In this article, we will explore “npm” ie., Node Package Manager in great details. Let’s get started.
What is npm?
npm is the default package manager for Node.js. It makes it super easy to handle packages by taking care of the nitty-gritty tasks of getting packages installed, updated, and organized in Node.js projects. So, it’s basically the helper that keeps everything running smoothly, letting developers add, share, and use lots of different packages to make their projects work better.
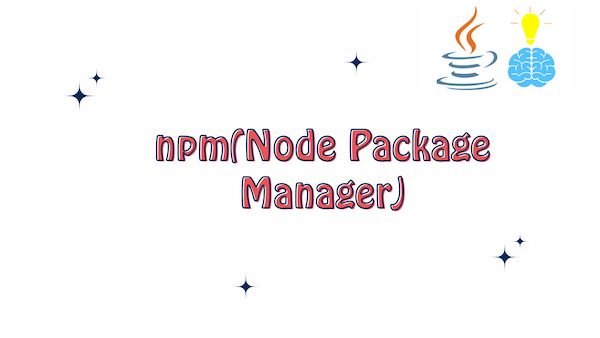
Key Features of npm
- Package Installation: npm is used to install packages (libraries or tools) globally or locally within a Node.js project.
- Dependency Management: It automatically manages project dependencies, ensuring that the correct versions are installed and avoiding version conflicts.
- Script Execution: npm allows developers to define and run scripts in the
package.json
file. Common scripts include start, test, and build. - Versioning: npm uses semantic versioning (SemVer) to specify package versions, making it easy to understand compatibility and update requirements.
- Registry: npm provides a centralized registry where developers can publish and discover open-source packages. The default registry is the npm public registry.
Usage of npm
Let’s look into the usage of npm.
Package Installation
Here’s a simple guide on how to install packages using npm with an example:
Install Node.js:
If you haven’t already, you need to install Node.js, which includes npm. You can download the installer from the official Node.js website.
Initialize a Node.js Project:
Open your terminal or command prompt, navigate to your project directory, and run the following command to initialize a new Node.js project. This will create a package.json
file.
npm init -y
Install a Package:
Use the npm install
command followed by the name of the package you want to install. For example, let’s install the lodash
library, a popular utility library:
npm install lodash
This command installs the latest version of lodash
and adds it as a dependency in your package.json
file.
Check package.json
:
Open your package.json
file, and you’ll see a new entry under “dependencies” for lodash
. It looks like this:
"dependencies": {
"lodash": "^4.17.21"
}
The ^
symbol indicates that your project can use versions of lodash
up to, but not including, the next major version.
Using the Installed Package:
Now that you’ve installed lodash
, you can use it in your JavaScript code. Create a file (e.g., app.js
) and import lodash
:
// app.js
const _ = require('lodash');
// Example usage
const numbers = [1, 2, 3, 4, 5];
const sum = _.sum(numbers);
console.log(`The sum of numbers is: ${sum}`);
Run Your Application:
If you created an app.js
file, you can run your application using:
node app.js
output
The sum of numbers is: 15
Installing Global Packages
Installing global packages using npm is useful for tools or libraries that you want to access from the command line across different projects. Here’s a simple guide with an example:
Install a Global Package:
Open your terminal or command prompt and run the following command to install a global package. In this example, we’ll install nodemon
, a tool that helps in automatically restarting Node.js applications:
npm install -g nodemon
The -g
flag stands for “global,” indicating that the package will be installed globally on your system.
Check Global Installation:
You can check the globally installed packages using the following command:
npm list -g --depth 0
This will display a list of globally installed packages without showing their dependencies. You should see nodemon
in the list.
├── corepack@0.20.0
├── nodemon@3.0.2
└── npm@10.1.0
Using the Global Package:
Since nodemon
is installed globally, you can use it from the command line to automatically restart your Node.js applications during development. For example, if you have a file named app.js
, you can run:
nodemon app.js
This will start your application, and nodemon
will monitor for changes. If you make edits to your code, it will automatically restart the application.
Note: Global packages are typically tools or utilities that you want to use across different projects. However, for project-specific dependencies, it’s recommended to install packages locally using npm install
.
Running Scripts
Running scripts using npm is a powerful feature that allows you to define and execute various tasks for your Node.js project. Here’s a guide with an example:
Add Scripts to package.json
:
Open your package.json
file in a text editor and add a “scripts” section. For example:
"scripts": {
"start": "node app.js",
"test": "echo \"No tests yet\"",
"lint": "eslint ."
}
In this example:
"start"
runs your application usingnode app.js
."test"
is a placeholder that echoes “No tests yet.”"lint"
runs ESLint for linting your code.
Install Dependencies:
If your scripts require additional dependencies, make sure to install them. For example, if you have a linting script using ESLint:
npm install eslint --save-dev
Run Scripts:
Use the npm run
command to execute your scripts. For instance:
To start your application:
npm run start
To run tests:
npm run test
To run code linting:
npm run lint
You can view all available scripts and their descriptions using:
npm run
Lifecycle scripts included in node-project@1.0.0:
start
node app.js
test
echo "No tests yet"
available via `npm run-script`:
lint
eslint .
Updating Packages
Updating packages using npm is a common task to ensure that your project dependencies are using the latest versions. Here’s a guide with examples:
Update Specific Package:
To update a specific package to its latest version, use the following command:
npm update <package-name>
Example: npm update lodash
Update All Packages:
To update all packages to their latest versions based on the version ranges specified in your package.json
, use:
npm update
Update Global Packages:
For globally installed packages, use the -g
flag:
npm update -g <package-name>
Example: npm update -g nodemon
Update DevDependencies:
In a Node.js project, the devDependencies
section in the package.json
file is used to specify dependencies that are needed only during the development and testing phases of a project, rather than in the production environment. These dependencies typically include tools, libraries, or frameworks that assist in tasks such as testing, linting, or building, but are not required for the actual execution of the application.
To specifically update packages listed under devDependencies
in your package.json
, use the --dev
flag:
npm update --dev
Update to the Latest Major Version:
To update a package to the latest major version, use the npm install
command with the -g
and -E
flags:
npm install -g -E nodemon
Update to a Specific Version:
To update a package to a specific version using npm, you can use the npm install
command with the @
symbol followed by the desired version.
npm install package-name@new-version
Example: npm install lodash@4.17.21
Publishing Packages
Publishing a package to the npm registry involves a series of steps. Here’s a simplified guide with an example:
Create a Package:
Create a directory for your package and add the necessary files, including at least a package.json
file. Ensure your package has a unique name.
// package.json
{
"name": "your-package-name",
"version": "1.0.0",
"description": "Description of your package",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": ["your", "keywords"],
"author": "Your Name",
"license": "MIT"
}
Login to npm:
If you haven’t logged in to npm from your terminal, use the following command:
npm login
Publish Your Package:
Run the following command to publish your package to the npm registry:
npm publish
This command will package your files, upload them to the npm registry, and make your package publicly accessible. Visit the npm website (https://www.npmjs.com/) and search for your package to verify that it has been published.
Removing Packages
Removing packages using npm is a straightforward process. Here are the steps and examples:
Removing a Local Package:
To remove a package from your local project
npm uninstall <package-name>
Example: npm uninstall lodash
Removing a Global Package:
To remove a globally installed package:
npm uninstall -g <package-name>
Example: npm uninstall -g nodemon
Removing a DevDependency:
If the package is listed under devDependencies
, use the --save-dev
flag:
npm uninstall --save-dev <package-name>
Example: npm uninstall --save-dev eslint
Removing a Specific Version:
If you want to remove a specific version of a package, you can specify the version number:
npm uninstall <package-name@specific-version>
Example: npm uninstall lodash@4.17.21
Removing Multiple Packages:
You can remove multiple packages at once:
npm uninstall <package1> <package2>
Removing Unused Packages:
To remove packages that are listed in your package.json
but are not used in your project:
npm prune
Differentiating between production and development dependencies
Differentiating between production and development dependencies in a Node.js project is crucial for maintaining a clean and efficient project structure. This distinction is typically made through the use of two key sections in the package.json
file: dependencies
and devDependencies
.
dependencies
:
- Purpose: These are dependencies that are required for the application to run in a production environment. They include libraries and modules that the application needs to execute its core functionality.
- Installation Command: Installed using
npm install
without the--save-dev
or-D
flags. - Example:
"dependencies": {
"express": "^4.17.1",
"lodash": "^4.17.21"
}
devDependencies
:
- Purpose: These are dependencies that are needed during the development and testing phases of the project. They include tools, testing frameworks, linters, and other utilities that aid development but are not required for the actual execution of the application in production.
- Installation Command: Installed using
npm install --save-dev
ornpm install -D
flags. - Example:
"devDependencies": {
"eslint": "^7.32.0",
"mocha": "^9.0.0"
}
Conclusion: npm(Node Package Manager)
This comprehensive exploration of npm, the Node Package Manager, has shed light on its pivotal role in Node.js development. npm serves as an indispensable tool for simplifying the management of packages, effortlessly handling tasks such as installation, updating, and organization within Node.js projects.
Key features of npm, including package installation, dependency management, script execution, versioning, and a centralized registry, contribute to its effectiveness in streamlining the development process. The article has provided practical insights into the usage of npm, covering package installation, global package installation, running scripts, updating packages, and the essential steps involved in publishing and removing packages.
Developers, whether installing local dependencies for project-specific needs or leveraging global packages for broader utility, have been guided through clear examples. The article also emphasizes the significance of distinguishing between production and development dependencies, maintaining a structured project layout for optimal efficiency.