In this comprehensive guide on Adapter Design Pattern in Java, we will do in-depth exploration of different aspects of Adapter Design pattern in Java. So, let’s get started.
What is Adapter Design Pattern in Java ?
Adapter design pattern in Java is a Structural Design pattern helps in making two incompatible interfaces to work together.It is basically a bridge between two incompatible interfaces.We need to note the fact that here the interfaces may be incompatible but the inner functionality suits the requirement.
Real-life example: Memory Card reader acts like an adapter between a laptop and a memory card. Our mobile charger is actually an adapter between power supply and the mobile.The mobile charger converts the 220 volts from the power supply to around 9 volts which is needed by the mobile.
Class Diagram: Adapter Design Pattern in Java
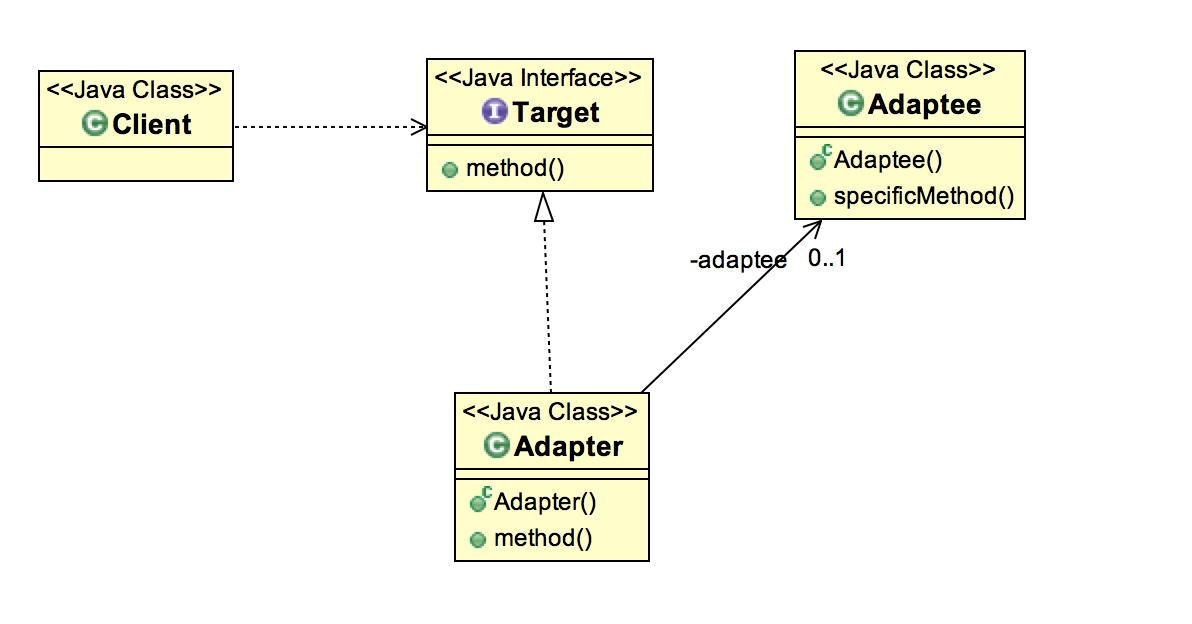
As seen in the above class diagram , we have the following four components.
- Client : This component actually needs the functionality.
- Target:This interface is used by the client for achieving the needed functionality.
- Adapter: This class implements the Target interface and it is responsible for getting the needed functionality from the adaptee.
- Adaptee: The component which has the functionality needed by the client.
When to use Adapter Design Pattern in Java
- This pattern can be used when our code is dependent on some external API which is prone to change frequently.We don’t have control on external libraries and they may change any time.Here we need to use adapter pattern,
so that we don’t get into trouble when an external library changes its code.Whenever any change happens in the external API, we need to make changes in the Adapter class only. - It is also useful when dealing with legacy code whose source code can’t be modified. So adapter pattern makes existing classes work with others without modifying their source code.
Example: Adapter Design Pattern in Java
Let us take a very simple example, wherein we need to use an existing Logger functionality whose interface does not quite match that of the client.We put the Adapter in between to act as the bridge between the client and the Logger class.
