In this article, we will take a detailed look at Interface in Java and understand it thoroughly. So, let’s get started.
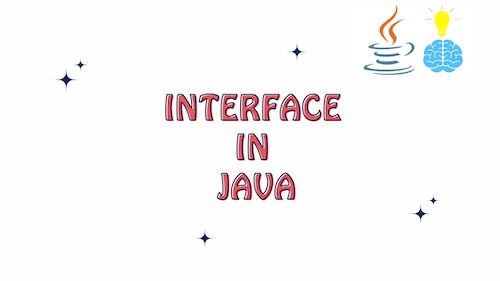
What is Interface in Java ?
Interface in Java defines a set of methods that classes must implement. It allows us to achieve multiple inheritance and create code that’s more flexible and reusable. Interfaces help ensure that classes adhere to a certain contract, making our code more organized and adaptable.
Interface in Java Example
Here’s a simple example of an interface in Java:
// Define an interface named Shape
interface Shape {
void draw(); // An abstract method without implementation
}
// Implement the Shape interface in a class
class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
// Implement the Shape interface in another class
class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle");
}
}
// Main class to demonstrate interface usage
public class Main {
public static void main(String[] args) {
Shape circle = new Circle();
Shape rectangle = new Rectangle();
circle.draw(); // Output: Drawing a circle
rectangle.draw(); // Output: Drawing a rectangle
}
}
In this example, the Shape
interface defines a method draw()
. The Circle
and Rectangle
classes implement this interface and provide their own implementations of the draw()
method.
Difference between Class and Interface in Java
Aspect | Class | Interface |
---|---|---|
Definition | Blueprint for creating objects. | Contract for method implementation. |
Inheritance | Extends one class. | Implemented by multiple classes. |
Multiple Inheritance | Not supported (extends one class). | Supported (implements multiple interfaces). |
Constructors | Can have constructors. | Cannot have constructors. |
Fields | Can have instance variables (fields). | Can have constants (static final fields). |
Method Definition | Can have methods with implementation. | Methods are abstract by default (no implementation). |
Abstract Classes | Can be abstract with some methods not implemented. | Cannot extend multiple classes (single inheritance). |
Instantiation | Objects instantiated from classes. | Objects not directly instantiated from interfaces. |
Access Modifiers | Public, private, protected, default. | Methods are implicitly public and abstract. |
Extending | Extends another class using ‘extends’. | Implements an interface using ‘implements’. |
Purpose | Represents objects with attributes and behaviors. | Defines method contract for implementing classes. |
Use Case | Creating objects and defining behaviors. | Achieving multiple inheritance and enforcing contracts. |
Rules for Creating Interface in Java
Here are the rules for creating an interface in Java:
- Access Modifier: An interface can be declared with the access modifiers
public
ordefault
. - Method Declaration: Declare methods without specifying the method body. Methods are implicitly
public
andabstract
. - Constant Fields: Declare constants (static final fields) in the interface. They are implicitly
public
,static
, andfinal
. - Extending Interfaces: An interface can extend multiple interfaces using the
extends
keyword. - Method Signatures: Implementing classes must provide method implementations with the exact method signatures defined in the interface.
- Method Access Modifier: Implementing methods should have an access modifier that is either
public
orprotected
. - Overriding Object Class Methods: Interfaces cannot override the
equals
,hashCode
, andtoString
methods from theObject
class. - Inheritance Hierarchy: Sub-interfaces inherit methods and constants from parent interfaces.
- Implementing Classes: Classes that implement an interface must provide concrete implementations for all interface methods.
- Abstract Classes: Interfaces cannot be declared as
abstract
since they are inherently abstract. - Constructor: Interfaces cannot have constructors as they cannot be instantiated directly.
- Default Methods (Java 8+): Interfaces can have default methods with method bodies. Implementing classes inherit default method implementations.
- Static Methods (Java 8+): Interfaces can have static methods with method bodies, which can be called using the interface name.
- Private Methods (Java 9+): Interfaces can have private methods with method bodies, primarily to assist in code organization within the interface.
- Functional Interfaces (Java 8+): An interface with a single abstract method is considered a functional interface and can be used with lambda expressions.
Types of Interfaces in Java
In Java, there are three types of interfaces based on their functionality and purpose. Here are the main types of interfaces:
- Normal Interface: This is the most common type of interface, which declares a set of abstract methods that implementing classes need to provide implementations for. Normal interfaces do not have any special qualifiers.
- Marker Interface: Also known as a “tag” interface, a marker interface doesn’t declare any methods. It’s used to mark a class for a certain behavior or capability. For example, the
Serializable
interface is a marker interface that indicates a class can be serialized. - Functional Interface: A functional interface has exactly one abstract method and is used for functional programming with lambda expressions. It’s often used in conjunction with Java’s lambda expressions or method references. The
java.util.function
package contains several functional interfaces likePredicate
,Consumer
,Function
, etc.
Commonly used Built-in Interfaces in Java
Here are some commonly used built-in interfaces in Java:
- Comparable: This interface is used to provide a natural ordering of objects. Classes that implement this interface can be sorted using methods like
Collections.sort()
. - Runnable: This interface is used to create threads by providing a
run()
method that contains the code to be executed by the thread. - Cloneable: This interface is used to indicate that a class can be cloned using the
Object.clone()
method. - Serializable: This interface is used to indicate that a class can be serialized, allowing its instances to be converted into a byte stream for storage or transmission.
- Iterable: This interface is used to make an object iterable, enabling it to be used in enhanced for loops. It requires the implementation of the
iterator()
method. - Collection: This interface defines the basic methods for working with collections of objects, such as adding, removing, and querying elements.
- List: This interface extends the
Collection
interface and represents an ordered collection of elements. It allows duplicate elements and maintains their insertion order. - Set: This interface also extends the
Collection
interface but represents a collection of unique elements. It does not allow duplicate elements. - Map: This interface represents a collection of key-value pairs. It does not extend the
Collection
interface, as it focuses on the association between keys and values. - Queue: This interface represents a collection designed for holding elements prior to processing. It typically follows a first-in, first-out (FIFO) order.
- Deque: Short for “double-ended queue,” this interface extends the
Queue
interface to support operations at both ends. - ListIterator: This interface extends the
Iterator
interface and provides additional methods for iterating over lists, allowing bidirectional traversal. - Function: This functional interface represents a function that takes an argument and produces a result. It is often used with lambda expressions.
- Predicate: Another functional interface, it represents a predicate (a condition) that takes an argument and returns a boolean value.
- Consumer: This functional interface represents an operation that takes an argument and performs an action without returning any result.
- Supplier: This functional interface represents a supplier of results, generating or providing values without taking any input.
Conclusion: Interface in Java
We explored the concept of interface in Java in great details. Interfaces serve as contracts that define methods to be implemented by classes, enabling flexible and reusable code. By allowing multiple inheritance and enforcing method contracts, interfaces enhance code organization and adaptability.
The provided code example illustrated how interfaces work, where classes implement the interface’s methods. We also explored the key distinctions between classes and interfaces, ranging from constructors to inheritance and access modifiers.
Additionally, we learned the essential rules for creating interfaces, such as access modifiers, method declarations, constant fields, and more. Different types of interfaces, including normal, marker, and functional interfaces, were discussed, each serving distinct purposes in Java programming.
Finally, we highlighted commonly used built-in interfaces, from Comparable for sorting to functional interfaces like Predicate and Supplier. These built-in interfaces play a crucial role in Java’s versatility, offering solutions for tasks like multithreading, collection manipulation, and functional programming.