In this article, we will discuss the the importance of required = false attribute in @Autowired ie., @Autowired(required = false).
@Autowired annotation is used to inject dependency beans into a bean. You can also read @Autowired vs @Inject vs @Resource for more details.
The default value of the required attribute is true. So, when required = true, the injection of the dependency bean is mandatory. So in the absence of any auto-wiring candidate , the auto-wiring will fail and Spring will throw an exception.
In the below example, if there is no auto-wiring candidate or qualifying bean for Class1, then Spring will throw an example.
package com.spring.beans;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class Class2 {
@Autowired //required=true by default
private Class1 Class1;
public Class1 getClass1() {
return Class1;
}
public void setClass1(Class1 class1) {
Class1 = class1;
}
}
Field Class1 in com.spring.beans.Class2 required a bean of type 'com.spring.beans.Class1' that could not be found.
The injection point has the following annotations:
- @org.springframework.beans.factory.annotation.Autowired(required=true)
Action:
Consider defining a bean of type 'com.spring.beans.Class1' in your configuration.
So, in case you are not sure about the availability of auto-wiring for the dependency or if the dependency is not mandatory, then you can specify @Autowired(required=false).
Setting the required attribute to false indicates that the property is not required for autowiring purposes, and the property is ignored if it cannot be autowired.
Let’s build a small Java project for our use case.
Technology Stack
- Spring Boot
- Spring Core
- Core Java
Project Structure
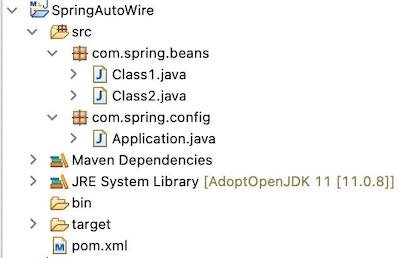
Code
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>SpringAutoWire</groupId>
<artifactId>SpringAutoWire</artifactId>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.1.RELEASE</version>
<relativePath />
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
</project>
package com.spring.beans;
public class Class1 {
}
package com.spring.beans;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class Class2 {
@Autowired(required = false) //Specfied required=false explicitly
private Class1 Class1;
public Class1 getClass1() {
return Class1;
}
public void setClass1(Class1 class1) {
Class1 = class1;
}
}
package com.spring.config;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
import com.spring.beans.Class2;
@SpringBootApplication(scanBasePackages = "com.spring.beans")
public class Application implements CommandLineRunner {
@Autowired
private ApplicationContext context;
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Override
public void run(String...args) throws Exception {
Class2 class2 = context.getBean(Class2.class);
System.out.println("Class1:" + class2.getClass1());
System.exit(1);
}
}
Class1:null
Download Code
Related Articles:
- Top 35 Microservices Interview Questions & Answers 2023
- Spring Boot Interview Questions: Ultimate Guide 2023
- Top 40 Java 8 Interview Questions & Answers 2023
- Basic Spring Annotations
Recommended Book: Spring in Action