In this article, we will go through Owasp Top 10 Vulnerabilities and how to mitigate these in Spring Boot Applications. Let’s get started.
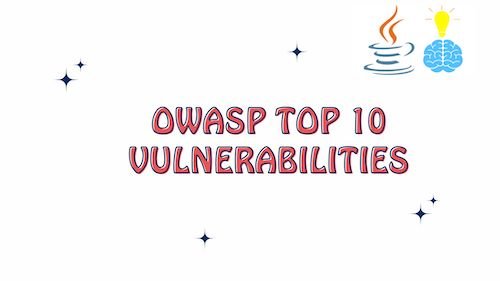
What is Owasp ?
OWASP stands for the Open Web Application Security Project. It is a nonprofit organization that focuses on improving the security of software. OWASP provides resources, tools, and guidelines to help organizations develop and maintain secure applications and web services.
What is OWASP Top 10?
OWASP Top 10 is a list of the ten most critical web application security risks, periodically updated by the Open Web Application Security Project to guide organizations in addressing prevalent security vulnerabilities.
- Injection: Vulnerabilities allowing attackers to inject malicious code into applications, commonly seen in SQL injection, NoSQL injection, and other similar attacks.
- Broken Authentication: Flaws in authentication processes leading to unauthorized access, requiring mitigation through strong password policies, secure storage, and proper session management.
- Sensitive Data Exposure: Risks associated with inadequately protecting sensitive information, mitigated by encryption, secure session management, and logging practices.
- XML External Entities (XXE): Exploitation of weakly configured XML parsers, mitigated by using secure parsers, disabling DTD processing, and validating/sanitizing XML input.
- Broken Access Control: Failures to enforce restrictions on user access, mitigated by implementing proper authorization, avoiding direct object references, and conducting regular security audits.
- Security Misconfigurations: Risks arising from incorrect or insecure configurations, mitigated by avoiding default credentials, securing unnecessary services, and conducting regular security audits.
- Cross-Site Scripting (XSS): Vulnerabilities allowing attackers to inject malicious scripts into web pages, mitigated by validating user input, using secure frameworks, and implementing Content Security Policy (CSP).
- Insecure Deserialization: Risks associated with deserializing untrusted data, mitigated by avoiding default mechanisms, validating input data, and keeping libraries and dependencies updated.
- Using Components with Known Vulnerabilities: Security risks stemming from using outdated or vulnerable components, mitigated by regularly updating dependencies, tracking vulnerabilities, and implementing automated scanning.
- Insufficient Logging & Monitoring: Risks due to inadequate monitoring and logging, mitigated by implementing centralized logging, incident response planning, and using Security Information and Event Management (SIEM) solutions.
Let’s discuss the Top 10 security risks and it’s mitigation in Spring Boot Applications.
Injection
Injection attacks refer to a class of security vulnerabilities where an attacker is able to inject malicious input into an application, leading to the execution of unintended commands. One of the most common types of injection attacks is SQL injection, where an attacker injects SQL code into input fields to manipulate a database.
In the context of web applications, injection attacks can occur in various forms, including SQL injection, NoSQL injection, OS command injection, and LDAP injection, among others. Here’s a brief overview of SQL injection, one of the most well-known types of injection attacks:
SQL Injection:
Vulnerability: In SQL injection, an attacker inserts or manipulates SQL queries within input fields or parameters that are part of a database query. If the application does not properly validate or sanitize user input, the injected SQL code can be executed by the database, leading to unauthorized access, data manipulation, or data retrieval.
Example: Consider a login form where the application queries the database to check the credentials:
SELECT * FROM users WHERE username = 'input_username' AND password = 'input_password';
If an attacker enters the following in the username field: ' OR '1'='1'; --
, the SQL query becomes:
SELECT * FROM users WHERE username = '' OR '1'='1'; --' AND password = 'input_password';
This modification can result in the login being bypassed because the condition '1'='1'
is always true.
Mitigation Strategies (in the context of Spring Boot):
Use Prepared Statements:
Utilize prepared statements or parameterized queries instead of dynamically constructing SQL queries with user input. Spring Data JPA and Hibernate, commonly used in Spring Boot applications, automatically handle parameterization.
// Spring Data JPA example
@Query("SELECT u FROM User u WHERE u.username = :username AND u.password = :password")
User findByUsernameAndPassword(@Param("username") String username, @Param("password") String password);
Input Validation and Sanitization:
Implement strict input validation and sanitization. Ensure that user input adheres to expected formats and reject any input that could be interpreted as SQL code.
Use Object-Relational Mapping (ORM):
If using a relational database, consider using Object-Relational Mapping (ORM) frameworks like Hibernate. These frameworks abstract away much of the raw SQL, reducing the risk of injection.
Least Privilege Principle:
Ensure that database accounts used by the application have the minimum necessary permissions. Avoid using accounts with unrestricted access.
Broken Authentication
“Boken Authentication” refers to vulnerabilities arising from incorrect implementation, configuration, or management of authentication mechanisms in a web application. Flaws in authentication processes can lead to unauthorized access, allowing attackers to compromise user accounts, gain unauthorized privileges, or perform other malicious actions.
Mitigation Strategies (in the context of Spring Boot):
Here are some common issues associated with broken authentication and recommended mitigation strategies, particularly in the context of Spring Boot applications:
Common Issues:
Weak Password Policies:
Mitigation: Enforce strong password policies, including minimum length, complexity, and expiration. Use password hashing algorithms (e.g., bcrypt) to securely store passwords.
Insecure Password Storage:
Mitigation: Hash and salt passwords before storing them. Spring Security provides robust password encoding mechanisms. Use BCryptPasswordEncoder
or other secure password encoders.
Inadequate Session Management:
Mitigation: Use secure and random session identifiers. Implement session timeout and logout functionality. Ensure that session tokens are not exposed in URLs, and use secure, HTTP-only cookies.
Failure to Invalidate Sessions:
Mitigation: Ensure that sessions are properly invalidated on logout or when a user logs in from a different location. Use the SessionRegistry
in Spring Security to manage and invalidate sessions.
Brute Force Attacks:
Mitigation: Implement account lockout mechanisms after a certain number of failed login attempts. Use CAPTCHAs to prevent automated brute-force attacks.
Insecure Password Recovery:
Mitigation: Implement secure password recovery mechanisms, such as sending reset tokens via email with proper expiration. Ensure that the password reset process is secure and resistant to attacks.
Additional Recommendations:
- Multi-Factor Authentication (MFA):
- Implement MFA to add an extra layer of security, requiring users to provide multiple forms of identification.
- Regular Security Audits:
- Conduct regular security audits, including code reviews and penetration testing, to identify and address potential vulnerabilities in the authentication process.
- Keep Dependencies Updated:
- Regularly update and patch dependencies, including Spring Security, to benefit from security fixes and improvements.
Sensitive Data Exposure
“Sensitive Data Exposure” is a security risk that occurs when an application fails to adequately protect sensitive information, such as financial data, personal details, or authentication credentials. If attackers gain access to this data, they may exploit it for identity theft, financial fraud, or other malicious activities. In the context of web applications, sensitive data exposure commonly involves the inadequate protection of data both in transit and at rest.
Mitigation Strategies (in the context of Spring Boot)
Here are some common issues related to sensitive data exposure and recommended mitigation strategies, particularly in the context of Spring Boot applications:
Common Issues:
Lack of Encryption:
- Mitigation: Use HTTPS to encrypt data in transit. Spring Boot applications can easily enable HTTPS by configuring a secure connection through properties or a configuration class.
Weak Encryption Algorithms:
Mitigation: Ensure that strong encryption algorithms and protocols are used. Stay informed about the latest security recommendations and vulnerabilities related to encryption standards.
Insecure Storage of Passwords:
- Mitigation: Hash and salt passwords securely before storing them. Use strong, adaptive hashing algorithms like bcrypt. Spring Security provides convenient ways to handle password hashing.
Inadequate Session Management:
- Mitigation: Implement secure session management practices. Ensure session tokens are securely generated, stored, and transmitted. Use secure, HTTP-only cookies.
Logging of Sensitive Data:
Mitigation: Avoid logging sensitive information such as passwords, credit card numbers, or other private data. Implement proper logging practices and use tools like Logback or Log4j to configure log levels and filters.
Additional Recommendations:
- Data Masking:
- Implement data masking techniques to conceal sensitive information in logs or other outputs.
- Regular Security Audits:
- Conduct regular security audits, including code reviews and penetration testing, to identify and address potential vulnerabilities related to sensitive data exposure.
- Keep Dependencies Updated:
- Regularly update and patch dependencies, including Spring Boot, to benefit from security fixes and improvements.
- Security Headers:
- Use security headers, such as Content Security Policy (CSP) and Strict-Transport-Security (HSTS), to enhance the security of your web application.
XML External Entities (XXE)
XML External Entity (XXE) attacks are a type of security vulnerability that can occur when an application parses XML input. XXE attacks involve the exploitation of weakly configured XML parsers to disclose internal files, execute remote code, or perform other malicious activities.
Mitigation Strategies (in the context of Spring Boot)
In the context of Spring Boot applications or any Java-based application dealing with XML processing, it’s crucial to be aware of XXE vulnerabilities and take appropriate measures to mitigate them.
Common Issues:
Insecure XML Parsers:
Mitigation: Use secure XML parsers that disable external entity processing. For Spring Boot applications, ensure that the XML processing libraries and configurations are secure.
Document Type Definition (DTD) Processing:
Mitigation: Disable DTD processing in XML parsers. In Spring Boot applications using libraries like Jackson or JAXB, ensure that DTD processing is turned off.
External Entity Resolution:
Mitigation: Configure XML parsers to reject external entities. In Spring Boot, use secure XML configurations to prevent XXE attacks.
Additional Recommendations:
- Input Validation:
- Validate and sanitize XML input to ensure it adheres to the expected structure and content. Reject any unexpected or malicious input.
- Use Whitelists:
- If XML processing is necessary, use whitelists to specify which elements and attributes are allowed. This helps prevent unexpected entities or content from being processed.
- Security Audits:
- Conduct security audits, including code reviews and penetration testing, to identify and address potential vulnerabilities related to XXE attacks.
- Keep Dependencies Updated:
- Regularly update and patch dependencies, including XML processing libraries, to benefit from security fixes and improvements.
Broken Access Control
“Broken Access Control” is a security risk that occurs when an application does not properly enforce restrictions on what authenticated users are allowed to do. This can lead to unauthorized access to sensitive data, privilege escalation, and other security issues. In the context of web applications, broken access control vulnerabilities can allow users to perform actions or access resources that they should not be allowed to.
Mitigation Strategies (in the context of Spring Boot)
Here are some common issues related to broken access control and recommended mitigation strategies, particularly in the context of Spring Boot applications:
Common Issues:
- Insufficient Authorization Checks:
- Mitigation: Implement proper authorization checks at both the server and client sides. In Spring Boot, use Spring Security for handling authentication and authorization.
- Direct Object References:
- Mitigation: Avoid exposing internal implementation details, such as database IDs, directly to clients. Use indirect references or object-level security to control access.
- Insecure Direct Object References (IDOR):
- Mitigation: Validate user input and ensure that users can only access resources that they are authorized to view or modify. Implement proper input validation and authorization checks.
- Overly Permissive Defaults:
- Mitigation: Ensure that default access permissions are restrictive and only grant necessary privileges. Regularly review and update access controls.
Additional Recommendations:
Role-Based Access Control (RBAC):
Implement RBAC principles to assign specific roles to users and grant permissions based on those roles. Spring Security provides robust support for RBAC.
Attribute-Based Access Control (ABAC):
Use ABAC to define access control policies based on attributes associated with users and resources.
Regular Security Audits:
Conduct regular security audits, including code reviews and penetration testing, to identify and address potential vulnerabilities related to broken access control.
Keep Dependencies Updated:
Regularly update and patch dependencies, including Spring Boot and Spring Security, to benefit from security fixes and improvements.
Use Security Headers:
Leverage security headers, such as Content Security Policy (CSP) and X-Frame-Options, to enhance the security of your web application.
Security Misconfigurations
“Security Misconfigurations” refer to security vulnerabilities that arise from incorrect or insecure configurations of an application, server, database, or any other component in a system. These misconfigurations can lead to unauthorized access, data exposure, or other security breaches. In the context of Spring Boot applications, ensuring secure configurations is crucial to prevent common security misconfigurations.
Mitigation Strategies (in the context of Spring Boot)
Here are some common security misconfigurations and recommended mitigation strategies, particularly in the context of Spring Boot applications:
Common Issues:
- Default Credentials:
- Mitigation: Change default usernames and passwords for applications, databases, and other components. Always use strong and unique credentials.
- Unnecessary Services and Features:
- Mitigation: Disable or remove unnecessary services and features. Only enable services that are required for the application to function.
- Incomplete or Weak Session Management:
- Mitigation: Configure secure session management settings, such as using secure, HTTP-only cookies, implementing session timeout, and enabling secure transmission of session tokens.
- Exposure of Sensitive Information:
- Mitigation: Avoid exposing sensitive information, such as stack traces and detailed error messages, to users. Implement proper error handling and logging practices.
- Insecure Default Configurations:
- Mitigation: Review and modify default configurations to meet security best practices. Spring Boot provides many secure defaults, but developers should review and adjust them based on application requirements.
- Unrestricted URL Access:
- Mitigation: Implement proper access controls and authorization checks on URLs and endpoints. Use Spring Security annotations or configuration to secure access to resources.
Additional Recommendations:
- Regular Security Audits:
- Conduct regular security audits, including code reviews and penetration testing, to identify and address potential security misconfigurations.
- Configuration Management:
- Use configuration management tools to ensure consistent and secure configurations across different environments (development, testing, production).
- Keep Dependencies Updated:
- Regularly update and patch dependencies, including Spring Boot and third-party libraries, to benefit from security fixes and improvements.
- Least Privilege Principle:
- Apply the principle of least privilege. Grant users and components only the permissions they absolutely need to perform their functions.
Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a type of security vulnerability that occurs when an application includes untrusted data in a web page that is then rendered by a user’s browser. This can enable attackers to inject malicious scripts into web pages that are viewed by other users. XSS attacks can have various impacts, including stealing sensitive information, session hijacking, or defacing websites.
Mitigation Strategies (in the context of Spring Boot)
In the context of Spring Boot applications, preventing XSS vulnerabilities is crucial. Here are some common issues related to XSS and recommended mitigation strategies:
Common Issues:
- Unvalidated User Input:
- Mitigation: Validate and sanitize all user inputs before rendering them in the HTML output. Avoid rendering user input directly within HTML markup.
- Improper Use of Framework Tags:
- Mitigation: Be cautious when using frameworks or template engines like Thymeleaf. Ensure that you are using them correctly to prevent unintentional script execution.
- Lack of Content Security Policy (CSP):
- Mitigation: Implement Content Security Policy headers to restrict the sources from which resources can be loaded. This helps mitigate the impact of XSS attacks.
- Inadequate Output Encoding:
- Mitigation: Use proper output encoding functions provided by your template engine or web framework to ensure that user input is treated as data, not HTML or JavaScript code.
Additional Recommendations:
- Avoid Inline JavaScript:
- Minimize the use of inline JavaScript in your application. Externalize JavaScript code and use nonces or hashes to enable only trusted scripts to execute.
- Use HTTP Only Cookies:
- Mark cookies as HTTP-only to prevent them from being accessed through client-side scripts, reducing the risk of session hijacking through XSS.
- Regular Security Audits:
- Conduct regular security audits, including code reviews and automated scanning, to identify and address potential XSS vulnerabilities.
- Keep Dependencies Updated:
- Regularly update and patch dependencies, including Spring Boot and third-party libraries, to benefit from security fixes and improvements.
Insecure Deserialization
Insecure deserialization is a security vulnerability that occurs when an application deserializes untrusted or manipulated data without proper validation. Deserialization is the process of converting serialized data (often in the form of binary data or JSON) into a usable object in the application. If this process is not properly secured, it can lead to various security risks, including remote code execution, data tampering, and denial-of-service attacks.
Mitigation Strategies (in the context of Spring Boot)
In the context of Spring Boot applications, which may involve Java object serialization or JSON deserialization, it’s important to be aware of insecure deserialization vulnerabilities. Here are some common issues and recommended mitigation strategies:
Common Issues:
- Default Deserialization:
- Mitigation: Avoid using default deserialization mechanisms, especially when deserializing data from untrusted sources. Implement custom deserialization logic with proper validation.
- Unexpected Deserialization of Untrusted Data:
- Mitigation: Validate and sanitize input data before deserialization. Ensure that only trusted and expected classes can be deserialized.
- Insecure Libraries and Components:
- Mitigation: Regularly update and patch serialization libraries and components, including Jackson or any other libraries used for deserialization.
- Denial-of-Service (DoS) Attacks:
- Mitigation: Implement rate limiting and size restrictions on deserialized objects to prevent DoS attacks. Set appropriate timeouts for deserialization operations.
Additional Recommendations:
- Use Object-Relational Mapping (ORM):
- When working with relational databases, use ORM frameworks like Hibernate to handle the conversion between database records and Java objects. ORM frameworks often have built-in security measures.
- Content Security Policy:
- Implement Content Security Policy (CSP) headers to restrict the sources from which resources can be loaded. This helps mitigate the impact of attacks that involve loading malicious content.
- Regular Security Audits:
- Conduct regular security audits, including code reviews and automated scanning, to identify and address potential insecure deserialization vulnerabilities.
- Keep Dependencies Updated:
- Regularly update and patch dependencies, including Spring Boot and third-party libraries, to benefit from security fixes and improvements.
Using Components with Known Vulnerabilities
Using components with known vulnerabilities is a significant security risk that can expose your application to exploits and attacks. It occurs when an application relies on libraries, frameworks, or other software components that have known security vulnerabilities. Attackers often target these vulnerabilities to compromise the security of the application.
Mitigation Strategies (in the context of Spring Boot)
Here are some common issues related to using components with known vulnerabilities and recommended mitigation strategies in the context of Spring Boot applications:
Common Issues:
- Outdated Libraries and Dependencies:
- Mitigation: Regularly check for updates and security patches for all libraries and dependencies used in your Spring Boot application. Set up a process to review and apply updates promptly.
- Lack of Version Management:
- Mitigation: Use tools like Dependency-Check to identify known vulnerabilities in your project dependencies. Set up automated processes to regularly check for updates and security advisories.
- No Vulnerability Tracking:
- Mitigation: Maintain an inventory of all the third-party components used in your application, including their versions. Subscribe to security mailing lists or use vulnerability databases to stay informed about known issues.
- Failure to Monitor Components:
- Mitigation: Implement a continuous monitoring system to detect and alert on security vulnerabilities in your application’s dependencies. Consider using automated tools that integrate with your build pipeline.
Additional Recommendations:
- Automated Dependency Scanning:
- Integrate automated dependency scanning tools into your CI/CD pipeline to identify and report vulnerabilities in your dependencies during the build process.
- Dependency Locking:
- Use dependency locking mechanisms to ensure that your application consistently uses the same versions of dependencies across different environments, reducing the risk of unintentional updates.
- Security Training and Awareness:
- Train developers and team members to be aware of the importance of keeping dependencies up to date and understanding the potential security implications of using components with known vulnerabilities.
- Risk Assessment:
- Perform risk assessments on third-party components before integrating them into your application. Consider factors such as the project’s activity, the responsiveness of maintainers to security issues, and the overall reputation of the component.
- Vendor and Component Trustworthiness:
- Prioritize dependencies from trusted vendors and well-maintained open-source projects. Assess the reputation and community support of the components you rely on.
Insufficient Logging & Monitoring
Insufficient logging and monitoring is a security risk that can lead to delayed detection and response to security incidents. Inadequate logging and monitoring make it challenging to identify and respond to suspicious activities or security breaches in a timely manner.
Mitigation Strategies (in the context of Spring Boot)
Effective logging and monitoring are crucial components of a comprehensive security strategy for Spring Boot applications.Here are some common issues related to insufficient logging and monitoring and recommended mitigation strategies:
Common Issues:
- Lack of Logging for Security Events:
- Mitigation: Implement logging for security-relevant events, such as authentication successes and failures, authorization attempts, and critical operations. Log sufficient details for effective analysis.
- Missing or Inadequate Error Handling:
- Mitigation: Ensure that errors and exceptions are logged with appropriate details. Avoid exposing sensitive information in error messages that could aid attackers.
- Failure to Monitor Logs:
- Mitigation: Set up a centralized logging system or use log aggregation tools to monitor logs effectively. Regularly review logs for signs of malicious activities.
- Lack of Incident Response Planning:
- Mitigation: Develop an incident response plan that includes logging and monitoring procedures. Define roles and responsibilities for incident response team members.
Additional Recommendations:
- Security Information and Event Management (SIEM):
- Implement SIEM solutions or log management platforms to centralize and analyze logs from various components of your Spring Boot application.
- Use Security Headers:
- Use security headers, such as Content Security Policy (CSP) and Strict-Transport-Security (HSTS), to enhance the security of your web application.
- Regular Log Reviews:
- Regularly review logs for signs of security incidents. Implement automated alerting based on predefined patterns or thresholds.
- Log Retention and Backups:
- Establish log retention policies to retain logs for an appropriate period. Ensure that logs are securely stored and backed up to prevent data loss.
Conclusion: Owasp Top 10
In this article, we explored the OWASP Top 10 vulnerabilities and discussed mitigation strategies for Spring Boot applications. Key takeaways include securing against injection by using prepared statements, enforcing strong authentication, preventing data exposure, and safeguarding against XML External Entities (XXE) attacks. We also covered securing access control, addressing security misconfigurations, mitigating Cross-Site Scripting (XSS), and handling insecure deserialization. Additionally, we discussed the importance of monitoring, logging, and keeping components updated to reduce the risk of vulnerabilities. Applying these measures enhances the overall security posture of Spring Boot applications.
Must-Read Spring Boot Related Articles: