In this article, we will look into various types of Classes in Java. So, let’s get started.
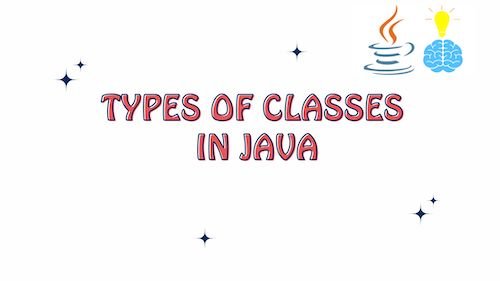
Different Types of Classes in Java
We have the following different types of Classes in Java
- Final Class
- Abstract Class
- Concrete Class
- Static Nested Class
- Inner Class
- Local Inner Class
- Anonymous Class
- Singleton Class
- Immutable Class
- POJO Class
Final Class
The Class marked with final keyword can not be inherited by a sub class. In simple words, the final class can not be extended.
public final class FinalClass {}
You can not do the below.
class childChild extends FinalClass{}
You will get compilation error.
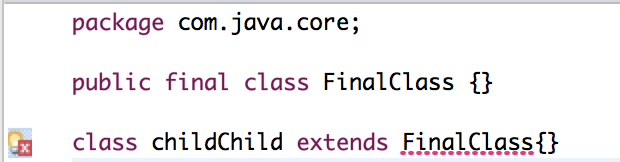

Final classes are not intended to be extended, such as utility classes or classes that have a specific implementation that should not be modified.
Read More : final keyword in Java: Valuable Insights
Abstract Class
An abstract class in Java is a class that cannot be instantiated on its own and is designed to serve as a blueprint or template for other classes.
It may contain both abstract (unimplemented) methods and concrete (implemented) methods.
Abstract classes provide a way to define common behavior and structure that can be shared among multiple subclasses, while allowing each subclass to provide its own specific implementations.
Here’s a simple example Abstract class in Java.
abstract class Animal {
// Abstract method
abstract void makeSound();
// Concrete method
void sleep() {
System.out.println("Zzz...");
}
}
class Dog extends Animal {
// Providing implementation for the abstract method
void makeSound() {
System.out.println("Dog barks!");
}
}
class Cat extends Animal {
// Providing implementation for the abstract method
void makeSound() {
System.out.println("Cat meows!");
}
}
class Main {
public static void main(String[] args) {
Dog dog = new Dog();
Cat cat = new Cat();
dog.makeSound();
dog.sleep();
cat.makeSound();
cat.sleep();
}
}
Output
Dog barks!
Zzz...
Cat meows!
Zzz...
Read More : Abstract Class in Java: Comprehensive Guide
Concrete Class
A concrete class in Java is a class that can be instantiated, meaning you can create objects of this class. It provides concrete implementations of methods defined in its interfaces or abstract superclasses. Here’s an explanation along with a code example:
// Abstract class with an abstract method
abstract class Shape {
abstract void draw(); // Abstract method
}
// Concrete class that extends the abstract class and provides implementations
class Circle extends Shape {
@Override
void draw() {
System.out.println("Drawing a circle");
}
}
class Rectangle extends Shape {
@Override
void draw() {
System.out.println("Drawing a rectangle");
}
}
// Main class to demonstrate using concrete classes
public class Main {
public static void main(String[] args) {
Shape circle = new Circle();
Shape rectangle = new Rectangle();
circle.draw(); // Output: Drawing a circle
rectangle.draw(); // Output: Drawing a rectangle
}
}
n this example, we have an abstract class Shape
with an abstract method draw()
. We then have two concrete classes Circle
and Rectangle
that extend the Shape
class and provide implementations for the draw()
method. These concrete classes can be instantiated, and objects of Circle
and Rectangle
can be created.
Static Nested Class
We can create a class within a class. Nested classes that are declared static are called static nested classes.
- static nested classes do not have access to any instance members of the enclosing outer class; it can only access them through an object’s reference
- static nested classes can access all static members of the enclosing class, including private ones
- Java programming specification doesn’t allow us to declare the top-level class as static; only classes within the classes (nested classes) can be made as static
- We don’t need to create an instance of Outer class for creating an instance of the static class.
Code Example
class OuterClass {
// Outer class members and methods
// Static nested class
static class NestedClass {
void display() {
System.out.println("This is a static nested class.");
}
}
}
public class Main {
public static void main(String[] args) {
// Creating an instance of the static nested class
OuterClass.NestedClass nestedObj = new OuterClass.NestedClass();
nestedObj.display(); // Output: This is a static nested class.
}
}
In this example, we have an OuterClass
that contains a static nested class named NestedClass
. The NestedClass
is accessed using the syntax OuterClass.NestedClass
, and you can create an instance of it just like you would with any other class.
Read More : Static Nested and Inner Classes in Java
Non-static Nested Class (Inner Class)
Nested classes that are non-static are called inner classes.
- It has access to all members of the outer class, including both static and instance members.
- It is accessed using an instance of the outer class:
outerInstance.new InnerClass()
. - It can be instantiated only within an instance of the outer class.
- Useful for creating logically related classes that need access to each other’s members.
Here’s an example of a non-static nested class (inner class) in Java:
class OuterClass {
private int outerData = 10;
// Inner class
class InnerClass {
void display() {
System.out.println("Inner class accessing outer data: " + outerData);
}
}
}
public class Main {
public static void main(String[] args) {
OuterClass outer = new OuterClass();
OuterClass.InnerClass inner = outer.new InnerClass();
inner.display(); // Output: Inner class accessing outer data: 10
}
}
In this example, the OuterClass
contains an inner class called InnerClass
. The inner class can access both instance variables and methods of the outer class, including private members, as demonstrated by the display()
method accessing the outerData
variable. To instantiate the inner class, you need to create an instance of the outer class and then use that instance to create an instance of the inner class.
Local Inner Class
Local inner class in Java is a class that is defined within a method or a block of code (such as a loop or conditional statement) inside another class or method.
- Local classes can only be accessed from inside the method or scope block in which they are defined.
- Local classes can access members (fields and methods) of its enclosing class just like regular inner classes.
- Local classes can also access local variables inside the same method or scope block, provided these variables are declared final.
- Local classes can also be declared inside static methods. In that case the local class only has access to the static parts of the enclosing class.
Example
package com.java.core;
public class LocalInnerClassTest {
public void localMethod() {
class Local {
void localTestMethod() {
System.out.println("Inside Local Test Method");
}
}
Local local = new Local();
local.localTestMethod();
}
public static void main(String[] args) {
LocalInnerClassTest localInnerClassTest = new LocalInnerClassTest();
localInnerClassTest.localMethod();
}
}
Output
Inside Local Test Method
Read More : Local Inner Class in Java: Complete Guide
Anonymous Class
An anonymous class is a nested class that’s defined on the fly without a name.
We can instantiate an anonymous class from
- A Class (may be abstract or concrete).
- An Interface
Java Code Example
package com.java.core;
class TestClass {
void testmethod() {}
}
abstract class TestAbstract {
abstract void abstractMethod();
}
interface TestInterface {
void interfaceMethod();
}
public class AnonymousExample {
public static void main(String[] args) {
TestClass testClass = new TestClass() {
@Override
public void testmethod() {
System.out.println("Inside Test Method");
}
};
testClass.testmethod();
TestAbstract testAbstract = new TestAbstract() {
public void abstractMethod() {
System.out.println("Inside abstract Method");
}
};
testAbstract.abstractMethod();
TestInterface testInterface = new TestInterface() {
public void interfaceMethod() {
System.out.println("Inside interface Method");
}
};
testInterface.interfaceMethod();
}
}
Output
Inside Test Method
Inside abstract Method
Inside interface Method
Read More : Anonymous Class in Java: Comprehensive Guide
Singleton Class
Singleton class in Java ensures a class has only one instance and provides a global point of access to that instance.
Code Example
public class Singleton {
// Private static instance variable
private static Singleton instance;
// Private constructor to prevent external instantiation
private Singleton() {
// Initialization code, if needed
}
// Public static method to provide access to the single instance
public static Singleton getInstance() {
if (instance == null) {
// Create a new instance if it doesn't exist
instance = new Singleton();
}
return instance;
}
// Other methods and variables can be added here
}
public class Main {
public static void main(String[] args) {
// Get the instance of the Singleton class
Singleton singleton1 = Singleton.getInstance();
Singleton singleton2 = Singleton.getInstance();
// Both instances are the same
System.out.println(singleton1 == singleton2); // Output: true
}
}
In this example, the Singleton
class follows the Singleton design pattern, ensuring that only one instance of the class can be created. The private constructor prevents external instantiation, and the getInstance()
method provides access to the single instance. The first time getInstance()
is called, it creates an instance of the class, and subsequent calls return the same instance. This ensures that there’s only one instance of the Singleton
class throughout the application.
Read More : Singleton Class in Java: Deep Insights
Immutable Class
An immutable class in Java is one whose instances cannot be changed after they have been created. This means that once an immutable class object is formed, its internal state cannot be altered.
For creating an immutable class in Java, we need to do the following things:
1. Make the class final
2. Make all the fields private and final.
3.Don’t provide any method that allows to change the values of the fields. For example don’t have any setter methods.Only getters should be there.
Java Code Example
public final class ImmutablePerson {
private final String name;
private final int age;
public ImmutablePerson(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Read More : Immutable Class in Java
POJO Class
A POJO (Plain Old Java Object) class is a Java class that encapsulates data and provides getter and setter methods to access and modify that data. POJOs are used to represent entities, data structures, or data transfer objects in a Java application. They are typically used in various layers of an application, such as the data access layer or the user interface layer.
Key characteristics of a POJO class include:
- Plainness: A POJO is a standard Java class without any special annotations or dependencies on specific frameworks.
- Encapsulation: It encapsulates data by using private instance variables and provides getter and setter methods to access and modify that data.
- Serializable: POJOs often implement the
Serializable
interface to enable serialization and deserialization. - No Business Logic: POJOs focus on representing data and do not contain complex business logic. They are primarily used for holding and transferring data between different parts of an application.
Java Code Example
public class Student {
// Private instance variables
private String firstName;
private String lastName;
private int age;
// Default constructor
public Student() {
// Default constructor
}
// Parameterized constructor
public Student(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
// Getter and setter methods for instance variables
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
// toString method for object representation
@Override
public String toString() {
return "Student{" +
"firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
", age=" + age +
'}';
}
// Other methods can be added here
}
Conclusion : Types of Classes in Java
In this article, we delved into different types of Classes in Java. We covered a spectrum of class types, from the unmodifiable nature of Final Classes to the flexible structure of POJO Classes. We delved into the concepts of Abstract Classes, Concrete Classes, Static Nested Classes, Inner Classes, Local Inner Classes, Anonymous Classes, Singleton Classes, and Immutable Classes, each bringing its own unique purpose and utility.