In this article, we will explore multiple ways for Exception handling in Spring Boot. So, let’s get started.
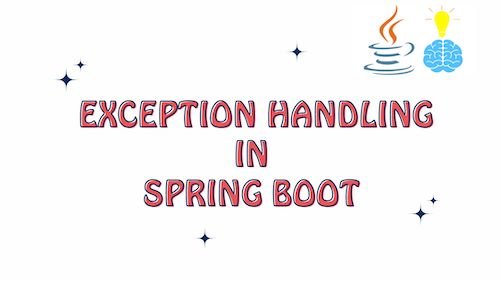
Different Ways: Exception Handling in Spring Boot
In Spring Boot, we have multiple ways of Exception handling in Spring Boot. Let’s explore these.
Global Exception Handling
Spring Boot allows you to define a global exception handler using the @ControllerAdvice
annotation. This class can contain methods annotated with @ExceptionHandler
, which handle specific types of exceptions. This approach centralizes error handling logic, making it easier to manage and maintain.
Example:
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.http.ResponseEntity;
import org.springframework.http.HttpStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
// Customize the response entity with error details
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An internal server error occurred.");
}
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFoundException(ResourceNotFoundException e) {
// Customize the response entity for the specific exception
return ResponseEntity.status(HttpStatus.NOT_FOUND)
.body("Resource not found: " + e.getMessage());
}
// Add more exception handlers for different types of exceptions
}
In this example, we’ve defined a global exception handler using the @ControllerAdvice
annotation. The class GlobalExceptionHandler
contains methods annotated with @ExceptionHandler
to handle specific types of exceptions.
When an exception occurs in any part of your application, Spring Boot will automatically route the exception to the appropriate method based on its type. The method then returns a ResponseEntity
with the desired HTTP status code and response body, allowing you to provide meaningful error messages.
Handling Exception Using @RestControllerAdvice
In addition to @ControllerAdvice
, Spring Boot offers @RestControllerAdvice
, which combines @ControllerAdvice
and @ResponseBody
. It’s useful when you want to return response data directly from the exception handling methods.
Here’s an example of using @RestControllerAdvice
for global exception handling in a Spring Boot application:
import org.springframework.web.bind.annotation.RestControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.http.ResponseEntity;
import org.springframework.http.HttpStatus;
@RestControllerAdvice
public class GlobalRestControllerAdvice {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
// Customize the response entity with error details
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An internal server error occurred.");
}
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFoundException(ResourceNotFoundException e) {
// Customize the response entity for the specific exception
return ResponseEntity.status(HttpStatus.NOT_FOUND)
.body("Resource not found: " + e.getMessage());
}
// Add more exception handlers for different types of exceptions
}
In this example, we’re using @RestControllerAdvice
instead of @ControllerAdvice
. The purpose of @RestControllerAdvice
is the same as before, but it combines the behavior of @ControllerAdvice
and @ResponseBody
, allowing you to return response data directly from the exception handling methods.
By using @RestControllerAdvice
, you can handle exceptions globally while returning JSON or XML responses directly from the exception handling methods. This approach is particularly useful when building RESTful APIs where you want to provide consistent and meaningful error responses to clients.
Using Custom Error Pages
Custom error pages are a way to enhance the user experience by providing user-friendly and informative error messages when an exception occurs in your Spring Boot application. These error pages are displayed to users when they encounter errors, helping them understand the problem and suggesting potential solutions. Here’s how you can create custom error pages in a Spring Boot application:
Create Error Page HTML Files: In your src/main/resources/templates
directory, create HTML files for different error status codes. For example, if you want to create a custom error page for a 404 Not Found error, create a file named error-404.html
.
Configure Custom Error Pages: In your application.properties
or application.yml
file, configure the custom error pages by specifying the mapping of error status codes to the corresponding HTML file names. For example:
server.error.whitelabel.enabled=false
server.error.path=/error
By setting server.error.path
, you can map error responses to a specific URL endpoint.
Handle Uncaught Exceptions: Custom error pages are displayed for uncaught exceptions. To handle specific exceptions, you can still use global or specific exception handlers as shown in the previous examples.
Testing Custom Error Pages: To test your custom error pages, intentionally trigger an error by accessing a non-existent endpoint or causing an exception. The custom error page corresponding to the error status code should be displayed.
Here’s a simple example of a custom error page:
Create an HTML file named error-404.html
in the src/main/resources/templates
directory:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>404 Not Found</title>
</head>
<body>
<h1>404 Not Found</h1>
<p>The requested page could not be found.</p>
<p>Please check the URL or go back to the <a href="/">homepage</a>.</p>
</body>
</html>
When a 404 error occurs in your application, the custom error page will be displayed to the user, providing a user-friendly explanation of the error.
Conclusion: Exception Handling in Spring Boot
In this comprehensive article “Exception Handling in Spring Boot”, we delved into various ways of handling exceptions in Spring Boot applications. Here are the key takeaways:
- Global Exception Handling: Spring Boot facilitates the creation of a global exception handler using
@ControllerAdvice
. This approach consolidates error handling logic, leading to better code organization and maintenance. We examined how to define methods annotated with@ExceptionHandler
to handle specific exceptions, enabling central error management. - Handling Exceptions Using @RestControllerAdvice: The introduction of
@RestControllerAdvice
combines the capabilities of@ControllerAdvice
and@ResponseBody
. By presenting a code example, we demonstrated how this annotation streamlines the process of returning response data directly from exception handling methods. This is particularly advantageous for APIs that require consistent and meaningful error responses. - Custom Error Pages: Creating custom error pages offers users a more intuitive experience when encountering errors. We explored the steps to design and configure HTML-based error pages for different error status codes. This method promotes user engagement and assists them in diagnosing issues by providing clear error explanations and potential solutions.
By incorporating these approaches into your Spring Boot application, you can ensure robust error handling mechanisms that not only improve user satisfaction but also contribute to the overall reliability and usability of your application.
Related Articles on Spring Boot