = configuration.buildSessionFactory();
// Open a session
Session session = sessionFactory.openSession();
// Begin a transaction
Transaction transaction = session.beginTransaction();
// Define the value for the named parameter
String category = "Electronics";
// Execute the Named SQL Query
List<Product> products = session.getNamedQuery("findProductsByCategory")
.setParameter("category", category)
.list();
// Display the products
for (Product product : products) {
System.out.println("Product Name: " + product.getName() + ", Category: " + product.getCategory());
}
// Commit the transaction and close the session
transaction.commit();
session.close();
// Close the session factory
sessionFactory.close();
}
}
In this example, we defined a Named SQL Query named “findProductsByCategory” that retrieves products based on a category. We then use session.getNamedQuery("findProductsByCategory")
to retrieve the named query and set its parameter before executing it.
Named SQL Queries are particularly useful when you need to execute complex SQL statements, especially when HQL or Criteria API is insufficient for expressing the query logic. They help centralize query definitions and make it easier to maintain and modify queries as your application evolves.
How to use Native Query in Hibernate?
In Hibernate, you can use native SQL queries to execute SQL statements directly against the database. This can be useful when you need to perform complex queries or operations that are not easily achievable using HQL (Hibernate Query Language) or Criteria API. Here’s how you can use native SQL queries in Hibernate with an example:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.query.NativeQuery;
import org.hibernate.cfg.Configuration;
import java.util.List;
public class NativeQueryExample {
public static void main(String[] args) {
// Create Hibernate Configuration and SessionFactory
Configuration config = new Configuration().configure("hibernate.cfg.xml");
SessionFactory sessionFactory = config.buildSessionFactory();
// Open a Hibernate Session
Session session = sessionFactory.openSession();
try {
// Define your native SQL query
String sqlQuery = "SELECT * FROM your_table WHERE some_column = :param";
// Create a Native SQL Query
NativeQuery<MyEntity> nativeQuery = session.createNativeQuery(sqlQuery, MyEntity.class);
nativeQuery.setParameter("param", someValue);
// Execute the query and retrieve results
List<MyEntity> result = nativeQuery.getResultList();
// Process the results
for (MyEntity entity : result) {
System.out.println(entity.toString());
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// Close the Hibernate Session and SessionFactory
session.close();
sessionFactory.close();
}
}
}
What is Lazy Loading in Hibernate?
Lazy loading is a technique used in Hibernate (and other object-relational mapping frameworks) to improve application performance and manage database access more efficiently. It’s a feature that allows you to delay the loading of certain associations or attributes of an entity until the data is actually needed, rather than fetching all related data eagerly when the entity is initially loaded. Lazy loading is particularly useful when dealing with large object graphs or when optimizing database access.
In Hibernate, lazy loading is typically applied to associations between entities. There are two main types of lazy loading for associations:
Lazy Loading of Collections: When an entity has a collection of related entities (e.g., a one-to-many or many-to-many relationship), you can configure Hibernate to load that collection lazily. This means that the related entities are not fetched from the database until you explicitly access the collection in your code. Lazy loading of collections can help reduce the amount of data loaded from the database and improve performance.
Example in Hibernate mapping XML:
<set name="items" lazy="true">
<!-- Mapping for the collection -->
</set>
Example in JPA annotations:
@OneToMany(mappedBy = "parentEntity", fetch = FetchType.LAZY)
private Set<ChildEntity> items;
Lazy Loading of Attributes: In addition to collections, you can also apply lazy loading to individual attributes within an entity. This is useful when you have large or rarely used attributes that you want to load only when necessary.
Example in Hibernate mapping XML:
<property name="largeAttribute" lazy="true">
<!-- Mapping for the attribute -->
</property>
Example in JPA annotations:
@Basic(fetch = FetchType.LAZY)
@Column(name = "large_attribute")
private String largeAttribute;
It’s important to note that lazy loading can lead to issues like the “LazyInitializationException” if you try to access a lazy-loaded association or attribute outside of an active Hibernate session. To avoid such exceptions, you should make sure that the session is still open when accessing lazy-loaded data or consider using techniques like eager loading (fetching data eagerly at the time of the initial query) or DTO (Data Transfer Object) projections to fetch only the required data when needed.
What is Automatic Dirty Checking in Hibernate?
Automatic dirty checking in Hibernate is a mechanism that tracks changes made to persistent entities and automatically synchronizes those changes with the database during the transaction commit. It helps ensure that modifications to an entity’s state are reflected in the database without the need for explicit update statements. This feature simplifies the persistence process by eliminating the need for manual tracking and updating of changes.
Here’s an example of how automatic dirty checking works in Hibernate:
Suppose you have a Product
entity:
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Getters and setters
}
And you want to update the price of a product:
// Open a Hibernate Session
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
// Load a product from the database
Product product = session.get(Product.class, 1L);
// Modify the product's price
product.setPrice(29.99);
// The changes are automatically tracked by Hibernate
// No explicit update statement is needed
// Commit the transaction
transaction.commit();
// Close the session
session.close();
In this example:
- We open a Hibernate session and begin a transaction.
- We load a
Product
entity from the database with the ID1L
. - We modify the
price
attribute of the loadedProduct
entity. - We do not explicitly call
session.update(product)
to save the changes. Instead, Hibernate automatically detects that theproduct
entity has been modified within the transaction and generates the necessary SQL update statement to persist the changes when we commit the transaction. - We commit the transaction, and Hibernate flushes the changes to the database.
Can you declare an Entity class as final in Hibernate?
Declaring a Hibernate entity class as final
is allowed, but it can have implications, especially when it comes to performance optimizations like lazy loading.
Hibernate often uses proxies to handle lazy loading of associations. Proxies are dynamically generated subclasses of your entity classes. If you declare an entity class as final
, Hibernate cannot create a proxy for it, which may affect lazy loading and, in some cases, can lead to additional database queries when accessing associations.
So, while it’s possible to make an entity class final
, it’s generally not recommended because it can hinder some of Hibernate’s performance optimizations. It’s often better to leave entity classes open for extension unless you have a specific reason to make them final
and are aware of the potential consequences.
How can you make an immutable class in Hibernate?
In Hibernate, you can enforce immutability for an entity class by using the mutable
attribute in your Hibernate mapping (XML or annotations). The mutable
attribute is set to false
to indicate that instances of the entity should be treated as immutable. When you set mutable="false"
, Hibernate will not generate SQL UPDATE statements for that entity, effectively marking it as immutable.
Here’s how you can use the mutable
attribute in Hibernate mapping files (XML) for an entity:
<class name="com.example.ImmutableProduct" table="products" mutable="false">
<id name="id" type="java.lang.Long">
<column name="id" />
<generator class="native" />
</id>
<property name="name" type="string">
<column name="name" length="255" />
</property>
<property name="price" type="double">
<column name="price" />
</property>
</class>
In the example above, we’ve set mutable="false"
for the ImmutableProduct
entity, indicating that instances of this entity are considered immutable. Hibernate will not generate UPDATE statements for this entity when you modify its state.
Alternatively, if you’re using JPA annotations, you can use the @Immutable
annotation to achieve the same effect:
@Entity
@Immutable
@Table(name = "products")
public class ImmutableProduct {
// ... entity mapping
}
By setting @Immutable
, you’re indicating that the entity is immutable, and Hibernate will not generate UPDATE statements for it.
How do you configure Composite Primary Key in Hibernate?
In Hibernate, you can configure a composite primary key using one of two approaches: using @Embeddable
and @EmbeddedId
or using @IdClass
. Both approaches allow you to define a primary key composed of multiple columns. Below, I’ll explain each approach with examples:
1. Using @Embeddable
and @EmbeddedId
:
- Define a separate class (an
@Embeddable
class) to represent the composite primary key. - In your entity class, annotate a field of the
@Embeddable
class type with@EmbeddedId
. - Annotate the fields within the
@Embeddable
class with@Column
to map them to the database columns.
Example:
@Entity
public class OrderItem {
@EmbeddedId
private OrderItemId id;
// Other fields
// Getters and setters
}
@Embeddable
public class OrderItemId implements Serializable {
@Column(name = "order_id")
private Long orderId;
@Column(name = "product_id")
private Long productId;
// Getters and setters
}
2. Using @IdClass
:
- Define a separate class (an
@Entity
class) to represent the composite primary key. - In your entity class, annotate fields with
@Id
to indicate they are part of the composite primary key. - Associate the entity class with the primary key class using
@IdClass
.
Example:
@Entity
@IdClass(OrderItemId.class)
public class OrderItem {
@Id
@Column(name = "order_id")
private Long orderId;
@Id
@Column(name = "product_id")
private Long productId;
// Other fields
// Getters and setters
}
public class OrderItemId implements Serializable {
private Long orderId;
private Long productId;
// Getters and setters
}
What happens when no-args constructor is absent in the Entity in Hibernate?
Hibernate relies on the presence of a default constructor for various purposes, such as creating instances of entities when retrieving data from the database or creating new entities for persistence.
Without a no-args constructor, Hibernate won’t be able to instantiate the entity, resulting in runtime exceptions, typically a java.lang.InstantiationException
or org.hibernate.InstantiationException
.
Additionally, Hibernate may generate proxy classes for lazy loading or dynamic subclassing, which also require a default constructor.
To avoid these issues and ensure proper functioning with Hibernate, it is considered a best practice to provide a public no-args constructor in your entity classes, even if you have other constructors with arguments. This ensures that Hibernate can create instances of the entity when needed and prevents unexpected exceptions related to instantiation problems.
For example:
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
// No-args constructor is essential for Hibernate
public Employee() {
}
// Constructor with arguments
public Employee(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
// Getters and setters
}
Advanced Level : Hibernate Interview Questions
Now, let’s discuss some advanced level of Hibernate Interview Questions.
What are different types of associations between Entities?
In Hibernate (and JPA), there are several types of associations that define the relationships between entities. These associations determine how entities are related to each other in the database. Here are the main types of associations with small examples for each:
One-to-One (1:1) Association:
- In a one-to-one association, one entity is associated with exactly one other entity.Example:
Person
has a one-to-one relationship withPassport
.
@Entity
public class Person {
@Id
@GeneratedValue
private Long id;
private String name;
@OneToOne(mappedBy = "person")
private Passport passport;
// Getters and setters
}
@Entity
public class Passport {
@Id
@GeneratedValue
private Long id;
private String number;
@OneToOne
@JoinColumn(name = "person_id")
private Person person;
// Getters and setters
}
One-to-Many (1:N) Association:
- Represents a relationship where one entity is associated with a collection of other entities.
- Example:
Department
has a one-to-many relationship withEmployee
.
@Entity
public class Department {
@Id
@GeneratedValue
private Long id;
private String name;
@OneToMany(mappedBy = "department")
private List<Employee> employees;
// Getters and setters
}
@Entity
public class Employee {
@Id
@GeneratedValue
private Long id;
private String name;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
// Getters and setters
}
Many-to-One (N:1) Association:
- Represents a relationship where many entities are associated with one other entity.
- Example:
Employee
has a many-to-one relationship withDepartment
.
@Entity
public class Employee {
@Id
@GeneratedValue
private Long id;
private String name;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
// Getters and setters
}
@Entity
public class Department {
@Id
@GeneratedValue
private Long id;
private String name;
@OneToMany(mappedBy = "department")
private List<Employee> employees;
// Getters and setters
}
Many-to-Many (N:N) Association:
- In a many-to-many association, multiple instances of one entity can be associated with multiple instances of another entity.
- Example:
Student
has a many-to-many relationship withCourse
.
@Entity
public class Student {
@Id
@GeneratedValue
private Long id;
private String name;
@ManyToMany
@JoinTable(
name = "student_course",
joinColumns = @JoinColumn(name = "student_id"),
inverseJoinColumns = @JoinColumn(name = "course_id")
)
private List<Course> courses;
// Getters and setters
}
@Entity
public class Course {
@Id
@GeneratedValue
private Long id;
private String name;
@ManyToMany(mappedBy = "courses")
private List<Student> students;
// Getters and setters
}
What are different strategies to generate primary keys in Hibernate?
In Hibernate, primary key generation strategies determine how the primary key values for entities are generated and assigned when inserting new records into a database table. Hibernate provides various strategies for generating primary keys. Here are some of the most commonly used strategies:
GenerationType.AUTO (Default):
- The default strategy in JPA.It delegates the choice of the primary key generation strategy to the underlying database system.The actual strategy used depends on the database and JDBC driver in use.
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
GenerationType.IDENTITY (Auto-increment):
- Utilizes an auto-incremented (or identity) column in the database.Typically used with databases that support auto-incremented columns, such as MySQL, PostgreSQL, and SQL Server.
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
GenerationType.SEQUENCE (Database Sequence):
- Uses a database sequence to generate primary key values.Suitable for databases like Oracle that support sequences.
@Id
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "my_seq")
@SequenceGenerator(name = "my_seq", sequenceName = "my_sequence")
private Long id;
GenerationType.TABLE (Table-based):
- Involves using a separate table to store and manage primary key values.Provides portability across different database systems.
@Id
@GeneratedValue(strategy = GenerationType.TABLE, generator = "my_table")
@TableGenerator(name = "my_table", table = "id_generator", pkColumnName = "id_key", pkColumnValue = "my_entity", valueColumnName = "id_value")
private Long id;
GenerationType.UUID (Universally Unique Identifier):
- Generates primary key values as UUIDs (Universally Unique Identifiers).Suitable for scenarios where globally unique identifiers are needed.
@Id
@GeneratedValue(generator = "uuid")
@GenericGenerator(name = "uuid", strategy = "org.hibernate.id.UUIDGenerator")
@Column(name = "id", unique = true, nullable = false, updatable = false)
private UUID id;
Assigned (Manual Assignment):
- Allows you to manually assign primary key values to entities.Useful when you want to control the primary key assignment, especially for legacy or externally managed keys.
@Id
@Column(name = "id")
private Long id;
// Manually assign the ID
public void setId(Long id) {
this.id = id;
}
The choice of primary key generation strategy depends on your specific application requirements and the database system you are using. Each strategy has its own advantages and limitations, so it’s important to select the one that best suits your use case.
What are different types of joins in Hibernate
Hibernate, as an object-relational mapping (ORM) framework, provides support for different types of joins to retrieve data from associated entities in a relational database. Here are the primary types of joins you can use in Hibernate, along with examples:
- Inner Join (JOIN or INNER JOIN):
- Retrieves rows that have matching values in both tables being joined.
- In Hibernate, you can use implicit inner joins when navigating associations in HQL or JPQL queries.
SELECT e FROM Employee e JOIN e.department d WHERE d.name = 'HR'
- Left Outer Join (LEFT JOIN):
- Retrieves all rows from the left table and the matched rows from the right table.
- In Hibernate, you can use left outer joins to load entities and their associated entities even if there’s no matching record in the associated table.
SELECT d FROM Department d LEFT JOIN FETCH d.employees
- Right Outer Join (RIGHT JOIN):
- Retrieves all rows from the right table and the matched rows from the left table.
- Right outer joins are less common in Hibernate because it primarily focuses on the left outer join.
SELECT e FROM Employee e RIGHT JOIN e.department d
- Full Outer Join (FULL JOIN):
- Retrieves all rows when there is a match in either the left or the right table.
- Full outer joins are less commonly supported in Hibernate, and you may need to use native SQL for this type of join.
SELECT * FROM Employee e FULL OUTER JOIN Department d ON e.department_id = d.id
- Cross Join (CROSS JOIN):
- Generates the Cartesian product of the two tables, resulting in all possible combinations of rows.
- Cross joins are rarely used and should be used with caution due to their potential to produce large result sets.
SELECT e, d FROM Employee e CROSS JOIN Department d
- Self-Join:
- A special type of join where a table is joined with itself, typically used for hierarchical or recursive structures.
- Self-joins are not limited to specific keywords; you can use standard join syntax to achieve them.
SELECT e1, e2 FROM Employee e1, Employee e2 WHERE e1.manager = e2.id
What is cascading in Hibernate and what are different types of cascading in Hibernate?
Cascading in Hibernate refers to the process of propagating state transitions (such as persisting, updating, or deleting) from an entity to its associated entities. When you perform an operation on an entity (e.g., saving an entity), cascading ensures that the same operation is applied to its related entities automatically. It simplifies the management of associated entities’ lifecycle.
Hibernate supports several types of cascading operations, which are specified using cascading options. These options can be set on associations (e.g., one-to-many or many-to-one relationships) to define how changes to one entity affect associated entities. Here are the different types of cascading operations in Hibernate:
CascadeType.PERSIST:
@OneToMany(mappedBy = "parent", cascade = CascadeType.PERSIST)
private List<Child> children;
CascadeType.MERGE:
@OneToOne(mappedBy = "employee", cascade = CascadeType.MERGE)
private Address address;
CascadeType.REMOVE:
@ManyToMany(mappedBy = "students", cascade = CascadeType.REMOVE)
private List<Course> courses;
CascadeType.REFRESH:
@ManyToOne(cascade = CascadeType.REFRESH)
private Parent parent;
CascadeType.DETACH:
@OneToMany(mappedBy = "order", cascade = CascadeType.DETACH)
private List<OrderItem> items;
CascadeType.ALL:
@OneToMany(mappedBy = "customer", cascade = CascadeType.ALL)
private List<Order> orders;
What are different Inheritance Mapping Strategies in Hibernate?
Hibernate provides several inheritance mapping strategies that allow you to map inheritance hierarchies to database tables. These strategies define how data from classes in an inheritance hierarchy is stored in the database. Here are the main inheritance mapping strategies in Hibernate, along with examples:
Single Table Inheritance (STI):
- All classes in the hierarchy are mapped to a single database table.A discriminator column is used to determine the actual subclass for each row.This strategy is efficient but can result in a wide table with many nullable columns.
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name = "employee_type", discriminatorType = DiscriminatorType.STRING)
public abstract class Employee {
@Id
@GeneratedValue
private Long id;
private String name;
// Common fields and methods
}
@Entity
@DiscriminatorValue("FULL_TIME")
public class FullTimeEmployee extends Employee {
private double salary;
// Additional fields and methods
}
@Entity
@DiscriminatorValue("PART_TIME")
public class PartTimeEmployee extends Employee {
private double hourlyRate;
// Additional fields and methods
}
Table Per Class Inheritance (TPC):
- Each class in the hierarchy is mapped to its own database table.No discriminator column is used, and each table contains all the fields for its class.Results in a normalized database schema but may require joins for querying.
@Entity
@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
public abstract class Employee {
@Id
@GeneratedValue
private Long id;
private String name;
// Common fields and methods
}
@Entity
public class FullTimeEmployee extends Employee {
private double salary;
// Additional fields and methods
}
@Entity
public class PartTimeEmployee extends Employee {
private double hourlyRate;
// Additional fields and methods
}
Joined Table Inheritance (JTI):
- Each class in the hierarchy is mapped to its own database table.Common fields are mapped to a shared table.Requires joins for querying but results in a more normalized database schema.
@Entity
@Inheritance(strategy = InheritanceType.JOINED)
public abstract class Employee {
@Id
@GeneratedValue
private Long id;
private String name;
// Common fields and methods
}
@Entity
public class FullTimeEmployee extends Employee {
private double salary;
// Additional fields and methods
}
@Entity
public class PartTimeEmployee extends Employee {
private double hourlyRate;
// Additional fields and methods
}
Mapped Superclass:
- Used when you don’t need to create instances of the superclass.Superclass is marked with
@MappedSuperclass
, and its fields are inherited by subclasses.No table is created for the superclass; only tables for subclasses.
@MappedSuperclass
public abstract class Employee {
@Id
@GeneratedValue
private Long id;
private String name;
// Common fields and methods
}
@Entity
public class FullTimeEmployee extends Employee {
private double salary;
// Additional fields and methods
}
@Entity
public class PartTimeEmployee extends Employee {
private double hourlyRate;
// Additional fields and methods
}
What is the use of Projection in Hibernate?
In Hibernate, a projection is a technique that allows you to query and retrieve specific fields or expressions from the database, rather than fetching entire entities. Projections are useful when you only need a subset of the data from one or more tables, and they can improve query performance by minimizing the amount of data transferred between the database and the application.
Hibernate provides several ways to perform projections, including the use of constructor expressions, result transformers, and criteria queries. Below, I’ll provide an example of using constructor expressions to perform a projection in Hibernate.
Let’s assume you have an entity class called Product
:
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Constructors, getters, setters, etc.
}
Now, suppose you want to retrieve only the name
and price
attributes of Product
entities. You can use a projection to achieve this using constructor expressions. Here’s an example using Hibernate’s HQL (Hibernate Query Language):
String hql = "SELECT NEW com.example.ProductProjection(p.name, p.price) FROM Product p";
Query<ProductProjection> query = session.createQuery(hql, ProductProjection.class);
List<ProductProjection> projections = query.getResultList();
In this example:
We define a class called ProductProjection
with a constructor that takes the name
and price
as parameters
public class ProductProjection {
private String name;
private double price;
public ProductProjection(String name, double price) {
this.name = name;
this.price = price;
}
// Getters and setters
}
We write an HQL query that selects these specific attributes using the NEW
keyword and the fully qualified name of the ProductProjection
class.
