In this article, we will look into various aspects of “Delete Query in SQL” using various examples. Let’s get started.
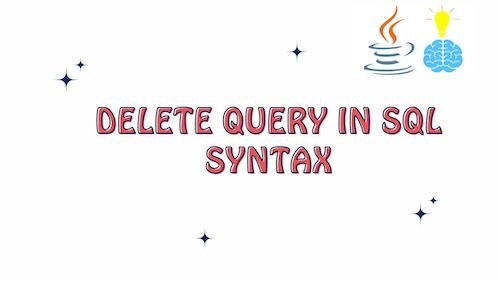
Delete Query in SQL Syntax
Below is the syntax for the DELETE query in SQL
DELETE FROM table_name
WHERE condition;
DELETE FROM table_name
: Specifies the table from which you want to delete rows.WHERE condition
: Optional. Specifies the condition that determines which rows to delete. If omitted, all rows in the table will be deleted.
Example:
Let’s assume you have a table named “employees” with the following structure:
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
department VARCHAR(50),
salary DECIMAL(10, 2)
);
You want to delete an employee with the employee_id
of 101 from this table. Here’s how you can do it with a DELETE query:
DELETE FROM employees
WHERE employee_id = 101;
In this example:
DELETE FROM employees
specifies that you want to delete rows from the “employees” table.WHERE employee_id = 101
is the condition that specifies you want to delete the row where the “employee_id” is equal to 101.
After executing this query, the employee with employee_id
101 will be removed from the “employees” table.
Deleting All Rows in a Table
The DELETE statement allows you to selectively delete rows based on conditions, but if you want to delete all rows, you can simply omit the WHERE clause.
DELETE FROM employees;
In this example:
DELETE FROM employees
specifies that you want to delete rows from the “employees” table.- The absence of a
WHERE
clause means that all rows in the table will be deleted.
After executing this SQL query, all the rows in the “employees” table will be removed, effectively emptying the table.
Please note that you can also use TRUNCATE statement : This removes all rows from a table quickly and efficiently. It’s faster than DELETE for large tables.
Example:
TRUNCATE TABLE table_name;
Deleting Rows from Multiple Tables
Deleting rows from multiple tables in SQL typically involves using JOINs and the DELETE statement in combination.
Here’s an example to illustrate how to delete rows from multiple related tables:
Suppose you have two tables, “orders” and “order_items,” and you want to delete all orders placed by a specific customer with a given customer ID.
DELETE o, oi
FROM orders o
INNER JOIN order_items oi ON o.order_id = oi.order_id
WHERE o.customer_id = 123;
In this example:
- We use an INNER JOIN to link the “orders” and “order_items” tables based on the “order_id” column.
- The WHERE clause specifies the condition for deleting rows related to a specific customer (customer_id = 123).
Deleting Rows with Subqueries
Deleting rows with subqueries in SQL can be a powerful way to remove specific data based on criteria from another table or subquery result. Here’s how you can do it with an example:
Suppose you have two tables, “employees” and “terminated_employees,” and you want to delete all employees from the “employees” table who are also listed in the “terminated_employees” table. The “terminated_employees” table contains a list of employee IDs for those who have been terminated.
Here’s the SQL query to achieve this using a subquery:
DELETE FROM employees
WHERE employee_id IN (SELECT employee_id FROM terminated_employees);
In this example:
- We use a subquery
(SELECT employee_id FROM terminated_employees)
to retrieve a list of employee IDs from the “terminated_employees” table. - The main DELETE statement removes all rows from the “employees” table where the “employee_id” matches any of the IDs obtained from the subquery.
- The
IN
operator is used to check if the “employee_id” in the “employees” table is in the list of terminated employees obtained from the subquery.
Executing this query will delete all rows from the “employees” table for employees who are also listed in the “terminated_employees” table.
Deleting Rows with LIMIT or TOP
In SQL, you can use the LIMIT (for databases like MySQL and PostgreSQL) or TOP (for databases like SQL Server) clauses to restrict the number of rows affected when deleting data from a table. These clauses allow you to control how many rows should be deleted, which can be useful when you want to delete a limited number of rows based on certain criteria. Below are examples for both LIMIT and TOP:
Using LIMIT (for MySQL and PostgreSQL)
Suppose you have a table named “orders” with columns “order_id” and “order_status,” and you want to delete only the first 10 rows where the “order_status” is ‘Cancelled’. You can use the LIMIT clause as follows:
DELETE FROM orders
WHERE order_status = 'Cancelled'
LIMIT 10;
In this SQL statement:
DELETE FROM orders
specifies that you want to delete rows from the “orders” table.WHERE order_status = 'Cancelled'
filters the rows based on the “order_status” column.LIMIT 10
restricts the deletion to the first 10 rows that meet the specified criteria.
