In this article, we will have a detailed exploration of “Window Functions in SQL” using various examples. Let’s get started.
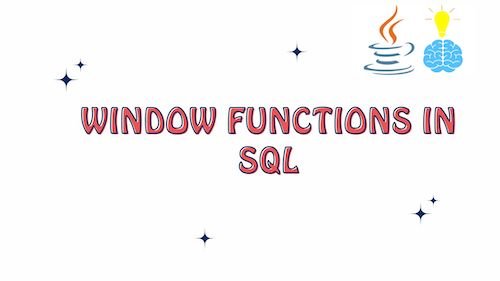
What are Window Functions in SQL ?
Window functions in SQL are a powerful and flexible set of functions that allow you to perform calculations across a set of table rows related to the current row within a result set. These functions operate within a “window” of rows defined by an OVER() clause, which can include a specified range of rows or a logical partition of the result set. Window functions are particularly useful for complex analytical queries and are commonly used in reporting and data analysis tasks.
Here are some key characteristics and features of window functions:
- Calculation Across Rows: Window functions enable you to perform calculations that involve multiple rows from a result set without reducing the number of rows in the output.
- Partitioning Data: You can partition your result set into logical groups or partitions using the PARTITION BY clause. Window functions are then applied independently within each partition.
- Ordering Data: You can specify the order of rows within each partition using the ORDER BY clause. This determines the sequence in which rows are processed by the window function.
- Access to Multiple Rows: Window functions can access data from rows that precede or follow the current row within the defined window frame. This allows for calculations involving both past and future data.
- Ranking and Aggregation: Window functions include various types, such as RANK(), DENSE_RANK(), ROW_NUMBER(), SUM(), AVG(), and more. These functions can be used for ranking rows, calculating running totals, aggregating data within partitions, and more.
- Flexibility: Window functions provide a high degree of flexibility for creating complex queries. You can combine multiple window functions, nest them, and use different partitioning and ordering criteria to achieve the desired results.
Common use cases for window functions in SQL include:
- Calculating running totals and cumulative sums.
- Ranking rows based on certain criteria within partitions.
- Calculating moving averages and trends in time-series data.
- Identifying gaps and overlaps in date ranges.
- Finding the first or last occurrence of an event within a group.
- Calculating percentiles and quartiles within partitions.
Popular database systems like PostgreSQL, SQL Server, MySQL, Oracle, and SQLite support window functions, although the syntax and available functions may vary slightly between database systems.
Types of Window Functions in SQL
Here are some common types of window functions:
Ranking Functions
- ROW_NUMBER(): Assigns a unique integer to each row within the result set, starting from 1. It doesn’t handle ties and assigns a new number to each row.
- RANK(): Assigns a unique rank to each row within the result set. Rows with the same values receive the same rank, and the next rank is skipped.
- DENSE_RANK(): Similar to RANK(), but it doesn’t skip ranks for tied rows. Tied rows receive the same rank, and the next rank is continuous.
Here’s an example of ranking functions in SQL, specifically using the RANK()
function:
Let’s say we have a table named sales
with the following data:
-- Create the sales table
CREATE TABLE sales (
Salesperson VARCHAR(255),
SalesAmount INT
);
-- Insert sample data into the sales table
INSERT INTO sales (Salesperson, SalesAmount)
VALUES
('Alice', 5000),
('Bob', 8000),
('Charlie', 5000),
('David', 7000),
('Eve', 9000);
| Salesperson | SalesAmount |
|-------------|-------------|
| Alice | 5000 |
| Bob | 8000 |
| Charlie | 5000 |
| David | 7000 |
| Eve | 9000 |
We want to rank the salespeople based on their sales amounts in descending order. In this case, both Alice and Charlie have the same sales amount, so they should have the same rank. We’ll use the RANK()
function to achieve this:
SELECT
Salesperson,
SalesAmount,
RANK() OVER (ORDER BY SalesAmount DESC) AS SalesRank
FROM
sales;
In this SQL query:
- We select the
Salesperson
andSalesAmount
columns from thesales
table. - We use the
RANK()
function as a window function. TheOVER
clause specifies that we want to rank the rows based on theSalesAmount
column in descending order (ORDER BY SalesAmount DESC
).
The result of this query will give us the ranking of each salesperson based on their sales amounts:
| Salesperson | SalesAmount | SalesRank |
|-------------|-------------|-----------|
| Eve | 9000 | 1 |
| Bob | 8000 | 2 |
| David | 7000 | 3 |
| Alice | 5000 | 4 |
| Charlie | 5000 | 4 |
Aggregate Functions with OVER()
Aggregate functions with the OVER()
clause are a powerful way to perform calculations across a specified window of rows within a result set. These functions allow you to calculate aggregations like sums, averages, counts, and more while maintaining the granularity of the data. Here’s an example using the SUM()
and AVG()
aggregate functions with the OVER()
clause:
Let’s use the above Sales Table. We want to calculate the total sales amount and the average sales amount for each salesperson while maintaining the individual sales rows. We can achieve this using the SUM()
and AVG()
aggregate functions with the OVER()
clause:
SELECT
Salesperson,
SalesAmount,
SUM(SalesAmount) OVER () AS TotalSales,
AVG(SalesAmount) OVER () AS AverageSales
FROM
sales;
In this SQL query:
- We select the
Salesperson
andSalesAmount
columns from thesales
table. - We use the
SUM(SalesAmount)
aggregate function withOVER ()
to calculate the total sales amount across all rows in the result set. The()
specifies that there is no partitioning or ordering, so it aggregates all rows. - We use the
AVG(SalesAmount)
aggregate function withOVER ()
to calculate the average sales amount across all rows in the result set.
The result of this query will include the individual sales rows along with the total sales and average sales calculated for all rows:
| Salesperson | SalesAmount | TotalSales | AverageSales |
|-------------|-------------|------------|--------------|
| Alice | 5000 | 34000 | 6800 |
| Bob | 8000 | 34000 | 6800 |
| Charlie | 5000 | 34000 | 6800 |
| David | 7000 | 34000 | 6800 |
| Eve | 9000 | 34000 | 6800 |
Lead and Lag Functions
The LAG()
and LEAD()
functions in SQL are used to access values from previous or subsequent rows within a specified window frame. These functions are particularly useful for time-series analysis and comparing data across consecutive rows. Here’s an example that demonstrates how to use both LAG()
and LEAD()
functions:
Suppose we have a table named sales
with the following data representing daily sales for a product:
-- Create the sales table
CREATE TABLE sales (
Date DATE,
SalesAmount INT
);
-- Insert sample data into the sales table
INSERT INTO sales (Date, SalesAmount)
VALUES
('2023-09-01', 100),
('2023-09-02', 150),
('2023-09-03', 200),
('2023-09-04', 180),
('2023-09-05', 220);
| Date | SalesAmount |
|------------|-------------|
| 2023-09-01 | 100 |
| 2023-09-02 | 150 |
| 2023-09-03 | 200 |
| 2023-09-04 | 180 |
| 2023-09-05 | 220 |
We want to calculate the daily change in sales (the difference in sales from the previous day) and also see the projected sales for the next day. We can use the LAG()
and LEAD()
functions to achieve this:
SELECT
Date,
SalesAmount,
SalesAmount - LAG(SalesAmount) OVER (ORDER BY Date) AS DailyChange,
LEAD(SalesAmount) OVER (ORDER BY Date) AS ProjectedSales
FROM
sales;
In this SQL query:
- We select the
Date
andSalesAmount
columns from thesales
table. - We use the
LAG(SalesAmount)
function withOVER (ORDER BY Date)
to access the sales amount from the previous day based on the order of dates. - We subtract the previous day’s sales amount from the current day’s sales amount to calculate the
DailyChange
. - We use the
LEAD(SalesAmount)
function withOVER (ORDER BY Date)
to access the sales amount for the next day based on the order of dates.
The result of this query will provide the daily sales, the change in sales compared to the previous day, and the projected sales for the next day:
| Date | SalesAmount | DailyChange | ProjectedSales |
|------------|-------------|-------------|----------------|
| 2023-09-01 | 100 | NULL | 150 |
| 2023-09-02 | 150 | 50 | 200 |
| 2023-09-03 | 200 | 50 | 180 |
| 2023-09-04 | 180 | -20 | 220 |
| 2023-09-05 | 220 | 40 | NULL |
Other Types of Window Functions
- Percentile and Ranking Functions:
- PERCENT_RANK(): Calculates the relative rank of a row within the result set as a fraction.
- CUME_DIST(): Calculates the cumulative distribution of rows within the window frame.
- These functions are often used in statistical analysis and distribution calculations.
- First and Last Value Functions:
- FIRST_VALUE(): Retrieves the value of an expression from the first row in the window frame.
- LAST_VALUE(): Retrieves the value of an expression from the last row in the window frame.
- These functions are useful for finding the earliest and latest values within partitions.
- Ntile Function:
- NTILE(n): Divides the result set into ‘n’ roughly equal parts and assigns a group number to each row. It’s often used for quantile calculations.
- Example:
NTILE(4)
divides the data into quartiles.
- Running Total and Moving Average Functions:
- These functions, often used in financial and time-series analysis, calculate running totals and moving averages across a specified window frame.
- Examples:
SUM(sales) OVER(ORDER BY date ROWS BETWEEN UNBOUNDED PRECEDING AND CURRENT ROW)
- Advanced Window Frame Specifications:
- Window functions can have flexible frame specifications using clauses like
ROWS BETWEEN
,RANGE BETWEEN
, andGROUPS BETWEEN
. These allow you to define precisely which rows are included in the window frame.
- Window functions can have flexible frame specifications using clauses like
Conclusion: Window Functions in SQL
In this article, we explored “Window Functions in SQL” comprehensively:
Window Functions Overview:
- Window functions in SQL enable calculations across rows while preserving data granularity.
- They define a “window” of rows using the OVER() clause, allowing for partitioning and ordering.
Key Features:
- Window functions work without reducing row count.
- Data can be partitioned and ordered.
- Access to preceding and following rows is possible.
- Types include ranking, aggregation, lead/lag, percentile, and more.
Common Uses:
- Running totals, cumulative sums.
- Ranking rows.
- Moving averages and time-series analysis.
- Date range analysis.
- Finding first/last events.
- Percentiles and quartiles.
Types of Window Functions in SQL:
- Ranking Functions: RANK(), DENSE_RANK(), ROW_NUMBER()
- Aggregate Functions with OVER(): SUM(), AVG(), COUNT(), MIN(), MAX()
- Lead and Lag Functions: LEAD(), LAG()
- Percentile and Ranking Functions: PERCENT_RANK(), CUME_DIST()
- First and Last Value Functions: FIRST_VALUE(), LAST_VALUE()
- Ntile Function: NTILE(n)
- Running Total and Moving Average Functions
- Advanced Window Frame Specifications
Practical examples showcased ranking and aggregate functions with the OVER() clause for real-world applications. Window functions are essential for advanced data analysis in SQL.
Related Articles :