The switch case in Java is a multi-way branch statement. It provides an easy way to dispatch execution to different parts of code based on the value of the expression. The expression can be byte, short, char, int primitive data types, Enums, the String and Wrapper classes.
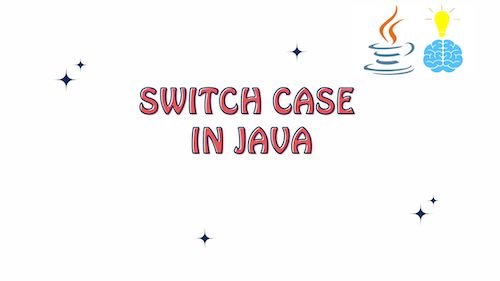
Rules of Switch Case in Java
Some Important rules for switch statements :
- Duplicate case values are not allowed.
- The value for a case must be of the same data type as the variable in the switch.
- The value for a case must be a constant or a literal. Variables are not allowed.
- The break statement is used inside the switch to terminate a statement sequence.
- The break statement is optional. If omitted, execution will continue on into the next case.
- The default statement is optional and can appear anywhere inside the switch block. In case, if it is not at the end, then a break statement must be kept after the default statement to omit the execution of the next case statement.
Code Example : Switch Case in Java
package com.java.core;
import java.util.stream.IntStream;
public class SwitchExample {
public void printWeekDay(int dayNumber) {
switch (dayNumber) {
case 1:
System.out.println("Monday!");
break;
case 2:
System.out.println("Tuesday!");
break;
case 3:
System.out.println("Wednesday!");
break;
case 4:
System.out.println("Thursday!");
break;
case 5:
System.out.println("Friday!");
break;
case 6:
System.out.println("Saturday!");
break;
case 7:
System.out.println("Sunday!");
break;
default:
System.out.println("Invalid day Number");
}
}
public static void main(String args[]) {
SwitchExample switchExample = new SwitchExample();
IntStream stream = IntStream.range(1, 7);
stream.forEach(i - > switchExample.printWeekDay(i));
switchExample.printWeekDay(10);
}
}
Monday!
Tuesday!
Wednesday!
Thursday!
Friday!
Saturday!
Invalid day Number
Limitations of Switch Case in Java
The switch-case statement in Java is a powerful construct for handling multiple cases efficiently, but it has some limitations and constraints that developers need to be aware of:
- Expression Constraints:
- The expression used in the switch statement must evaluate to a constant expression.
- Only certain data types are supported as switch expressions, including
int
,byte
,short
,char
,String
(since Java 7), and certain enumerated types.
- No Ranges or Complex Conditions:
- Switch-case doesn’t support ranges or complex conditions within cases. Each case must represent a specific value.
- No Direct Boolean Expressions:
- Boolean expressions (e.g.,
true
,false
) cannot be used directly as case values.
- Boolean expressions (e.g.,
- No Floating-Point Numbers:
- Floating-point numbers (e.g.,
float
,double
) cannot be used as case values due to potential precision issues.
- Floating-point numbers (e.g.,
- No Dynamic Values:
- Case values must be constants known at compile time. They cannot be computed or changed at runtime.
- No Complex Expressions:
- Complex expressions within cases (e.g., method calls, arithmetic operations) are discouraged, as they can make the code less readable and harder to debug.
Best Practices for Using Switch Case in Java
Using the switch case in Java effectively involves following certain best practices to ensure clean, maintainable, and bug-free code. Here are some best practices to consider:
- Use Enumerations and Constants:
- Prefer using enums and constants as case values. This enhances code readability and reduces the likelihood of errors due to typo-related issues.
- Use Default Case:
- Always include a
default
case to handle unexpected or unhandled values. This prevents unintended behavior if the switch expression doesn’t match any cases.
- Always include a
- Keep it Simple:
- Keep the code inside each case block concise and focused. Avoid complex expressions, method calls, or heavy logic within the case blocks.
- Group Related Cases:
- Group related cases together to improve code readability. This helps developers quickly understand the logic behind each group.
- Prefer switch Over if-else for Multiple Cases:
- Use switch-case over multiple if-else statements when dealing with multiple cases based on a single value. This improves code readability and performance.
- Avoid Complex Logic:
- Avoid complex logic or calculations within cases. If necessary, refactor the logic into separate methods or external functions for clarity.
- Use Break Carefully:
- Use the
break
statement judiciously to prevent unintended execution of subsequent cases.
- Use the
Conclusion
In this article, we explored Switch case in Java in great details. We looked into various rules of Switch case and illustrated the usage using a Sample Java Code. Then we discussed various limitations and best practices of Swift case in Java.