Using TOP (for SQL Server)
If you’re working with SQL Server, you can achieve a similar result using the TOP clause. Suppose you have the same “orders” table and want to delete the first 10 rows where the “order_status” is ‘Cancelled’:
DELETE TOP (10) FROM orders
WHERE order_status = 'Cancelled';
In this SQL statement:
DELETE TOP (10) FROM orders
specifies that you want to delete the first 10 rows from the “orders” table.WHERE order_status = 'Cancelled'
filters the rows based on the “order_status” column.
Both of these queries will delete a limited number of rows based on the specified criteria. Be cautious when using these clauses, especially with large datasets, to avoid unintended data loss. Always make sure your WHERE clause accurately selects the rows you want to delete.
Deleting Rows with Foreign Key Constraints
Now, let’s consider a scenario where you have two tables: “orders” and “order_details.” The “order_details” table has a foreign key constraint that references the “order_id” column in the “orders” table. If you want to delete an order and its associated order details, you can use SQL like this:
-- First, delete the corresponding order details
DELETE FROM order_details
WHERE order_id = 456;
-- Then, delete the order itself
DELETE FROM orders
WHERE order_id = 456;
In this example:
- You start by deleting the related order details from the “order_details” table, where the “order_id” matches the order you want to delete.
- After removing the associated order details, you proceed to delete the actual order from the “orders” table. The foreign key constraint ensures that you can’t delete an order while there are related order details, so you must delete them first.
Best Practices for Using Delete Query in SQL
Using the DELETE query in SQL is a crucial operation for maintaining and managing data within a database. To ensure that your SQL DELETE statements are efficient, safe, and follow best practices, consider the following guidelines:
- Use the WHERE Clause Carefully:
- Always include a WHERE clause in your DELETE statement to specify which rows to delete. Failing to do so will result in deleting all rows in the table, which can be catastrophic.
- Avoid Deleting All Rows:
- Be cautious when using DELETE without a WHERE clause. If you need to delete all rows, consider using TRUNCATE TABLE for better performance (where applicable) or consider other means of data archiving.
- Backup Data:
- Before performing mass deletions, especially in a production environment, ensure you have a recent backup of your data. This precaution can help recover lost data in case of accidental deletions.
- Transaction Management:
- Wrap your DELETE statements within transactions, especially when dealing with complex or multi-table deletes. Transactions provide a way to commit or roll back changes as a single unit, ensuring data consistency.
- Use JOINs with Caution:
- When deleting rows from a table with JOINs, ensure you fully understand the impact of the JOIN. Test your DELETE statements thoroughly to avoid accidentally deleting more data than intended.
- Check for Indexes:
- Ensure that your table has appropriate indexes on columns frequently used in WHERE clauses. Indexes can significantly improve DELETE query performance.
- Review Execution Plan:
- Use tools like EXPLAIN (or equivalent in your database system) to analyze the execution plan of your DELETE statements. This can help identify potential performance bottlenecks.
- Avoid Subqueries When Possible:
- While subqueries can be used in DELETE statements, they can make queries complex and harder to optimize. Use them judiciously, and consider other approaches like JOINs when applicable.
- Consider Cascading Deletes:
- In some cases, you may want to configure cascading deletes, especially when dealing with foreign key relationships. Cascading deletes automatically delete related rows in child tables when a parent row is deleted.
- Permissions and Security:
- Ensure that only authorized users have DELETE privileges on tables. Avoid giving DELETE permissions to users who don’t need them.
Conclusion: Delete Query in SQL
This article has provided a comprehensive overview of the “Delete Query in SQL” with various examples and scenarios. Here are the key takeaways:
- SQL DELETE Syntax: The basic syntax of the DELETE query involves specifying the table from which you want to delete rows and using a WHERE clause to determine which rows to delete. Omitting the WHERE clause will result in deleting all rows from the table.
- Deleting Specific Rows: You can use the DELETE query to remove specific rows from a table based on defined conditions, such as deleting an employee with a particular ID.
- Deleting All Rows: To delete all rows in a table, you can use the DELETE query without a WHERE clause. Alternatively, you can consider using the TRUNCATE TABLE statement for better performance when applicable.
- Deleting Rows from Multiple Tables: Deleting rows from multiple related tables usually involves using JOINs along with the DELETE statement to ensure data integrity.
- Deleting Rows with Subqueries: Subqueries can be employed within DELETE statements to remove data based on criteria from another table or subquery result. This approach is useful for complex data deletion scenarios.
- Deleting Rows with LIMIT or TOP: You can restrict the number of rows affected by a DELETE query using the LIMIT (for MySQL and PostgreSQL) or TOP (for SQL Server) clauses. These clauses allow you to control the quantity of deleted rows.
- Deleting Rows with Foreign Key Constraints: When working with tables that have foreign key constraints, it’s important to delete related rows in the correct order to maintain referential integrity.
- Best Practices: Follow best practices for using DELETE queries, such as using the WHERE clause carefully, backing up data before deletions, using transactions, and considering the impact of JOINs and subqueries. Also, review execution plans, optimize queries, and ensure proper permissions and security.
Related Articles :