In this article, we will delve into the concept of daemon thread in Java, explore their properties and uses, learn how to create them, check if a thread is a daemon thread, and see examples that demonstrate their behavior.
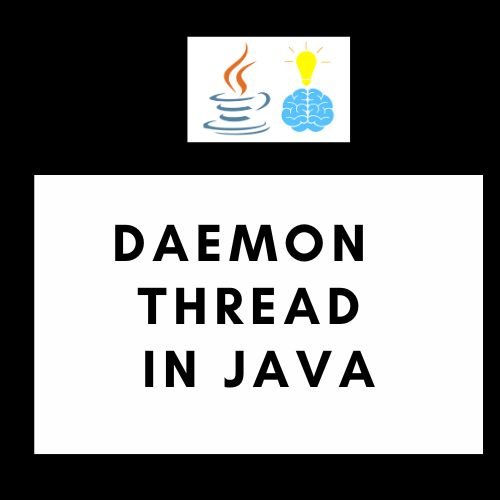
What is a Daemon Thread in Java?
A daemon thread in Java is a type of thread that runs in the background, providing support to user threads (also known as non-daemon threads).Daemon threads serve to perform tasks independently in the background.
What are the different properties of Daemon Thread?
The Daemon thread in Java has the following properties:
- Background Execution: Daemon threads run in the background and do not need user interaction.
- Automatic Termination: When all non-daemon threads complete their execution, the JVM automatically terminates daemon threads.
- Less Critical Tasks: Daemon threads are ideal for tasks that are supportive in nature and not critical for the application’s main functionality.
Usage of Daemon Thread
Some common uses of daemon thread in Java include:
- Garbage Collection: Daemon threads are involved in garbage collection activities, helping manage memory by removing unused objects.
- Background Processing: Tasks such as logging, event tracking, and data synchronization can be performed by daemon threads in the background, without affecting the primary tasks.
- Monitoring and Maintenance: Daemon threads can be used for monitoring system health, executing periodic maintenance tasks, or checking resource availability.
Methods for Daemon Thread
The Thread
class in Java provides two important methods related to daemon threads.
Method | Description |
---|---|
setDaemon(boolean on) | Marks the thread as a daemon thread if the parameter on is true , and as a user thread if the parameter is false . A daemon thread is intended to support user threads and does not prevent the JVM from exiting if they are the only threads left. |
isDaemon() | Returns true if the thread is a daemon thread, and false if it is a user thread. |
Java Code Example
Let’s create a Daemon Thread in Java in the below code.
public class DaemonThreadExample {
public static void main(String[] args) {
Thread daemonThread = new Thread(() -> {
while (true) {
System.out.println("Daemon thread is running.");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
daemonThread.setDaemon(true);
daemonThread.start();
System.out.println("Is daemonThread a daemon thread? " + daemonThread.isDaemon());
// Delaying the main thread to observe the behavior
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Output
Is daemonThread a daemon thread? true
Daemon thread is running.
Daemon thread is running.
Daemon thread is running.
Daemon thread is running.
In this example, we create a daemon thread in Java that keeps running in the background, while the main thread waits for 5 seconds. As soon as the main thread completes, the program terminates, and the daemon thread is stopped automatically.
Daemon Thread vs. User Thread
Property | Daemon Thread | User Thread |
---|---|---|
Creation | Created using the setDaemon(true) method while defining the thread | Created without specifying the daemon status |
Purpose | Support user threads and perform background tasks | Execute critical operations and interact with the user |
Priority | Typically has lower priority than user threads | Priorities can be adjusted as needed |
Impact on JVM | Does not prevent JVM from exiting if they are the only threads left | Prevent JVM from exiting until all user threads are finished |
Termination | Automatically terminated if no user threads are running | Continue running until their tasks are completed or explicitly stopped by code |
Execution Order | Lower priority in execution compared to user threads | Execution order can be managed using thread priorities |
Usage | Ideal for tasks like garbage collection and background tasks | Suitable for performing operations that require user interaction |
Parent-Child Relationship | Can be set as a child thread of a user thread | Can create child threads of user threads |
Handling Uncaught Exceptions | Any uncaught exception leads to an unexpected termination of the thread | Uncaught exceptions can be handled using a thread’s uncaught exception handler |
FAQ
- What is the purpose of daemon threads in Java? Daemon threads perform background tasks without direct user interaction, making them ideal for non-critical tasks that auto-terminate when user threads finish execution.
- Can we set a user thread as a daemon thread after starting it? No, once a thread is started, you cannot change its status from user to daemon or vice versa. The
setDaemon()
method should be called before starting the thread. - Can we prevent a daemon thread from terminating automatically? No, daemon threads are designed to terminate automatically when all non-daemon threads have completed their execution.
- How can I handle exceptions in daemon threads? It’s essential to handle exceptions properly in daemon threads using try-catch blocks. Uncaught exceptions in daemon threads can lead to unexpected program termination.
- Are daemon threads suitable for long-running tasks? No, daemon threads are not ideal for long-running tasks, especially if they require continuous execution. Long-running tasks should be assigned to user threads to ensure they complete successfully.
- Can a daemon thread prevent a user thread from completing its execution? No, daemon threads do not interfere with the execution of user threads. User threads will complete their execution regardless of the status of daemon threads.
- Can we set a daemon thread as a child of a user thread? Yes, daemon thread in Java can be set as children of user threads. If the parent user thread completes before the daemon child thread, the child continues to run with other non-daemon threads.
- Can a daemon thread access resources created by user threads? Yes, daemon threads can access resources created by user threads.
- Can we change the priority of a daemon thread? Yes, you can change the priority of a daemon thread using the
setPriority(int newPriority)
method of theThread
class. - Can multiple daemon threads be running simultaneously? Yes, multiple daemon threads can run concurrently, performing their background tasks.
Conclusion
Throughout this article, we explored the concept of daemon thread in Java, their properties, and various ways to create and identify them. We also looked into the differences between daemon threads and user threads, understanding when and where to use each type effectively.
In summary, mastering the concept of daemon thread in Java opens up possibilities for efficiently handling background tasks, enhancing the overall performance and user experience of Java applications.