In this article, we will explore various aspects of “DML Commands in SQL”. Let’s get started.
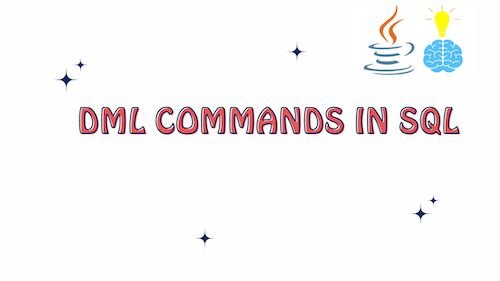
What are DML Commands in SQL ?
DML, or Data Manipulation Language, commands in SQL are a subset of SQL commands used to interact with and manipulate data stored in relational database management systems (RDBMS). DML commands are responsible for performing operations such as inserting, retrieving, updating, and deleting data within database tables. They are essential for managing the content of a database and ensuring that the data remains accurate and up-to-date.
The primary DML commands in SQL include:
- SELECT: The SELECT statement retrieves data from one or more database tables. It allows you to specify the columns to retrieve, filter data based on conditions, and sort the results.
- INSERT: The INSERT statement adds new rows of data into a table. You can either insert a single row or multiple rows in a single statement. Data can be inserted explicitly or by selecting from another table.Read More : Insert Query in SQL
- UPDATE: The UPDATE statement modifies existing data in a table. It allows you to change the values of one or more columns in specified rows, typically based on a condition. Read More : Update Query in SQL: Comprehensive Guide
- DELETE: The DELETE statement removes rows from a table based on specified conditions. It is used to permanently delete data from a table. Read More : Delete Query in SQL: Complete Insights
DML Commands vs DDL Commands in SQL
Here’s a comparison of DML (Data Manipulation Language) commands and DDL (Data Definition Language) commands in SQL:
Aspect | DML Commands | DDL Commands |
---|---|---|
Purpose | Perform operations on data, such as retrieval, insertion, update, and deletion. | Define and manage the structure of the database, including tables, schema, constraints, and indexes. |
Examples | SELECT, INSERT, UPDATE, DELETE | CREATE, ALTER, DROP, TRUNCATE |
Target | Data within tables. | Database objects like tables, schemas, indexes, views, etc. |
Transaction Impact | Can be part of a transaction and contribute to its ACID properties (Atomicity, Consistency, Isolation, Durability). | Typically, DDL commands are auto-committed, and they are not part of a transaction. |
Data Integrity | DML commands are primarily responsible for maintaining data integrity, ensuring data consistency within tables. | DDL commands focus on defining and enforcing constraints to maintain data integrity at the structural level. |
Impact on Schema | DML commands do not change the schema or structure of the database. | DDL commands are responsible for defining, altering, and dropping database objects, affecting the schema. |
Examples of Use | – SELECT retrieves customer information. – INSERT adds a new order to the sales table. – UPDATE modifies the price of a product. – DELETE removes inactive users from the user table. | – CREATE TABLE defines a new table structure. – ALTER TABLE adds a new column to an existing table. – DROP TABLE deletes an entire table. – CREATE INDEX creates an index on a column. |
Rollback and Commit | DML commands can be rolled back or committed as part of a transaction, allowing data changes to be undone or saved permanently. | DDL commands are auto-committed, so they cannot be rolled back. |
Committing and Rolling Back Transactions in DML Statements in SQL
In SQL, you can use transactions to group one or more DML (Data Manipulation Language) statements together and ensure that they are either all executed successfully (committed) or none of them are executed (rolled back) in case of an error or failure. Transactions are crucial for maintaining the consistency and integrity of the database. To commit or roll back transactions in DML statements, you typically use the COMMIT
and ROLLBACK
statements. Here’s how it works:
Committing a Transaction:
- When you want to permanently save the changes made by your DML statements, you issue a
COMMIT
statement.TheCOMMIT
statement makes all the changes within the transaction permanent and visible to other database users.After committing, you cannot roll back the changes; they become part of the database.The syntax to commit a transaction in SQL is simplyCOMMIT;
.
-- Start a transaction
BEGIN TRANSACTION;
-- Execute DML statements
INSERT INTO orders (order_id, customer_id, order_date) VALUES (1, 101, '2023-09-01');
UPDATE inventory SET quantity = quantity - 5 WHERE product_id = 1001;
-- Commit the transaction
COMMIT;
Rolling Back a Transaction:
- If any part of your DML statements encounters an error or you decide not to save the changes, you can roll back the entire transaction.
- Rolling back undoes all the changes made within the transaction, reverting the database to its state before the transaction started.
- The syntax to roll back a transaction in SQL is
ROLLBACK;
.
Example:
-- Start a transaction
BEGIN TRANSACTION;
-- Execute DML statements
INSERT INTO orders (order_id, customer_id, order_date) VALUES (1, 101, '2023-09-01');
-- Simulate an error or decide not to save changes
-- ROLLBACK to undo the changes
ROLLBACK;
Best Practices for Using DML Commands in SQL
Using DML commands in SQL, it’s essential to follow best practices to ensure data accuracy, maintainability, and security. Here are some best practices for using DML commands in SQL:
- Use Transactions: Wrap multiple DML statements within a transaction to ensure data consistency. Transactions allow you to commit or roll back changes as a single unit of work, preventing partial updates in case of errors or interruptions.
- Validate Data: Always validate data before executing DML commands. Ensure that the data meets the required constraints and business rules to prevent data integrity issues.
- Use Prepared Statements or Parameterized Queries: When working with dynamic data or data from user inputs, use prepared statements or parameterized queries to prevent SQL injection attacks. This practice helps to sanitize and escape input values.
- Specify Columns Explicitly: When inserting data, explicitly specify the columns you’re inserting into. This makes your intent clear and ensures that your query remains valid even if the table structure changes. Avoid using the shorthand
INSERT INTO table VALUES (...)
unless it’s necessary. - *Avoid SELECT : When updating or deleting data, avoid using
SELECT *
to retrieve all columns when only specific columns are needed. This reduces unnecessary data retrieval and improves query performance. - Limit the Use of DELETE without WHERE: Be cautious when using the
DELETE
statement without aWHERE
clause. If you need to delete all rows, consider usingTRUNCATE TABLE
(if applicable) or implement other means of data archiving. - Backup Data: Before performing mass updates or deletions, especially in a production environment, ensure you have a recent backup of your data. This precaution can help recover lost data in case of accidental changes.
- Use JOINS Carefully: When joining multiple tables in DML statements, ensure you understand the impact of the join. Test your queries thoroughly to avoid accidentally updating or deleting more data than intended.
- Check for Indexes: Ensure that your table has appropriate indexes on columns frequently used in WHERE clauses or join conditions. Indexes can significantly improve DML command performance.
- Review Execution Plan: Use tools like
EXPLAIN
(or equivalent in your database system) to analyze the execution plan of your DML statements. This can help identify potential performance bottlenecks. - Use Conditional Updates: When updating data, use conditional statements (e.g.,
WHERE
clause) to update only the rows that meet specific criteria. This prevents unintentional updates to unrelated rows. - Avoid Using Cursors: In some cases, cursor-based DML operations can be inefficient. Whenever possible, use set-based operations to update or delete data, as these are generally more efficient.
- Limit Batch Sizes: When performing batch updates or deletes, consider limiting the batch size to avoid excessive locks or transaction log growth.
Conclusion: DML Commands in SQL
In this article, we explored various aspects of “DML Commands in SQL.” We began by understanding what DML (Data Manipulation Language) commands are and their importance in interacting with and manipulating data within relational database management systems (RDBMS). The primary DML commands, including SELECT, INSERT, UPDATE, and DELETE, were introduced, each serving specific purposes in managing database content.
Next, we compared DML commands with DDL (Data Definition Language) commands, highlighting their differences in purpose, target, transaction impact, data integrity, impact on schema, and examples of use. This comparison helped clarify when to use each type of command in SQL.
We also delved into the critical topic of committing and rolling back transactions in DML statements. Transactions were explained as essential for maintaining data consistency and integrity, with clear instructions on how to commit changes using the COMMIT statement and how to roll back changes using the ROLLBACK statement.
To conclude, we provided a set of best practices for using DML commands in SQL, emphasizing the importance of transactions, data validation, prepared statements, explicit column specification, query optimization, data backup, and more. Following these best practices ensures the reliability, security, and efficiency of DML operations while minimizing the risk of data-related issues.
Related Articles :