In Java programming, one of the common task is to convert milliseconds to minutes and seconds. In this article, we will explore how to achieve this conversion using Java 8 features.
Prerequisites: To follow along with the examples in this article, you will need a basic understanding of Java programming and familiarity with Java 8’s Date and Time API.
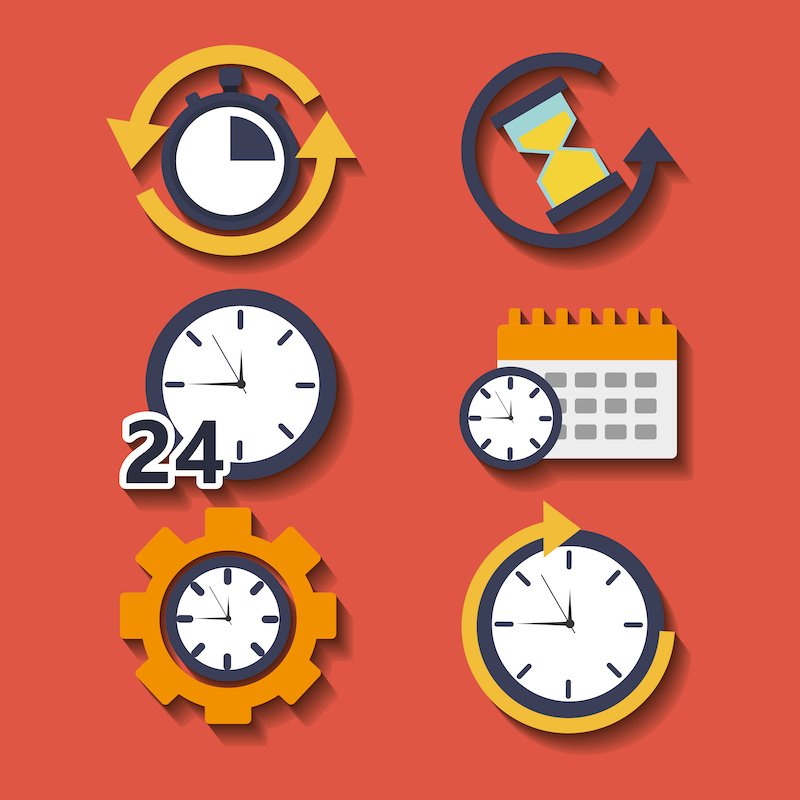
Convert Milliseconds to Minutes and Seconds Using Java 8’s Duration API
Step 1: Getting the Milliseconds Input:
First, we need to get the number of milliseconds as input from the user or any other data source. For the sake of simplicity, let’s assume we have a long variable named milliseconds that holds the value of milliseconds.
Step 2: Conversion Duration API:
Java 8 introduced the Duration class, which provides convenient methods for working with time intervals. To convert milliseconds to minutes and seconds, we can create a Duration object using the milliseconds and then extract the minutes and seconds components.
// Convert milliseconds to Duration
Duration duration = Duration.ofMillis(milliseconds);
// Extract minutes and seconds from Duration
long minutes = duration.toMinutes();
long seconds = duration.getSeconds() % 60;
Step 3: Displaying the Result: Once we have the minutes and seconds values, we can display them to the user or use them in further calculations. Here’s an example of how to display the converted values:
System.out.println("Converted Time:");
System.out.println("Minutes: " + minutes);
System.out.println("Seconds: " + seconds);
Example Usage: Let’s put it all together in a complete example:
import java.time.Duration;
public class MillisecondsToMinutesSecondsConverter {
public static void main(String[] args) {
long milliseconds = 1234567; // Example milliseconds value
// Convert milliseconds to Duration
Duration duration = Duration.ofMillis(milliseconds);
// Extract minutes and seconds from Duration
long minutes = duration.toMinutes();
long seconds = duration.getSeconds() % 60;
// Display the converted time
System.out.println("Converted Time:");
System.out.println("Minutes: " + minutes);
System.out.println("Seconds: " + seconds);
}
}
Converted Time:
Minutes: 20
Seconds: 34
Convert Milliseconds to Minutes and Seconds Using Java 8’s TimeUnit API
The TimeUnit class provides useful methods for time-related conversions. Here’s an alternative approach using TimeUnit:
import java.util.concurrent.TimeUnit;
public class MillisecondsToMinutesSecondsConverter {
public static void main(String[] args) {
long milliseconds = 1234567; // Example milliseconds value
// Convert milliseconds to minutes and seconds
long minutes = TimeUnit.MILLISECONDS.toMinutes(milliseconds);
long seconds = TimeUnit.MILLISECONDS.toSeconds(milliseconds) % 60;
// Display the converted time
System.out.println("Converted Time:");
System.out.println("Minutes: " + minutes);
System.out.println("Seconds: " + seconds);
}
}
Converted Time:
Minutes: 20
Seconds: 34
In this approach, we use the TimeUnit.MILLISECONDS.toMinutes(milliseconds) method to convert the milliseconds directly to minutes. Similarly, TimeUnit.MILLISECONDS.toSeconds(milliseconds) is used to convert the milliseconds to seconds. The % 60 operation is applied to calculate the remaining seconds after converting to minutes.
Conclusion
Both approaches achieve the same result, and you can choose the one that suits your coding style or specific requirements.