We create a query with the specified result class (ProductProjection.class
) and execute it to retrieve a list of ProductProjection
instances containing only the name
and price
attributes.
With this projection, you can avoid fetching unnecessary data from the database, which can be especially beneficial when dealing with large datasets or when you need to optimize query performance. Projections are a powerful feature in Hibernate that allows you to tailor your query results to your specific needs.
What are different Fetch strategies in Hibernate?
Hibernate provides different fetch strategies that allow you to control how data is loaded from the database when querying for entities and their associations. These fetch strategies determine when related data is loaded—either eagerly or lazily. Choosing the appropriate fetch strategy is essential for optimizing database access and application performance. Here are the primary fetch strategies in Hibernate, along with examples:
Eager Fetching (FetchType.EAGER):
- Eager fetching loads related data along with the main entity, typically using a SQL JOIN query.Use it when you usually need the associated data every time you fetch the entity.
@OneToMany(fetch = FetchType.EAGER)
@JoinColumn(name = "department_id")
private List<Employee> employees;
Lazy Fetching (FetchType.LAZY):
- Lazy fetching loads related data only when it’s accessed for the first time, reducing the initial query’s complexity.Use it when you want to avoid loading related data until needed, improving performance for read-heavy scenarios.
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "department_id")
private Department department;
Extra Lazy Fetching (Collection’s Extra Lazy):
- Extra lazy fetching is an extension of lazy loading, primarily used for collections (e.g., lists, sets, maps).It allows you to fetch individual elements of a collection lazily, rather than loading the entire collection eagerly.
@OneToMany(fetch = FetchType.LAZY)
@JoinColumn(name = "department_id")
@LazyCollection(LazyCollectionOption.EXTRA)
private List<Employee> employees;
Batch Fetching (Hibernate-specific):
- Batch fetching is a Hibernate-specific strategy that aims to minimize the number of SQL queries generated for lazy loading.It loads multiple lazy associations in a single query using an IN clause.
@Entity
public class Department {
// ...
@OneToMany(fetch = FetchType.LAZY)
@JoinColumn(name = "department_id")
@BatchSize(size = 10) // Batch fetch size
private List<Employee> employees;
}
Join Fetching (HQL/JPQL):
- Join fetching allows you to use an explicit JOIN FETCH clause in your HQL or JPQL queries to specify eager fetching.It fetches the associated data in the same query, reducing the number of database round trips.
String hql = "SELECT d FROM Department d JOIN FETCH d.employees WHERE d.name = 'HR'";
Query<Department> query = session.createQuery(hql, Department.class);
Department department = query.getSingleResult();
Subselect Fetching (HQL/JPQL):
- Subselect fetching is another option in HQL and JPQL that allows you to fetch associated collections using a subquery.It can be useful when you need to control how and when associated collections are loaded.
String hql = "SELECT d FROM Department d WHERE d.id = :deptId";
Query<Department> query = session.createQuery(hql, Department.class);
query.setParameter("deptId", 1L);
Department department = query.getSingleResult();
// Load associated employees using a subquery
department.getEmployees().size(); // Triggers the subquery
What are different Hibernate practices to avoid SQL Injection attacks?
SQL injection is a serious security vulnerability that can occur when user input is improperly handled in SQL queries, allowing malicious users to manipulate the database. To prevent SQL injection attacks in Hibernate, you should follow best practices for input validation and parameterization. Here are several Hibernate-specific practices to avoid SQL injection attacks:
- Use Parameterized Queries (Prepared Statements):
- Always use parameterized queries or prepared statements to build SQL queries with user input.
- Hibernate and JPA support parameterized queries, where you can use placeholders for parameters instead of embedding user input directly into the SQL query.
- Named Parameters:
- When using HQL or JPQL, prefer named parameters over positional parameters.
- Named parameters are more self-explanatory and easier to maintain.
- Criteria Queries with Restrictions:
- When using Hibernate’s Criteria API, utilize restrictions and criteria objects to build queries with parameters.
- Avoid constructing SQL fragments manually.
- Input Validation and Sanitization:
- Always validate and sanitize user input before using it in queries.
- Ensure that input data conforms to expected formats and constraints.
- Avoid Dynamic SQL Queries:
- Minimize the use of dynamic SQL queries constructed by concatenating user input.
- Prefer static queries or parameterized queries whenever possible.
- Use Native SQL Queries Safely:
- If you must use native SQL queries, make sure to use bind parameters (placeholders) and avoid concatenating user input directly into the query string.
String sql = "SELECT * FROM Employee e WHERE e.department_id = :deptId";
NativeQuery<Employee> query = session.createNativeQuery(sql, Employee.class);
query.setParameter("deptId", departmentId);
What are Hibernate’s Callback Interfaces?
Hibernate provides callback interfaces that allow you to hook into various points in the object lifecycle of persistent entities. These callback interfaces enable you to execute custom code before or after certain database operations. The main callback interfaces in Hibernate are:
Entity Lifecycle Callbacks:
@PrePersist
: Executed before the entity is saved (persisted) to the database.@PostPersist
: Executed after the entity has been saved (persisted) to the database.@PreUpdate
: Executed before the entity is updated in the database.@PostUpdate
: Executed after the entity has been updated in the database.@PreRemove
: Executed before the entity is removed (deleted) from the database.@PostRemove
: Executed after the entity has been removed (deleted) from the database.Example:
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@PrePersist
public void onPrePersist() {
// Custom logic before persisting
}
@PostPersist
public void onPostPersist() {
// Custom logic after persisting
}
// Other callback methods for update and remove
}
Event Listeners:
- Hibernate provides event listeners that allow you to define custom logic for various events such as entity loading, saving, updating, and deleting.You can implement event listener interfaces and register them with Hibernate’s
SessionFactory
.
public class CustomSaveOrUpdateEventListener implements SaveOrUpdateEventListener {
@Override
public void onSaveOrUpdate(SaveOrUpdateEvent event) throws HibernateException {
// Custom logic before save or update
}
}
// Register the event listener with the SessionFactory
sessionFactory.registerListener(EventType.SAVE_UPDATE, new CustomSaveOrUpdateEventListener());
Interceptors:
- Hibernate allows you to define custom interceptors that can be used to customize or modify the behavior of Hibernate’s session and entity operations.You can create a custom interceptor class that implements the
Interceptor
interface.
public class CustomInterceptor extends EmptyInterceptor {
@Override
public boolean onSave(Object entity, Serializable id, Object[] state, String[] propertyNames, Type[] types) {
// Custom logic before entity save
return super.onSave(entity, id, state, propertyNames, types);
}
}
// Configure the interceptor in Hibernate configuration or SessionFactory
Configuration configuration = new Configuration();
configuration.setInterceptor(new CustomInterceptor());
EntityListeners (JPA):
- JPA (Java Persistence API) provides the
@EntityListeners
annotation to specify entity listeners at the class level.Entity listeners are classes that contain callback methods annotated with@PrePersist
,@PostPersist
,@PreUpdate
, etc.
@Entity
@EntityListeners(EmployeeListener.class)
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
}
public class EmployeeListener {
@PrePersist
public void onPrePersist(Employee employee) {
// Custom logic before persisting
}
@PostPersist
public void onPostPersist(Employee employee) {
// Custom logic after persisting
}
// Other callback methods for update and remove
}
These callback interfaces and mechanisms provided by Hibernate and JPA give you flexibility to integrate custom logic at various stages of the object lifecycle and database interactions, allowing you to implement behaviors like auditing, validation, and complex data manipulation.
What is Hibernate Proxy?
In Hibernate, a proxy is a dynamically generated subclass of an entity class. Hibernate creates these proxy classes to enable lazy loading of associations and optimize database access.
The main purpose of Hibernate proxies is to avoid loading related entities until they are actually needed. When you retrieve an entity from the database, Hibernate might not fetch its associated entities immediately. Instead, it creates a proxy for each associated entity. These proxies are lightweight placeholders that stand in for the actual associated entities.
The key features of Hibernate proxies are:
- Lazy Loading: Hibernate proxies allow for lazy loading of associations. Lazy loading means that associated entities are loaded from the database only when they are accessed for the first time, rather than being fetched eagerly along with the main entity. This can significantly improve performance by reducing the number of database queries.
- Transparent: From the perspective of your application code, working with a Hibernate proxy is no different from working with a regular entity object. You can call methods on the proxy and access its properties just like you would with a real entity.
- Initialization: When you access a property or method of a Hibernate proxy that requires loading the associated entity from the database, Hibernate automatically initializes the proxy by fetching the real entity’s data. This process is often referred to as “proxy initialization.”
- Same Interface: Proxies implement the same interface or extend the same class as the actual entity they represent. This means that your application code doesn’t need to be aware of whether it’s working with a proxy or a fully initialized entity.
What is the role of JMX in Hibernate?
Java Management Extensions (JMX) is a Java technology that provides a standard way to manage and monitor Java applications, including Hibernate-based applications. In the context of Hibernate, JMX can be used to monitor and manage various aspects of Hibernate’s behavior and performance. Here are some key roles of JMX in Hibernate:
- Monitoring Hibernate Statistics:
- JMX can be used to access and monitor Hibernate statistics, which provide valuable insights into how Hibernate is interacting with the database.
- You can gather statistics on query execution times, cache usage, and various other performance-related metrics.
- Monitoring these statistics can help identify performance bottlenecks and optimize database interactions.
- Configuration Tuning:
- JMX can be used to dynamically adjust Hibernate configuration settings at runtime.
- This allows you to fine-tune Hibernate’s behavior without the need to restart your application.
- For example, you can adjust the logging level or cache settings on the fly.
- Query Analysis:
- JMX can provide a means to analyze and profile SQL queries generated by Hibernate.
- You can monitor the SQL statements being executed, their execution plans, and query parameters.
- This helps in identifying poorly performing queries or potential SQL injection vulnerabilities.
- Cache Management:
- JMX can be used to manage Hibernate’s second-level cache.
- You can clear cache regions, monitor cache statistics, and adjust cache settings as needed.
- Fine-tuning the cache can have a significant impact on application performance.
- Session and Connection Pool Management:
- JMX can provide insights into Hibernate’s session management and connection pooling.
- You can monitor the number of active sessions, track connection pool statistics, and identify resource leaks.
- This helps in managing database connections efficiently.
- Runtime Monitoring and Troubleshooting:
- JMX can be a valuable tool for monitoring the health of your Hibernate-based application in production.
- You can use JMX consoles or tools to check the status of various Hibernate components and troubleshoot issues.
- Custom Monitoring and Management Beans (MBeans):
- You can create custom MBeans to expose specific Hibernate-related functionalities or metrics that are relevant to your application.
- This allows you to tailor JMX management to your application’s specific needs.
How do we get Hibernate Statistics?
To obtain Hibernate statistics, you can use Hibernate’s built-in statistics collection feature. Hibernate provides a Statistics
interface and a set of classes for collecting and exposing statistics about Hibernate’s behavior, including query execution times, cache usage, and more. Here’s how you can enable and retrieve Hibernate statistics:
Enable Statistics: To enable Hibernate statistics, you need to configure it in your Hibernate configuration or SessionFactory
creation.
In your Hibernate configuration file (hibernate.cfg.xml
) or Java configuration code, add the following property:
<!-- Enable Hibernate statistics -->
<property name="hibernate.generate_statistics">true</property>
If you’re using Java configuration, you can enable statistics programmatically:
Configuration configuration = new Configuration();
configuration.setInterceptor(new CustomInterceptor()); // Your custom interceptor, if needed
configuration.setProperty("hibernate.generate_statistics", "true");
SessionFactory sessionFactory = configuration.buildSessionFactory();
Accessing Statistics: Once you’ve enabled Hibernate statistics, you can access the statistics via the SessionFactory
or Session
object. Here are some common statistics you can retrieve:
SessionFactory Statistics: To access statistics at the SessionFactory
level (application-wide statistics), you can use the following code:
Statistics statistics = sessionFactory.getStatistics();
// Print various statistics
System.out.println("Entity Insert Count: " + statistics.getEntityInsertCount());
System.out.println("Entity Update Count: " + statistics.getEntityUpdateCount());
System.out.println("Query Execution Count: " + statistics.getQueryExecutionCount());
// ... and more
Session Statistics: To access statistics at the Session
level (specific to a particular session), you can use the following code:
Session session = sessionFactory.openSession();
Statistics sessionStatistics = session.getStatistics();
// Print session-specific statistics
System.out.println("Session Entity Load Count: " + sessionStatistics.getEntityLoadCount());
System.out.println("Session Entity Fetch Count: " + sessionStatistics.getEntityFetchCount());
// ... and more
session.close(); // Close the session when done
Available Statistics: Hibernate provides a wide range of statistics that you can access via the Statistics
interface. Some of the commonly used statistics include:
getEntityLoadCount()
: Number of entity loads.getEntityFetchCount()
: Number of entity fetches.getQueryExecutionCount()
: Number of query executions.getSecondLevelCacheHitCount()
: Number of second-level cache hits.getSecondLevelCacheMissCount()
: Number of second-level cache misses.getSecondLevelCachePutCount()
: Number of second-level cache puts.getCollectionLoadCount()
: Number of collection loads.getCollectionFetchCount()
: Number of collection fetches.getCollectionUpdateCount()
: Number of collection updates.
How to use Versioning in Hibernate?
Versioning in Hibernate is a technique used to handle concurrent access and updates to database records. It helps prevent conflicts when multiple users or processes attempt to modify the same data simultaneously. Hibernate implements versioning using a special column in the database table to keep track of the record’s version. Here’s how to use versioning in Hibernate:
Add a Version Column:
- To enable versioning in Hibernate, add a version column to your entity’s corresponding database table.This column typically holds a numeric value (e.g., an integer or a timestamp) representing the version of the record.You can use the
@Version
annotation to specify which property in your entity should be mapped to the version column.
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
@Version
private int version; // Version column
// Getters and setters
}
Optimistic Locking:
Versioning in Hibernate is typically used with optimistic locking, which means that you assume conflicts are rare, and you allow multiple users to read and modify data concurrently.
When an entity is retrieved, Hibernate records the version number.
When the entity is updated and persisted, Hibernate checks if the version number in the database matches the version number in the entity.
If they match, the update proceeds; if not, an exception (e.g., OptimisticLockException
) is thrown.
Performing Updates:
- When you want to update an entity, retrieve it, make changes, and then persist it using Hibernate’s
Session
orEntityManager
.Hibernate will automatically increment the version number in the database during the update.
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Product product = session.get(Product.class, productId); // Retrieve the entity
product.setName("New Name"); // Modify the entity
session.update(product); // Persist the updated entity
transaction.commit();
session.close();
Handling Version Conflicts:
- If a version conflict occurs (i.e., the version in the database doesn’t match the version in the entity being updated), Hibernate will throw an exception.To handle version conflicts gracefully, catch the exception and implement conflict resolution logic, such as merging changes, displaying a conflict resolution UI, or notifying the user.
try {
// Attempt to update the entity
session.update(product);
transaction.commit();
} catch (OptimisticLockException ex) {
// Handle version conflict
// Display a message to the user or implement conflict resolution logic
transaction.rollback();
}
Using versioning with optimistic locking in Hibernate helps ensure data integrity and consistency when multiple users or processes are modifying the same records concurrently. It’s a valuable technique for managing concurrent access in database applications.
How to perform Concurrency Control in Hibernate?
Concurrency control in Hibernate is essential to manage concurrent access and updates to database records to ensure data integrity and consistency. Below are the approaches to perform concurrency control in Hibernate:
1. Optimistic Locking with Versioning:
- This is the most commonly used approach for concurrency control in Hibernate.
- It involves adding a version column to your entity, as described earlier.
- When multiple users or processes attempt to update the same record simultaneously, Hibernate compares the version number in the database with the version number in the entity.
- If the versions match, the update proceeds; otherwise, a concurrency exception is thrown.
- Developers need to handle concurrency exceptions gracefully by merging changes, displaying conflict resolution UI, or notifying users.
Example:
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Product product = session.get(Product.class, productId); // Retrieve the entity
product.setName("New Name"); // Modify the entity
session.update(product); // Persist the updated entity
transaction.commit();
session.close();
2. Pessimistic Locking:
- Pessimistic locking is an alternative approach where a record is locked for exclusive access by one user or process.
- Hibernate provides different types of pessimistic locks, including
LockMode.UPGRADE
,LockMode.UPGRADE_NOWAIT
, andLockMode.WRITE
. - You can apply a pessimistic lock to an entity by specifying the lock mode when retrieving the entity.
- Pessimistic locks block other transactions from accessing the locked record until the lock is released, which can lead to contention and reduced concurrency.
Example:
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Product product = session.get(Product.class, productId, LockMode.UPGRADE); // Apply a pessimistic lock
// Perform updates
transaction.commit(); // Lock is released when the transaction is committed
session.close();
3. Custom Locking Mechanisms:
- In some cases, you may need to implement custom locking mechanisms beyond what Hibernate provides.
- You can use native SQL queries or stored procedures to implement custom locking strategies specific to your application’s requirements.
Example using native SQL:
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Query query = session.createSQLQuery("SELECT * FROM products WHERE id = :productId FOR UPDATE");
query.setParameter("productId", productId);
// Execute the native SQL query with locking
transaction.commit(); // Lock is released when the transaction is committed
session.close();
4. Versionless Locking:
- In certain scenarios, you may not need versioning or locking at all, especially if your application relies on non-relational databases or has specific use cases where concurrent updates are acceptable.
- Be cautious when opting for versionless locking, as it may lead to data inconsistencies in a multi-user environment.
What is the Hibernate Connection Pool?
A Hibernate Connection Pool is a crucial component in Hibernate-based applications that manages and maintains a pool of database connections. This pool helps improve application performance by reusing database connections instead of creating a new connection for each database operation, which can be resource-intensive and slow.
Commonly used connection pool options in Hibernate include:
- C3P0: C3P0 is a popular connection pool library that provides robust connection pooling and configuration options.
- HikariCP: HikariCP is a high-performance, lightweight connection pool library that is known for its speed and efficiency.
- Apache DBCP: Apache DBCP (Database Connection Pooling) is another widely used connection pool library that is part of the Apache Commons project.
Here’s a simple example of configuring Hibernate with HikariCP as the connection pool:
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.service.ServiceRegistry;
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class HibernateUtil {
private static final SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory() {
try {
// Create a HikariCP configuration
HikariConfig hikariConfig = new HikariConfig();
hikariConfig.setJdbcUrl("jdbc:mysql://localhost:3306/your_database");
hikariConfig.setUsername("your_username");
hikariConfig.setPassword("your_password");
hikariConfig.setDriverClassName("com.mysql.cj.jdbc.Driver");
// Create a HikariCP data source
HikariDataSource dataSource = new HikariDataSource(hikariConfig);
// Create a Hibernate configuration
Configuration configuration = new Configuration();
configuration.configure("hibernate.cfg.xml"); // You can also configure Hibernate programmatically
// Set the data source for Hibernate
configuration.setProperty("hibernate.connection.datasource", dataSource.getDataSourceName());
// Register the Hibernate service registry
ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder()
.applySettings(configuration.getProperties())
.build();
// Build the SessionFactory
return configuration.buildSessionFactory(serviceRegistry);
} catch (Exception ex) {
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
In this example, we use HikariCP as the connection pool library. You need to replace "jdbc:mysql://localhost:3306/your_database"
, "your_username"
, and "your_password"
with your actual database connection details. Also, make sure to configure Hibernate according to your application’s requirements.
With this setup, you can obtain a Hibernate Session
from the SessionFactory
and use it to perform database operations efficiently, as the connection pooling is handled by HikariCP.
How do you perform Hibernate Tuning?
Hibernate tuning is an essential aspect of optimizing the performance and efficiency of Hibernate-based applications. Proper tuning ensures that your application works efficiently and scales well. Here are some key steps and strategies for Hibernate tuning:
- Database Schema Design:
- Ensure that your database schema is well-designed with proper indexing and normalization.
- Lazy Loading and Eager Loading:
- Use lazy loading (the default in Hibernate) for associations by default, but consider eager loading for cases where it’s more efficient to fetch related data upfront.
- Batch Fetching:
- Configure batch fetching to reduce the number of queries executed. Hibernate allows you to fetch multiple related entities in a single query.
- Caching:
- Hibernate provides both first-level (session-level) and second-level (application-level) caching. Configure these appropriately to reduce database hits and improve response times. Popular caching providers include Ehcache, Infinispan, and Hazelcast.
- Query Optimization:
- Use the Hibernate Query Language (HQL) or Criteria API efficiently. Avoid using complex queries that can lead to performance bottlenecks. Use database-specific query optimization techniques like indexing.
- Connection Pooling:
- Choose an appropriate connection pool (e.g., HikariCP, C3P0) and configure it with optimal settings. Monitor and adjust pool size as needed to handle varying loads.
- Transaction Management:
- Use appropriate transaction isolation levels to balance concurrency and data consistency. Consider using read-only transactions for read-heavy operations.
- Logging and Monitoring:
- Enable Hibernate logging and monitoring tools to identify and analyze performance bottlenecks. Tools like Hibernate Profiler and database-specific monitoring tools can be helpful.
- Fetch Plans and Fetch Profiles:
- Use fetch plans and fetch profiles to customize how Hibernate fetches data for specific use cases. This can help reduce over-fetching or under-fetching of data.
- Optimistic Locking:
- Implement optimistic locking to handle concurrent updates efficiently. This prevents lost updates and reduces contention.
- Hibernate Versioning:
- Implement versioning for entities to track changes and enable efficient conflict resolution in concurrent scenarios.
What are the different ORM levels in Hibernate?
1. Pure Relational ORM:
- Developers have full control over SQL queries, making it possible to fine-tune queries for optimal performance.
- SQL-based ORM is suitable for legacy systems where the database schema is already established and complex.
- It may require more effort to maintain and update SQL queries as the application evolves.
// SQL query to retrieve employee by ID
String sql = "SELECT * FROM employees WHERE employee_id = :employeeId";
Map<String, Object> parameters = new HashMap<>();
parameters.put("employeeId", 123);
// Execute the SQL query and process the result
ResultSet resultSet = executeSQLQuery(sql, parameters);
Employee employee = mapResultSetToEmployee(resultSet);
2. Light Object Mapping:
- Offers a simple and straightforward mapping of entities to tables, reducing the complexity of the data access layer.
- Well-suited for applications with a small number of entities and straightforward data access requirements.
- May not provide as much flexibility or automated support for complex queries and relationships compared to higher ORM levels.
// Entity class for Employee manually mapped to the 'employees' table
public class Employee {
private Long id;
private String firstName;
private String lastName;
// Getters and setters
}
// Data access code to retrieve an Employee
public Employee getEmployeeById(Long employeeId) {
// Execute SQL query internally, map result to Employee, and return
}
3. Medium Object Mapping:
- Strikes a balance between object-oriented and relational paradigms, making it suitable for a wide range of applications.
- Reduces the need for manual SQL query writing for basic CRUD operations.
- Supports basic associations between entities, simplifying data retrieval and navigation.
// Entity class for Employee using Hibernate annotations
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
// Getters and setters
}
// Using Hibernate's session to retrieve an Employee
Session session = sessionFactory.openSession();
Employee employee = session.get(Employee.class, 123L); // Hibernate generates SQL
session.close();
4. Full Object Mapping:
- Offers advanced ORM features, including support for inheritance hierarchies, polymorphism, and composite objects.
- Provides transparent fetching strategies to optimize data retrieval, reducing the risk of N+1 query problems.
- Requires less manual SQL and provides a high level of abstraction, promoting cleaner and more maintainable code.
- Enables efficient caching mechanisms to improve application performance.
// Entity class for Employee using Hibernate annotations
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
// Getters and setters
}
// Fetching an Employee with associated Department using Hibernate
Session session = sessionFactory.openSession();
Employee employee = session.get(Employee.class, 123L); // Hibernate handles joins and relationships
session.close();
Which Design Patterns are used in the Hibernate Framework?
Hibernate, as an ORM (Object-Relational Mapping) framework, incorporates several design patterns to address various architectural and structural concerns. Some of the notable design patterns used in Hibernate are:
Factory Method Pattern:
- The Factory Method Pattern is used in Hibernate for creating instances of the
SessionFactory
.TheSessionFactory
is typically created using theSessionFactoryBuilder
, which follows the Factory Method Pattern.
Configuration configuration = new Configuration();
configuration.configure("hibernate.cfg.xml"); // Load configuration
ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder()
.applySettings(configuration.getProperties())
.build();
SessionFactory sessionFactory = configuration.buildSessionFactory(serviceRegistry);
Singleton Pattern:
- The Singleton Pattern ensures that there is only one instance of the
SessionFactory
in a Hibernate application.TheSessionFactory
is a heavyweight object, and having a single instance throughout the application is a common optimization strategy.
public class HibernateUtil {
private static final SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory() {
// ... Factory creation code ...
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
DAO (Data Access Object) Pattern:
- Hibernate encourages the use of the DAO pattern for separating the data access logic from the business logic.Developers create DAO classes to encapsulate CRUD operations and queries for specific entities.
public class EmployeeDAO {
public void save(Employee employee) {
// Hibernate session and transaction management for saving an employee
}
public Employee getById(Long id) {
// Hibernate session and query to retrieve an employee by ID
}
// Other data access methods...
}
Proxy Pattern:
- Hibernate uses proxy objects for achieving lazy loading of associations and optimizing database access.When you fetch an entity, Hibernate may return a proxy object that loads the actual data from the database when accessed.This enhances performance by avoiding unnecessary database queries.
Employee employee = session.load(Employee.class, 1L);// Returns a proxy, not a fully initialized object
// When accessing employee properties or associations, the proxy fetches data from the database
Observer Pattern:
- Hibernate employs the Observer Pattern to notify entities and applications about changes in the state of managed entities.When entities are modified and changes are flushed to the database, Hibernate triggers events that can be observed by registered listeners.These listeners can then react to changes in the entity state.
@EntityListeners(EmployeeListener.class)
public class Employee {
// Entity mapping and attributes...
}
public class EmployeeListener {
@PreUpdate
public void beforeUpdate(Employee employee) {
// Perform actions before an employee entity is updated
}
}
What are different ways to integrate Hibernate with Spring ?
ntegrating Hibernate with Spring is a common practice in Java enterprise applications. Spring provides various approaches for integrating Hibernate seamlessly. Here are different ways to integrate Hibernate with Spring:
Spring ORM (Object-Relational Mapping) Integration:
- Spring’s ORM module provides comprehensive support for Hibernate integration.
- It offers declarative transaction management, exception translation, and easy configuration.
Spring Data JPA with Hibernate:
- Spring Data JPA is a higher-level abstraction built on top of JPA (Java Persistence API) that works well with Hibernate as the JPA provider.
- Spring Data JPA simplifies data access by providing repository interfaces and automatic query generation.
Using HibernateTemplate:
HibernateTemplate
is a Spring-provided utility class designed to simplify and streamline Hibernate data access code within a Spring application.
Note : Starting from Spring 3.1, the use of HibernateTemplate
has been discouraged, and it is considered obsolete.
How to solve the N+1 SELECT problem in Hibernate?
The N+1 SELECT problem is a common performance issue that occurs in Hibernate and other ORMs (Object-Relational Mapping) when retrieving data from a relational database. It happens when, for a given entity, Hibernate issues one query to fetch the main data and then N additional queries to fetch the associated collections or entities, resulting in N+1 queries. This can lead to a significant performance overhead, especially when dealing with large datasets.
Here’s a brief explanation of the problem and ways to solve it:
Problem Explanation: Suppose you have a Department
entity with a one-to-many relationship to Employee
. When you retrieve a list of departments, Hibernate first fetches the department data with one query. However, when you access the list of employees for each department, Hibernate issues a separate query for each department to load its associated employees. This leads to N+1 queries, where N is the number of departments.
Solutions:
- Eager Fetching:
- Use eager fetching to load the associated entities or collections along with the main entity in a single query. This can be done using Hibernate annotations like
@ManyToOne(fetch = FetchType.EAGER)
or HQL queries with theJOIN FETCH
clause. - Eager fetching should be used with caution, as it may lead to loading more data than necessary and can affect performance.
- Use eager fetching to load the associated entities or collections along with the main entity in a single query. This can be done using Hibernate annotations like
- Lazy Loading:
- By default, Hibernate uses lazy loading for associations, which means the associated data is loaded on-demand when accessed.
- You can keep lazy loading for associations but use batch fetching to reduce the number of queries. Batch fetching allows you to load multiple associations in a single query, reducing the N+1 problem.
- HQL or Criteria Fetching:
- Use HQL (Hibernate Query Language) or the Criteria API to fetch data selectively and avoid the N+1 problem.
- You can use
JOIN FETCH
in HQL or create more specific queries that retrieve only the necessary data.
- Fetch Profiles:
- Hibernate allows you to define fetch profiles to specify how associations should be loaded for different use cases.
- By configuring fetch profiles, you can control whether associations are eagerly or lazily loaded based on the context.
- Second-Level Cache:
- Implement a second-level cache (e.g., Ehcache, Hazelcast) to cache frequently accessed data, reducing the need for repeated database queries.
- Caching can be particularly effective for read-heavy applications.
- DTO (Data Transfer Object) Projection:
- Instead of fetching full entities, create DTOs that project only the required data.
- This reduces the amount of data fetched from the database and can help mitigate the N+1 problem.
- Pagination and Batching:
- When dealing with large result sets, use pagination and batching to limit the number of entities fetched in a single query.
- Fetch only a subset of the data at a time to avoid loading unnecessary records.
What are Fetch Profiles in Hibernate?
Fetch Profiles in Hibernate are a mechanism that allows you to specify how associations should be loaded based on different use cases or scenarios. They provide a way to customize the fetching strategy for associations on a per-query basis. This can help optimize database queries and reduce the risk of the N+1 SELECT problem.
Here’s a brief explanation and example of Fetch Profiles in Hibernate:
Explanation:
- Fetch Profiles allow you to define named profiles with specific fetching strategies for associations.
- These profiles can be activated when executing queries to load data based on the defined fetching strategies.
Example: Suppose you have an entity Department
with a one-to-many relationship to Employee
, and you want to control how the employees
collection is loaded. You can define a Fetch Profile for this purpose.
@Entity
@Table(name = "departments")
@FetchProfile(name = "department-employees", fetchOverrides = {
@FetchProfile.FetchOverride(entity = Department.class, association = "employees", mode = FetchMode.JOIN)
})
public class Department {
// Entity mapping and attributes...
@OneToMany(mappedBy = "department")
private Set<Employee> employees = new HashSet<>();
// Getters and setters...
}
In this example:
- We’ve defined a Fetch Profile named
"department-employees"
. - We specify that when this profile is activated, the
employees
collection of theDepartment
entity should be fetched using aJOIN
strategy.
To activate the Fetch Profile when executing a query, you can use the setFetchProfile
method provided by the Hibernate Session
:
session.enableFetchProfile("department-employees");
Query query = session.createQuery("FROM Department WHERE id = :departmentId");
query.setParameter("departmentId", 1L);
Department department = (Department) query.uniqueResult();
// The 'employees' collection will be loaded according to the fetch profile
By enabling the Fetch Profile "department-employees"
before executing the query, you ensure that the specified fetching strategy is applied for the employees
collection for this specific query. Fetch Profiles provide a way to fine-tune how associations are loaded in Hibernate queries, improving performance and reducing unnecessary database round-trips.
How to do Batch Processing in Hibernate?
Batch processing in Hibernate involves processing a large number of database records efficiently in smaller batches to reduce memory usage and improve performance. Hibernate provides a feature called “batch processing” that allows you to work with large datasets more effectively.
To enable batch processing in Hibernate and set the hibernate.jdbc.batch_size
property.
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Other Hibernate properties and configurations -->
<!-- Set the batch size for JDBC batching -->
<property name="hibernate.jdbc.batch_size">50</property>
<!-- More Hibernate properties and configurations -->
</session-factory>
</hibernate-configuration>
In Java code, you can use the Hibernate Session
and transactions to perform batch processing.
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
List<Employee> employeesToUpdate = ... // List of Employee objects to update
int batchSize = 50; // Set the batch size
for (int i = 0; i < employeesToUpdate.size(); i++) {
Employee employee = employeesToUpdate.get(i);
session.update(employee);
if (i % batchSize == 0) {
// Flush and clear the session to process the batch
session.flush();
session.clear();
}
}
tx.commit();
session.close();
What is the purpose of Hibernate’s @NaturalId annotation?
The @NaturalId
annotation in Hibernate is used to mark a property of an entity as a natural identifier. A natural identifier is a business key or a unique identifier that is not generated by the database but is meaningful in the context of the application. The purpose of @NaturalId
is to provide a more efficient way to look up entities by their natural identifiers.
Example: Suppose you have an entity called Product
with a unique SKU (Stock Keeping Unit) that serves as a natural identifier. You can use the @NaturalId
annotation to mark the SKU property:
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@NaturalId
@Column(unique = true)
private String sku;
// Other entity properties and methods...
}
In this example, the sku
property is marked as a natural identifier using @NaturalId
. This indicates that Hibernate should optimize queries and caching for lookups based on the SKU, improving efficiency when retrieving products by their SKU values.
Now, to fetch a Product
entity by its sku
natural identifier, you can use the byNaturalId
method:
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
String skuToLookup = "ABC123"; // The SKU you want to look up
Product product = session.byNaturalId(Product.class)
.using("sku", skuToLookup)
.load();
tx.commit();
session.close();
if (product != null) {
// Product found by SKU
System.out.println("Product Name: " + product.getName());
} else {
// Product not found
System.out.println("Product with SKU " + skuToLookup + " not found.");
}
What are Hibernate’s @Type and @TypeDef annotations used for ?
Hibernate’s @Type
and @TypeDef
annotations are used to work with custom user-defined types (UDTs) in your Hibernate entity mappings. These annotations allow you to map database columns to Java objects of your custom types, providing flexibility when dealing with non-standard data types in your domain model. Here’s an explanation of each annotation:
@Type:
The @Type
annotation is used at the field or property level within an entity class to specify the Hibernate type associated with a specific property. It allows you to map a property to a custom user-defined type.
Example:
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "employee_name")
private String name;
@Type(type = "com.example.CustomDateType")
@Column(name = "join_date")
private CustomDate hireDate;
// Other entity properties, getters, setters, etc.
}
In this example, we use @Type
to map the hireDate
property to a custom user-defined type called CustomDateType
.
@TypeDef:
- The
@TypeDef
annotation is used at the class level of an entity to define a custom user-defined type and associate it with a name that can be referenced using the@Type
annotation within entity properties.
Example:
@Entity
@TypeDef(name = "customDateType", typeClass = com.example.CustomDateType.class)
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "employee_name")
private String name;
@Type(type = "customDateType")
@Column(name = "join_date")
private CustomDate hireDate;
// Other entity properties, getters, setters, etc.
}
In this example, we use @TypeDef
to define the custom type customDateType
and associate it with the Java class com.example.CustomDateType
. Then, we reference this type using @Type
on the hireDate
property.