In this article, we will explore different ways to sort an ArrayList, along with code snippets. So, let’s get started.
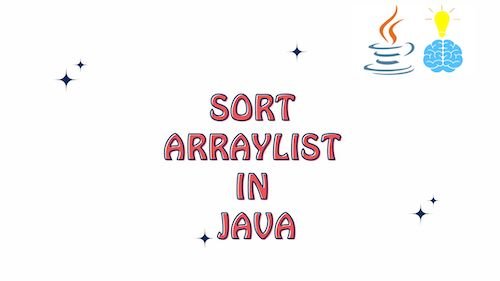
How to Sort ArrayList in Java?
Let’s look into various ways to Sort ArrayList in Java in Ascending and Descending Order.
Sort ArrayList in Java Using Collections Class Methods
We can sort an ArrayList in Java using various methods provided by the Collections
class.
Using Collections.sort() Method
The most straightforward way to sort ArrayList in Java is by using the Collections.sort()
method. This method sorts the elements of a list in their natural order or using a custom comparator.
Here’s an example of sorting an ArrayList of integers in ascending order:
import java.util.ArrayList;
import java.util.Collections;
public class ArrayListSortingUsingCollections {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(30);
numbers.add(10);
numbers.add(50);
numbers.add(20);
// Sorting ArrayList in ascending order using Collections.sort()
Collections.sort(numbers);
// Displaying the sorted ArrayList
System.out.println("Sorted ArrayList (ascending): " + numbers);
}
}
Output:
Sorted ArrayList (ascending): [10, 20, 30, 50]
Using Collections.reverseOrder() with Collections.sort()
To sort ArrayList in Java in descending order, you can use the Collections.reverseOrder()
comparator along with the Collections.sort()
method:
import java.util.ArrayList;
import java.util.Collections;
public class ArrayListSortingUsingCollections {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(30);
numbers.add(10);
numbers.add(50);
numbers.add(20);
// Sorting ArrayList in descending order using Collections.sort() and reverseOrder()
Collections.sort(numbers, Collections.reverseOrder());
// Displaying the sorted ArrayList in descending order
System.out.println("Sorted ArrayList (descending): " + numbers);
}
}
Output:
Sorted ArrayList (descending): [50, 30, 20, 10]
Sorting with a Custom Comparator
You can also sort an ArrayList based on a custom sorting order using a Comparator
. Here’s an example:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
public class ArrayListSortingDemo {
public static void main(String[] args) {
ArrayList<String> fruits = new ArrayList<>();
fruits.add("banana");
fruits.add("apple");
fruits.add("grape");
fruits.add("orange");
// Sorting ArrayList using a custom comparator
Collections.sort(fruits, new Comparator<String>() {
public int compare(String fruit1, String fruit2) {
return fruit1.compareTo(fruit2);
}
});
// Displaying the sorted ArrayList
System.out.println("Sorted ArrayList: " + fruits);
}
}
Output:
Sorted ArrayList: [apple, banana, grape, orange]
Sorting Objects with Comparable
If your ArrayList contains custom objects, you can implement the Comparable
interface in those objects to define a natural sorting order.
import java.util.ArrayList;
import java.util.Collections;
class Student implements Comparable<Student> {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public int compareTo(Student other) {
return this.name.compareTo(other.name);
}
public String toString() {
return name + " (" + age + " years old)";
}
}
public class ArrayListSortingDemo {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("Alice", 22));
students.add(new Student("Bob", 20));
students.add(new Student("Eve", 21));
// Sorting ArrayList of Student objects
Collections.sort(students);
// Displaying the sorted ArrayList
System.out.println("Sorted ArrayList: " + students);
}
}
Output:
Sorted ArrayList: [Alice (22 years old), Bob (20 years old), Eve (21 years old)]
Sort ArrayList in Java using ArrayList.sort() Method (Java 8+)
Starting from Java 8, the ArrayList class itself has a sort()
method that can be used for sorting. This method allows you to provide a custom comparator if needed.
import java.util.ArrayList;
public class ArrayListSortingDemo {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(30);
numbers.add(10);
numbers.add(50);
numbers.add(20);
// Using ArrayList.sort() to sort the ArrayList
numbers.sort(null); // Using natural order (ascending)
// Displaying the sorted ArrayList
System.out.println("Sorted ArrayList: " + numbers);
}
}
Output:
Sorted ArrayList: [10, 20, 30, 50]
You can use the ArrayList.sort()
method with a custom comparator to sort the ArrayList in descending order.
import java.util.ArrayList;
import java.util.Comparator;
public class ArrayListSortingDescending {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(30);
numbers.add(10);
numbers.add(50);
numbers.add(20);
// Sorting ArrayList in descending order using ArrayList.sort() and custom comparator
numbers.sort(Comparator.reverseOrder());
// Displaying the sorted ArrayList
System.out.println("Sorted ArrayList (descending): " + numbers);
}
}
Output:
Sorted ArrayList (descending): [50, 30, 20, 10]
Sort ArrayList in Java using the Stream API
The Stream API was introduced in Java 8 and allows you to perform various operations on collections, including sorting. Here’s how you can use the Stream API to sort ArrayList in Java:
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class ArrayListSortingWithStream {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(30);
numbers.add(10);
numbers.add(50);
numbers.add(20);
// Sorting ArrayList using Stream API
List<Integer> sortedNumbers = numbers.stream()
.sorted() // Using natural order (ascending)
.collect(Collectors.toList());
// Displaying the sorted ArrayList
System.out.println("Original ArrayList: " + numbers);
System.out.println("Sorted ArrayList: " + sortedNumbers);
}
}
Output:
Original ArrayList: [30, 10, 50, 20]
Sorted ArrayList: [10, 20, 30, 50]
You can also use a custom comparator with the sorted()
method to achieve a different sorting order.
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.stream.Collectors;
class Employee {
private String name;
private int age;
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public String toString() {
return name + " (" + age + " years old)";
}
}
public class ArrayListSortingWithCustomComparator {
public static void main(String[] args) {
ArrayList<Employee> employees = new ArrayList<>();
employees.add(new Employee("Alice", 30));
employees.add(new Employee("Bob", 25));
employees.add(new Employee("Eve", 28));
// Sorting ArrayList using Stream API with a custom comparator
List<Employee> sortedEmployees = employees.stream()
.sorted(Comparator.comparing(Employee::getName)) // Custom comparator
.collect(Collectors.toList());
// Displaying the sorted ArrayList
System.out.println("Original ArrayList: " + employees);
System.out.println("Sorted ArrayList: " + sortedEmployees);
}
}
Output:
Original ArrayList: [Alice (30 years old), Bob (25 years old), Eve (28 years old)]
Sorted ArrayList: [Alice (30 years old), Bob (25 years old), Eve (28 years old)]
You can use the Stream API to sort the ArrayList in descending order using the sorted()
method with a custom comparator.
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.stream.Collectors;
public class ArrayListSortingDescending {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(30);
numbers.add(10);
numbers.add(50);
numbers.add(20);
// Sorting ArrayList in descending order using Stream API
List<Integer> sortedNumbers = numbers.stream()
.sorted(Comparator.reverseOrder())
.collect(Collectors.toList());
// Displaying the sorted ArrayList
System.out.println("Original ArrayList: " + numbers);
System.out.println("Sorted ArrayList (descending): " + sortedNumbers);
}
}
Output:
Original ArrayList: [30, 10, 50, 20]
Sorted ArrayList (descending): [50, 30, 20, 10]
Conclusion: Sort ArrayList in Java
In this comprehensive post, we thoroughly explored different ways to sort ArrayList in Java using different methods.
Related Article: