In this article, we will look into the concept of “Having clause in SQL“. Let’s get started.
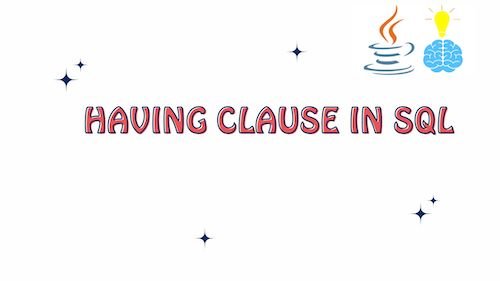
What is the use of Having Clause in SQL ?
The HAVING
clause in SQL is used to filter the results of a GROUP BY
operation based on the results of aggregate functions applied to grouped data. In other words, it allows you to specify conditions for selecting groups of rows after they have been grouped using the GROUP BY
clause and after aggregate functions have been applied to those groups.
Here’s a breakdown of the key uses and purposes of the HAVING
clause in SQL:
- Filtering Grouped Data: The primary use of the
HAVING
clause is to filter groups of rows that meet specific criteria based on the results of aggregate functions. This is particularly useful when you want to include or exclude groups based on aggregated values. - Aggregation and Comparison: You can use the
HAVING
clause to compare the results of aggregate functions (e.g.,SUM
,COUNT
,AVG
,MAX
,MIN
) to specific values or conditions. For example, you can filter groups where the sum of values is greater than a certain threshold. - Post-Grouping Filtering: Unlike the
WHERE
clause, which filters rows before grouping, theHAVING
clause filters groups after grouping and aggregation. This allows you to work with summarized data and apply conditions to the result of the grouping and aggregation. - Working with Summarized Data: The
HAVING
clause is essential when working with summarized or aggregated data, such as calculating statistics, identifying trends, or generating reports that present data in a structured and meaningful way. - Complex Queries: It is commonly used in complex queries that involve multiple tables,
JOIN
operations, and groupings, where you need to filter groups based on the results of aggregate calculations.
Syntax of Having Clause in SQL
The HAVING
clause in SQL is used to filter the results of a GROUP BY
operation based on the results of aggregate functions applied to grouped data. Here is the syntax of the HAVING
clause:
SELECT column1, column2, ..., aggregate_function(column)
FROM table_name
GROUP BY column1, column2, ...
HAVING condition;
Here’s a simple example to illustrate the syntax of the HAVING
clause:
Suppose you have a table named sales
with columns product_category
and total_sales
, and you want to find product categories where the total sales exceed $10,000.
SELECT product_category, SUM(total_sales) as category_sales
FROM sales
GROUP BY product_category
HAVING SUM(total_sales) > 10000;
Having vs Where Clause in SQL
The HAVING
clause and the WHERE
clause in SQL serve different purposes, even though they are both used to filter data in SQL queries. Understanding their distinctions is crucial for writing accurate and effective SQL queries. Here are the key differences between the two:
1. Use Case:
- WHERE Clause: The
WHERE
clause is used to filter rows from a table before any grouping or aggregation takes place. It operates on individual rows in the original table. You use it to specify conditions that individual rows must meet to be included in the result set. - HAVING Clause: The
HAVING
clause, on the other hand, is used to filter groups of rows that result from aGROUP BY
operation. It operates on the result of the grouping and aggregation and is used to filter groups based on aggregate values or calculations.
2. Application:
- WHERE Clause: It is typically used with non-aggregated columns and is applied before grouping or aggregation. You can use it to filter rows based on conditions involving individual column values.
- HAVING Clause: It is applied after grouping and aggregation and is used with aggregate functions. You use it to filter groups based on the results of aggregate calculations.
3. Aggregation:
- WHERE Clause: It does not work with aggregate functions. It filters individual rows based on conditions applied to non-aggregated columns.
- HAVING Clause: It works with aggregate functions (e.g.,
SUM
,COUNT
,AVG
,MAX
,MIN
) and filters groups based on the results of these aggregate functions.
4. Placement:
- WHERE Clause: Appears before the
GROUP BY
clause in a query. - HAVING Clause: Appears after the
GROUP BY
clause and any aggregate functions in a query.
5. Example:
Consider a scenario where you have a sales table with columns product_category
and total_sales
, and you want to find product categories where the total sales exceed $10,000.
- Using WHERE:
SELECT product_category, SUM(total_sales) as category_sales
FROM sales
WHERE total_sales > 10000
GROUP BY product_category;
- Using HAVING:
SELECT product_category, SUM(total_sales) as category_sales
FROM sales
GROUP BY product_category
HAVING SUM(total_sales) > 10000;
In the WHERE
clause example, rows with individual total_sales
values greater than $10,000 are filtered before grouping. In the HAVING
clause example, the SUM(total_sales)
is calculated for each product category group, and then the groups where the sum exceeds $10,000 are selected.
Use Cases of Having Clause in SQL
The HAVING
clause in SQL is primarily used in combination with the GROUP BY
clause to filter groups of rows based on the results of aggregate functions. Its main use cases include:
Filtering Groups by Aggregate Results: The most common use of the HAVING
clause is to filter groups based on the outcome of aggregate functions such as SUM
, COUNT
, AVG
, MAX
, and MIN
. This is useful when you want to include or exclude groups of data based on specific criteria derived from summarized or grouped data.
SELECT department, AVG(salary) as avg_salary
FROM employees
GROUP BY department
HAVING AVG(salary) > 50000;
In this example, the HAVING
clause filters out departments with an average salary less than $50,000.
Applying Conditions to Grouped Data: You can use the HAVING
clause to apply conditions to grouped data that are not straightforward to express in a WHERE
clause because they involve aggregate functions. For instance, finding groups with a certain percentage of a total or groups with a specific range of aggregated values.
SELECT product_category, SUM(total_sales) as category_sales
FROM sales
GROUP BY product_category
HAVING SUM(total_sales) > 10000 AND SUM(total_sales) < 50000;
In this example, the HAVING
clause filters product categories with total sales between $10,000 and $50,000.
Filtering by Count or Frequency: You can use the HAVING
clause to filter groups based on the count or frequency of occurrences within each group. For instance, finding groups with more than a certain number of records.
SELECT customer_id, COUNT(order_id) as order_count
FROM orders
GROUP BY customer_id
HAVING COUNT(order_id) >= 5;
In this example, the HAVING
clause selects customers who have placed at least 5 orders.
Filtering by Calculated Values: The HAVING
clause allows you to filter groups based on calculations that involve multiple aggregate functions or columns. This is useful for complex conditions that require evaluating the group as a whole.
SELECT product_category, AVG(sales_amount / order_count) as avg_amount_per_order
FROM sales
GROUP BY product_category
HAVING AVG(sales_amount / order_count) > 50;
In this example, the HAVING
clause filters product categories with an average amount per order exceeding $50.
Working with Data Trends: When dealing with time-series data or data with temporal aspects, the HAVING
clause can be used to identify trends or patterns. For example, finding months where sales have increased by a certain percentage compared to the previous month.
SELECT MONTH(order_date), SUM(order_amount) as monthly_sales
FROM orders
GROUP BY MONTH(order_date)
HAVING (monthly_sales - LAG(monthly_sales, 1, 0) / NULLIF(LAG(monthly_sales, 1, 0), 0)) > 0.1;
In this example, the HAVING
clause filters months where sales have increased by more than 10% compared to the previous month.
Best Practices for Using the Having Clause in SQL
Using the HAVING
clause in SQL effectively is crucial for filtering and extracting meaningful insights from grouped and aggregated data. Here are some best practices for using the HAVING
clause:
- Use with GROUP BY: The
HAVING
clause is most commonly used in conjunction with theGROUP BY
clause. Ensure that your query includes a validGROUP BY
clause before applying theHAVING
clause. - Choose the Right Aggregates: Select the appropriate aggregate functions (e.g.,
SUM
,COUNT
,AVG
,MAX
,MIN
) based on what you want to measure or filter. Consider the nature of your data and analysis goals. - Keep It Simple: Write clear and straightforward conditions in the
HAVING
clause. Complex conditions may be difficult to maintain and understand. If your condition involves multiple criteria, break it down into separate clauses. - Optimize Query Performance: Grouping and aggregating large datasets can be resource-intensive. Optimize your query’s performance by using indexing, appropriate join strategies, and other database-specific optimization techniques.
- Consider NULL Values: Be aware of how NULL values are handled in your
HAVING
clause conditions. Depending on your database system, NULL values might affect the results differently. Use functions likeCOALESCE
orIFNULL
to handle NULL values as needed. - Avoid Subqueries if Possible: While subqueries can be used in the
HAVING
clause, excessive use of subqueries can lead to performance issues. Whenever possible, consider using joins or common table expressions (CTEs) instead.
Conclusion: Having Clause in SQL
In conclusion, the “HAVING” clause in SQL is a powerful tool for filtering and extracting meaningful insights from grouped and aggregated data. It allows you to apply conditions to groups of rows after they have been grouped using the “GROUP BY” clause and after aggregate functions have been applied to those groups. Some key takeaways from this article include:
- The primary use of the “HAVING” clause is to filter groups of rows based on the results of aggregate functions, making it particularly useful for including or excluding groups based on aggregated values.
- It enables you to compare the results of aggregate functions (e.g., SUM, COUNT, AVG, MAX, MIN) to specific values or conditions, helping you extract relevant data from summarized or grouped data.
- Unlike the “WHERE” clause, which filters rows before grouping, the “HAVING” clause filters groups after grouping and aggregation, allowing you to work with summarized data effectively.
- The “HAVING” clause is essential for complex queries involving multiple tables, JOIN operations, and groupings, where you need to filter groups based on the results of aggregate calculations.
To use the “HAVING” clause effectively, it’s essential to understand its syntax, how it differs from the “WHERE” clause, and its various use cases. Additionally, following best practices, such as choosing the right aggregates, optimizing query performance, and keeping conditions simple, will help you write accurate and efficient SQL queries when working with grouped and aggregated data.
Related Articles :