In this article “HashMap vs Hashtable”, we will explore the differences between HashMap
and Hashtable
, along with code examples and scenarios where each is more suitable.
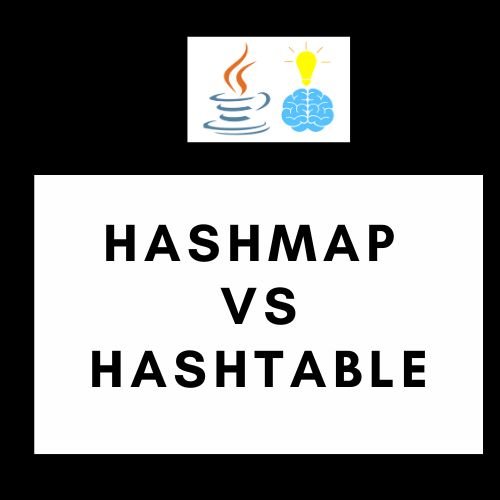
What is HashMap?
HashMap
is a part of the Java Collections Framework and is used to store elements in key-value pairs. HashMap
allows null values for both keys and values and is not synchronized, making it more efficient in single-threaded scenarios.
What is Hashtable?
Hashtable
, like HashMap
, is used to store key-value pairs, but it is a legacy class and not part of the Java Collections Framework. It is synchronized, making it thread-safe for use in multi-threaded environments. Unlike HashMap
, Hashtable
does not allow null values for keys or values.
HashMap vs Hashtable: Key Differences
Here are the key differences between
and HashMap
:Hashtable
Feature | HashMap | Hashtable |
---|---|---|
Introduction | Introduced in JDK 1.2 | Legacy class introduced in JDK 1.0 |
Synchronization | Not synchronized – Not thread-safe | Synchronized – Thread-safe for multi-threaded use |
Null Keys/Values | Null keys/values are allowed | Null keys/values are not allowed |
Performance | Faster due to no synchronization | Slower due to synchronization |
Iterator | Iterator used for iteration | Enumeration used for iteration |
Inheritance | Extends AbstractMap class | Extends Dictionary class |
Iterating Order | Iterator may not guarantee insertion order | Enumeration does not guarantee any order |
Java Code Example: HashMap vs Hashtable
Let’s see a code example to illustrate the differences between
and HashMap
:Hashtable
import java.util.*;
public class HashTableVsHashMapExample {
public static void main(String[] args) {
// Hashtable example
Hashtable<Integer, String> ht = new Hashtable<>();
ht.put(1, "One");
ht.put(2, "Two");
ht.put(3, "Three");
// HashMap example
HashMap<Integer, String> hm = new HashMap<>();
hm.put(1, "One");
hm.put(2, "Two");
hm.put(3, "Three");
// Iterating through Hashtable
System.out.println("Hashtable:");
Enumeration<Integer> htKeys = ht.keys();
while (htKeys.hasMoreElements()) {
Integer key = htKeys.nextElement();
System.out.println("Key: " + key + ", Value: " + ht.get(key));
}
// Iterating through HashMap
System.out.println("\nHashMap:");
for (Map.Entry<Integer, String> entry : hm.entrySet()) {
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
}
}
Output
Hashtable:
Key: 3, Value: Three
Key: 2, Value: Two
Key: 1, Value: One
HashMap:
Key: 1, Value: One
Key: 2, Value: Two
Key: 3, Value: Three
When to Use HashMap and When to Use Hashtable
- Use
HashMap
when you need a faster and more efficient data structure for single-threaded scenarios and are sure about no concurrent modifications. - Use
Hashtable
when you require thread-safe operations and synchronization for multi-threaded environments. - If you need the ability to store null values for keys or values, use
HashMap
, asHashtable
does not allow nulls.
Conclusion: HashMap vs Hashtable
In this article “HashMap vs Hashtable”, we looked into key differences between a HashMap and a Hashtable. We then implemented a Java class to show the differences between HashMap and Hashtable.
In summary, both Hashtable
and HashMap
are used to store key-value pairs in Java, but they have significant differences in their implementation and behavior. Hashtable
is a legacy class that provides synchronization, making it thread-safe, while HashMap
does not provide synchronization for better performance in single-threaded scenarios.
Consider your specific requirements and the environment in which the data structure will be used to decide between Hashtable
and HashMap
in your Java applications.