In this article, we’ll explore the syntax, nuances of column definitions, and the importance of primary keys and constraints in the context of “Create Table in SQL.” Through real-world examples, you’ll not only grasp the fundamentals but also learn how to create tables tailored to your specific data storage needs, from straightforward tables to intricate ones complete with foreign keys and default values.
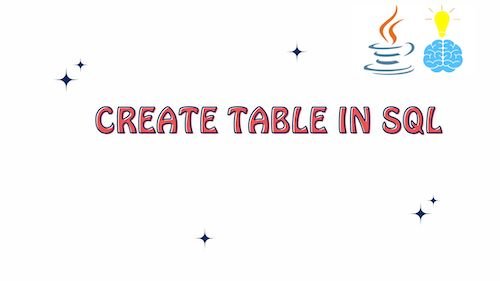
Basic Syntax: Create Table in SQL
The basic syntax for creating tables in SQL is done using the CREATE TABLE statement. Here is the fundamental structure of this SQL statement:
CREATE TABLE table_name (
column1 datatype,
column2 datatype,
column3 datatype,
...
);
Let’s break down the components of this syntax:
CREATE TABLE
: This is the SQL command that indicates you want to create a new table.table_name
: Replace this with the name you want to give to your table.( ... )
: Inside the parentheses, you define the columns that will be part of your table.column1
,column2
,column3
, …: These are the names of the columns in your table.datatype
: For each column, specify the data type that defines what kind of data the column can hold (e.g., INTEGER, VARCHAR, DATE).
Here’s an example of creating a simple table called “employees” with three columns: “employee_id,” “first_name,” and “last_name,” along with their data types:
CREATE TABLE employees (
employee_id INT,
first_name VARCHAR(50),
last_name VARCHAR(50)
);
In this example:
CREATE TABLE
is the SQL command to create a table.employees
is the name of the table.- Inside the parentheses, we have three columns:
employee_id
with a data type ofINT
(integer).first_name
with a data type ofVARCHAR(50)
(variable-length character string with a maximum length of 50 characters).last_name
with the same data type asfirst_name
.
CREATE TABLE IF NOT EXISTS
The CREATE TABLE IF NOT EXISTS
statement in SQL is used to create a new table in a database only if the table does not already exist. This statement is particularly useful when you want to ensure that a table is created, but you don’t want to encounter an error if the table has already been defined.
Here is the basic syntax for CREATE TABLE IF NOT EXISTS
:
CREATE TABLE IF NOT EXISTS table_name (
column1 datatype,
column2 datatype,
...
);
Here’s an example of using CREATE TABLE IF NOT EXISTS
:
CREATE TABLE IF NOT EXISTS employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
hire_date DATE
);
In this example, a table named “employees” will be created with columns for employee information (employee_id, first_name, last_name, hire_date) if it doesn’t already exist. If the table “employees” already exists, this statement will have no effect and won’t raise an error.
Creating Table with Primary Keys
To create a table with a primary key in SQL, you can use the CREATE TABLE
statement along with the PRIMARY KEY
constraint. A primary key uniquely identifies each row in a table. Here’s the syntax:
CREATE TABLE table_name (
column1 datatype PRIMARY KEY,
column2 datatype,
column3 datatype,
...
);
You can also create composite primary keys, which consist of multiple columns. To do this, simply list the columns within the parentheses that follow the PRIMARY KEY
constraint. Here’s an example of creating a table called “students” with a composite primary key consisting of two columns: “student_id” and “course_id”:
CREATE TABLE students (
student_id INT,
course_id INT,
course_grade CHAR(1),
PRIMARY KEY (student_id, course_id)
);
In this example:
- We create a table named “students.”
- It has three columns: “student_id,” “course_id,” and “course_grade.”
- The primary key is defined as a combination of both “student_id” and “course_id” using the
PRIMARY KEY
constraint. - This means that each combination of “student_id” and “course_id” must be unique within the table.
Create Table in SQL with different Constraints
When creating a table in SQL, you can apply various constraints to define rules and restrictions on the data that can be stored in the table. Here are some common constraints that you can use while creating a table:
Primary Key Constraint: Ensures that each row in the table has a unique identifier. It also enforces that the primary key column cannot contain NULL values.
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50)
);
Unique Constraint: Enforces that values in a column (or a set of columns) are unique across all rows in the table, but unlike a primary key, it allows NULL values.
CREATE TABLE products (
product_id INT UNIQUE,
product_name VARCHAR(100) UNIQUE,
price DECIMAL(10, 2)
);
Check Constraint: Defines a condition that values in a column must satisfy when inserting or updating data.
CREATE TABLE orders (
order_id INT,
order_date DATE,
order_status VARCHAR(20) CHECK (order_status IN ('Processing', 'Shipped', 'Delivered'))
);
Foreign Key Constraint: Establishes a link between two tables, where the values in one column (usually the primary key) in the current table must exist in another table.
CREATE TABLE order_details (
order_detail_id INT PRIMARY KEY,
order_id INT,
product_id INT,
quantity INT,
FOREIGN KEY (order_id) REFERENCES orders(order_id),
FOREIGN KEY (product_id) REFERENCES products(product_id)
);
Default Constraint: Sets a default value for a column if no value is provided during insertion.
CREATE TABLE students (
student_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
enrollment_year INT DEFAULT 2023
);
Not Null Constraint: Ensures that a column must have a value (it cannot be NULL) during insertion.
CREATE TABLE customers (
customer_id INT PRIMARY KEY,
first_name VARCHAR(50) NOT NULL,
last_name VARCHAR(50) NOT NULL,
email VARCHAR(100)
);
Create Table in SQL with Default Values for Columns
In SQL, you can set default values for columns when creating a table using the DEFAULT
constraint. Default values are used when you insert a new row into the table and do not provide a value for the column with the default constraint. Here’s the syntax to set default values while creating a table:
CREATE TABLE table_name (
column1 datatype DEFAULT default_value,
column2 datatype DEFAULT default_value,
column3 datatype DEFAULT default_value,
...
);
Here’s an example of creating a “users” table with default values for columns:
CREATE TABLE users (
user_id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) DEFAULT 'user@example.com',
registration_date DATE DEFAULT CURRENT_DATE
);
The “email” column has a default value of ‘user@example.com,’ which will be used if no email is provided during insertion.
Creating Temporary Table in SQL
Creating Temporary Tables in SQL allows you to store and manipulate data temporarily within a session or transaction. Temporary tables are useful for holding intermediate results, performing complex calculations, or breaking down complex queries into simpler parts. Here’s how you can create temporary tables in SQL:
-- Create a temporary table with a unique name within the session
CREATE TEMPORARY TABLE temp_table_name (
column1 datatype1,
column2 datatype2,
-- Add more columns as needed
);
-- Insert data into the temporary table
INSERT INTO temp_table_name (column1, column2)
VALUES (value1, value2),
(value3, value4),
-- Insert more rows as needed
;
-- Query the temporary table
SELECT * FROM temp_table_name;
-- Drop the temporary table when it is no longer needed
DROP TEMPORARY TABLE temp_table_name;
Key points to note:
- Temporary tables are session-specific, meaning they exist only for the duration of the session in which they are created. They are automatically dropped when the session ends.
- You can use temporary tables to store intermediate results, perform complex joins or calculations, or simplify complex queries by breaking them into smaller parts.
- Temporary tables can be helpful when working with large datasets or when you need to isolate data within a session.
- Temporary tables follow similar syntax to regular tables in SQL, but they are created with the
CREATE TEMPORARY TABLE
statement. - You can insert, update, and delete data in temporary tables just like regular tables.
- Don’t forget to drop temporary tables when you are done using them to free up resources.
Create Table in SQL from Existing Table
Creating tables from existing tables is a common task in SQL, and it’s often done to create copies of tables, apply transformations, or filter data for specific purposes. The SQL statement used for this operation is typically referred to as a “CREATE TABLE AS” statement or a “SELECT INTO” statement. Here’s an example of how to create a new table from an existing table:
Suppose you have an existing table called “employees” with the following structure:
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
department VARCHAR(50),
salary DECIMAL(10, 2)
);
Now, let’s say you want to create a new table called “high_paid_employees” that contains only the employees with a salary greater than $60,000. You can achieve this by using the following SQL statement:
CREATE TABLE high_paid_employees AS
SELECT * FROM employees
WHERE salary > 60000;
In this example:
- We use the “CREATE TABLE” statement to create a new table called “high_paid_employees.”
- The “AS” keyword indicates that we are creating the new table as the result of a query.
- The “SELECT * FROM employees” query selects all columns and rows from the existing “employees” table.
- The “WHERE” clause filters the rows to include only those where the “salary” column is greater than $60,000.
After executing this SQL statement, a new table called “high_paid_employees” will be created, containing only the employees who meet the salary condition. The structure of the new table will be the same as the “employees” table.
Modifying Existing Tables
Modifying existing tables in SQL is a crucial aspect of database management. You can alter tables by adding, modifying, or dropping columns, changing data types, and more. Here are multiple examples of how to modify existing tables in SQL:
- Adding a New Column:
To add a new column to an existing table, you can use the ALTER TABLE statement. For example, let’s add an “email” column to a “customers” table:
ALTER TABLE customers
ADD email VARCHAR(100);
- Modifying Column Data Type:
You can change the data type of an existing column. For instance, if you want to change the “age” column from INT to SMALLINT:
ALTER TABLE employees
ALTER COLUMN age TYPE SMALLINT;
- Renaming a Column:
To rename an existing column, use the ALTER TABLE statement along with the RENAME COLUMN clause:
ALTER TABLE products
RENAME COLUMN product_name TO name;
- Dropping a Column:
If you want to remove a column from a table, you can use the ALTER TABLE statement with the DROP COLUMN clause. Here, we remove the “phone” column from the “contacts” table:
ALTER TABLE contacts
DROP COLUMN phone;
- Adding a Primary Key:
To add a primary key constraint to an existing table, you can use the ALTER TABLE statement with the ADD CONSTRAINT clause:
ALTER TABLE orders
ADD CONSTRAINT pk_orders PRIMARY KEY (order_id);
- Adding a Foreign Key:
You can add a foreign key constraint to link two tables. For example, let’s link the “orders” table to the “customers” table using the “customer_id” column:
ALTER TABLE orders
ADD CONSTRAINT fk_customer_id
FOREIGN KEY (customer_id)
REFERENCES customers (customer_id);
- Adding an Index:
To improve query performance, you can add an index to one or more columns of a table:
CREATE INDEX idx_last_name
ON employees (last_name);
- Adding a Check Constraint:
Check constraints ensure that values meet specific criteria. Here, we add a check constraint to ensure that ages are greater than 18:
ALTER TABLE employees
ADD CONSTRAINT chk_age CHECK (age > 18);
- Adding a Unique Constraint:
Unique constraints ensure that values in a column are unique. Let’s add a unique constraint to the “email” column:
ALTER TABLE customers
ADD CONSTRAINT uniq_email UNIQUE (email);
- Setting a Default Value:
You can set a default value for a column to be used when no value is specified during insertion:
ALTER TABLE employees
ALTER COLUMN hire_date SET DEFAULT '2023-01-01';
Dropping Tables
The DROP TABLE statement is used to permanently remove an existing table, including all its data, indexes, constraints, and associated objects. The syntax for dropping a table is as follows:
DROP TABLE table_name;
Example:
DROP TABLE employees;
Best Practices for Creating and Managing Tables
Creating and managing tables in a relational database is a critical task that impacts the overall performance and efficiency of your database system. Here are some best practices for creating and managing tables effectively:
1. Use Descriptive Table Names: Choose meaningful and descriptive names for your tables. Avoid using vague or abbreviated names that can make it difficult to understand the table’s purpose.
2. Define Primary Keys: Each table should have a primary key that uniquely identifies each record. This key can be a single column or a combination of columns. Primary keys are crucial for data integrity and efficient querying.
3. Use Indexes Wisely: Indexes help speed up query performance, but they come at a cost in terms of storage and maintenance. Use indexes on columns frequently used in search conditions, joins, and sorting. Be mindful of the trade-off between query performance and storage.
4. Consider Constraints: Apply constraints like UNIQUE, NOT NULL, and CHECK to ensure data consistency and accuracy. Constraints help enforce data integrity rules and prevent invalid data from being inserted into tables.
5. Define Relationships: Use foreign keys to define relationships between tables. Properly defined relationships maintain referential integrity and make it easier to navigate and query related data.
6. Normalize Your Data: Normalize your database to reduce data redundancy and improve data integrity. Follow standard normalization rules (e.g., 1NF, 2NF, 3NF) to organize data efficiently.
7. Use Appropriate Data Types: Choose the right data types for your columns to optimize storage and query performance. Use integers for whole numbers, strings for text, and appropriate date/time data types for temporal data.
8. Avoid Storing Computed Values: Avoid storing computed values that can be derived from existing data. Instead, calculate these values on-the-fly in queries. Storing computed values can lead to data inconsistencies.
9. Consider Security: Implement appropriate access controls and security measures to protect your data. Limit access to tables based on user roles and privileges.
Conclusion: Create Table in SQL
Creating and managing tables in SQL is a fundamental aspect of database design and maintenance. Understanding the basic syntax for creating tables, defining primary keys, and applying constraints is essential for ensuring data integrity and efficient querying.
Furthermore, the ability to modify existing tables, create temporary tables, and generate new tables from existing data provides flexibility and scalability in database management.
Adhering to best practices, such as using descriptive table names, defining relationships, and optimizing data types, is crucial for creating well-structured and efficient databases. Additionally, maintaining proper security measures and access controls helps safeguard sensitive data and prevent unauthorized access.
By following these practices and continuously monitoring and optimizing your tables, you can create a robust and reliable database system that meets your specific data storage and retrieval needs.
Related Articles :