In this article, we will look into the high level view of Java Collections Framework.
The Java Collections Framework is a powerful and extensive library built into the Java programming language. It provides a set of interfaces, classes, and algorithms to efficiently store, manipulate, and process collections of objects.
Collection Interface and Sub Interfaces

In the above figure, we have the Java Collections hierarchy , starting with the Collection interface.
Interface | Description | Concrete Classes |
---|---|---|
Collection | Prescribes the basic operations for a collection of objects. | |
Set | Extends Collection interface. Represents a set of unique elements. | HashSet, LinkedHashSet |
SortedSet | Extends Set interface. Stores unique elements in sorted order. | TreeSet |
List | Extends the Collection interface. Maintains a sequence of elements. | ArrayList, Vector, LinkedList |
Queue | Extends the Collection interface. Represents a collection designed for holding elements prior to processing. Supports operations like insertion, removal, and retrieval. | LinkedList, PriorityQueue |
Concrete Classes
Concrete Class | Interface | Is Duplicate Allowed? | Ordered/Sorted | Data Structure |
---|---|---|---|---|
ArrayList | List | Yes | Insertion Order | Resizable array |
LinkedList | List | Yes | Insertion Order | Linked list |
Vector | List | Yes | Insertion Order | Resizable array |
HashSet | Set | No | No Order | Hash table |
LinkedHashSet | Set | No | Insertion Order | Hash table and doubly-linked list |
TreeSet | SortedSet | No | Sorted | Balanced Tree |
LinkedList | Queue | Yes | Insertion Order | Linked list |
PriorityQueue | Queue | No | No Order | Heap |
Methods of Collection interface
Some commonly used methods in the Java Collection interface are mentioned below:
Method | Description |
---|---|
boolean add(E element) | Adds the specified element to the collection. |
boolean contains(Object o) | Returns true if the collection contains the specified element. |
boolean remove(Object o) | Removes the specified element from the collection. |
int size() | Returns the number of elements in the collection. |
boolean isEmpty() | Returns true if the collection is empty. |
void clear() | Removes all elements from the collection. |
Iterator<E> iterator() | Returns an iterator over the elements in the collection. |
boolean addAll(Collection<? extends E> c) | Adds all elements from the specified collection to the collection. |
boolean removeAll(Collection<?> c) | Removes all elements in the specified collection from the collection. |
boolean retainAll(Collection<?> c) | Retains only the elements in the collection that are contained in the specified collection. |
boolean containsAll(Collection<?> c) | Returns true if the collection contains all elements in the specified collection. |
Object[] toArray() | Returns an array containing all elements in the collection. |
boolean equals(Object o) | Compares the specified object with the collection for equality. |
int hashCode() | Returns the hash code value for the collection. |
Queue Hierarchy
Let us have a look into the Queue hierarchy.
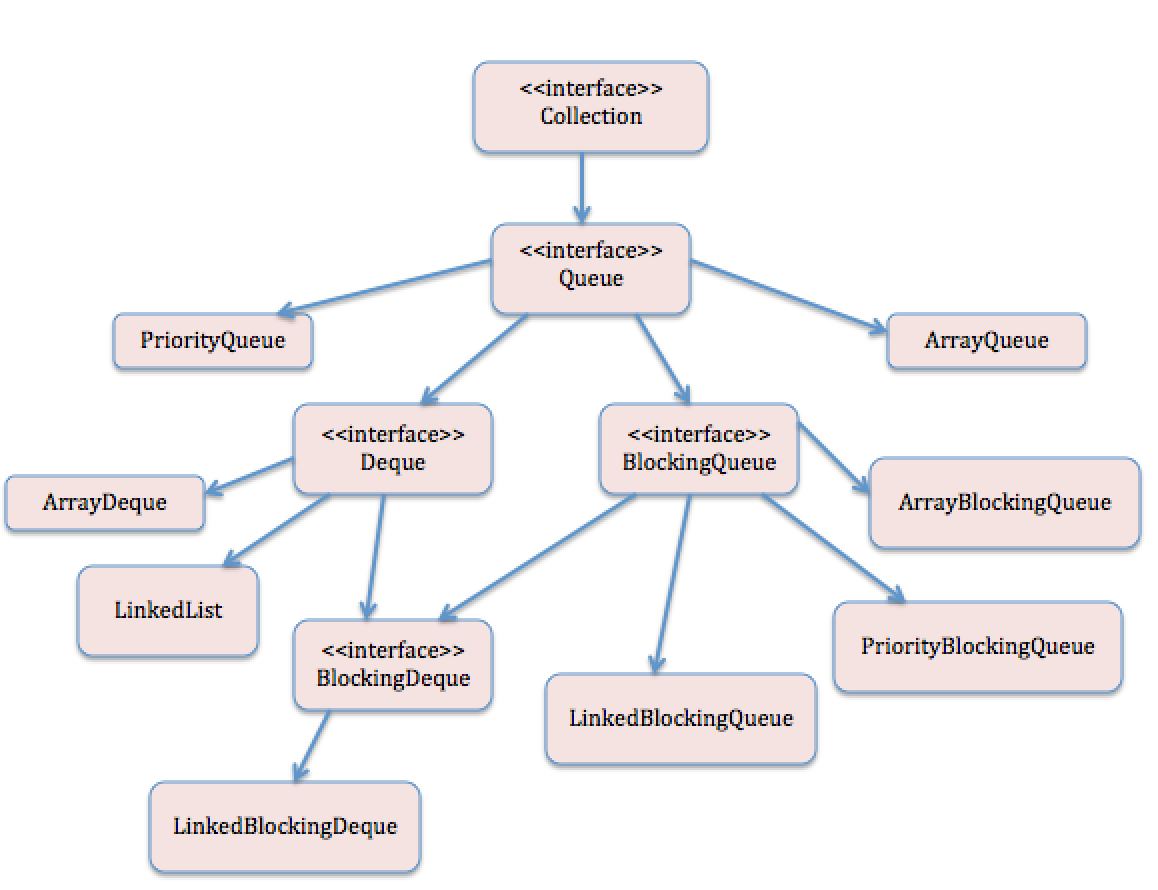
Interface | Description | Concrete Classes |
---|---|---|
Queue | It extends the Collection interface. Designed to have elements inserted at the end and removed from the beginning. | ArrayQueue, PriorityQueue |
Deque | Extends the Queue interface. Supports adding and removing elements from both ends. | ArrayDeque, LinkedList |
BlockingQueue | Extends the Queue interface. Supports blocking operations for waiting on elements and space availability. | ArrayBlockingQueue, PriorityBlockingQueue, LinkedBlockingQueue |
BlockingDeque | Extends both the Deque and BlockingQueue interfaces. Supports blocking operations for adding and removing elements. | LinkedBlockingDeque |
Let us look into some of the important implementations for Queue.
Concrete Class | Interface | Data Structure |
---|---|---|
ArrayQueue | Queue | Array |
PriorityQueue | Queue | Array |
ArrayBlockingQueue | BlockingQueue | Array |
PriorityBlockingQueue | BlockingQueue | Array |