Class Diagram

Java Code
package com.design.adapter;
public interface ILogger {
public void warn(String message);
public void info(String message);
public void severe(String message);
}
package com.design.adapter;
import java.util.logging.Level;
public class LoggerAdapter implements ILogger {
private Logger logger;
public LoggerAdapter() {
this.logger = new Logger();
}
@Override
public void warn(String message) {
logger.logMesage(Level.WARNING, message);
}
@Override
public void info(String message) {
logger.logMesage(Level.INFO, message);
}
@Override
public void severe(String message) {
logger.logMesage(Level.SEVERE, message);
}
}
package com.design.adapter;
import java.util.logging.Level;
public class Logger {
public void logMesage(Level loglevel, String message) {
System.out.println(loglevel.getName() + ":" + message);
}
}
package com.design.adapter;
public class LoggerClient {
public static void main(String[] args) {
ILogger iLogger = new LoggerAdapter();
iLogger.info("Everything fine");
iLogger.warn("Something is not correct");
iLogger.severe("System is going down");
}
}
Output
INFO:Everything fine
WARNING:Something is not correct
SEVERE:System is going down
Advantages of the Adapter Design Pattern in Java
The Adapter Design Pattern in Java offers several advantages that make it a valuable tool for bridging the gap between incompatible interfaces or classes. Here are some key advantages of using the Adapter Design Pattern:
- Interoperability: The primary advantage of the Adapter pattern is its ability to allow different classes or interfaces to work together, even if they were not designed to do so. It enables components developed independently to communicate and cooperate effectively.
- Reuse of Existing Code: Adapters allow you to reuse existing classes or components that might have the desired functionality but don’t match the required interface. By creating an adapter, you can use these existing components without having to modify their code.
- Flexibility and Extendability: Adapters provide a way to introduce new functionality to existing classes or systems without altering their core implementation. This promotes a flexible and extendable design, as new adapters can be added to adapt new components in the future.
- Legacy System Integration: When integrating new systems with legacy code or systems, the Adapter pattern can be used to create adapters that allow new code to interact seamlessly with the old code. This avoids the need for extensive modifications to the legacy codebase.
- Separation of Concerns: The Adapter pattern promotes the separation of concerns by isolating the adaptation logic in the adapter class. This keeps the adaptation logic distinct from the main business logic, improving maintainability and readability.
- Enhanced Testability: Adapters can improve testability by allowing the use of mock objects or stubs during testing. By adapting interfaces to a common one, it becomes easier to substitute components with testing-specific implementations.
- Object Reusability: Adapters can make otherwise incompatible objects reusable in different contexts or projects, as long as their interfaces are adapted to match the required contracts.
- Client Simplification: Adapters simplify client code by allowing them to interact with a common interface. Clients don’t need to know about the internal complexities of the adapted classes.
- Migration Support: During the migration from one framework, library, or technology to another, adapters can facilitate the transition by allowing the new components to work with the old ones until a complete migration is achieved.
Disadvantages of the Adapter Design Pattern in Java
While the Adapter Design Pattern in Java offers several advantages, it also comes with certain disadvantages and considerations that developers should be aware of. Here are some key disadvantages of using the Adapter Design Pattern in Java:
- Complexity: The introduction of adapter classes can add complexity to the codebase. There’s an additional layer of abstraction that needs to be managed, which might make the overall design harder to understand and maintain.
- Performance Overhead: Adapters introduce an extra layer of indirection, which can result in a slight performance overhead. While this overhead is usually negligible, it might be a concern in highly performance-sensitive applications.
- Overuse: It’s possible to overuse the Adapter pattern, creating adapters for every interaction between different components. This can lead to excessive complexity and reduced clarity in the code.
- Maintenance Challenges: If the interfaces being adapted change frequently, maintaining the adapters to accommodate these changes can become a challenge. Each change in the adapted interface might require updates in the corresponding adapters.
Conclusion: Adapter Design Pattern in Java
In this comprehensive guide on the Adapter Design Pattern in Java, we’ve delved into various aspects of this structural design pattern. Let’s recap the key points discussed:
What is Adapter Design Pattern in Java? The Adapter Design Pattern in Java is a structural pattern that facilitates making two incompatible interfaces work together.
When to Use Adapter Design Pattern in Java: Adapter pattern is beneficial when dealing with external APIs that might change frequently, or when integrating legacy code that can’t be modified. It enables different components to interact without altering their source code.
Example: Adapter Design Pattern in Java: We explored a simple example where an existing Logger functionality with an incompatible interface was adapted using an adapter.
Advantages of the Adapter Design Pattern in Java: The Adapter Design Pattern offers several advantages, including interoperability, code reuse, flexibility, legacy system integration, separation of concerns, enhanced testability, object reusability, and simplification of client code.
Disadvantages of the Adapter Design Pattern in Java: Disadvantages include added complexity, potential performance overhead, overuse leading to code clutter, and challenges in maintaining adapters if adapted interfaces change frequently.
Adapter Design Pattern in Java is a powerful tool for bridging the gap between incompatible interfaces or classes. It enables components to collaborate effectively, reuses existing code, and enhances code flexibility. However, it should be used judiciously to avoid unnecessary complexity and maintenance challenges. By understanding its advantages and disadvantages, developers can effectively utilize this pattern to create more cohesive and adaptable software systems.
Must Read Articles: