The Java forEach method provides a convenient and expressive way to iterate over elements in a collection and perform an action on each element. In this article, we will delve into the details of the forEach
method in Java 8, its benefits, and various examples to understand its usage in different scenarios.
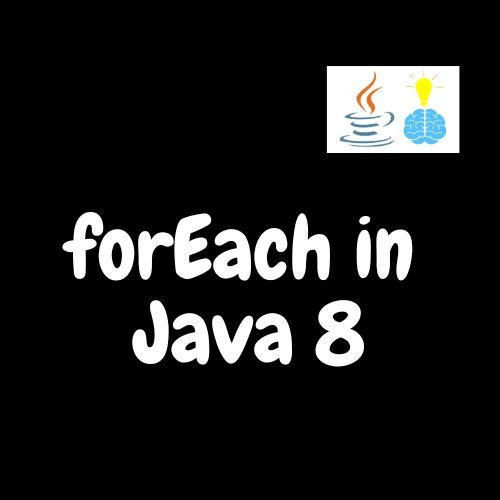
Different ways of using Java forEach method
Iterating over a List
The forEach
method can be used to iterate over a List
and perform an action on each element. Let’s consider an example where we have a list of names, and we want to print each name to the console.
Java Code
import java.util.Arrays;
import java.util.List;
public class ListIterationExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Alice", "Michael");
names.forEach(name -> System.out.println(name));
}
}
Output
John
Alice
Michael
Iterating over a Set
Here’s an example of iterating over a Set
using the forEach
method in Java:
import java.util.HashSet;
import java.util.Set;
public class SetIterationExample {
public static void main(String[] args) {
Set<String> fruits = new HashSet<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
fruits.forEach(fruit -> System.out.println(fruit));
}
}
Output:
Apple
Banana
Orange
Iterating over a Map
The forEach
method can be used to iterate over a Map
and perform an action on each key-value pair. Let’s consider an example where we have a map of products and their prices, and we want to print each product along with its price.
Java Code
import java.util.HashMap;
import java.util.Map;
public class MapIterationExample {
public static void main(String[] args) {
Map<String, Double> products = new HashMap<>();
products.put("Apple", 1.99);
products.put("Banana", 0.99);
products.put("Orange", 2.49);
products.forEach((product, price) -> System.out.println(product + ": $" + price));
}
}
Output
Apple: $1.99
Banana: $0.99
Orange: $2.49
Benefits of using Java forEach method
Using the Java forEach method provides several benefits and follows best practices for coding. Let’s explore some of them:
- Improved Readability: The
forEach
enhances code readability by expressing the intent of iterating over elements and performing an action on each element in a clear and concise manner. It eliminates the need for traditional loops, making the code more declarative and expressive. - Streamlined Data Processing: With
forEach
, you can easily process collections of data without the need for manual iteration. It abstracts away the details of iteration, allowing you to focus on the action to be performed on each element. This streamlining of data processing leads to cleaner and more efficient code. - Functional Programming Paradigm: The
forEach
method aligns with the functional programming paradigm introduced in Java 8. It promotes immutability and separation of concerns by treating the collection as a stream of data and applying operations on each element in a declarative manner. This approach results in more maintainable and modular code. - Avoidance of External Iterators: The use of
forEach
eliminates the need for explicit external iterators, such asfor
orwhile
loops. By encapsulating iteration within theforEach
method, it reduces the chances of common iterator-related errors, such as off-by-one errors or infinite loops. - Concise and Efficient Code:
forEach
provides a compact and efficient way to process collections, reducing the amount of boilerplate code required for iteration. It enables you to focus on the logic of the action to be performed, leading to more readable and efficient code.
Best Practices for forEach in Java 8
- Ensure that the action passed to
forEach
is stateless and does not have side effects. This ensures the functional purity of the operation. - Leverage method references when possible to enhance code readability and promote reusability.
- Be cautious when using
forEach
in parallel streams, as the order of element processing may not be guaranteed. - Consider the use of other stream operations, such as
filter
ormap
, in combination withforEach
to perform more complex transformations on the data.
By following these best practices, you can harness the full potential of forEach
and create code that is easier to understand, maintain, and debug.
Conclusion
The forEach
in Java 8 provides a powerful and concise way to iterate over collections and perform actions on each element. It promotes a functional programming style and reduces boilerplate code. By leveraging the Java forEach method, developers can write more expressive and readable code, enhancing productivity and improving the overall quality of their Java programs.