In this article, we will explore different types of data structures in Java.
Introduction
Data structures are ordered and specialized formats used in programming for efficiently storing, managing, and manipulating data. They offer a systematic method of organizing and storing data elements, making it easier to conduct various actions on the data. Data structures serve as the foundation for software systems, allowing programmers to create efficient algorithms and optimize memory and processing resource usage.
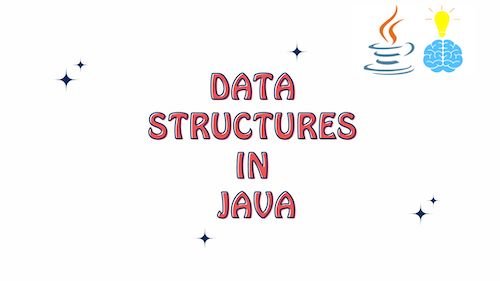
Different Types of Data Structures in Java
There are many different types of data structures in Java that can be used to organize and manipulate data in various ways. Here are some examples of common data structures:
- Array: An array is a fixed-size collection of elements of the same type. Arrays are useful for storing and manipulating a large amount of data in a single variable.
- Linked List: A linked list is a dynamic collection of elements that are linked together using pointers. Each element, called a node, contains a reference to the next element in the list. Linked lists are useful for storing and manipulating data that is frequently added or removed.
- Stack: A stack is a last-in-first-out (LIFO) data structure that allows elements to be added and removed from only one end. Stacks are useful for implementing algorithms such as recursion and undo/redo functionality.
- Queue: A queue is a first-in-first-out (FIFO) data structure that allows elements to be added at one end and removed from the other end. Queues are useful for implementing algorithms such as breadth-first search and task scheduling.
- Hash Table: A hash table is a data structure that allows for constant-time access to elements based on a unique key. Hash tables are useful for implementing efficient search and retrieval operations.
- Tree: A tree is a hierarchical data structure that consists of nodes and edges. Trees are useful for organizing and manipulating data in a hierarchical manner, such as in file systems and decision-making algorithms.
- Graph: A graph is a collection of nodes and edges that can be used to represent relationships between objects. Graphs are useful for modeling networks, such as social networks, transportation networks, and the internet.
- Trie: A trie (prefix tree) is a tree-based data structure that is used to store a collection of strings in a way that allows for efficient prefix-based search.
- Heap: A heap is a specialized tree-based data structure that is used to implement efficient algorithms for sorting and searching data.
Advantages of Data Structures in Java
Data structures in Java play a pivotal role in the efficient management and manipulation of data within software applications. These structures offer several advantages that significantly impact the performance, organization, and overall functionality of programs. Here are some key advantages of using data structures in Java:
- Efficient Data Access and Retrieval:
- Well-designed data structures enable quick and direct access to data elements. This efficiency is crucial for operations that involve searching, reading, or modifying data.
- Optimized Memory Utilization:
- Data structures allow for optimized memory allocation and usage. They ensure that memory is allocated only for the data elements needed, reducing wastage and enhancing memory management.
- Faster Data Manipulation:
- Specific data structures are tailored to perform certain operations efficiently. For example, arrays are excellent for random access, while linked lists are efficient for insertions and deletions.
- Enhanced Algorithm Performance:
- The choice of the right data structure can significantly improve the performance of algorithms. Efficient data structures lead to faster execution of algorithms, contributing to better overall program performance.
- Order and Organization:
- Data structures maintain order and organization among data elements. This is essential for scenarios where maintaining a specific sequence or arrangement is crucial.
- Data Integrity and Validity:
- By enforcing rules and constraints on data elements, data structures contribute to data integrity and validity. This prevents incorrect or inconsistent data from entering the system.
- Code Reusability and Maintainability:
- Well-defined data structures enable code reusability across different projects and scenarios. This streamlines development and maintenance efforts.
- Scalability and Growth:
- Suitable data structures allow applications to scale seamlessly as data volumes increase. This ensures that performance remains consistent as the application grows.
- Algorithmic Problem Solving:
- Proficiency in using data structures is essential for solving complex algorithmic problems efficiently. The right data structure can make intricate problems more approachable.
- Concise and Readable Code:
- Properly chosen data structures enhance code readability and maintainability. Code that uses appropriate data structures is often more intuitive and easier to understand.
Conclusion: Data Structures in Java
Data structures are fundamental tools that programmers use to effectively store, organize, and modify data in the broad world of programming. These frameworks allow for the development of sophisticated software solutions that are both powerful and effective through a complex dance of organization and algorithms.