In this article, we will explore Anonymous Class in Java in great details. So, let’s get started.
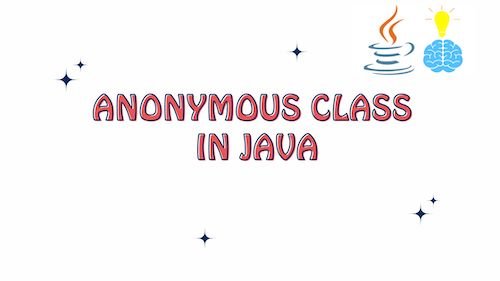
What is Anonymous Class in Java ?
In very simple words, an anonymous class is a nested class that’s defined on the fly without a name.
How to Create Anonymous Class in Java ?
We can instantiate an anonymous class from
- A Class (may be abstract or concrete).
- An Interface
Example : Create Anonymous Class in Java
In the below example we use instantiate anonymous classes from a concrete class, an abstract class and an interface.
An anonymous class declaration is an expression, hence it must be a part of a statement. We have put a semicolon at the end of the statement.
package com.java.core;
class TestClass {
void testmethod() {}
}
abstract class TestAbstract {
abstract void abstractMethod();
}
interface TestInterface {
void interfaceMethod();
}
public class AnonymousExample {
public static void main(String[] args) {
TestClass testClass = new TestClass() {
@Override
public void testmethod() {
System.out.println("Inside Test Method");
}
};
testClass.testmethod();
TestAbstract testAbstract = new TestAbstract() {
public void abstractMethod() {
System.out.println("Inside abstract Method");
}
};
testAbstract.abstractMethod();
TestInterface testInterface = new TestInterface() {
public void interfaceMethod() {
System.out.println("Inside interface Method");
}
};
testInterface.interfaceMethod();
}
}
Inside Test Method
Inside abstract Method
Inside interface Method
Differences between Normal and Anonymous Class in Java
Metric | Normal Class | Anonymous Class |
---|---|---|
Name | Has a distinct name | No explicit name |
Usage | Can be reused | Typically used for one-time use |
Inheritance | Can extend a single class | Can extend a single class or implement an interface |
Constructors | Can have multiple constructors | No constructors |
Accessibility | Can have public, protected, or package-private access | Cannot have access modifiers other than final |
Instantiation | Requires separate instantiation with new keyword | Instantiated during object creation |
Code Locality | Defined in separate file | Defined inline within other code |
Readability | Offers better code readability | May lead to less readable code |
State and Behavior | Can have instance variables and methods | Can have instance variables and methods |
Reusability | Promotes code reusability | Typically used for single use cases |
Use-Cases: Anonymous Class in Java
Here are some common use-cases for anonymous classes in Java:
- Event Listeners: Anonymous classes are often used as event listeners to handle events such as button clicks, mouse actions, and key presses.
- Customizing Interfaces: When implementing interfaces with a single or limited usage, anonymous classes provide a convenient way to define the implementation inline.
- Thread Creation: Creating threads with the
Thread
class orRunnable
interface can be achieved using anonymous classes, allowing you to define therun()
method inline. - Comparator Implementation: Anonymous classes are useful for providing custom comparator implementations for sorting collections of objects.
- Test Implementations: In unit testing, you might need to create small mock implementations of interfaces to test certain methods.
- Lambda Expression Alternatives: Before the introduction of lambda expressions in Java 8, anonymous classes were commonly used to achieve similar functional behavior.
- Collections Initialization: When initializing collections, you might need to provide specific implementations for certain elements without creating separate classes.
- Singleton Patterns: Though less common, you can use anonymous classes to implement singleton patterns without defining a separate class.
Advantages : Anonymous Class in Java
Here are the main advantages of using Anonymous Class in Java:
- Concise Implementation: Anonymous class allows you to define and implement a class with a single instance in a compact and inline manner. This can make your code shorter and more focused.
- Inline Customization: They are well-suited for providing custom implementations of interfaces or subclasses with a specific behavior, directly where they are needed.
- Simplifying Code Structure: For small and localized implementations, using anonymous classes can simplify your code structure by avoiding the need to define separate classes for minor behavior.
- Reduced Boilerplate: Anonymous classes help reduce boilerplate code that you might otherwise need to write for defining new classes and their methods.
- On-the-Fly Customization: They allow you to customize a behavior, such as a callback or interface implementation, without cluttering the code with additional classes.
- Functional Style: In Java versions before 8, anonymous classes provided a way to achieve some functional programming style constructs, like providing behavior as arguments to methods.
Limitations: Anonymous Class in Java
Here are some few limitations of anonymous classes in Java:
- Limited Reusability: Anonymous classes are designed for one-time use and lack reusability. They can’t be instantiated multiple times or shared across different parts of your code.
- Lack of Explicit Name: Since anonymous classes don’t have explicit names, debugging and identifying issues in stack traces can be challenging.
- Inheritance Limitations: Anonymous classes can either extend a single class or implement a single interface, limiting their ability to inherit behavior from multiple sources.
- No Constructors: Anonymous classes don’t have constructors that you can use to initialize instance variables. This can make them less suitable for more complex scenarios.
- Limited Accessibility: Anonymous classes are restricted in terms of access modifiers. They can only be marked as
final
, and their access is limited to where they are defined. - Limited to Non-Static Contexts: Anonymous classes are typically used within non-static contexts like methods. They can’t define static members or be used in static methods.
- Limited to Single Instances: Each instance of an anonymous class is unique, so you can’t create multiple instances of the same anonymous class.
Conclusion : Anonymous Class in Java
To sum it up, we’ve delved into the world of Anonymous Class in Java, discovering their unique characteristics and advantages. These on-the-fly defined classes bring simplicity and concise implementation to your code.
With the ability to provide customized implementations inline, they enhance code readability and structure for small-scale needs. Their reduction in boilerplate code minimizes complexity, while their functional style empowers methods with behaviors.
However, it’s important to be aware of their limitations. Anonymous classes are best suited for one-time use, lacking the reusability of named classes. Debugging might pose challenges due to their absence of explicit names. Inheritance and constructors come with restrictions, constraining their versatility. Their accessibility and context usage are confined, and multiple instances of the same anonymous class cannot be created.
Knowing these little details helps you use anonymous classes for what they’re good at, and also remember their limits. This understanding helps you decide when and how to use them wisely in your Java projects.
Related Article: Static Nested and Inner Classes in Java