In this article, we will learn about various concepts associated with "Stored Procedure in SQL"
. Let’s get started.
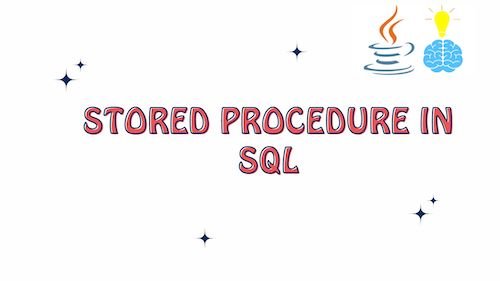
What is a Stored Procedure in SQL ?
A stored procedure in SQL is a precompiled and reusable set of one or more SQL statements that are stored in the database. It is a database object that allows you to encapsulate a series of SQL statements into a single, named unit. Stored procedures are typically used to perform specific tasks or operations within a database.
Characteristics of Stored Procedure in SQL
- Precompiled: Stored procedures are precompiled, which means the database system compiles them into an execution plan once when they are created. This compilation step can lead to better performance when the procedure is executed repeatedly.
- Reusable: Stored procedures can be executed multiple times with different input values, making them reusable. This reduces the need to write the same SQL code repeatedly in applications.
- Named and Parameterized: Stored procedures have names and can accept parameters, allowing for flexibility in executing the same procedure with different inputs.
- Encapsulation: Stored procedures can encapsulate complex SQL logic and business rules, making it easier to manage and maintain database code.
Syntax of Stored Procedure in SQL
The syntax for creating a stored procedure in SQL varies slightly between different database management systems (DBMS), but the fundamental structure is generally similar. Here’s a common syntax outline for creating a stored procedure in SQL:
CREATE [OR REPLACE] PROCEDURE procedure_name
[parameter1 datatype, parameter2 datatype, ...]
AS
BEGIN
-- SQL statements and logic for the procedure
-- You can use parameters within your SQL statements
END;
Here’s an example of a simple stored procedure that accepts two parameters and performs an INSERT operation. Please note the below is using MySQL(version 8.1.0) syntax.
DELIMITER //
CREATE PROCEDURE InsertEmployee(
IN EmployeeID INT,
IN EmployeeName NVARCHAR(50)
)
BEGIN
INSERT INTO Employees (EmployeeID, EmployeeName, HireDate)
VALUES (EmployeeID, EmployeeName, NOW());
END;
//
DELIMITER ;
IN and OUT Parameters in Stored Procedure in SQL
In the context of stored procedures, IN
and OUT
parameters are used to pass data into and out of the procedure, respectively. Here’s an explanation of each parameter type:
- IN Parameter:
- An
IN
parameter is used to pass input values into a stored procedure. - It allows you to provide data to the procedure for processing.
- Inside the procedure, you can use the input value(s) in SQL statements or perform operations.
IN
parameters are read-only within the procedure, meaning you cannot modify their values within the procedure; they are treated as constants.- They are typically used for passing values or criteria to filter, process, or manipulate data within the procedure.
- Example:
IN search_employee_id INT
wheresearch_employee_id
is an input parameter of integer data type.
- An
- OUT Parameter:
- An
OUT
parameter is used to return output values from a stored procedure to the caller. - It allows the procedure to send data back to the caller or the calling program.
- Inside the procedure, you can assign a value to the
OUT
parameter, which will be accessible to the caller after the procedure execution. OUT
parameters are write-only within the procedure, meaning you cannot read their values within the procedure; they are used to return data.- They are typically used when a procedure needs to return one or more values or results to the caller.
- Example:
OUT total_sales DECIMAL(10, 2)
wheretotal_sales
is an output parameter of decimal data type.
- An
Here’s a MySQL stored procedure with one IN
parameter and one OUT
parameter:
DELIMITER //
CREATE PROCEDURE SampleProcedure(
IN input_param INT,
OUT output_param VARCHAR(50)
)
BEGIN
-- Logic to manipulate the input parameter
SET output_param = CONCAT('Result: ', input_param * 2);
END;
//
DELIMITER ;
Stored Procedure in SQL Examples
Let’s look into various Stored Procedures Examples.
Simple Stored Procedure with No parameters
Let’s first create the employees table and insert 5 records.
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
job_title VARCHAR(100),
salary DECIMAL(10, 2),
hire_date DATE
);
-- Insert 5 sample employee records
INSERT INTO employees (employee_id, first_name, last_name, job_title, salary, hire_date)
VALUES
(1, 'John', 'Doe', 'Software Engineer', 75000.00, '2023-09-11'),
(2, 'Alice', 'Smith', 'Data Analyst', 65000.00, '2023-09-12'),
(3, 'Bob', 'Johnson', 'Product Manager', 85000.00, '2023-09-13'),
(4, 'Eva', 'Brown', 'Marketing Specialist', 70000.00, '2023-09-14'),
(5, 'Michael', 'Williams', 'Sales Manager', 90000.00, '2023-09-15');
Now, let’s create a simple stored procedure to select all records from an employees
table:
DELIMITER //
CREATE PROCEDURE SelectEmployees()
BEGIN
-- Select all records from the 'employees' table
SELECT * FROM employees;
END;
//
DELIMITER ;
Within the procedure, we used SELECT * FROM employees
to retrieve all records from the employees
table.
You can call this stored procedure as follows:
CALL SelectEmployees();
This will execute the procedure and retrieve all records from the employees
table.
+-------------+------------+-----------+----------------------+---------+------------+
| employee_id | first_name | last_name | job_title | salary | hire_date |
+-------------+------------+-----------+----------------------+---------+------------+
| 1 | John | Doe | Software Engineer | 75000.00| 2023-09-11 |
| 2 | Alice | Smith | Data Analyst | 65000.00| 2023-09-12 |
| 3 | Bob | Johnson | Product Manager | 85000.00| 2023-09-13 |
| 4 | Eva | Brown | Marketing Specialist | 70000.00| 2023-09-14 |
| 5 | Michael | Williams | Sales Manager | 90000.00| 2023-09-15 |
+-------------+------------+-----------+----------------------+---------+------------+
Stored Procedure with Multiple IN Parameters
Let’s create a stored procedure for the “employees” table with multiple parameters. In this example, we’ll create a stored procedure that allows you to search for employees based on different criteria, such as first name, last name, or job title.
Here’s a stored procedure named SearchEmployees
with multiple parameters:
DELIMITER //
CREATE PROCEDURE SearchEmployees(
IN search_first_name VARCHAR(50),
IN search_last_name VARCHAR(50),
IN search_job_title VARCHAR(100)
)
BEGIN
-- Procedure logic to search for employees based on parameters
SELECT * FROM employees
WHERE
(search_first_name IS NULL OR first_name = search_first_name)
AND (search_last_name IS NULL OR last_name = search_last_name)
AND (search_job_title IS NULL OR job_title = search_job_title);
END;
//
DELIMITER ;
- The procedure is named
SearchEmployees
. - It accepts three input parameters:
search_first_name
,search_last_name
, andsearch_job_title
, which allow you to search for employees based on these criteria. - Inside the procedure, we use a
SELECT
statement to retrieve employees from the “employees” table based on the provided parameters. - The
WHERE
clause filters the results based on the provided parameters, and it handles cases where parameters are NULL, indicating that you want to search for employees regardless of that specific criterion.
You can call this stored procedure and pass different values for the parameters to search for specific employees. Here’s an example:
-- Search for employees with a specific first name and job title
CALL SearchEmployees('John', NULL, 'Software Engineer');
This will execute the procedure and return the employees who match the provided criteria.
+-------------+------------+-----------+----------------------+---------+------------+
| employee_id | first_name | last_name | job_title | salary | hire_date |
+-------------+------------+-----------+----------------------+---------+------------+
| 1 | John | Doe | Software Engineer | 75000.00| 2023-09-11 |
+-------------+------------+-----------+----------------------+---------+------------+
Stored Procedure with both IN and OUT parameters Example
Here’s a simple example of a stored procedure with both IN
and OUT
parameters:
DELIMITER //
CREATE PROCEDURE CalculateSalary(
IN employee_id INT,
OUT annual_salary DECIMAL(10, 2)
)
BEGIN
-- Calculate the annual salary based on employee_id
SELECT salary * 12 INTO annual_salary
FROM employees
WHERE employee_id = employee_id;
END;
//
DELIMITER ;
In this example:
CalculateSalary
is a stored procedure that accepts anIN
parameter,employee_id
, to specify the employee for whom we want to calculate the annual salary.- It also has an
OUT
parameter,annual_salary
, to return the calculated annual salary to the caller. - Inside the procedure, we use the
SELECT
statement to retrieve the monthly salary from the “employees” table, multiply it by 12 to calculate the annual salary, and assign the result to theannual_salary
parameter.
