In this article, we will understand Inheritance in Java and look into various types of Inheritance in Java. So, let’s get started.
What is Inheritance in Java ?
Inheritance in Java is a fundamental concept of Object-Oriented Programming (OOP) that allows a new class to inherit the properties and behaviors (methods and fields) of an existing class, known as the “parent” or “superclass.” The class that inherits these properties is called the “child” or “subclass.” Inheritance establishes a “is-a” relationship between classes, indicating that a subclass is a specialized version of its superclass.
Key points about Inheritance in Java
Let’s look into the below key points about inheritance in Java:
- Code Reusability: Inheritance promotes code reusability by allowing subclasses to inherit and reuse the methods and fields defined in the superclass. This avoids redundant code writing and improves maintainability.
- Extending Functionality: Subclasses can extend the functionality of the superclass by adding new methods or fields specific to their needs.
- Overriding Methods: Subclasses can override (modify) the methods inherited from the superclass to provide specialized implementations. This enables polymorphism, where different objects of the same superclass can exhibit different behaviors.
- Access Control: In Java, access modifiers (public, protected, default, private) control the visibility of superclass members in subclasses. Subclasses can access public and protected members, but private members are not directly accessible.
- Constructors: Constructors of the superclass are not inherited by subclasses. However, a subclass constructor can call a superclass constructor using the
super
keyword to initialize inherited members. - Single Inheritance: Java supports single inheritance, which means a class can inherit from only one superclass. This helps avoid ambiguity and complexity in the class hierarchy.
- Super Keyword: The
super
keyword is used to refer to the superclass, allowing access to its methods, fields, and constructors. It is often used to call superclass constructors or methods from the subclass. - IS-A Relationship: Inheritance establishes an “IS-A” relationship between the superclass and subclass. For example, if you have a
Vehicle
superclass and aCar
subclass, you can say that “a Car is a Vehicle.”
What are different types of Inheritance in Java ?
In Java, inheritance can be categorized into the below types.
- Single Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Multiple Inheritance
- Hybrid Inheritance
Single Inheritance in Java
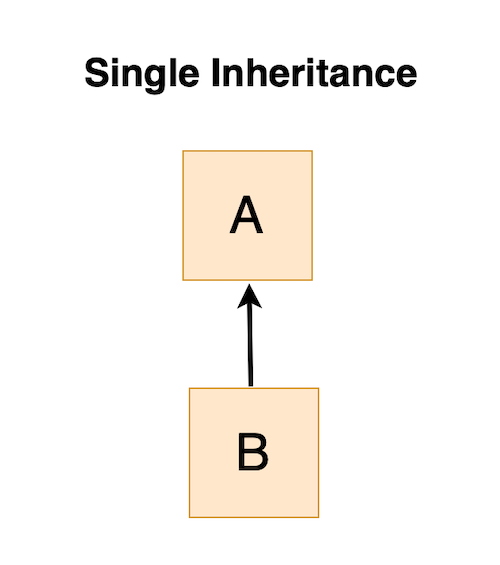
Single inheritance in Java refers to the situation where a class can inherit the traits and behavior of only one superclass. This means that a subclass can have only one immediate parent class.
The superclass from which the subclass inherits is often referred to as the base class, superclass, or parent class. Similarly, the class that inherits from the superclass is known as the derived class, child class, or subclass.
Single inheritance helps maintain simplicity and clarity in the class hierarchy.
Java Code Example: Single Inheritance in Java
// Base class (Superclass)
class Animal {
void eat() {
System.out.println("The animal is eating.");
}
}
// Derived class (Subclass)
class Dog extends Animal {
void bark() {
System.out.println("The dog is barking.");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.eat(); // Calling the inherited method from the base class
dog.bark(); // Calling the method from the derived class
}
}
In this example, we have a base class Animal
with a method eat()
. The Dog
class is a derived class that inherits from the Animal
class. It also has its own method bark()
. In the Main
class, we create an instance of Dog
and call both the inherited eat()
method from the Animal
class and the bark()
method from the Dog
class.
Multi-level Inheritance in Java

Multi-level inheritance in Java refers to a scenario where a class inherits from another class, which in turn inherits from another class.
This creates a chain of classes. Each class in this chain becomes the subclass of the class above it, and it can inherit both the attributes and methods of its immediate parent class as well as its grandparent class, and so on.
Here’s an example of multi-level inheritance in Java:
// Grandparent class
class Animal {
void eat() {
System.out.println("The animal is eating.");
}
}
// Parent class
class Dog extends Animal {
void bark() {
System.out.println("The dog is barking.");
}
}
// Child class
class Labrador extends Dog {
void playFetch() {
System.out.println("The Labrador is playing fetch.");
}
}
public class Main {
public static void main(String[] args) {
Labrador labrador = new Labrador();
labrador.eat(); // Inherited from Animal
labrador.bark(); // Inherited from Dog
labrador.playFetch(); // Method of the Labrador class
}
}
In this example, we have a class hierarchy with Animal
as the grandparent class, Dog
as the parent class inheriting from Animal
, and Labrador
as the child class inheriting from Dog
. The Labrador
class can access methods from both its parent class (Dog
) and its grandparent class (Animal
), creating a chain of inheritance.
Hierarchical Inheritance in Java
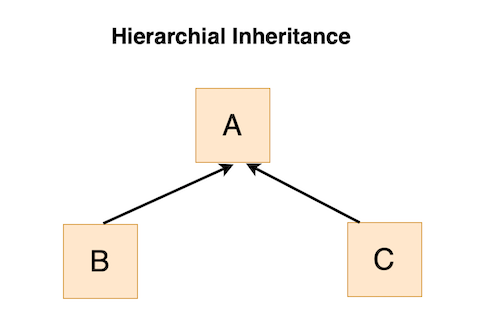
Hierarchical inheritance in Java refers to a situation where a single parent class is inherited by multiple child classes. In other words, multiple classes inherit from the same superclass, creating a hierarchy or tree-like structure. Each child class will have its own set of additional attributes and methods while also inheriting the attributes and methods of the common parent class.
Here’s an example of hierarchical inheritance in Java:
// Parent class
class Shape {
void draw() {
System.out.println("Drawing a shape.");
}
}
// Child class 1
class Circle extends Shape {
void drawCircle() {
System.out.println("Drawing a circle.");
}
}
// Child class 2
class Rectangle extends Shape {
void drawRectangle() {
System.out.println("Drawing a rectangle.");
}
}
// Child class 3
class Triangle extends Shape {
void drawTriangle() {
System.out.println("Drawing a triangle.");
}
}
public class Main {
public static void main(String[] args) {
Circle circle = new Circle();
circle.draw(); // Inherited from Shape
circle.drawCircle(); // Method of Circle class
Rectangle rectangle = new Rectangle();
rectangle.draw(); // Inherited from Shape
rectangle.drawRectangle(); // Method of Rectangle class
Triangle triangle = new Triangle();
triangle.draw(); // Inherited from Shape
triangle.drawTriangle(); // Method of Triangle class
}
}
In this example, we have a class hierarchy with Shape
as the parent class, and three child classes (Circle
, Rectangle
, and Triangle
) that inherit from the Shape
class. Each child class inherits the draw()
method from the parent class and also has its own specific methods (drawCircle()
, drawRectangle()
, and drawTriangle()
).
Hierarchical inheritance allows multiple classes to share common behavior from a single parent class while still having the flexibility to extend and specialize their own functionality.
Multiple Inheritance in Java(Using Interfaces)
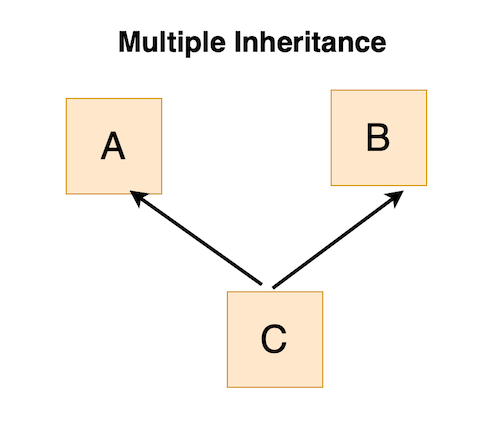
In Java, multiple inheritance of classes (i.e., inheriting from more than one class) is not allowed to avoid the complexities and ambiguities that can arise from such scenarios.However, Java allows a class to implement several interfaces using interface inheritance. Interfaces specify a contract that classes must follow, but they do not provide any implementation. A class can inherit behavior from many sources by implementing numerous interfaces. This is a typical method for achieving “multiple inheritance” in Java.
Here’s an example demonstrating multiple inheritance using interfaces in Java:
// First interface
interface Swimmer {
void swim();
}
// Second interface
interface Jumper {
void jump();
}
// Class implementing multiple interfaces
class Athlete implements Swimmer, Jumper {
@Override
public void swim() {
System.out.println("The athlete is swimming.");
}
@Override
public void jump() {
System.out.println("The athlete is jumping.");
}
}
public class Main {
public static void main(String[] args) {
Athlete athlete = new Athlete();
athlete.swim(); // Method from Swimmer interface
athlete.jump(); // Method from Jumper interface
}
}
In this example, we have two interfaces, Swimmer
and Jumper
, each with a single abstract method. The Athlete
class implements both interfaces and provides implementations for both the swim()
and jump()
methods.
Read More: Multiple Inheritance in Java: Exploring Alternatives and Solutions
Hybrid Inheritance in Java

In Java, “Hybrid Inheritance” typically refers to a combination of inheritance mechanisms, specifically the blending of single inheritance (class inheriting from one superclass) with interface-based multiple inheritance (class implementing multiple interfaces). This allows classes to inherit attributes and behaviors from both classes and interfaces, enabling a broader range of code reuse and flexibility.
Here’s a Java example that demonstrates Hybrid Inheritance in Java.