LinkedBlockingQueue | BlockingQueue | Linked list |
ArrayDeque | Deque | Array |
LinkedList | Deque | Linked list |
LinkedBlockingDeque | BlockingDeque | Doubly linked list |
Map Interface
The Map
interface is a key component of the Java Collections Framework. It represents a collection of key-value pairs, where each key is unique and maps to a corresponding value. The Map
interface provides methods for adding, retrieving, and manipulating key-value pairs, making it a versatile data structure for various applications.
Please note that Map interface does not extend the Collection interface as Map is not a collection. It contains entries of key-value pairs.
Let us have a look into the Map hierarchy.

Interface | Description | Concrete Classes |
---|---|---|
Map | Defines operations for maintaining key-value mappings. | HashMap, Hashtable, LinkedHashSet |
SortedMap | Extends the Map interface. Mappings are sorted by key order. | TreeMap |
Concrete Class | Interface | Is Duplicate Key Allowed? | Ordered/Sorted | Data Structure |
---|---|---|---|---|
HashMap | Map | No | No Order | Hash table |
LinkedHashMap | Map | No | Key insertion order | Hash table and doubly-linked list |
Hashtable | Map | No | No Order | Hash table |
TreeMap | SortedMap | No | Sorted in Key order | Balanced Tree |
Java Iterator Interface
The Java Iterator
interface provides a way to iterate over elements in a collection sequentially. It allows you to traverse and access elements in a collection without exposing the underlying implementation details. The Iterator
interface is part of the Java Collections Framework and is widely used across various collection classes.
Here is an overview of some commonly used methods in the Iterator
interface:
Method | Description |
---|---|
boolean hasNext() | Returns true if there are more elements in the collection, otherwise returns false . |
E next() | Returns the next element in the collection. |
void remove() | Removes the last element returned by next() from the underlying collection (optional operation). |
default void forEachRemaining(Consumer<? super E> action) | Performs the specified action on each remaining element in the collection until all elements have been processed or an exception is encountered. |
To use an Iterator
, you typically obtain an instance of it by calling the iterator()
method on a collection object. Here’s an example that demonstrates the usage of Iterator
:
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
Iterator<String> iterator = names.iterator();
while (iterator.hasNext()) {
String name = iterator.next();
System.out.println(name);
if (name.equals("Bob")) {
iterator.remove();
}
}
In this example, we create an ArrayList
called names
and add three names to it. We obtain an iterator using the iterator()
method and use it to traverse the collection. The hasNext()
method checks if there are more elements, and the next()
method returns the next element in the collection. We can perform operations on each element, and if needed, remove elements using the remove()
method.
The Iterator
interface provides a standardized way to iterate over collections, making it easier to work with various types of collections in a consistent manner.
Conclusion: Java Collections Framework
In summary, the Collections in Java encompass a robust and all-encompassing framework consisting of interfaces, classes, and algorithms designed for efficient handling of object collections in the Java programming language. It provides developers with a comprehensive view of diverse data structures and algorithms, empowering them to effectively store, manipulate, and process collections of elements.
Throughout this article, we have delved into the fundamental components of the Java Collections Framework, including interfaces like Collection, List, Set, Queue, and Map, as well as their respective implementations.
By harnessing the power of the Java Collections Framework, developers can unlock the benefits of optimized performance, increased productivity, and elevated code quality, ultimately leading to more effective software development and delivery.