In this article, we will explore various concepts associated with “Group By Clause” in SQL. Let’s get started.
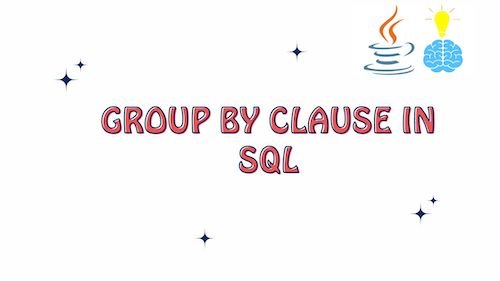
What is the use of GROUP BY Clause in SQL ?
The GROUP BY
clause in SQL is used to group rows from a database table based on the values in one or more columns. It is a fundamental SQL operation that allows you to aggregate data, perform calculations on grouped data, and obtain summary information from a dataset. The primary uses of the GROUP BY
clause in SQL are as follows:
- Data Aggregation: One of the main purposes of the
GROUP BY
clause is to aggregate data. It allows you to apply aggregate functions such asSUM
,COUNT
,AVG
,MAX
, andMIN
to calculate summary statistics for each group of rows. - Summarizing Data:
GROUP BY
is useful for summarizing large datasets into more manageable and meaningful information. - Categorizing Data: It is often used to categorize data into groups based on specific criteria or attributes. For instance, you can group customer orders by region, products by category, or employees by department, allowing you to see how data is distributed among different categories.
- Filtering Groups: You can use the
HAVING
clause in combination withGROUP BY
to filter groups of rows based on aggregate values. For example, you can retrieve only those product categories where the total sales exceed a certain threshold. - Data Analysis: The
GROUP BY
clause is essential for performing various data analysis tasks, such as calculating statistics, identifying trends, and generating reports or dashboards that present data in a structured and meaningful way. - Joining and Combining Data:
GROUP BY
can be used in conjunction withJOIN
operations to combine data from multiple tables and then group and aggregate the combined dataset. This is particularly useful when working with relational databases and complex data models.
Syntax of GROUP BY Clause in SQL
The syntax of the GROUP BY
clause in SQL is used to group rows from a database table based on the values in one or more columns. When using the GROUP BY
clause, you typically also use aggregate functions like SUM
, COUNT
, AVG
, MAX
, or MIN
to perform calculations on the grouped data. Here’s the basic syntax:
SELECT column1, column2, ..., aggregate_function(column)
FROM table_name
WHERE condition
GROUP BY column1, column2, ...;
Here’s an example of the GROUP BY
clause in action:
SELECT department, AVG(salary) as avg_salary
FROM employees
WHERE hire_date >= '2023-01-01'
GROUP BY department;
In this example:
- We want to retrieve the average salary for each department.
- We specify the
department
column in theGROUP BY
clause, indicating that we want to group the data by department. - We apply the
AVG
aggregate function to thesalary
column, calculating the average salary for each department. - We use the
WHERE
clause to filter employees hired after January 1, 2023, before applying theGROUP BY
operation.
Group By Clause with Aggregate Functions Examples
Here are various examples of using the GROUP BY
clause with various aggregate functions in SQL:
Example 1: Calculate Total Sales by Product Category
Suppose you have a table called sales
with information about individual sales transactions, including the product sold, quantity, and price. You want to calculate the total sales amount for each product category.
SELECT product_category, SUM(sales_amount) as total_sales
FROM sales
GROUP BY product_category;
In this example:
- We’re grouping the data by the
product_category
column. - The
SUM
aggregate function calculates the total sales amount for each product category. - The result will be a list of product categories and their corresponding total sales amounts.
Example 2: Count the Number of Orders by Customer
Suppose you have an orders
table with information about customer orders, including the customer’s name and order details. You want to count the number of orders placed by each customer.
SELECT customer_name, COUNT(order_id) as order_count
FROM orders
GROUP BY customer_name;
In this example:
- We’re grouping the data by the
customer_name
column. - The
COUNT
aggregate function calculates the number of orders for each customer. - The result will be a list of customer names and the count of orders each customer has placed.
Example 3: Find the Maximum and Minimum Salary by Department
Suppose you have an employees
table with information about employees, including their department and salary. You want to find the maximum and minimum salary for each department.
SELECT department, MAX(salary) as max_salary, MIN(salary) as min_salary
FROM employees
GROUP BY department;
In this example:
- We’re grouping the data by the
department
column. - The
MAX
aggregate function calculates the maximum salary for each department. - The
MIN
aggregate function calculates the minimum salary for each department. - The result will be a list of departments, along with their maximum and minimum salaries.
Example 4: Calculate Average Order Amount by Month
Suppose you have an orders
table with order information, including the order date and order amount. You want to calculate the average order amount for each month.
SELECT DATE_FORMAT(order_date, '%Y-%m') as month, AVG(order_amount) as avg_order_amount
FROM orders
GROUP BY month;
In this example:
- We’re grouping the data by the
month
portion of theorder_date
column using theDATE_FORMAT
function. - The
AVG
aggregate function calculates the average order amount for each month. - The result will be a list of months and their corresponding average order amounts.
Group by Multiple Columns Example
Grouping by multiple columns in SQL allows you to create more specific groups based on combinations of column values. Here’s an example of using the GROUP BY
clause with multiple columns:
Suppose you have a table called sales
with information about sales transactions, including the product sold, the year of the sale, and the sales amount. You want to calculate the total sales amount for each combination of product and year.
SELECT product, year, SUM(sales_amount) as total_sales
FROM sales
GROUP BY product, year;
In this example:
- We’re grouping the data by both the
product
andyear
columns. This means that rows with the same values in both columns will be grouped together. - The
SUM
aggregate function calculates the total sales amount for each combination of product and year. - The result will be a list of rows, each representing a unique combination of product and year, along with the total sales amount for that combination.
Group by with Having Clause for Filtering Groups Examples
The HAVING
clause is used in conjunction with the GROUP BY
clause to filter groups of rows based on aggregate values. It allows you to specify conditions that must be met by the grouped data. Here are some examples of using the GROUP BY
clause with the HAVING
clause for filtering groups:
Example 1: Find Departments with More Than 5 Employees
Suppose you have an employees
table with information about employees, including their department. You want to find departments that have more than 5 employees.
SELECT department, COUNT(*) as employee_count
FROM employees
GROUP BY department
HAVING COUNT(*) > 5;
In this example:
- We’re grouping the data by the
department
column. - The
COUNT
aggregate function calculates the number of employees in each department. - The
HAVING
clause filters the groups to include only those with more than 5 employees. - The result will be a list of departments and their employee counts, but only for departments with more than 5 employees.
Example 2: Identify Customers with More Than $1,000 in Total Purchases
Suppose you have a customers
table with customer information and an orders
table with order information, including the order amount. You want to identify customers who have made purchases totaling more than $1,000.
SELECT c.customer_id, c.customer_name, SUM(o.order_amount) as total_purchases
FROM customers c
JOIN orders o ON c.customer_id = o.customer_id
GROUP BY c.customer_id, c.customer_name
HAVING SUM(o.order_amount) > 1000;
In this example:
- We’re joining the
customers
table with theorders
table to link customers to their orders. - We’re grouping the data by
customer_id
andcustomer_name
to get a summary for each customer. - The
SUM
aggregate function calculates the total purchases for each customer. - The
HAVING
clause filters the groups to include only customers with total purchases exceeding $1,000. - The result will be a list of customers who meet the criteria, along with their total purchase amounts.
Example 3: Find Products with More Than 50 Units in Stock
Suppose you have a products
table with information about products, including their stock quantities. You want to find products that have more than 50 units in stock.
SELECT product_id, product_name, SUM(stock_quantity) as total_stock
FROM products
GROUP BY product_id, product_name
HAVING SUM(stock_quantity) > 50;
In this example:
- We’re grouping the data by
product_id
andproduct_name
to get a summary for each product. - The
SUM
aggregate function calculates the total stock quantity for each product. - The
HAVING
clause filters the groups to include only products with a total stock quantity exceeding 50. - The result will be a list of products that meet the criteria, along with their total stock quantities.
GROUP BY with Joining Tables
Suppose you have two tables, orders
and order_details
. The orders
table contains order information, and the order_details
table contains details about each product within an order. You want to find the total quantity of each product sold across all orders.
SELECT od.product_id, p.product_name, SUM(od.quantity) as total_quantity
FROM order_details od
JOIN products p ON od.product_id = p.product_id
GROUP BY od.product_id, p.product_name;
In this example:
- We’re joining the
order_details
table with theproducts
table using theproduct_id
column as the join key. - We’re grouping the data by both
od.product_id
andp.product_name
to get a summary for each product. - The
SUM
aggregate function calculates the total quantity sold for each product across all orders. - The result will be a list of products, along with their total quantities sold.
Best Practices for using Group By Clause in SQL
Using the GROUP BY
clause effectively in SQL is crucial for aggregating data and deriving meaningful insights from your database. Here are some best practices for using the GROUP BY
clause:
- Choose the Right Columns: Select the appropriate columns for grouping. The columns you choose should represent the criteria for forming groups in a meaningful way. Avoid grouping by irrelevant columns that do not provide valuable insights.
- Use Aggregate Functions Wisely: When applying aggregate functions (e.g.,
SUM
,COUNT
,AVG
,MAX
,MIN
), consider what you want to measure. Choose the right aggregate function that best suits your analysis goals. - Be Mindful of the HAVING Clause: If you need to filter groups based on aggregate values, use the
HAVING
clause. This is especially useful when you want to include or exclude groups based on specific conditions after theGROUP BY
operation. - Optimize Your Queries:
GROUP BY
queries can be resource-intensive, especially on large datasets. Use indexing, query optimization techniques, and database-specific features (e.g., materialized views) to improve query performance. - Test Queries Thoroughly: Always test your
GROUP BY
queries on a subset of your data or in a development/staging environment before running them on production data. Ensure that the results match your expectations. - Consider NULL Values: Be aware of how NULL values are handled in your
GROUP BY
queries. Depending on your database system, NULL values might affect grouping and aggregation differently. - Avoid Overusing Subqueries: While subqueries can be powerful, excessive use of subqueries in
GROUP BY
queries can lead to performance issues. Whenever possible, consider using joins or common table expressions (CTEs) instead. - Keep SQL Injection in Mind: If your
GROUP BY
queries involve user input or dynamic values, use parameterized queries or prepared statements to prevent SQL injection.
Conclusion: GROUP BY Clause in SQL
In this article, we delved into the fundamental concepts associated with the “GROUP BY Clause in SQL”. We explored the versatile uses of this clause, which serves as a powerful tool for grouping rows based on specific columns and performing aggregations. The key takeaways from this article include:
- Purpose of GROUP BY: The GROUP BY clause in SQL is instrumental in aggregating data, performing calculations on grouped data, and obtaining summary information from datasets. It allows us to organize data into meaningful groups for analysis.
- Primary Uses:
- Data Aggregation: GROUP BY facilitates the application of aggregate functions like SUM, COUNT, AVG, MAX, and MIN to calculate summary statistics.
- Summarizing Data: It condenses large datasets into manageable and meaningful summaries, aiding analysis.
- Categorizing Data: GROUP BY categorizes data into groups based on specific criteria or attributes.
- Filtering Groups: The HAVING clause filters groups based on aggregate values.
- Data Analysis: It’s indispensable for calculating statistics, identifying trends, and generating structured reports or dashboards.
- Joining and Combining Data: GROUP BY can be used with JOIN operations to combine data from multiple tables before grouping and aggregating.
- Syntax: We examined the syntax of the GROUP BY clause in SQL and its usage, often accompanied by aggregate functions and a WHERE clause to filter data before grouping.
- Examples: The article provided various SQL examples to illustrate the practical applications of GROUP BY, including calculating total sales by product category, counting orders by customer, finding maximum and minimum salaries by department, and calculating average order amounts by month.
- Grouping by Multiple Columns: We explored the ability to group by multiple columns, which allows for more granular data summarization based on combinations of column values.
- Using the HAVING Clause for Filtering: The HAVING clause, in conjunction with GROUP BY, was demonstrated for filtering groups based on aggregate values, enabling us to extract specific insights from grouped data.
- Best Practices: To conclude, the article highlighted best practices for effective usage of the GROUP BY clause, such as selecting the right columns, using aggregate functions judiciously, optimizing queries, testing thoroughly, considering NULL values, avoiding overuse of subqueries, and safeguarding against SQL injection.
Related Articles :