// Interface for swimming behavior
interface Swimmer {
void swim();
}
// Base class for animals
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
// Class representing a land animal
class LandAnimal extends Animal {
void walk() {
System.out.println("Land animal is walking");
}
}
// Class representing a bird that can fly
class Bird extends Animal implements Swimmer {
public void fly() {
System.out.println("Bird is flying");
}
public void swim() {
System.out.println("Bird is swimming");
}
}
// Class representing a fish that can swim
class Fish implements Swimmer {
public void swim() {
System.out.println("Fish is swimming");
}
}
// Class representing a duck that can walk, swim, and fly
class Duck extends LandAnimal implements Swimmer {
public void swim() {
System.out.println("Duck is swimming");
}
}
In this example:
Animal
is the base class.LandAnimal
inherits fromAnimal
and adds a method for walking behavior.Bird
inherits fromAnimal
and implements theSwimmer
interface.Fish
implements theSwimmer
interface.Duck
inherits fromLandAnimal
and implements theSwimmer
interface.
This example showcases a combination of single inheritance (LandAnimal
extending Animal
) and interface-based multiple inheritance (Bird
, Fish
, and Duck
implementing the Swimmer
interface).
Advantages of Inheritance in Java
Advantages of Inheritance in Java include:
- Code Reusability and Minimizing Duplicate Code: Inheritance allows sharing common code among subclasses, reducing redundancy and promoting efficient code management.
- Flexibility and Code Modularity: By dividing code into parent and child classes, inheritance enhances code modularity and adaptability to changes.
- Code Maintainability: Inheritance simplifies code maintenance, enabling developers to make changes in one place, affecting all derived classes.
- Polymorphism and Overriding: Inheritance supports polymorphism through method overriding, allowing objects of different classes to be treated uniformly, enhancing code adaptability.
- Encapsulation and Data Hiding: Inheritance enables control over data visibility, restricting modifications in derived classes.
- Code Design and Standardization: Inheritance aligns with Object-Oriented Programming (OOP) principles, contributing to standardized and collaboratively manageable code.
- Promoting Code Flexibility: Inheritance encourages the creation of new classes with added features while inheriting characteristics, boosting code flexibility and reuse.
- Enhancing Collaboration and Encapsulation: Effective inheritance leads to well-designed, encapsulated code, suitable for collaboration among multiple developers.
Disadvantages of Inheritance in Java
Disadvantages of Inheritance in Java include:
- Tight Coupling and Fragile Base Class Problem: Modifying the parent class affects child classes, necessitating careful initial design to prevent unintended consequences.
- Inflexibility and Execution Overhead: Derived classes must conform to the base class structure, leading to reduced flexibility and increased execution time due to inheritance processing.
- Overuse and Complex Hierarchies: Excessive inheritance can lead to convoluted code, intricate class hierarchies, and challenges in managing the codebase.
- Limited Independence and Refactoring Difficulties: Inherited classes are interdependent, limiting their independent use, and removing a superclass can require significant refactoring.
- Performance Impact and Code Complexity: Inheritance can introduce execution overhead, potentially slowing down code, and excessive use can lead to codebase complexity, hindering collaboration and testing.
- Promoting Rigid Designs: Inheritance can result in inflexible designs, making adaptation to changing requirements more difficult. It might also constrain code flexibility and complicate testing.
Conclusion: Inheritance in Java
This article provides a comprehensive understanding of Inheritance in Java and explores various aspects of it. Inheritance is a fundamental concept in Object-Oriented Programming (OOP), allowing classes to inherit properties and behaviors from other classes. This establishes an “is-a” relationship between the parent (superclass) and child (subclass) classes. Key points covered include code reusability, extending functionality, method overriding, access control, and constructors.
The article also delves into different types of inheritance in Java:
- Single Inheritance: A subclass inherits traits from only one superclass, ensuring a clear and straightforward class hierarchy.
- Multi-level Inheritance: This scenario involves chains of classes inheriting from one another, providing a structured way to pass down behavior and attributes.
- Hierarchical Inheritance: One parent class is inherited by multiple child classes, enabling shared behavior while allowing subclasses to specialize further.
- Multiple Inheritance (through Interfaces): Java avoids multiple inheritance of classes to prevent complexity but permits multiple inheritance through interfaces. Interfaces define contracts for behavior, fostering code adaptability and reuse.
- Hybrid Inheritance: Though not a formal term in Java, it can be thought of as combining single inheritance with interface-based multiple inheritance. This approach maximizes code flexibility and reuse.
The advantages of inheritance include promoting code reusability, enhancing code modularity, improving maintainability, supporting polymorphism, and enabling better collaboration among developers. However, there are also disadvantages to consider, such as tight coupling, inflexibility, complexity, and potential performance issues.
Understanding inheritance is crucial for building well-structured and adaptable Java programs. Through careful design and thoughtful implementation, developers can harness the benefits of inheritance while mitigating its potential drawbacks.
Related Article: OOPs Concepts in Java