We can call this procedure and retrieve the annual_salary
value after execution using the below.
-- Declare a variable to hold the output value
SET @result = NULL;
-- Call the stored procedure and pass the employee_id as an input parameter
CALL CalculateSalary(1, @result);
-- Retrieve the result from the output variable
SELECT @result AS annual_salary;
+---------------+
| annual_salary |
+---------------+
| 900000.00 |
+---------------+
Benefits of Using Stored Procedure in SQL
- Improved Performance: Precompiled stored procedures can be more efficient as they reduce the overhead of parsing and optimizing SQL statements each time they are executed.
- Security: You can control access to stored procedures, limiting who can execute them and what they can do within the database.
- Abstraction of Logic: Complex business logic can be abstracted and centralized in stored procedures, simplifying application code.
- Reduced Network Traffic: Executing stored procedures on the database server reduces the amount of data transmitted over the network, which can be especially beneficial for remote clients.
- Transaction Control: You can manage transactions within stored procedures, ensuring data integrity and consistency.
- Code Reusability: Reusable stored procedures reduce code duplication, making it easier to maintain and update logic.
- Modularity: You can break down a complex task into smaller, modular procedures that can be called independently or as part of larger processes.
Best Practices for Using Stored Procedure in SQL
Using stored procedures in SQL effectively involves following best practices to ensure maintainability, performance, and security. Here are some best practices for using stored procedures in SQL:
- Modularization: Break down complex logic into smaller, reusable stored procedures. Each stored procedure should have a single responsibility, making it easier to maintain and debug.
- Parameterization: Use parameters in your stored procedures to make them versatile. This allows you to pass values dynamically, reducing the risk of SQL injection and improving query plan caching.
- Error Handling: Implement robust error handling within your stored procedures. Use appropriate error-handling mechanisms for your database system to gracefully handle errors and provide meaningful error messages.
- Naming Conventions: Adopt a consistent naming convention for your stored procedures. Use prefixes or naming patterns to indicate the purpose and context of the procedure.
- Documentation: Document your stored procedures thoroughly. Include information about their purpose, input parameters, output parameters, and any expected behavior.
- Testing: Thoroughly test your stored procedures before deploying them in a production environment. Include positive and negative test cases, edge cases, and boundary conditions to ensure they work correctly.
- Transaction Management: Use transactions when necessary to ensure data consistency. Begin and commit or rollback transactions within your stored procedures when dealing with multiple SQL statements that should be treated as a single unit of work.
- Avoid Overuse: Don’t overuse stored procedures for simple queries or operations that can be handled by basic SQL statements. Reserve stored procedures for complex logic, business rules, or frequently executed tasks.
- Performance Optimization: Pay attention to performance optimization. Use indexes appropriately, avoid unnecessary table scans, and consider execution plans when writing complex queries within stored procedures.
- Security: Be mindful of security concerns. Avoid dynamic SQL whenever possible, and if you must use it, validate and sanitize inputs. Grant only necessary permissions to users and applications calling the stored procedures.
- Version Control: Include your stored procedures in version control, just like application code. This helps track changes, collaborate with other developers, and manage deployments more effectively.
Conclusion: Stored Procedure in SQL
Stored procedures in SQL are powerful tools for encapsulating and executing sets of SQL statements within a database. They offer various advantages, including improved performance, security, and code reusability. Stored procedures can help streamline complex business logic and reduce network traffic by executing logic on the database server.
This article has covered the fundamental concepts of stored procedures, including their characteristics, syntax, and the use of IN and OUT parameters. We’ve explored practical examples of stored procedures, from simple procedures to more complex ones with multiple parameters. Additionally, we’ve highlighted best practices for effectively using stored procedures in SQL databases.
By following these best practices and understanding how to create, use, and manage stored procedures, database developers and administrators can harness the full potential of this SQL feature to build efficient and maintainable database applications.
Related Articles