This article digs into the complexities of the ArrayIndexOutOfBoundsException in Java, explaining why it arises, the scenarios that cause it, and the tactics for efficiently handling and preventing it.
Introduction
Arrays are essential in programming because they allow the storage of numerous elements under one name. However, incorrect use can lead to runtime issues, such as the infamous “ArrayIndexOutOfBoundsException.”
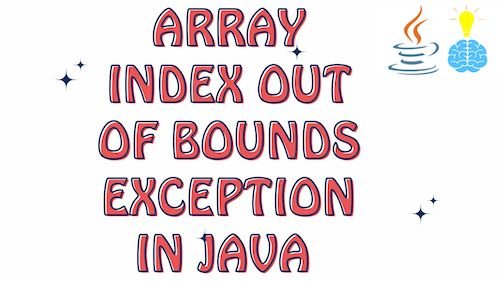
Causes of ArrayIndexOutOfBoundsException in Java
Negative Index Access
Attempting to access an array element with a negative index will throw ArrayIndexOutOfBoundsException in Java.
int[] array = {1, 2, 3};
int element = array[-1]; // Causes ArrayIndexOutOfBoundsException
Index Out of Bounds
Accessing an index that exceeds or equals the array’s length will throw ArrayIndexOutOfBoundsException in Java.
int[] array = {1, 2, 3};
int element = array[3]; // Causes ArrayIndexOutOfBoundsException
Loop Boundaries Mismatch:
Incorrect loop conditions leading to accessing elements beyond array bounds.
int[] array = {1, 2, 3};
for (int i = 0; i <= array.length; i++) {
// Accessing array[i] when i is equal to array.length causes ArrayIndexOutOfBoundsException
}
Handling ArrayIndexOutOfBoundsException in Java
Handling the ArrayIndexOutOfBoundsException
in Java is essential to prevent crashes and ensure a smooth user experience. By using a try-catch
block, you can gracefully manage this exception and provide meaningful feedback to users. Here’s how to do it with a code example:
public class ArrayIndexHandlingExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30};
try {
int index = 3; // Change index to see different outcomes
int value = numbers[index];
System.out.println("Value at index " + index + ": " + value);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Array index out of bounds!");
}
}
}
In this example, the try
block attempts to access an element at index 3
in the numbers
array. If the index is within the valid range (0 to length – 1), the value is printed. However, if the index is out of bounds, the catch
block catches the ArrayIndexOutOfBoundsException
and displays an error message. This prevents the program from crashing and informs the user about the issue.
Preventing ArrayIndexOutOfBoundsException in Java
To prevent ArrayIndexOutOfBoundsException
, it’s crucial to validate array indices before accessing elements. By checking if the index is within the valid range, you can avoid errors. Here’s a code example demonstrating this:
public class ArrayIndexPreventionExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30};
int indexToAccess = 2; // Change index to see different outcomes
if (indexToAccess >= 0 && indexToAccess < numbers.length) {
int value = numbers[indexToAccess];
System.out.println("Value at index " + indexToAccess + ": " + value);
} else {
System.out.println("Invalid index!");
}
}
}
In this example, before accessing an array element at indexToAccess
, the program checks if the index is within the valid range of 0
to numbers.length - 1
. If the index is valid, the element is accessed and its value is printed. If the index is not valid, an “Invalid index!” message is displayed. This preventive approach ensures that only valid indices are used to access array elements, reducing the risk of ArrayIndexOutOfBoundsException
.
Best Practices
- Validate Indices: Always validate array indices before accessing elements. Check if the index is within the range of
0
tolength - 1
before performing any operations. - Use Loop Boundaries Carefully: When using loops to iterate through arrays, ensure that the loop boundaries are set correctly to avoid accessing elements beyond the valid range.
- Consider
for-each
Loop: When iterating through arrays, consider using the enhancedfor-each
loop (for (type element : array)
) as it automatically manages indices and helps prevent off-by-one errors. - Immutable Arrays: If possible, consider using immutable collections (like
List
implementations) that handle dynamic resizing and index management internally, reducing the risk of index-related errors. - Exception Handling: When handling
ArrayIndexOutOfBoundsException
, provide meaningful error messages to users. These messages can aid debugging and provide users with actionable information.
FAQs:
1. What is ArrayIndexOutOfBoundsException in Java?
ArrayIndexOutOfBoundsException is a runtime exception in Java that occurs when trying to access an array element with an index that is either negative or beyond the valid range of indices for the array.
2. What causes ArrayIndexOutOfBoundsException?
This exception occurs when:
- Accessing an array element with a negative index.
- Accessing an array element with an index greater than or equal to the array’s length.
- Iterating through an array with incorrect loop boundaries.
3. How can I handle ArrayIndexOutOfBoundsException?
Handle it using a try-catch
block. Catch the exception and provide appropriate error-handling mechanisms, such as displaying user-friendly messages or implementing fallback strategies.
4. How can I prevent ArrayIndexOutOfBoundsException?
Prevent it by:
- Validating indices before accessing array elements.
- Using loop boundaries that stay within the valid index range.
- Using enhanced
for-each
loops for safer iteration.
5. Is ArrayIndexOutOfBoundsException a checked or unchecked exception?
It is an unchecked exception, meaning you’re not required to explicitly catch or declare it. However, handling it improves code robustness.
6. Can other data structures also cause ArrayIndexOutOfBoundsException?
While arrays are common sources of this exception, other data structures like lists or strings can also lead to similar index-related errors if used improperly.
7. Is there a way to prevent ArrayIndexOutOfBoundsException at compile time?
At compile time, Java’s type system doesn’t provide direct mechanisms to prevent this exception. Proper validation and coding practices are essential to prevent it.
8. Can ArrayIndexOutOfBoundsException occur with multidimensional arrays?
Yes, it can occur in multidimensional arrays if you access elements using invalid indices for any of the dimensions.
9. Is it recommended to use negative indices for arrays?
No, Java arrays are zero-indexed, so negative indices are not valid and will result in ArrayIndexOutOfBoundsException. Always use non-negative indices.
10. Why is handling and preventing ArrayIndexOutOfBoundsException important?
Handling and preventing this exception enhances program stability and user experience. It prevents unexpected crashes and provides informative error messages, leading to more reliable software.
Conclusion: ArrayIndexOutOfBoundsException in Java
We’ve looked at the scenarios that cause the ArrayIndexOutOfBoundsException in Java , such as accessing negative indices, going beyond array bounds, and mismatches in loop boundaries. We’ve also looked into numerous approaches for gracefully handling exceptions with the try-catch block, as well as presenting meaningful error messages to users. Furthermore, we’ve learnt how to avoid the exception by checking indices meticulously, using proper loop conditions, and considering the use of enhanced for-each loops.