In this article, we will explore Map Interface in Java. So, let’s get started.
What is the Use of Map Interface in Java ?
The Map
interface is a key component of the Java Collections Framework. It represents a collection of key-value pairs, where each key is unique and maps to a corresponding value. The Map
interface provides methods for adding, retrieving, and manipulating key-value pairs, making it a versatile data structure for various applications.
Hierarchy of Map Interface in Java
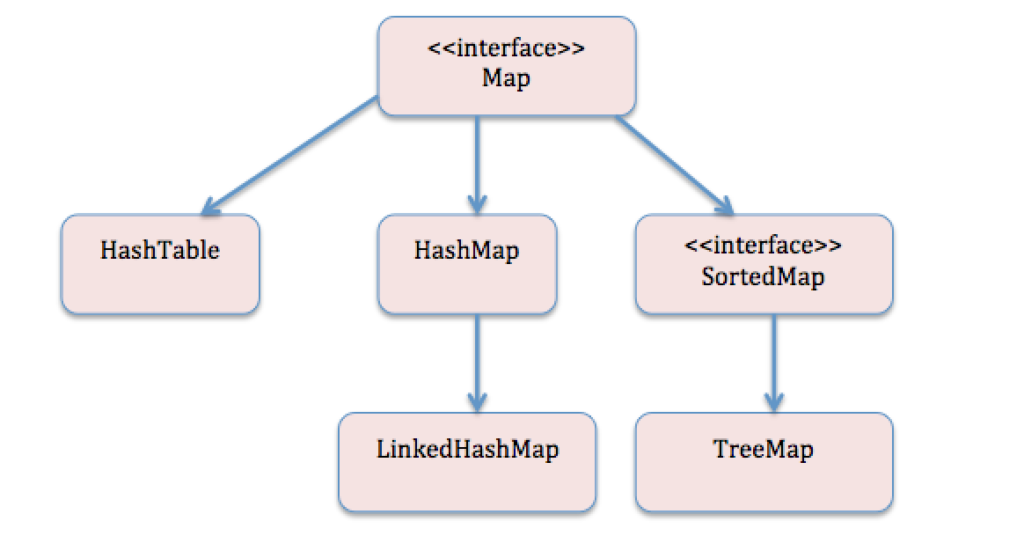
Interface | Description | Concrete Classes |
---|---|---|
Map | Defines operations for maintaining key-value mappings. | HashMap, Hashtable, LinkedHashSet |
SortedMap | Extends the Map interface. Mappings are sorted by key order. | TreeMap |
Concrete Class | Interface | Is Duplicate Key Allowed? | Ordered/Sorted | Data Structure |
---|---|---|---|---|
HashMap | Map | No | No Order | Hash table |
LinkedHashMap | Map | No | Key insertion order | Hash table and doubly-linked list |
Hashtable | Map | No | No Order | Hash table |
TreeMap | SortedMap | No | Sorted in Key order | Balanced Tree |
Important Methods of Map Interface in Java
Some commonly used methods of the Java Map
interface are:
Method | Description |
---|---|
V put(K key, V value) | Inserts or updates the key-value pair in the map. |
V get(Object key) | Retrieves the value associated with the given key. |
boolean containsKey(Object key) | Checks if the map contains the specified key. |
boolean containsValue(Object value) | Checks if the map contains the specified value. |
V remove(Object key) | Removes the key-value pair associated with the given key. |
int size() | Returns the number of key-value pairs in the map. |
boolean isEmpty() | Checks if the map is empty. |
Set<K> keySet() | Returns a set containing all the keys in the map. |
Collection<V> values() | Returns a collection containing all the values in the map. |
Set<Map.Entry<K, V>> entrySet() | Returns a set of key-value pairs (entries) in the map. |
void clear() | Removes all the key-value pairs from the map. |
V getOrDefault(Object key, V defaultValue) | Retrieves the value associated with the given key, or a default value if the key is not present. |
default V putIfAbsent(K key, V value) | Inserts the key-value pair if the key is not already present. Returns the previous value or null . |
default V replace(K key, V value) | Replaces the entry for the specified key with the specified value. Returns the previous value or null . |
default boolean remove(Object key, Object value) | Removes the entry for the specified key only if it is currently mapped to the specified value. |
default boolean replace(K key, V oldValue, V newValue) | Replaces the entry for the specified key only if it is currently mapped to the specified value. |
default void forEach(BiConsumer<? super K, ? super V> action) | Performs the given action for each key-value pair in the map. |
Sample Code: Map Interface in Java
import java.util.*;
public class MapExample {
public static void main(String[] args) {
// Create a Map using HashMap
Map<String, Integer> map = new HashMap<>();
// Adding key-value pairs
map.put("Apple", 5);
map.put("Banana", 3);
map.put("Orange", 8);
// Accessing values using keys
int appleCount = map.get("Apple");
System.out.println("Apple count: " + appleCount);
// Checking if a key exists
boolean containsBanana = map.containsKey("Banana");
System.out.println("Contains Banana? " + containsBanana);
// Iterating through key-value pairs
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
// Removing a key-value pair
map.remove("Orange");
// Size of the map
int size = map.size();
System.out.println("Map size: " + size);
// Clearing the map
map.clear();
// Checking if the map is empty
boolean isEmpty = map.isEmpty();
System.out.println("Is map empty? " + isEmpty);
}
}
Output
Apple count: 5
Contains Banana? true
Key: Apple, Value: 5
Key: Banana, Value: 3
Key: Orange, Value: 8
Map size: 2
Is map empty? true
Conclusion: Map Interface in Java
In this article, we delved into the Map Interface in Java, a vital component of the Java Collections Framework. The Map interface serves as a key-value store, allowing unique keys to map to corresponding values. It offers a rich set of methods for managing, retrieving, and manipulating key-value pairs, making it a versatile tool for various programming scenarios.
We explored the hierarchy of the Map interface, highlighting its concrete implementations such as HashMap, Hashtable, LinkedHashMap, and TreeMap.
The article emphasized commonly used methods of the Map interface, from inserting and retrieving key-value pairs to checking for key and value existence, iterating through entries, and managing the map’s size and emptiness. We also provided a concise Java code example showcasing these methods in action, illustrating how to create, populate, and interact with a map.