In this article, we will explore IOException in Java, causes of IOException, handling IOException and Best practices.
What is IOException in Java?
IOException is a common Checked Exception in Java. It is the superclass of all exceptions related to input and output operations, which are common when dealing with files, streams, and other types of I/O in Java.
Being a checked exception, IOException must either be caught using a try-catch
block or declared using the throws
keyword in the method signature.
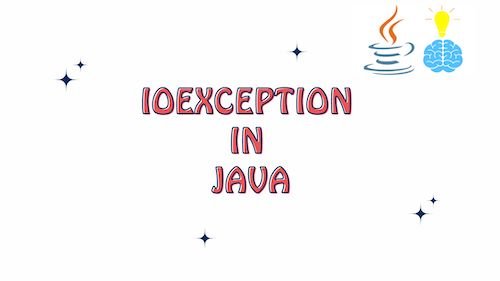
Causes of IOException in Java
Let’s go through a few examples of situations that can cause an IOException
in Java along with the corresponding code and outputs.
Example 1: File Not Found
import java.io.FileInputStream;
import java.io.IOException;
public class FileNotFoundExample {
public static void main(String[] args) {
try {
FileInputStream fileInputStream = new FileInputStream("nonexistent.txt");
} catch (IOException e) {
System.out.println("Caught IOException: " + e.getMessage());
}
}
}
Output
Caught IOException: nonexistent.txt (No such file or directory)
Example 2: Access Denied
import java.io.FileOutputStream;
import java.io.IOException;
public class AccessDeniedExample {
public static void main(String[] args) {
try {
FileOutputStream fileOutputStream = new FileOutputStream("/etc/system.properties");
} catch (IOException e) {
System.out.println("Caught IOException: " + e.getMessage());
}
}
}
Output
Caught IOException: /etc/system.properties (Permission denied)
Example 3: Connection Error (Socket)
import java.io.IOException;
import java.net.Socket;
public class SocketExample {
public static void main(String[] args) {
try {
Socket socket = new Socket("localhost", 12345);
} catch (IOException e) {
System.out.println("Caught IOException: " + e.getMessage());
}
}
}
Output
Caught IOException: Connection refused (Connection refused)
Handling IOException in Java
Here’s how you can handle IOException
in Java effectively:
Using try-catch
Blocks: Wrap the code that might throw an IOException
inside a try
block and catch the IOException
in a catch
block. This allows you to handle the exception gracefully without causing your program to crash.
try {
// Code that may cause IOException
FileInputStream fileInputStream = new FileInputStream("myfile.txt");
// ... other I/O operations ...
} catch (IOException e) {
// Handle the exception
System.err.println("An error occurred: " + e.getMessage());
}
Multiple catch
Blocks: If you’re dealing with different types of exceptions, you can use multiple catch
blocks to handle them differently. For example, you might handle a FileNotFoundException
differently from a general IOException
.
try {
// Code that may cause IOException
FileInputStream fileInputStream = new FileInputStream("myfile.txt");
// ... other I/O operations ...
} catch (FileNotFoundException e) {
System.err.println("File not found: " + e.getMessage());
} catch (IOException e) {
System.err.println("An error occurred: " + e.getMessage());
}
Finally Block: You can use a finally
block to include code that needs to be executed regardless of whether an exception occurred. This is often used for resource cleanup.
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream("myfile.txt");
// ... other I/O operations ...
} catch (IOException e) {
System.err.println("An error occurred: " + e.getMessage());
} finally {
if (fileInputStream != null) {
try {
fileInputStream.close();
} catch (IOException e) {
System.err.println("Error while closing file: " + e.getMessage());
}
}
}
Rethrowing Exceptions: Sometimes, you might want to catch an exception, perform some actions, and then rethrow the exception for higher-level error handling.
try {
// Code that may cause IOException
} catch (IOException e) {
System.err.println("An error occurred: " + e.getMessage());
throw e; // Rethrow the exception
}
throws
Clause: If a method can potentially cause an IOException
, you can declare it in the method signature using the throws
keyword. This indicates to the caller that they need to handle the exception.
public void readFile(String filePath) throws IOException {
// Code that may cause IOException
}
Best Practices: IOException in Java
Here are some best practices for working with IOException
in Java:
- Use Specific Exception Types: Whenever possible, catch more specific exceptions (subclasses of
IOException
) rather than catchingIOException
itself. This allows you to handle different types of errors more precisely. - Keep Catch Blocks Short: Keep the code within your
catch
blocks concise. If you need to perform complex error handling or cleanup, consider creating helper methods or using afinally
block. - Log Exceptions: Use a logging framework (e.g., Java’s built-in
java.util.logging
, Log4j, SLF4J) to log the exception details. Logging helps in debugging and understanding the root cause of issues. - Close Resources Properly: Ensure that any resources (streams, files, sockets) you open are properly closed, either within a
finally
block or by using the try-with-resources statement introduced in Java 7. - Graceful Degradation: Provide meaningful error messages to users when an
IOException
occurs. This helps users understand what went wrong and what actions they can take. - Retry Mechanism: Implement a retry mechanism for certain types of
IOException
, especially those that might be transient, like network-related issues. However, be cautious not to create infinite loops or excessively long retries. - Separate Concerns: Separate your I/O operations from the rest of your application logic. This can make error handling more focused and simplify your code.
- Use Buffered I/O: When performing read and write operations, consider using buffered streams (
BufferedReader
,BufferedWriter
) to improve performance and reduce the likelihood ofIOException
. - Check for Valid Input: Before performing I/O operations, validate inputs (e.g., filenames, URLs) to ensure they’re in the expected format and that the resources actually exist.
- Use Well-Defined Error Codes: If you’re building APIs or libraries, consider using well-defined error codes or custom exceptions that provide more context about the error to the caller.
Conclusion: IOException in Java
In this article, we explored IOException in Java in great details. We looked into various causes of IOException and discussed different ways to handle IOException. At last, we examined various best practices for handling IO operations.
Read More : List of Checked Exception in Java