We can make a bean ineligible for auto wiring by specifying the autowireCandidate attribute as false in the Bean annotation like the below.
@Bean(autowireCandidate = false)
Class1 class1() {
return new Class1();
}
We will use Spring Boot for our example.
Here, we will create the bean of Class1 with autowireCandidate as false and then try to auto-wire the Class1 bean in Class2. Let’s see what happens.
Project Structure
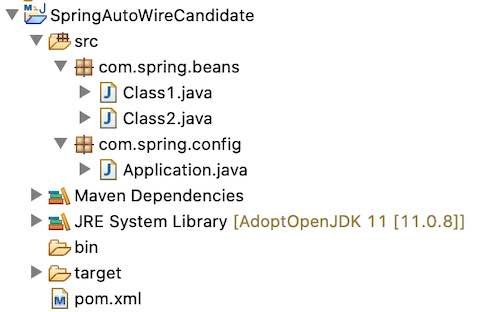
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>SpringAutoWireCandidate</groupId>
<artifactId>SpringAutoWireCandidate</artifactId>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.1.RELEASE</version>
<relativePath />
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
</project>
package com.spring.beans;
public class Class1 {
}
package com.spring.beans;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class Class2 {
@Autowired
private Class1 Class1;
public Class1 getClass1() {
return Class1;
}
public void setClass1(Class1 class1) {
Class1 = class1;
}
}
package com.spring.config;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Bean;
import com.spring.beans.Class1;
import com.spring.beans.Class2;
@SpringBootApplication(scanBasePackages="com.spring.beans")
public class Application implements CommandLineRunner{
@Autowired
private ApplicationContext context;
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean(autowireCandidate = false)
Class1 class1() {
return new Class1();
}
@SuppressWarnings("unused")
@Override
public void run(String... args) throws Exception {
Class1 class1 = context.getBean(Class1.class);
Class2 Class2 = context.getBean(Class2.class);
}
}
Output
***************************
APPLICATION FAILED TO START
***************************
Description:Field Class1 in com.spring.beans.Class2 required a bean of type ‘com.spring.beans.Class1’ that could not be found.
The injection point has the following annotations:- @org.springframework.beans.factory.annotation.Autowired(required=true)
The following candidates were found but could not be injected:- User-defined bean method ‘class1’ in ‘Application’
Action:Consider revisiting the entries above or defining a bean of type ‘com.spring.beans.Class1’ in your configuration.
So, the Spring Container could not find any bean for the Class1 for auto-wiring as we have made Class1 ineligible for auto-wiring by using autowireCandidate as false.
Code Download
Please go through the below related articles.