In this detailed article on Abstract Class in Java, we will learn about the basics of Abstract Class in Java, understand about the differences between abstract class and interface, learn about some in-built abstract classes in java, then we will look into various advantages and disadvantages of abstract class in Java. Let’s get started.
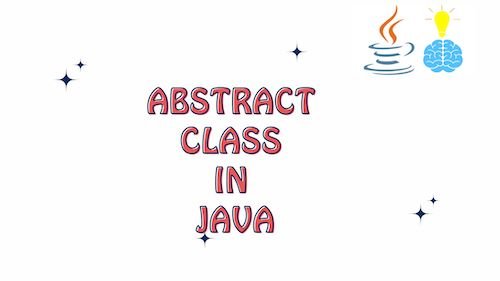
What is Abstraction in Java ?
Before jumping into Abstract class in Java, let’s try to understand Abstraction in Java briefly.
Abstraction in Java is a way to simplify complex things by focusing on their essential aspects while hiding unnecessary details. It allows you to create a blueprint for a class that outlines its structure and methods without specifying the exact implementation.
In simple words, think of abstraction as using a TV remote control. You don’t need to know how the remote works internally; you just press buttons to change channels or adjust volume. The remote abstracts the complex workings of the TV, allowing you to interact with it in a simplified manner.
Read More : Abstraction,Encapsulation and Data hiding
How do we achieve Abstraction in Java ?
In Java, abstraction is achieved through abstract classes and interfaces. These concepts allow you to create a blueprint for classes while hiding the implementation details.
What is Abstract Class in Java?
An abstract class in Java is a class that cannot be instantiated on its own and is designed to serve as a blueprint or template for other classes.
It may contain both abstract (unimplemented) methods and concrete (implemented) methods.
Abstract classes provide a way to define common behavior and structure that can be shared among multiple subclasses, while allowing each subclass to provide its own specific implementations.
Key Characteristics of Abstract Class in Java
Abstract class in Java have several key characteristics that set them apart from regular classes. Here are the main characteristics of abstract class:
- Cannot Be Instantiated: Abstract classes cannot be instantiated directly using the
new
keyword. This is because abstract classes are meant to serve as blueprints or templates for other classes, so they need to be extended by subclasses. - Abstract Methods: Abstract classes can have abstract methods, which are method declarations without implementations. These methods are marked with the
abstract
keyword and end with a semicolon, without providing any method body. Subclasses that extend the abstract class must provide implementations for these abstract methods. - Concrete Methods: Abstract classes can also have concrete methods with implementations. These methods are regular methods with method bodies. Subclasses can inherit these concrete methods and use them as-is, without being required to override them.
- Partial Implementation: Abstract classes can provide a combination of abstract and concrete methods. This allows abstract classes to offer some default behavior while leaving specific methods to be overridden by subclasses. This approach promotes code reusability and consistency among related classes.
- Inheritance: Subclasses of an abstract class inherit the fields, constructors, and methods (both abstract and concrete) defined in the abstract class. This facilitates code reuse and ensures that subclasses share a common structure.
- Method Override: Subclasses of an abstract class must provide implementations for all inherited abstract methods. If a subclass fails to implement any abstract method, it must also be declared as abstract.
- Keyword
abstract
: Abstract classes are defined using theabstract
keyword before theclass
keyword in their class declaration.
Abstract Class in Java Example
Here’s a simple example Abstract class in Java.
abstract class Animal {
// Abstract method
abstract void makeSound();
// Concrete method
void sleep() {
System.out.println("Zzz...");
}
}
class Dog extends Animal {
// Providing implementation for the abstract method
void makeSound() {
System.out.println("Dog barks!");
}
}
class Cat extends Animal {
// Providing implementation for the abstract method
void makeSound() {
System.out.println("Cat meows!");
}
}
class Main {
public static void main(String[] args) {
Dog dog = new Dog();
Cat cat = new Cat();
dog.makeSound();
dog.sleep();
cat.makeSound();
cat.sleep();
}
}
Output
Dog barks!
Zzz...
Cat meows!
Zzz...
In this example, Animal
is an abstract class with an abstract method makeSound()
and a concrete method sleep()
. The subclasses Dog
and Cat
extend the Animal
class, providing implementations for the abstract method and inheriting the concrete method.
Constructors of Abstract Class in Java
An abstract class in Java can have constructors just like any other class. Constructors in an abstract class are used to initialize the fields of the class.
Although you cannot directly instantiate an abstract class, constructors of the abstract class play a role when objects of concrete subclasses are created. Subclasses may call the constructor of the abstract class using super()
to initialize fields inherited from the abstract class.
Here’s a simple example demonstrating the use of constructors in an abstract class:
abstract class Shape {
private String name;
// Constructor in the abstract class
public Shape(String name) {
this.name = name;
}
// Abstract method
abstract void draw();
// Getter method
public String getName() {
return name;
}
}
class Circle extends Shape {
private int radius;
// Constructor in the subclass
public Circle(String name, int radius) {
super(name); // Call the constructor of the abstract class
this.radius = radius;
}
// Implementing the abstract method
void draw() {
System.out.println("Drawing a circle with radius " + radius);
}
}
public class MainClass {
public static void main(String[] args) {
Circle circle = new Circle("Circle 1", 5);
circle.draw();
System.out.println("Shape name: " + circle.getName());
}
}
Output
Drawing a circle with radius 5
Shape name: Circle 1
In this example, the abstract class Shape
has a constructor that initializes the name
field. The Circle
subclass extends Shape
and has its own constructor that calls the constructor of the abstract superclass using super()
. This ensures that the name
field is initialized properly when a Circle
object is created.
Why we use Abstract Class in Java
Abstract class in Java is used for several reasons that help in organizing code, promoting reusability, and enforcing a consistent structure in class hierarchies. Here are the main reasons why we use abstract class in Java:
- Common Base: Abstract classes serve as a common base or blueprint for related classes. They allow you to define common attributes and methods that are shared among a group of classes. This promotes code reusability and reduces redundancy.
- Partial Implementation: Abstract classes can provide a partial implementation of methods by combining abstract and concrete methods. This way, you can establish a default behavior that subclasses can inherit and optionally override.
- Contract Enforcement: Abstract classes can define a contract by including abstract methods that subclasses must implement. This ensures that all subclasses adhere to a certain set of behaviors and guarantees consistency.
- Method Template: Abstract classes can provide a template for how certain methods should be structured, with concrete methods defining the overall flow and abstract methods leaving specific details to subclasses.
- Forcing Method Override: Abstract classes force subclasses to provide implementations for specific methods. This helps in creating a structure where certain methods must be customized according to the subclass’s needs.
- Hierarchy Creation: Abstract classes enable the creation of class hierarchies. They provide a top-level structure with common attributes and methods, while subclasses extend and specialize the behavior.
- Flexibility: Abstract classes provide a balance between flexibility and structure. They allow common methods to be shared across subclasses while enabling customization where needed.
- Polymorphism: Abstract classes facilitate polymorphism, allowing you to treat objects of different subclasses as instances of the abstract class. This simplifies code and makes it more adaptable to changes.
- API Design: Abstract classes play a crucial role in designing APIs (Application Programming Interfaces). They define the core concepts and behaviors of an API, allowing developers to extend and implement custom functionality.
Limitations of Abstract Class in Java
While abstract classes offer various benefits in Java, they also come with certain limitations that developers should be aware of. Here are some of the limitations of abstract class in Java:
- Limited Inheritance: A Java class can extend only one abstract class. This limits the ability to inherit from multiple abstract classes simultaneously, which could be a constraint in complex class hierarchies.
- Inflexible for Some Designs: In cases where a class hierarchy doesn’t exhibit a clear “is-a” relationship (a subclass is a type of its superclass), using abstract classes might not be suitable. This inflexibility could lead to design challenges.
- Abstract Method Overhead: Abstract classes with abstract methods create a contract that subclasses must adhere to. However, it also means that each subclass must implement these abstract methods, which can lead to extra work and maintenance.
- Tight Coupling: Abstract classes often introduce tight coupling between the superclass and its subclasses. Changes made to the abstract class can potentially impact all its subclasses, making the design less flexible.
- Code Duplication: If multiple subclasses share similar behaviors but cannot inherit from a common abstract class, you might need to duplicate code across those subclasses.
- Complexity: As class hierarchies grow, abstract classes can introduce complexity, especially if they include numerous methods and have a deep inheritance hierarchy.
Best practices for Using Abstract Class in Java
Using abstract class in Java effectively involves following best practices to ensure clean, maintainable, and efficient code. Here are some best practices for using abstract classes:
- Follow the “is-a” Relationship: Use abstract classes when there’s a clear “is-a” relationship between the abstract class and its subclasses. Subclasses should be a specific type of the abstract class.
- Keep It Simple: Keep abstract classes focused on defining common attributes and methods that are shared among subclasses. Avoid including unrelated or complex functionality.
- Use Abstract Classes for Code Reuse: Use abstract classes to promote code reuse by providing a common base for related classes. This helps in reducing redundancy and maintaining a consistent structure.
- Use Abstract Methods Sparingly: Use abstract methods when you want to enforce a contract that all subclasses must adhere to. Avoid creating too many abstract methods in a single abstract class, as it might make the implementation burdensome for subclasses.
- Provide Default Implementations: Include concrete methods with default implementations to provide common behavior that subclasses can inherit. This helps in reducing code duplication.
- Minimize Coupling: Aim to minimize the coupling between the abstract class and its subclasses. Design the abstract class in a way that changes to it have minimal impact on subclasses.
- Avoid Deep Inheritance Hierarchies: Avoid creating deep inheritance hierarchies with many levels of abstract classes and subclasses. This can lead to complexity and maintenance challenges.
- Design for Extension: Design abstract classes with the idea that they will be extended by subclasses. Provide extension points (abstract methods) that allow subclasses to customize behavior.
- Avoid Overusing Abstract Classes: While abstract classes are useful, consider other alternatives like interfaces or composition when abstraction is not appropriate. Don’t use abstract classes solely for the sake of having a superclass.
- Use Interfaces When Appropriate: If the goal is to define a contract without any default implementations, consider using interfaces instead of abstract classes.
- Keep Abstract Classes Independent: Avoid creating dependencies between abstract classes and concrete classes. Keep the abstract class independent of specific implementations.
Difference between Abstract class and Interface
Here’s the main differences between abstract class and interface in Java:
Aspect | Abstract Class | Interface |
---|---|---|
Inheritance | Supports both abstract and concrete methods. | Supports only abstract method declarations. |
Instantiation | Cannot be instantiated directly. | Cannot be instantiated directly. |
Keyword | Defined using the abstract keyword. | Defined using the interface keyword. |
Method Definitions | Can have both abstract and concrete methods. | Can only have abstract method declarations. |
Method Access Modifier | Can use any access modifier for methods. | Methods are implicitly public and abstract . |
Fields | Can have instance variables (fields). | Can have only public , static , and final fields. |
Constructor | Can have constructors for initialization. | Cannot have constructors. |
Multiple Inheritance | Supports single class inheritance. | Supports multiple interface implementation. |
Code Reusability | Provides a way to share code through inheritance. | Provides a way to share code through implementation. |
Purpose | Used to create a base class with shared behavior. | Used to define a contract for classes to implement. |
Implementation | Subclasses inherit methods and fields. | Classes implement methods declared in the interface. |
Relationship | Represents an “is-a” relationship with subclasses. | Represents a “has-a” or “can-do” relationship. |
Default Methods | Can have default and static methods (Java 8+). | Can have default and static methods (Java 8+). |
Accessibility | Can have various access modifiers for methods. | Methods are implicitly public . |
Interface Compatibility | Cannot define instance variables. | Can be used for achieving multiple inheritance. |
Flexibility | Less flexible as a class can extend only one abstract class. | More flexible as a class can implement multiple interfaces. |
Inbuilt Abstract classes in Java
Here are some of the notable built-in abstract classes in Java:
java.io.InputStream
andjava.io.OutputStream
: These abstract classes provide the basis for input and output operations. They are used for reading and writing data from and to streams, such as files, network connections, and more.java.io.Reader
andjava.io.Writer
: Similar to input and output streams, these abstract classes deal with character-based input and output operations. They are often used for reading and writing textual data.java.util.AbstractList
,java.util.AbstractSet
, andjava.util.AbstractMap
: These abstract classes serve as templates for creating custom implementations of lists, sets, and maps. They provide common methods and a structure for custom collection classes.javax.servlet.http.HttpServlet
: This abstract class is used for creating servlets in Java web applications. Subclasses ofHttpServlet
override its methods to handle HTTP requests and responses.
Conclusion: Abstract Class in Java
In this comprehensive exploration of Abstract Class in Java, we’ve covered a wide range of topics to deepen your understanding of this fundamental concept. Let’s recap what we’ve learned in this article:
- Abstraction in Java: We began by understanding the concept of abstraction in Java.
- Achieving Abstraction: We learned that abstraction is achieved through abstract classes and interfaces in Java.
- Abstract Class Defined: An abstract class in Java is a class that serves as a blueprint or template for other classes. It cannot be instantiated on its own. It can contain both abstract (unimplemented) methods and concrete (implemented) methods. Abstract classes provide a foundation for shared behavior among subclasses.
- Key Characteristics: We explored the key characteristics of abstract classes.
- Abstract Class Example: We provided a code example illustrating the implementation of an abstract class in Java.
- Constructors of Abstract Class: We discussed how constructors work in abstract classes and how they play a role when objects of concrete subclasses are created.
- Why Use Abstract Class: We delved into the reasons why abstract classes are used in Java.
- Limitations of Abstract Class: We discussed the limitations of abstract classes.
- Best Practices: We provided a set of best practices for using abstract class in Java effectively.
- Difference Between Abstract Class and Interface: We highlighted the differences between abstract classes and interfaces.
- Inbuilt Abstract Classes: We introduced several built-in abstract classes in Java, such as
java.io.InputStream
,java.util.AbstractList
,javax.servlet.http.HttpServlet
, and others.
Understanding abstract classes is crucial for designing well-structured, reusable, and maintainable Java applications. Abstract class in Java provide a powerful mechanism for creating a common base and promoting code consistency while allowing flexibility for specialized implementations. By mastering the concepts covered in this article, you’re equipped to make informed decisions and leverage the full potential of abstract classes in your Java programming journey.
Must Read Articles: