In this article, we will explore the non in-built Popular Node.js Modules. Let’s get started.
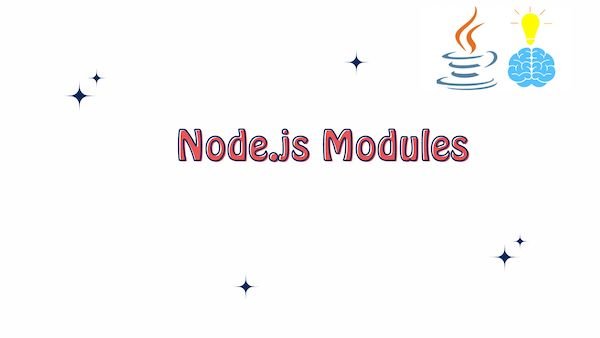
List of Popular Node.js Modules
Node.js, with its efficient and scalable runtime, has become a powerhouse in modern web development. One of its strengths lies in the extensive ecosystem of modules that simplify complex tasks, enhance functionality, and streamline development. Let’s delve into a selection of popular Node.js modules, exploring their use cases.
Express.js
Purpose: Web application framework for building robust and scalable web applications.
Example:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(3000, () => {
console.log('Express server listening on port 3000');
});
Socket.io
Purpose: Enables real-time, bidirectional communication between clients and servers.
Example:
const io = require('socket.io')(httpServer);
io.on('connection', (socket) => {
console.log('A user connected');
socket.emit('message', 'Welcome!');
});
Mongoose
Purpose: Elegant MongoDB object modeling for Node.js applications.
Example:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/test', { useNewUrlParser: true, useUnifiedTopology: true });
const Cat = mongoose.model('Cat', { name: String });
const kitty = new Cat({ name: 'Fluffy' });
kitty.save().then(() => console.log('Meow!'));
Axios
Purpose: A promise-based HTTP client for the browser and Node.js.
Example:
const axios = require('axios');
axios.get('https://jsonplaceholder.typicode.com/posts/1')
.then(response => console.log(response.data))
.catch(error => console.error(error));
Lodash
Purpose: Utility library for working with arrays, objects, and other data types.
Example:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const sum = _.sum(numbers);
console.log('Sum:', sum);
Joi
Purpose: A schema description language and data validator for JavaScript objects.
Example:
const Joi = require('joi');
const schema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
password: Joi.string().pattern(new RegExp('^[a-zA-Z0-9]{3,30}$')),
});
const result = schema.validate({ username: 'JohnDoe', password: 'password123' });
console.log(result);
Body-parser
Purpose: Middleware for handling HTTP POST requests in Express.js applications.
Example:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/api/users', (req, res) => {
const user = req.body;
// Process the user data
res.json(user);
});
Passport
Purpose: Authentication middleware for Node.js. Supports various authentication strategies.
Example:
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
passport.use(new LocalStrategy(
(username, password, done) => {
// Validate user credentials
return done(null, user);
}
));
Winston
Purpose: A versatile logging library for Node.js applications.
Example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'error.log', level: 'error' }),
],
});
logger.info('Hello, Winston!');
Nodemailer
Purpose: A module for sending emails with Node.js.
Example:
const nodemailer = require('nodemailer');
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'your.email@gmail.com',
pass: 'your_password',
},
});
const mailOptions = {
from: 'your.email@gmail.com',
to: 'recipient@example.com',
subject: 'Hello from Nodemailer',
text: 'This is a test email from Nodemailer.',
};
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.error(error);
} else {
console.log('Email sent: ' + info.response);
}
});
Conclusion: Popular Node.js Modules
In summary, this article explored key non-in-built Node.js modules that empower developers to build robust applications. Modules like Express.js, Socket.io, Mongoose, Axios, Lodash, Joi, Body-parser, Passport, Winston, and Nodemailer offer diverse functionalities, from web application frameworks to real-time communication, database modeling, and more. Each module was illustrated with concise examples, demonstrating their practical implementation. As the Node.js ecosystem continues to flourish, these modules play a vital role in enhancing development efficiency and fostering innovation.