This article will delve into the concept of Copy Constructor in Java, explaining how to implement it, providing a sample example, highlighting their advantages, and comparing them to the Clone method.
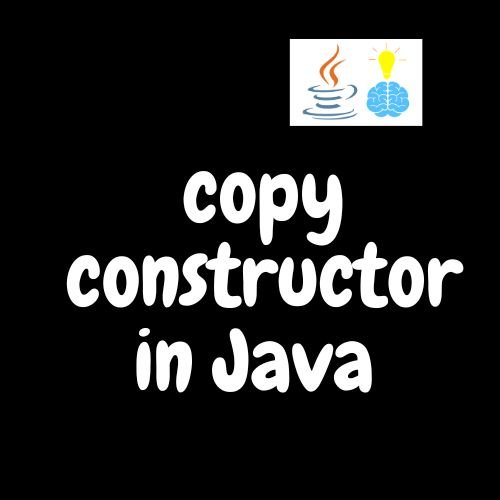
What is Copy Constructor in Java?
A Copy Constructor in Java is a special constructor that creates a new object by copying the values from an existing object of the same class. It provides a convenient way to create a deep copy of an object, ensuring that any changes made to the copied object do not affect the original object.
Steps to Implement Copy Constructor in Java
To implement a Copy Constructor in Java, follow these steps:
- Declare a new constructor with the same class name as the class you want to copy.
- Pass an object of the same class as a parameter to the Copy Constructor.
- Inside the Copy Constructor, initialize the instance variables of the current object using the values from the parameter object.
Java Code Snippet
Let’s consider an example to illustrate the implementation of a Copy Constructor in Java:
public class Student {
private String name;
private int age;
// Normal Constructor
public Student(String name, int age) {
this.name = name;
this.age = age;
}
// Copy Constructor
public Student(Student student) {
this.name = student.name;
this.age = student.age;
}
// Getter for name
public String getName() {
return name;
}
// Getter for age
public int getAge() {
return age;
}
// Other constructors and methods...
// Main method
public static void main(String[] args) {
Student student1 = new Student("John", 20);
Student student2 = new Student(student1); // Copying student1 to student2
System.out.println("Student 1: " + student1.getName() + ", " + student1.getAge());
System.out.println("Student 2: " + student2.getName() + ", " + student2.getAge());
}
}
Output
Student 1: John, 20
Student 2: John, 20
In the above example, we have added a normal constructor public Student(String name, int age)
which takes the name and age as parameters and initializes the instance variables. The Copy Constructor public Student(Student student)
takes an object of the Student
class as a parameter and initializes a new Student
object with the same values.
Advantages of using Copy Constructor in Java
There are several advantages to using Copy Constructor in Java:
- Simplified object creation: Copy Constructors provide a straightforward way to create new objects by copying the values of existing objects.
- Deep copy of objects: Copy Constructors ensure that a deep copy of an object is created, preventing any unwanted side effects or dependencies between the original and copied objects.
- Enhanced immutability: Copy Constructors can be used to create immutable objects by copying the values and preventing direct modifications to the original object.
- Code reusability: Copy Constructors allow for the reuse of existing code, as they provide a standard way to create new objects based on existing ones.
Copy Constructor vs Clone Method
While both the Copy Constructor and the Clone method can be used to create copies of objects, there are some differences between them:
Aspect | Copy Constructor | Clone Method |
---|---|---|
Accessibility | Accessible only within the class | Public, can be accessed from outside the class |
Object Creation | Creates a new object by explicitly copying values from an existing object | Creates a new object by creating a bit-wise copy of the existing object |
Interface Requirement | No interface implementation required | Requires implementation of the Cloneable interface |
Flexibility | Provides greater control over object creation | Provides a standard way to create object copies |
Deep Copy | Can be implemented to create deep copies | Default implementation creates shallow copies |
Code Reusability | Allows for reuse of existing code | Requires additional implementation for each class |
Overriding | Not subject to inheritance considerations | Can be overridden to provide custom cloning logic |
Conclusion
Copy Constructor in Java provide a convenient way to create new objects by copying the values from existing objects. They offer advantages such as simplified object creation, deep copying, enhanced immutability, and code reusability. While both Copy Constructors and the Clone method serve similar purposes, Copy Constructors are more accessible and offer greater control over object creation. By understanding and utilizing Copy Constructors effectively, Java developers can enhance the flexibility and efficiency of their code.