In this article, we will have a detailed look into Inner Join in SQL and discuss various aspects of it. So, let’s get started.
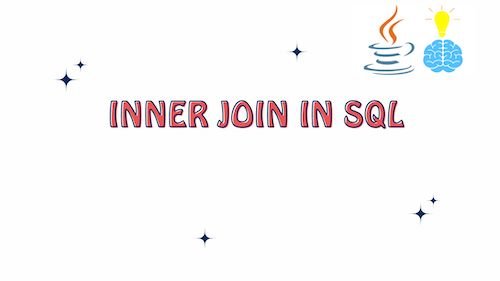
What is Inner Join in SQL ?
An inner join in SQL is used to combine rows from two or more tables based on a related column between them. The result of an inner join includes only the rows for which there is a match in both tables, according to the specified join condition.
Here’s the basic syntax for an inner join in SQL:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column_name = table2.column_name;
Example: Inner Join in SQL
Now, let’s illustrate the concept with examples using two tables: Customers
and Orders
.
Customers Table:
CustomerID | CustomerName | ContactName | Country |
---|---|---|---|
1 | Customer A | John Smith | USA |
2 | Customer B | Jane Doe | UK |
3 | Customer C | Alice Brown | Canada |
4 | Customer D | Bob Johnson | Australia |
Orders Table:
OrderID | CustomerID | OrderDate | ShipDate |
---|---|---|---|
101 | 1 | 2023-01-10 | 2023-01-15 |
102 | 2 | 2023-02-05 | 2023-02-10 |
103 | 1 | 2023-03-20 | 2023-03-25 |
104 | 4 | 2023-04-15 | 2023-04-20 |
Example 1: Inner Join Customers and Orders
In this example, we’ll perform an inner join between the Customers
and Orders
tables using the CustomerID
column as the common key.
SELECT Customers.CustomerName, Orders.OrderDate
FROM Customers
INNER JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
The result of this inner join will include rows where there is a match between the CustomerID
in the Customers
table and the CustomerID
in the Orders
table:
CustomerName | OrderDate |
---|---|
Customer A | 2023-01-10 |
Customer B | 2023-02-05 |
Customer A | 2023-03-20 |
Customer D | 2023-04-15 |
Example 2: Inner Join with Aliases
You can use table aliases to make your SQL code more concise:
SELECT c.CustomerName, o.OrderDate
FROM Customers AS c
INNER JOIN Orders AS o
ON c.CustomerID = o.CustomerID;
This query achieves the same result as the previous example but uses aliases c
for Customers
and o
for Orders
to make the code more readable.
Example 3: Inner Join between three Tables
Here’s an example of joining three tables using aliases in SQL. In this example, we have three tables: Employees
, Departments
, and Salaries
, and we want to retrieve information about employees, their departments, and their salaries.
Employees Table:
EmployeeID | EmployeeName | DepartmentID |
---|---|---|
1 | John | 101 |
2 | Emily | 102 |
3 | Michael | 103 |
4 | Sarah | 101 |
5 | David | 104 |
Departments Table:
DepartmentID | DepartmentName |
---|---|
101 | HR |
102 | IT |
103 | Sales |
104 | Marketing |
Salaries Table:
EmployeeID | Salary |
---|---|
1 | 5000 |
2 | 5500 |
3 | 6000 |
4 | 4800 |
5 | 5200 |
Now, let’s perform an inner join between these three tables using aliases to retrieve employee information along with their departments and salaries:
SELECT e.EmployeeName, d.DepartmentName, s.Salary
FROM Employees AS e
INNER JOIN Departments AS d
ON e.DepartmentID = d.DepartmentID
INNER JOIN Salaries AS s
ON e.EmployeeID = s.EmployeeID;
The result of this query will provide employee names, department names, and their respective salaries:
EmployeeName | DepartmentName | Salary |
---|---|---|
John | HR | 5000 |
Emily | IT | 5500 |
Michael | Sales | 6000 |
Sarah | HR | 4800 |
David | Marketing | 5200 |
Advantages of Inner Join in SQL
- Precise Data Retrieval: Inner joins retrieve only the rows that have matching values in both tables based on the specified join condition. This ensures that you get precise and relevant data.
- Efficient Query Performance: Inner joins are typically more efficient than other types of joins (e.g., cross joins or full outer joins) because they reduce the result set by only including matching rows. This can lead to faster query execution, especially with large datasets.
- Data Integrity: Inner joins enforce data integrity by ensuring that you retrieve data from related tables where relationships exist. This helps maintain the integrity of your database.
- Support for Multiple Tables: Inner joins can be used to combine more than two tables, enabling complex queries that involve multiple related tables.
Disadvantages of Inner Join in SQL
- Complex Query Writing: Writing SQL queries with multiple inner joins can become complex, especially when dealing with many tables and complex join conditions. This complexity can make queries harder to read and maintain.
- Potential Performance Issues: While inner joins are generally efficient, they can become less performant if the tables being joined are large and not properly indexed. It’s essential to consider indexing strategies for optimal performance.
- Limited Use in Some Scenarios: Inner joins are not suitable for scenarios where you need to include rows from one table even if there are no matches in the other table. In such cases, other types of joins (e.g., left joins or right joins) should be used.
- Ambiguity in Column Names: If the tables being joined have columns with the same name, it can lead to ambiguity in query results. To address this, you may need to use table aliases or explicitly qualify column names.
Conclusion: Inner Join in SQL
In this article, we delved into the world of Inner Join in SQL, covering various aspects and practical examples.
We explored the basic syntax of inner joins and examined real-world examples using tables like Customers and Orders.
Additionally, we learned how to enhance the readability of SQL queries by using table aliases, making the code more concise and manageable.
Furthermore, we delved into advanced scenarios by illustrating a three-table inner join involving Employees, Departments, and Salaries.
Then we discussed the advantages of inner joins, such as precise data retrieval, efficient query performance, and support for multiple tables. We also highlighted potential drawbacks, including complex query writing, performance concerns with large unindexed tables, and limitations in certain scenarios.
Related Articles :